










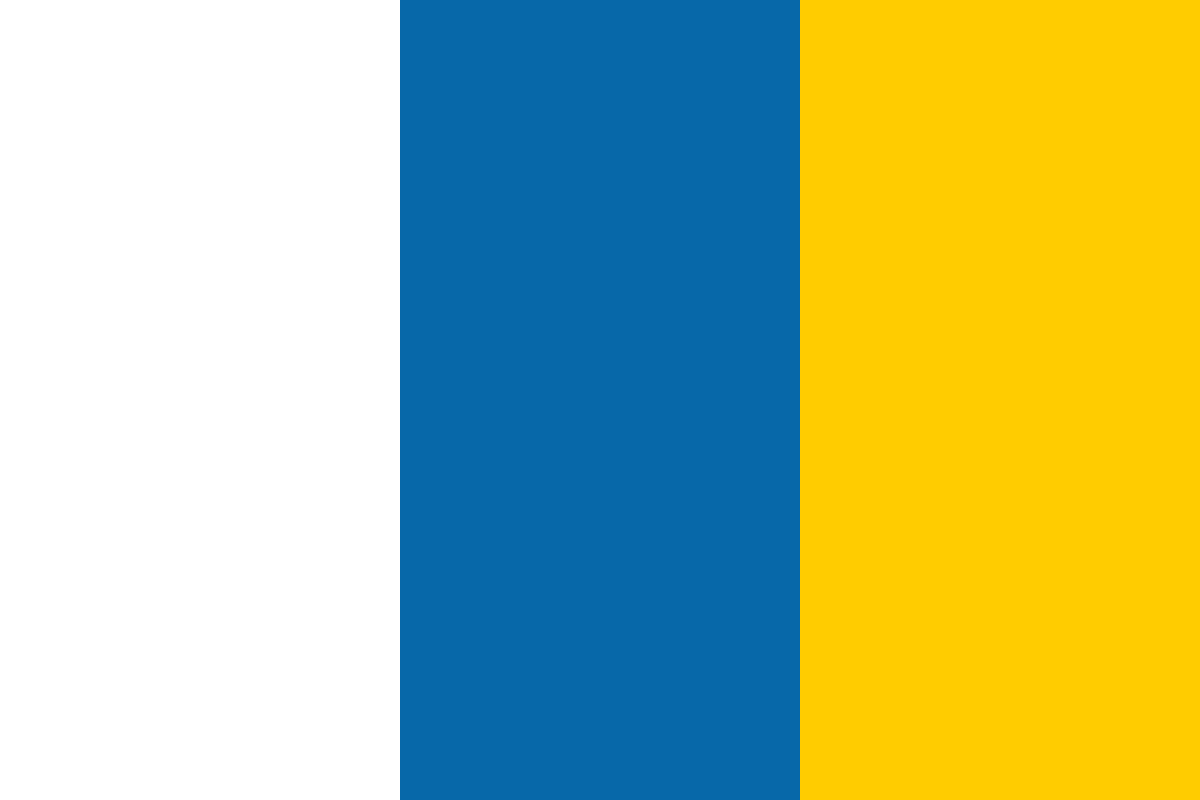

































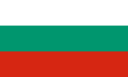








Read more about this book |
(For more resources related to this subject, see here.)
At the time of publication, working with contacts requires us to develop native applications. But we can use JavaScript with the PhoneGap framework for building these kinds of applications for the iPhone. This is the main reason for applying this framework for the recipes included in this article.
This article focuses on issues related to calls, SMS, and contacts. We'll learn how to handle contacts and how to send an SMS or place a phone call simply by interacting with the user interface.
In this recipe, you'll learn how to call a number when a user clicks on a button in the user interface. Specifically, we're going to build a simple list containing our contacts where each represents a person and their phone number. After clicking on one of these elements the dial screen will be opened and the user will only need to click on the green button for calling the specified number.
We only need to build a simple XHTML file for this recipe. It can be found at code/ch08/call.html in the code bundle provided on the Packtpub site.
Code files can be downloaded at the Packt website.
This recipe requires the use of the iWebKit framework.
Open your favorite text editor or IDE and create a new file called call.html.
<link href="../iwebkit/css/style.css" rel="stylesheet
media="screen" type="text/css" />
<script src="../iwebkit/javascript/functions.js"
type="text/javascript"></script>
<body class="list">
<div id="topbar">
<div id="title">Call</div>
</div>
<div id="content">
<ul>
<li class="title">Contacts</li>
<li><a class="noeffect" href="tel:555-666-777">
<span class="name">Aaron Stone</span></a></li>
<li><a class="noeffect" href="tel:555-888-999">
<span class="name">Ben Jackson</span></a></li>
<li><a class="noeffect" href="tel:555-222-333">
<span class="name">Bob McKenzie</span></a></li>
<li><a class="noeffect" href="tel:555-444-666">
<span class="name">Luke Johnson</span></a></li>
<li><a class="noeffect" href="tel:555-333-666">
<span class="name">Michael Sterling</span></a></li>
</ul>
</div>
The most important part of the code in this recipe is the anchor element inside each <li> tag. The href attribute of the anchor element is using a string, which represents a specific protocol followed by a number. In fact, tel identifies the protocol and the iPhone, understanding its meaning displays the dial screen allowing the user to call a number. On the other hand, the class noeffect was applied to each anchor for opening the dial screen in fullscreen mode.
From a strict point of view, iWebkit is not a must for calling numbers. Safari Mobile indentifies the tel protocol by default. However, we used the mentioned framework because it helps us to easily construct a list with many items.
What happens if you are using an iOS device other than the iPhone? It is not possible to call numbers from an iPad or iPod touch as of now. The code developed for this recipe works differently in these devices. Instead of calling numbers, iPad and iPod touch will ask you if you want to save numbers in the address book.
The previous recipe explained how you can call a number from your applications. This recipe will show you how to send an SMS to a selected number. For simplicity we're going to use the same approach. We'll develop a simple XHTML file displaying a list with different contacts. When the user clicks on one of the contacts the iPhone will display the screen for sending an SMS.
As in the previous recipe we will use the iWebKit framework. As both recipes are similar, we're going to use the same XHTML file developed for the previous recipe.
