










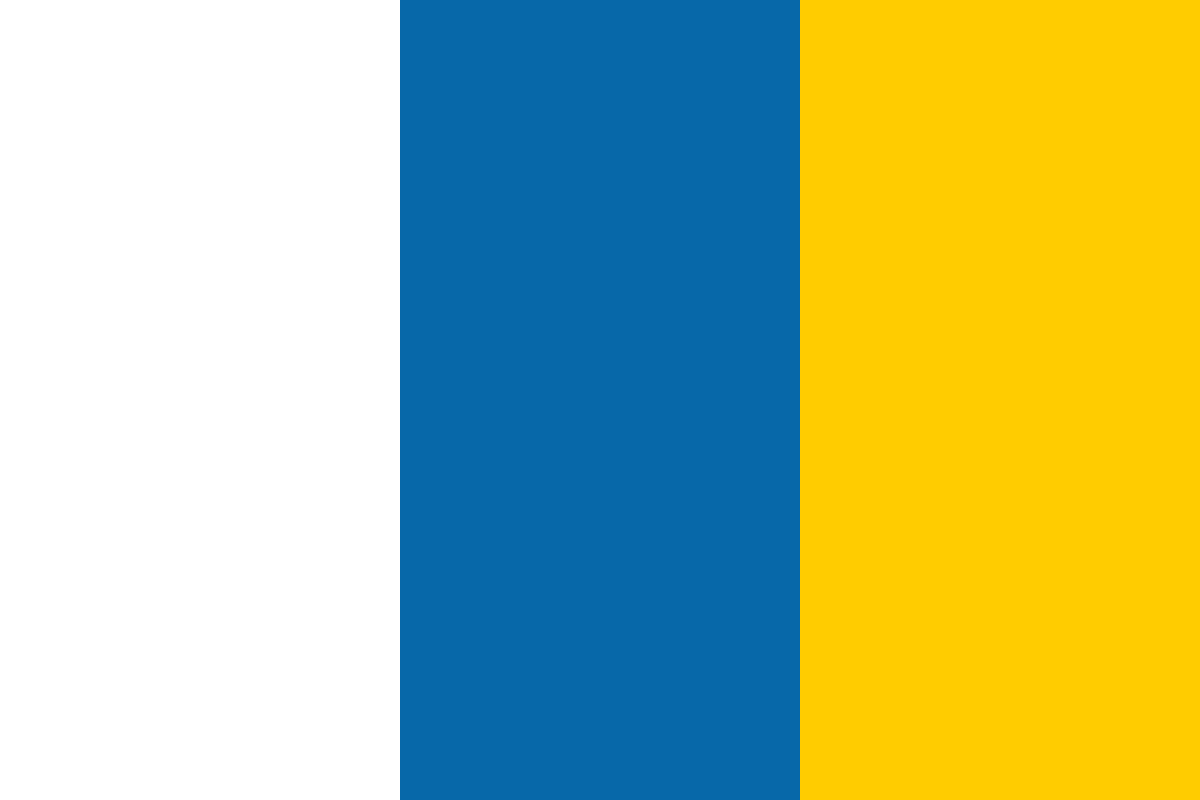

































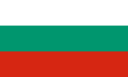








Over 50 recipes for making your Moodle system more dynamic and responsive with JavaScript
In this article, we will cover:
There are a lot of common tasks that need to be performed when writing JavaScript. A large proportion of this simply involves dealing with differences between web browsers. The need for a way to hide or abstract the specifics of each browser into a standard interface gave rise to sets of tools known as JavaScript libraries. One of the leading libraries in use on the web today is the Yahoo! User Interface Library (YUI).
Moodle includes a copy of the YUI as its preferred JavaScript library. YUI provides developers with access to a wide range of tools for enhancing their web applications:
The YUI Library is a set of utilities and controls, written with JavaScript and CSS, for building richly interactive web applications using techniques such as DOM scripting, DHTML and AJAX. YUI is available under a BSD license and is free for all uses. YUI is proven, scalable, fast, and robust. Built by frontend engineers at Yahoo! and contributors from around the world, it's an industrial-strength JavaScript library for professionals who love JavaScript.
Yahoo! Developer Network
http://developer.yahoo.com/yui/
In this article, we will learn the basics of working with YUI. We will learn how to initialize the YUI and make it ready for use within our code and load additional modules from versions 2 and 3 of the YUI. We will also learn how to manage the execution of code by attaching events to our controls, and finally how to debug our code with YUI logging tools.
In this recipe, we will learn how to initialize the YUI 3 environment within Moodle, which will get us ready to start using YUI 3 features. Moodle takes care of most of the initial setup, namely loading the required CSS and JavaScript files, so all we need to be concerned with is activating the YUI environment.
This example will show how to execute JavaScript code from within the YUI environment. We will set up a small YUI script which will simply display a message including the version number of the active YUI environment in a JavaScript alert box.
This provides a simple view of what is required to get YUI up and running that we will build on further in the subsequent recipes.
We begin by setting up a new PHP file yui_init.php in the cook directory with the following content:
<?php
require_once(dirname(__FILE__) . '/../config.php');
$PAGE->set_context(get_context_instance(CONTEXT_SYSTEM));
$PAGE->set_url('/cook/yui_init.php');
$PAGE->requires->js('/cook/yui_init.js');
echo $OUTPUT->header();
echo $OUTPUT->footer();
?>
Notice that the preceding code references a JavaScript file yui_init.js, which has the following content:
YUI().use
(
function(Y)
{
alert('Hello from YUI ' + Y.version);
}
);
We have created a PHP file that sets up a Moodle programming environment and includes a JavaScript file, in a way now familiar to us from previous recipes.
What is new here is the content of the JavaScript file, which is where we will make ready the YUI 3 environment.
