










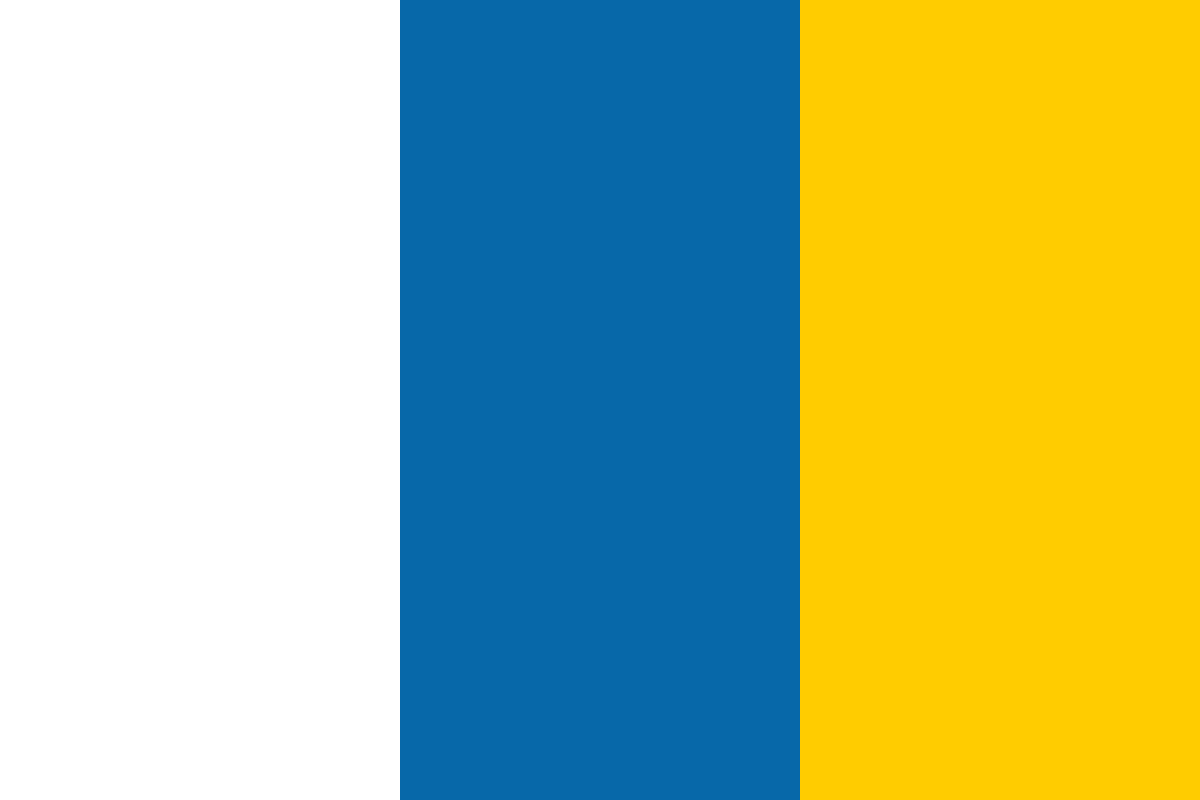

































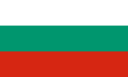








In this article by Chetan Giridhar, author of the book, Learning Python Design Patterns - Second Edition, we will get introduced to the Façade design pattern and how it is used in software application development. We will work with a sample use case and implement it in Python v3.5.
In brief, we will cover the following topics in this article:
(For more resources related to this topic, see here.)
Façade is generally referred to as the face of the building, especially an attractive one. It can be also referred to as a behavior or appearance that gives a false idea of someone's true feelings or situation. When people walk past a façade, they can appreciate the exterior face but aren't aware of the complexities of the structure within. This is how a façade pattern is used. Façade hides the complexities of the internal system and provides an interface to the client that can access the system in a very simplified way.
Consider an example of a storekeeper. Now, when you, as a customer, visit a store to buy certain items, you're not aware of the layout of the store. You typically approach the storekeeper who is well aware of the store system. Based on your requirements, the storekeeper picks up items and hands them over to you. Isn't this easy? The customer need not know how the store looks and s/he gets the stuff done through a simple interface, the storekeeper.
The Façade design pattern essentially does the following:
We will now discuss the Façade pattern with the help of the following UML diagram:
As we observe the UML diagram, you'll realize that there are three main participants in this pattern:
You will now learn a little more about the three main participants from the data structure's perspective.
The following points will give us a better idea of Façade:
For example, if the client is looking for some work to be accomplished, it need not have to go to individual subsystems but can simply contact the interface (Façade) that gets the work done
In the Façade world, System is an entity that performs the following:
For instance, when the client requests the Façade for a certain service, Façade chooses the right subsystem that delivers the service based on the type of service
Here's how we can describe the client:
To demonstrate the applications of the Façade pattern, let's take an example that we'd have experienced in our lifetime.
Consider that you have a marriage in your family and you are in charge of all the arrangements. Whoa! That's a tough job on your hands. You have to book a hotel or place for marriage, talk to a caterer for food arrangements, organize a florist for all the decorations, and finally handle the musical arrangements expected for the event.
In yesteryears, you'd have done all this by yourself, such as talking to the relevant folks, coordinating with them, negotiating on the pricing, but now life is simpler. You go and talk to an event manager who handles this for you. S/he will make sure that they talk to the individual service providers and get the best deal for you.
From the Façade pattern perspective we will have the following three main participants:
Let's develop an application in Python v3.5 and implement this use case. We start with the client first. It's you! Remember, you're the one who has been given the responsibility to make sure that the marriage preparations are done and the event goes fine!
However, you're being clever here and passing on the responsibility to the event manager, isn't it? Let's now look at the You class. In this example, you create an object of the EventManager class so that the manager can work with the relevant folks on marriage preparations while you relax.
class You(object):
def __init__(self):
print("You:: Whoa! Marriage Arrangements??!!!")
def askEventManager(self):
print("You:: Let's Contact the Event Manager\n\n")
em = EventManager()
em.arrange()
def __del__(self):
print("You:: Thanks to Event Manager, all preparations done! Phew!")
Let's now move ahead and talk about the Façade class. As discussed earlier, the Façade class simplifies the interface for the client. In this case, EventManager acts as a façade and simplifies the work for You. Façade talks to the subsystems and does all the booking and preparations for the marriage on your behalf. Here is the Python code for the EventManager class:
class EventManager(object):
def __init__(self):
print("Event Manager:: Let me talk to the folks\n")
def arrange(self):
self.hotelier = Hotelier()
self.hotelier.bookHotel()
self.florist = Florist()
self.florist.setFlowerRequirements()
self.caterer = Caterer()
self.caterer.setCuisine()
self.musician = Musician()
self.musician.setMusicType()
Now that we're done with the Façade and client, let's dive into the subsystems. We have developed the following classes for this scenario:
class Hotelier(object):
def __init__(self):
print("Arranging the Hotel for Marriage? --")
def __isAvailable(self):
print("Is the Hotel free for the event on given day?")
return True
def bookHotel(self):
if self.__isAvailable():
print("Registered the Booking\n\n")
class Florist(object):
def __init__(self):
print("Flower Decorations for the Event? --")
def setFlowerRequirements(self):
print("Carnations, Roses and Lilies would be used for Decorations\n\n")
class Caterer(object):
def __init__(self):
print("Food Arrangements for the Event --")
def setCuisine(self):
print("Chinese & Continental Cuisine to be served\n\n")
class Musician(object):
def __init__(self):
print("Musical Arrangements for the Marriage --")
def setMusicType(self):
print("Jazz and Classical will be played\n\n")
you = You()
you.askEventManager()
The output of the preceding code is given here:
In the preceding code example:
As you have learned in the initial parts of this article, the Façade provides a unified system that makes subsystems easy to use. It also decouples the client from the subsystem of components. The design principle that is employed behind the Façade pattern is the principle of least knowledge.
The principle of least knowledge guides us to reduce the interactions between objects to just a few friends that are close enough to you. In real terms, it means the following::
We began the article by first understanding the Façade design pattern and the context in which it's used. We understood the basis of Façade and how it is effectively used in software architecture. We looked at how Façade design patterns create a simplified interface for clients to use. It simplifies the complexity of subsystems so that the client benefits.
The Façade doesn't encapsulate the subsystem and the client is free to access the subsystems even without going through the Façade. You also learned the pattern with a UML diagram and sample code implementation in Python v3.5. We understood the principle of least knowledge and how its philosophy governs the Façade design patterns.
Further resources on this subject: