










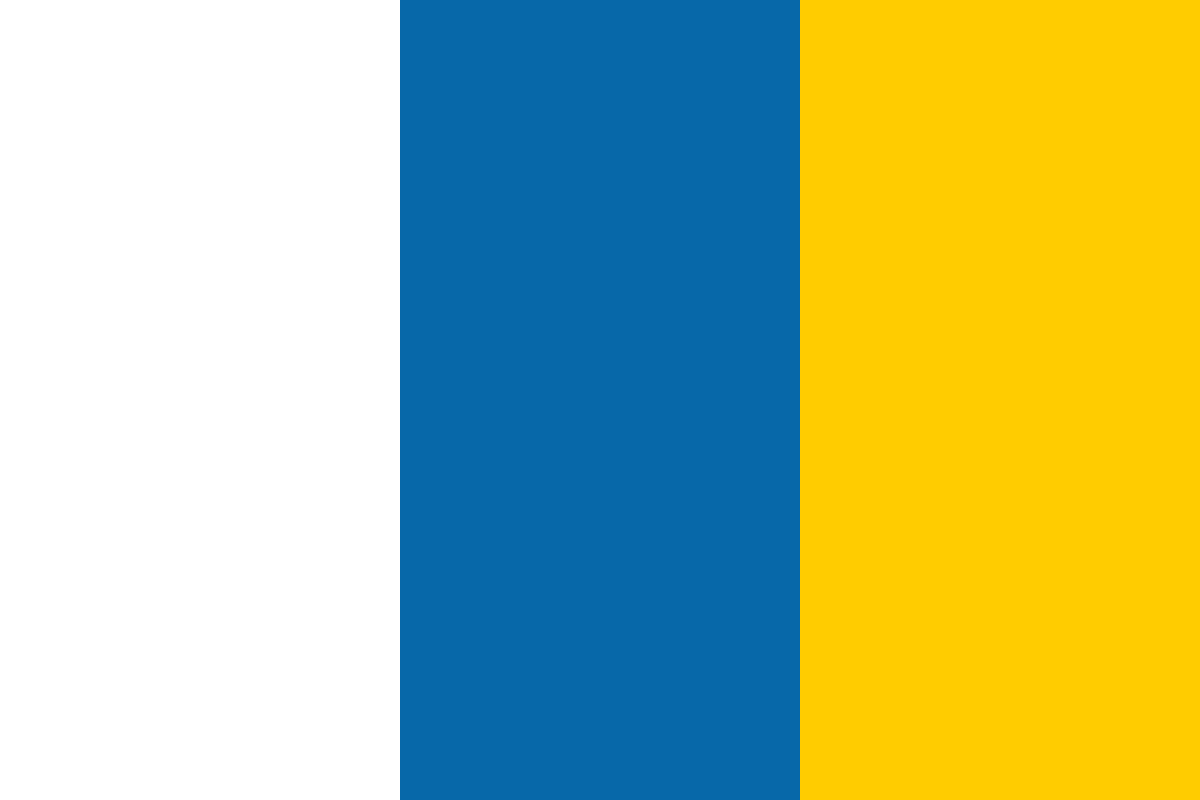

































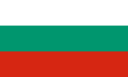








(For more resources on iPhone Development, see here.)
There is a lot of fun stuff to cover, so let's get started.
If you are running Mac OSX 10.5, chances are your machine is already running Xcode. These are located within the /Developer/Applications folder. Apple also makes this freely available through the Apple Developer Connection at http://developer.apple.com/.
The iPhone SDK includes a suite of development tools to assist you with your development of your iPhone, and other iOS device applications. We describe these in the following table.
COMPONENT | DESCRIPTION |
Xcode | This is the main Integrated Development Environment (IDE) that enables you to manage, edit, and debug your projects. |
DashCode | This enables you to develop web-based iPhone and iPad applications, and Dashboard widgets. |
iPhone Simulator | The iPhone Simulator is a Cocoa-based application, which provides a software simulator to simulate an iPhone or iPad on your Mac OSX. |
Interface Builder |
|
Instruments | These are the Analysis tools, which help you optimize your applications and monitor for memory leaks in real-time. |
The Xcode tools require an Intel-based Mac running Mac OS X version 10.6.4 or later in order to function correctly.
Xcode 4 is a complete toolset for building Mac OSX (Cocoa-Based) and iOS applications. The new single-windowed development interface has been redesigned to be a lot easier and even more helpful to use than it has been in previous releases. It can now also identify mistakes in both syntax and logical errors, and will even fix your code for you.
It provides you with the tools to enable you to speed up your development process, therefore becoming more productive. It also takes care of the deployment of both your Mac OSX and iOS applications.
The Integrated Development Interface (IDE) allows you to do the following:
Xcode incorporates many new features and improvements, apart from the redesigned user interface; it features a new and improved LLVM (Low Level Virtual Machine) debugger, which has been supercharged to run 3 times faster and 2.5 times more efficient.
This new compiler is the next generation compiler technology designed for high-performance projects and completely supports C, Objective-c, and now C++. It is also incorporated into the Xcode IDE and compiles twice as fast and quickly as GCC and your applications will run faster.
The following list includes the many improvements made to this release.
Xcode allows you to customize an unlimited number of build and debugging tools, and executable packaging. It supports several source-code management tools, namely, CVS "Version control software which is an important component of the Source Configuration Management (SCM)" and Subversion, which allows you to add files to a repository, commit changes, get updated versions and compare versions using the Version Editor tool.
The iPhone Simulator is a very useful tool that enables you to test your applications without using your actual device, whether this being your iPhone or any other iOS device. You do not need to launch this application manually, as this is done when you Build and run your application within the Xcode Integrated Development Environment (IDE). Xcode installs your application on the iPhone Simulator for you automatically.
The iPhone Simulator also has the capability of simulating different versions of the iPhone OS, and this can become extremely useful if your application needs to be installed on different iOS platforms, as well as testing and debugging errors reported in your application when run under different versions of the iOS.
While the iPhone Simulator acts as a good test bed for your applications, it is recommended to test your application on the actual device, rather than relying on the iPhone Simulator for testing. The iPhone Simulator can be found at the following location /Developer/Platforms/iPhoneSimulator.Platform/Developer/Applications.
According to Apple, they describe the set of frameworks and technologies that are currently implemented within the iOS operating system as a series of layers. Each of these layers is made up of a variety of different frameworks that can be used and incorporated into your applications.
Layers of the iOS Architecture
We shall now go into detail and explain each of the different layers of the iOS Architecture; this will give you a better understanding of what is covered within each of the Core layers.
This is the bottom layer of the hierarchy and is responsible for the foundation of the Operating system, which the other layers sit on top of. This important layer is in charge of managing memory - allocating and releasing of memory once it has finished with it, taking care of file system tasks, handles networking, and other Operating System tasks. It also interacts directly with the hardware.
The Core OS Layer consists of the following components:
COMPONENT NAME | COMPONENT NAME |
OS X Kernel | Mach 3.0 |
BSD | Sockets |
Security | Power Management |
Keychain | Certificates |
File System | Bonjour |
The Core Services layer provides an abstraction over the services provided in the Core OS layer. It provides fundamental access to the iPhone OS services. The Core Services Layer consists of the following components:
COMPONENT NAME | COMPONENT NAME |
Collections | Address Book |
Networking | File Access |
SQLite | Core Location |
Net Services | Threading |
Preferences | URL Utilities |
The Media Layer provides Multimedia services that you can use within your iPhone, and other iOS devices. The Media Layer is made up of the following components:
COMPONENT NAME | COMPONENT NAME |
Core Audio | OpenGL |
Audio Mixing | Audio Recording |
Video Playback | Image Formats: JPG, PNG and TIFF |
Quartz | |
Core Animations | OpenGL ES |
The Cocoa-Touch layer provides an abstraction layer to expose the various libraries for programming the iPhone, and other IOS devices. You probably can understand why Cocoa-Touch is located at the top of the hierarchy due to its support for Multi-Touch capabilities. The Cocoa-Touch Layer is made up of the following components:
COMPONENT NAME | COMPONENT NAME |
Cocoa-Touch Layer | Multi-Touch Events |
Multi-Touch Controls | Accelerometer/Gyroscope |
View Hierarchy | Localization/Geographical |
Alerts | Web Views |
People Picker | Image Picker |
Controllers |
Cocoa is defined as the development framework used for the development of most native Mac OSX applications. A good example of a Cocoa related application is Mail or Text Edit.
This framework consists of a collection of shared object code libraries known as the Cocoa frameworks. It consists of a runtime system and a development environment. These set of frameworks provide you with a consistent and optimized set of prebuilt code modules that will speed up your development process.
Cocoa provides you with a rich-layer of functionality, as well as a comprehensive object-oriented like structure and APIs on which you can build your applications. Cocoa uses the Model-View-Controller (MVC) design pattern.
Design Patterns represent and handle specific solutions to problems that arise when developing software within a particular context. These can be either a description or a template, on how to go about to solve a problem in a variety of different situations.
Cocoa-Touch is the programming language framework that drives user interaction on iOS. It consists and uses technology derived from the cocoa framework and was redesigned to handle multi-touch capabilities. The power of the iPhone and its User Interface are available to developers throughout the Cocoa-Touch frameworks.
Cocoa-Touch is built upon the Model-View-Controller structure; it provides a solid stable foundation for creating mind blowing applications. Using the Interface builder developer tool, developers will find it both very easy and fun to use the new drag-and-drop method when designing their next great masterpiece application on iOS.
The Model-View-Controller (or MVC) comprises a logical way of dividing up the code that makes up the GUI (Graphical User Interface) of an application. Object-Oriented applications like Java and .Net have adopted the MVC design pattern.
The MVC model comprises three distinctive categories:Model : This part defines your application's underlying data engine. It is responsible for maintaining the integrity of that data.