<li>
<a class="noeffect" href="sms:555-666-777">
<span class="name">Aaron Stone</span>
</a>
</li>
As you've learned in the previous recipe, Safari Mobile identifies the tel protocol for calling numbers. In the same way, sms is used for sending SMS's. Also for this recipe the iWebKit is used for building the user interface.
Thanks to this recipe we'll learn how to select a contact from the address book of the iPhone and to display its related information. Our goal is quite simple; when the user clicks on a button, a new screen will display all available contacts. After clicking on one of these elements, the complete name of the selected contact will be displayed in the main screen.
Although this recipe is very simple, you can apply it for building complex applications that require dealing with contacts available through the address book of the iPhone.
The complete code for this recipe can be reached at code/ch08/queryAddress in the code bundle provided on the Packtpub site.
For this recipe we'll use three different frameworks: PhoneGap, XUI, and UiUIKit. Before continuing, make sure you have these frameworks installed on your machine. Also, remember you'll need a Mac OS X computer with the iOS SDK and Xcode installed.
<script type="text/javascript" src="xui-2.0.0..min.js"></script>
<link rel="stylesheet" href="uiuikit/stylesheets/iphone.css" />
<style type="text/css">
#mybtn {
margin-right: 12px;
}
</style>
function searchContact() {
navigator.contacts.chooseContact(onSucessContact);
}
function onSucessContact(contact) {
var selectedContact = contact.name;
x$('#ul_contacts').html( 'inner', "<li>" + selectedContact +
"</li>");
}
<div id="header">
<h1>Contacts</h1>
</div>
<h1>Working with contacts</h1>
<p id="p_btn">
<a href="#" id="mybtn" onclick="searchContact()"
class="button white">Select</a>
</p>
<ul id='ul_contacts'></ul>
Before continuing and after applying all changes inside the code, we need to copy some files to the www directory of our project. Specifically, we're going to copy the uiuikit directory and the xui-2.0.0.min.js file. Remember, these components belong to the UiUIKit and XUI framework respectively.
Save your project and click on the Build and Run button of Xcode for testing your new application. After loading the application in the iPhone Simulator, you can see a screen similar to next screenshot:
When the user clicks on the button Select, a list with all the available contacts will be displayed as shown in the following screenshot:
After selecting one of the items showed in the list, our application will display the data for the selected contact:
PhoneGap provides a JavaScript function for accessing the predefined screen of the iPhone for selecting contacts. The name of this function is ChooseContact() and it is invoked through the navigator.contacts predefined object. It encapsulates the access to the address book of the iPhone. As a parameter, this function requires a callback method that will be invoked when a contact is selected. In our case, we implemented a function called onSuccessContact(), which captures the complete name of the contact displaying the result in a simple list. onSuccesContact uses a parameter called contact, which represents an object containing the information related to the selected contact from the address book.
The XUI framework allows us to add new items to our ul list by manipulating the DOM of the HTML page. On the other hand, the UiUIKit framework was used for building the user interface for our small application.
We used a bit of CSS for centering our button. Actually, we modified the right margin of this widget. The CSS style for this appears before the JavaScript code, also inside the head section.