










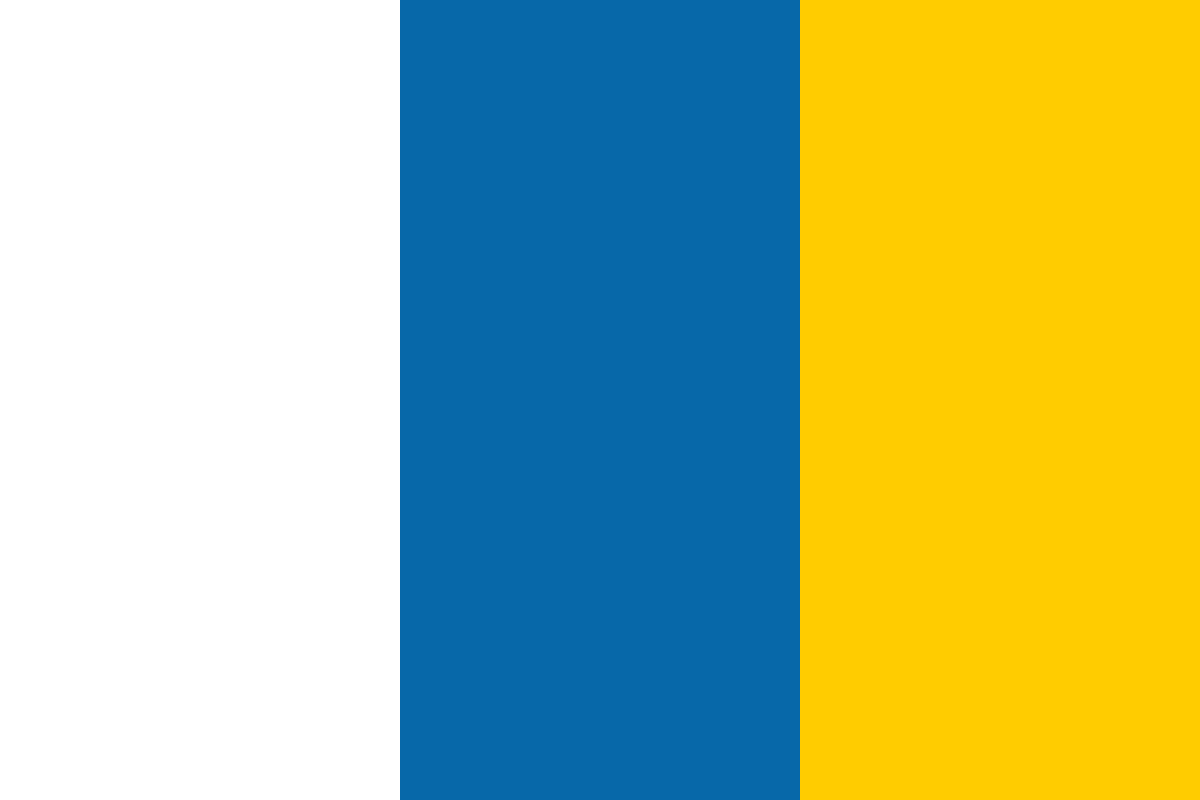

































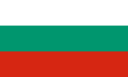








(For more resources related to this topic, see here.)
JavaScript has become a formidable language in its own right. Google's work on the V8 JavaScript engine has created something very performant, and has enabled others to develop Node.js, and with it, allow the development of JavaScript on the serverside. This article will take a look at how we can serve data using NodeJS, specifically using a framework known as express.
We will need to set up a simple project before we can get started:
{
"name": "highcharts-cookbook-nodejs",
"description": "An example application for using highcharts
with nodejs",
"version": "0.0.1",
"private": true,
"dependencies": {
"express": "3.4.4"
}
}
npm install
If we wanted to install packages globally, we could have instead done the following:
npm install -g
Create a file nodejs/bower.json to list our JavaScript dependencies for the page:
{
"name": "highcharts-cookbook-chapter-8",
"dependencies": {
"jquery": "^1.9",
"highcharts": "~3.0"
}
}
Create a file nodejs/.bowerrc to configure where our JavaScript dependencies will be installed:
{ "directory": "static/js" }
Let’s begin:
Create an example file nodejs/static/index.html for viewing our charts
<html> <head> </head> <body> <div id='example'></div> <script src = './js/jquery/jquery.js'></script> <script src = './js/highcharts/highcharts.js'></script> <script type = 'text/javascript'>
$(document).ready(function() { var options = {
chart: { type: 'bar', events: {
load: function () { var self = this; setInterval(function() {
$.getJSON('/ajax/series', function(data) { var series = self.series[0];
series.setData(data); }); }, 1000); } } }, title: { text: 'Using AJAX for polling charts'
}, series: [{ name: 'AJAX data (series)', data: [] }] }; $('#example').highcharts(options); }); </script> </body> </html>
var express = require('express');
Create a new express application:
var app = express();
Tell our application where to serve static files from:
var app = express(); app.use(express.static('static'));
Create a method to return data:
app.use(express.static('static')); app.get('/ajax/series', function(request, response) { var count = 10, results = []; for(var i = 0; i < count; i++) { results.push({ "y": Math.random()*100 }); } response.json(results); });
Listen on port 8888:
response.json(results); }); app.listen(8888);
Start our application:
node app.js
Most of what we've done in our application is fairly simple: create an express instance, create request methods, and listen on a certain port.
With express, we could also process different HTTP verbs like POST or DELETE. We can handle these methods by creating a new request method. In our example, we handled GET requests (that is, app.get) but in general, we can use app.VERB (Where VERB is an HTTP verb). In fact, we can also be more flexible in what our URLs look like: we can use JavaScript regular expressions as well. More information on the express API can be found at http://expressjs.com/api.html.
Django is likely one of the more robust python frameworks, and certainly one of the oldest. As such, Django can be used to tackle a variety of different cases, and has a lot of support and extensions available. This recipe will look at how we can leverage Django to provide data for Highcharts.
From within the django folder, run the following to create a new project:
django-admin.py startproject example
Create a file django/bower.json to list our JavaScript dependencies
{ "name": "highcharts-cookbook-chapter-8", "dependencies": { "jquery": "^1.9", "highcharts": "~3.0" } }
Create a file django/.bowerrc to configure where our JavaScript dependencies will be installed.
{ "directory": "example/static/js" }
To get started, follow the instructions below:
Create a folder example/templates, and include a file index.html as follows:
{% load staticfiles %} <html> <head> </head> <body> <div class='example' id='example'></div> <script src = '{% static "js/jquery/jquery.js" %}'></script> <script src = '{% static "js/highcharts/highcharts.js" %}'></script> <script type='text/javascript'> $(document).ready(function() { var options = { chart: { type: 'bar', events: { load: function () { var self = this; setInterval(function() { $.getJSON('/ajax/series', function(data) { var series = self.series[0]; series.setData(data); }); }, 1000); } } }, title: { text: 'Using AJAX for polling charts' }, series: [{ name: 'AJAX data (series)', data: [] }] }; $('#example').highcharts(options); }); </script> </body> </html>
Edit example/example/settings.py and include the following at the end of the file:
STATIC_URL = '/static/' TEMPLATE_DIRS = ( os.path.join(BASE_DIR, 'templates/') ) STATICFILES_DIRS = ( os.path.join(BASE_DIR, 'static/'), )
Create a file example/example/views.py and create a handler to show our page:
from django.shortcuts import render_to_response def index(request): return render_to_response('index.html')
Edit example/example/views.py and create a handler to serve our data:
import json from random import randint from django.http import HttpResponse from django.shortcuts import render_to_response def index(request): return render_to_response('index.html') def series(request): results = [] for i in xrange(1, 11): results.append({ 'y': randint(0, 100) }) json_results = json.dumps(results) return HttpResponse(json_results, mimetype='application/json')
from django.conf.urls import patterns, include, url from django.contrib import admin admin.autodiscover() import views urlpatterns = patterns('', # Examples: # url(r'^$', 'example.views.home', name='home'), # url(r'^blog/', include('blog.urls')), url(r'^admin/', include(admin.site.urls)), url(r'^/?$', views.index, name='index'), url(r'^ajax/series/?$', views.series, name='series'), )