Moodle has already included all the JavaScript files required for YUI (this happened when we output the value returned from $OUTPUT->header();). This means we now have a global object named YUI available within our JavaScript code.
We create a new instance of the YUI object with the statement YUI() and then immediately call the use method.
The only parameter we will pass to the use method is an anonymous function. This is just like a regular JavaScript function, except that it has no name specified; hence it is "anonymous". A name is not required, as it is not referred to again, but it is simply passed directly to the use method. This function itself accepts a single input parameter Y, which will be a reference to the new instance of the YUI object. (Note that the use method is also used to load additional YUI modules; this is the subject of the next recipe.)
The anonymous function just created is the most important part of the code to take note of as this is where we will be putting our entire code that will be making use of the YUI features. In this example, you can see that we are just creating a JavaScript alert with a short message including the value of Y.version, which is simply a string containing the version number of YUI that has been loaded as seen in the following screenshot:
Here, we can see that our code has successfully initialized the YUI 3.2.0 environment and is ready for us to start using the features available within the YUI and its additional modules.
We have created a new instance of the global object YUI, and called the use method, passing in an anonymous function that contains the code we wish to run. When the new instance of the YUI object is fully loaded, it makes a call to our anonymous function, and our code is executed.
In this example, our code displays a short message containing the version number of the YUI instance we created, confirming that we have a fully functional YUI 3 environment as a basis to implement further YUI features.
YUI has a whole host of additional modules providing a very wide range of functionalities. Some examples of commonly used functionalities provided by additional modules include:
For a current list of all the modules available, please refer to the Yahoo! Developer Network website for YUI 3 at the URL: http://developer.yahoo.com/yui/3/
The loading of additional modules is achieved via the use method of the YUI object. In the previous recipe we learned how to run code via the use method with the following syntax:
YUI().use
( function(Y) { /* <code to execute> */ } );
Note that the use method takes an arbitrarily long number of arguments (one or more) and only the last argument must be the anonymous function described in the previous recipe. The preceding arguments are a list of one or more modules you wish to load. So for example, to load the Animation module (anim) and the DOM Event Utility module (event), we would use the following syntax in place of the preceding one:
YUI().use
( "anim", "event", function(Y) { /* <code to execute> */ } );
Now all of the features of these two additional modules (anim and event) will be available within the anonymous function that contains the code we want to execute.
This technique will be used to load the modules we require in the examples contained in the subsequent recipes.