










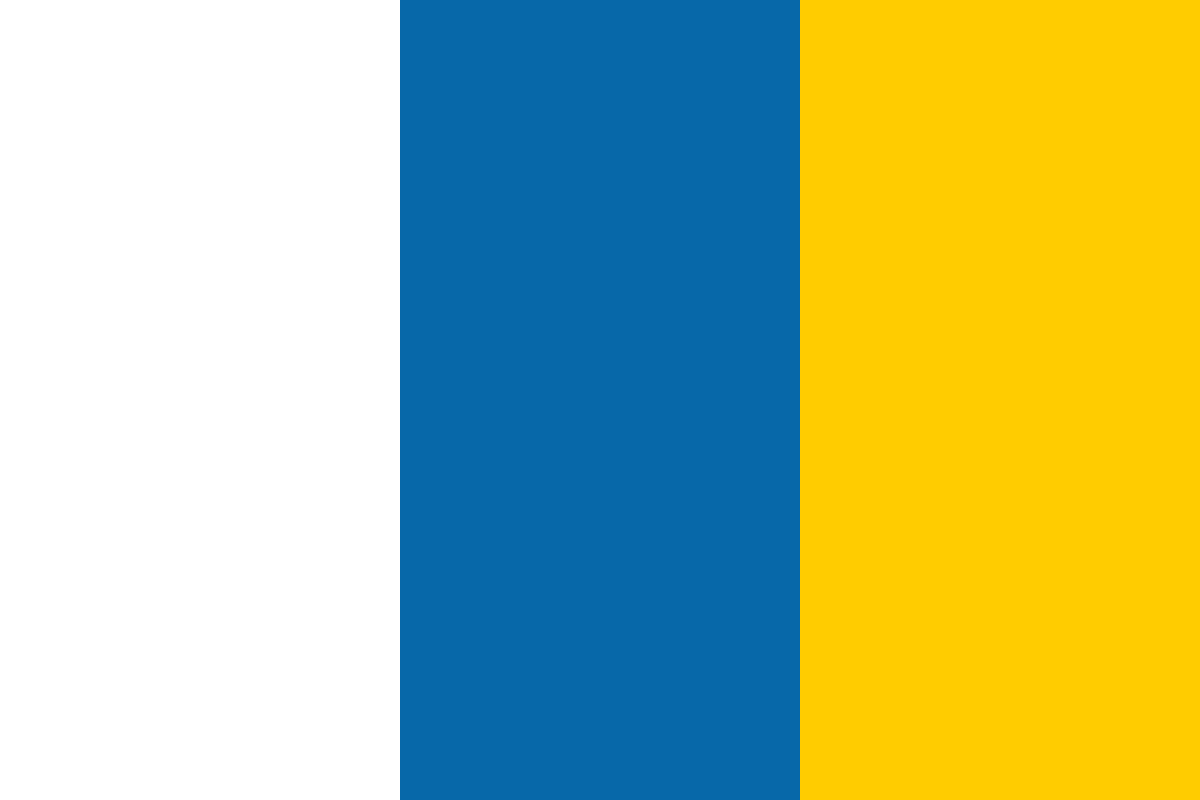

































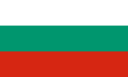








(For more resources related to this topic, see here.)
To create a new Java project, navigate to File | New | Project . You will be presented with the New Project wizard window that is shown in the following screenshot:
Choose the Java Project option, and click on Next . The next page of the wizard contains the basic configuration of the project that you will create. The JRE section allows you to use a specific JRE to compile and run your project. The Project layout section allows you to choose if both source and binary files are created in the project's root folder or if they are to be separated into different folders (src and bin by default). The latter is the default option.
You can create your project inside a working set. This is a good idea if you have too many projects in your workspace and want to keep them organized. Check the Creating working sets section of this article for more information on how to use and manage working sets.
The next page of the wizard contains build path options. In the Managing the project build path section of this article , we will talk more about these options. You can leave everything as the default for now, and make the necessary changes after the project is created.
To create a new Java class, right-click on the project in the Package Explorer view and navigate to New | Class . You will be presented with the New Java Class window, where you will input information about your class. You can change the class's superclass, and add interfaces that it implements, as well as add stubs for abstract methods inherited from interfaces and abstract superclasses, add constructors from superclasses, and add the main method.
To create your class inside a package, simply enter its name in the appropriate field, or click on the Browse button beside it and select the package. If you input a package name that doesn't exist, Eclipse will create it for you. New packages can also be created by right-clicking on the project in the Package Explorer and navigating to New | Package . Right-clicking on a package instead of a project in the Project Explorer and navigating to New | Class will cause the class to be created inside that package.
Working sets provide a way to organize your workspace's projects into subsets. When you have too many projects in your workspace, it gets hard to find the project you're looking for in the Package Explorer view. Projects you are not currently working on, for example, can be kept in separate working sets. They won't get in the way of your current work but will be there in case you need them.
To create a new working set, open the Package Explorer's view menu (white triangle in the top-right corner of the view), and choose Select Working Set . Click on New and select the type of projects that the working set will contain (Java , in this case). On the next page, insert the name of the working set, and choose which projects it will contain. Once the working set is created, choose the Selected Working Sets option, and mark your working set. Click on OK , and the Package Explorer will only display the projects inside the working set you've just created.
Once your working sets are created, they are listed in the Package Explorer's view menu. Selecting one of them will make it the only working set visible in the Package Explorer. To view more than one working set at once, choose the Select Working Set option and mark the ones you want to show. To view the whole workspace again, choose Deselect Workspace in the view menu. You can also view all the working sets with their nested projects by selecting working sets as the top-level element of the Package Explorer view. To do this, navigate to Top Level Elements | Working Sets in the view menu.
Although you don't see projects that belong to other working sets when a working set is selected, they are still loaded in your workspace, and therefore utilize resources of your machine. To avoid wasting these resources, you can close unrelated projects by right-clicking on them and selecting Close Project . You can select all the projects in a working set by using the Ctrl + A keyboard shortcut.
If you have a big number of projects, but you never work with all of them at the same time (personal/business projects, different clients' projects, and so on), you can also create a specific workspace for each project set. To create a new workspace, navigate to File | Switch Workspace | Other in the menu, enter the folder name of your new workspace, and click on OK . You can choose to copy the current workspace's layout and working sets in the Copy Settings section.
If you are going to work on an existing project, there are a number of different ways you can import it into Eclipse, depending on how you have obtained the project's source code. To open the Import wizard, navigate to File | Import . Let's go through the options under the General category:
Projects that are stored in Version Control Servers can be imported directly into Eclipse. There's a number of version control softwares, each with its pros and cons, and most of them are supported by Eclipse via plugins. GIT is one of the most used softwares for version control. CVS is the only version control system supported by default. To import a project managed by it, navigate to CVS | Projects from CVS in the Import wizard. Fill in the server information on the following page, and click on Finish .
Eclipse's user interface consists of elements called views. The following sections will introduce the main views related to Java development.
The Package Explorer view is the default view used to display a project's contents. As the name implies, it uses the package hierarchy of the project to display its classes, regardless of the actual file hierarchy. This view also displays the project's build path.
The following screenshot shows how the Package Explorer view looks:
The Java Editor is the Eclipse component that will be used to edit Java source files. It is the main view in the Java perspective and is located in the middle of the screen.
The following screenshot shows the Java Editor view:
The Java Editor is much more than an ordinary text editor. It contains a number of features that makes it easy for newcomers to start writing Java code and increases the productivity of experienced Java programmers. Let's talk about some of these features.
As you will see in the Building and running section with more details, Eclipse builds your code automatically after every saved modification by default. This allows Eclipse to get the Java Compiler output and mark errors and warnings through the code, making it easier to spot and correct them. Warnings are underlined in yellow and errors in red.
This is probably the most used Java Editor feature both by novice and experienced Java programmers. It allows you to list all the methods callable by a given instance, along with their documentation. This feature will work by default for all Java classes and for the ones in your workspace. To enable it for external libraries, you will have to configure the build path for your project. We'll talk more about build paths further in this article in the Managing the project build path section.
To see this feature in action, open a Java Editor, and create a new String instance.
String s = new String();
Now add a reference to this String instance, followed by a dot, and press Ctrl + Space bar . You will see a list of all the String() and Object() methods. This is way more practical than searching for the class's API in the Java documentation or memorizing it.
The following screenshot shows the content assist feature in action:
This list can be filtered by typing the beginning of the method's name after the dot. Let's suppose you want to replace some characters in this String instance. As a novice Java programmer, you are not sure if there's a method for that; and if there is, you are not sure which parameters it receives. It's a fair guess that the method's name probably starts with replace, right? So go ahead and type:
s.replace
When you press Ctrl along with the space bar, you will get a list of all the String() methods whose name starts with replace. By choosing one of them and pressing Enter , the editor completes the code with the rest of the method's name and its parameters. It will even suggest some variables in your code that you might want to use as parameters, as shown in the following screenshot:
Content assist will work with all classes in the project's classpath. You can disable content assist's automatic activation by unmarking Enable auto activation inside the Preferences window and navigating to Java | Editor | Content Assist .
When the project you are working on is big enough, finding a class in the Package Explorer can be a pain. You will frequently find yourself asking, "In which package is that class again?". You can leave the source code of the classes you are working on open in different tabs, but soon enough you will have more open tabs than you would like to have.
Eclipse has an easy solution for this. In the toolbar, select Navigate | Open Type . Now, just type in the class's name, and click on OK . If you don't remember the full name of the class, you can use the wildcard characters, ? (matches one character) and * (matches any number of characters). You can also use only the uppercase letters for the CamelCase names (for example, SIOOBE for StringIndexOutOfBoundsException). The shortcut for the Open Type dialog is Ctrl + Shift + T . There's also an equivalent feature for finding and opening resources other than Java classes, such as HTML files, images, and plain text files. The shortcut for the Open Resource dialog is Ctrl + Shift + R .
You can also navigate to a class' source file by holding Ctrl and clicking on a reference to that class in the code. To navigate to a method's implementation or definition directly, hold Ctrl and click on the method's call.
Another useful feature that makes it easy to browse through your project's source files is the Link With Editor feature in the Package Explorer view, as shown in the following screenshot:
By enabling it, the selected resource in the Package Explorer will always be the one that's open in the editor. Using this feature together with OpenType is certainly the easiest way of finding a resource in the Package Explorer.
Whenever there's an error or warning marker in your code, Eclipse might have some suggestions on how to get rid of it. To open the Quick Fix menu containing the suggestions, place the caret on the marked piece of code related to the error or warning, right-click on it, and choose Quick Fix . You can also use the shortcut by pressing Ctrl + 1 with the caret placed on the marked piece of code.
The following screenshot shows the quick fix feature suggesting you to either get rid of the unused variable, create getters and setters for it, or add a SuppressWarnings annotation:
Let's see some of the most used quick fixes provided by Eclipse. You can take advantage of these quick fixes to speed up your code writing. You can for example, deliberately call a method that throws an exception without the try/catch block, and use the quick fix to generate it instead of writing the try/catch block yourself.
int b = 4;
int c = 5;
int a = performOperation(b,c);
The method call will be marked with an error that says performOperation is undefined. To create a stub for this method, place the caret over the method's name, open the Quick Fix menu, and choose create method performOperation(int, int) . A private method will be created with the correct parameters and return type as well as a TODO marker inside it, reminding you that you have to implement the method. You can also use a quick fix to create methods in other classes. Using the same previous example, you can create the performOperation() method in a different class, such as the following:
OperationPerformer op = new OperationPerformer(); int a = op.performOperation(b,c);
Speaking of classes, quick fix can also create one if you add a call to a non-existing class constructor.
Non-existing variables can also be created with quick fix. Like with the method creation, just refer to a variable that still doesn't exist, place the caret over it, and open the Quick Fix menu. You can create the variable either as a local variable, a field, or a parameter.
Like almost everything in Eclipse, you can customize the Java Editor's appearance and behavior. There are plenty of configurations in the Preferences window (Window | Preferences ) that will certainly allow you to tailor the editor to suit your needs. Appearance-related configurations are mostly found in General | Appearance | Colors and Fonts and behavior and feature configurations are mostly under General | Editors | Text Editors . Since there are lots of different categories and configurations, the filter text in the Preferences window might help you find what you want. A short list of the preferences you will most likely want to change is as follows: