In this tutorial, we will look at the installation process of TypeScript and the editor setup for TypeScript development. Microsoft does well in providing easy-to-perform steps to install TypeScript on all platforms, namely Windows, macOS, and Linux.
[box type=”shadow” align=”” class=”” width=””]The following excerpt is taken from the book TypeScript 2.x By Example written by Sachin Ohri. This book presents hands-on examples and projects to learn the fundamental concepts of the popular TypeScript programming language.[/box]
Installation of TypeScript
TypeScript’s official website is the best source to install the latest version. On the website, go to the Download section. There, you will find details on how to install TypeScript. Node.js and Visual Studio are the two most common ways to get it. It supports a host of other editors and has plugins available for them in the same link.
We will be installing TypeScript using Node.js and using Visual Studio Code as our primary editor. You can use any editor of your choice and be able to run the applications seamlessly. If you use full-blown Visual Studio as your primary development IDE, then you can use either of the links, Visual Studio 2017 or Visual Studio 2013, to download the TypeScript SDK. Visual Studio does come with a TypeScript compiler but it’s better to install it from this link so as to get the latest version.
To install TypeScript using Node.js, we will use npm (node package manager), which comes with Node.js. Node.js is a popular JavaScript runtime for building and running server-side JavaScript applications. As TypeScript compiles into JavaScript, Node is an ideal fit for developing server-side applications with the TypeScript language. As mentioned on the website, just running the following command in the Terminal (on macOS) / Command Prompt (on Windows) window will install the latest version:
npm install -g typescript
To load any package from Node.js, the npm command starts with npm install; the -g flag identifies that we are installing the package globally. The last parameter is the name of the package that we are installing. Once it is installed, you can check the version of TypeScript by running the following command in the Terminal window:
tsc -v
You can use the following command to get the help for all the other options that are available with tsc:
tsc -h
TypeScript editors
One of the outstanding features of TypeScript is its support for editors. All the editors provide support for language services, thereby providing features such as IntelliSense, statement completion, and error highlighting.
If you are coming from a .NET background, then Visual Studio 2013/2015/2017 is a good option for you. Visual Studio does not require any configuration and it’s easy to start using TypeScript. As we discussed earlier, just install the SDK and you are good to go.
If you are from a Java background, TypeScript supports Eclipse as well. It also supports plugins for Sublime, WebStorm, and Atom, and each of these provides a rich set of features.
Visual Studio Code (VS Code) is another good option for an IDE. It’s a smaller, lighter version of Visual Studio and primarily used for web application development. VS Code is lightweight and cross-platform, capable of running on Windows, Linux, and macOS. It has an ever-increasing set of plugins to help you write better code, such as TSLint, a static analysis tool to help TypeScript code for readability, maintainability, and error checking. VS Code has a compelling case to be the default IDE for all sorts of web application development.
In this post, we will briefly look at the Visual Studio and VS Code setup for TypeScript.
Visual Studio
Visual Studio is a full-blown IDE provided by Microsoft for all .NET based development, but now Visual Studio also has excellent support for TypeScript with built-in project templates. A TypeScript compiler is integrated into Visual Studio to allow automatic transpiling of code to JavaScript. Visual Studio also has the TypeScript language service integrated to provide IntelliSense and design-time error checking, among other things.
With Visual Studio, creating a project with a TypeScript file is as simple as adding a new file with a .ts extension. Visual Studio will provide all the features out of the box.
VS Code
VS Code is a lightweight IDE from Microsoft used for web application development. VS Code can be installed on Windows, macOS, and Linux-based systems. VS Code can recognize the different type of code files and comes with a huge set of extensions to help in development. You can install VS Code from https://code.visualstudio.com/download.
VS Code comes with an integrated TypeScript compiler, so we can start creating projects directly. The following screenshot shows a TypeScript file opened in VS Code:
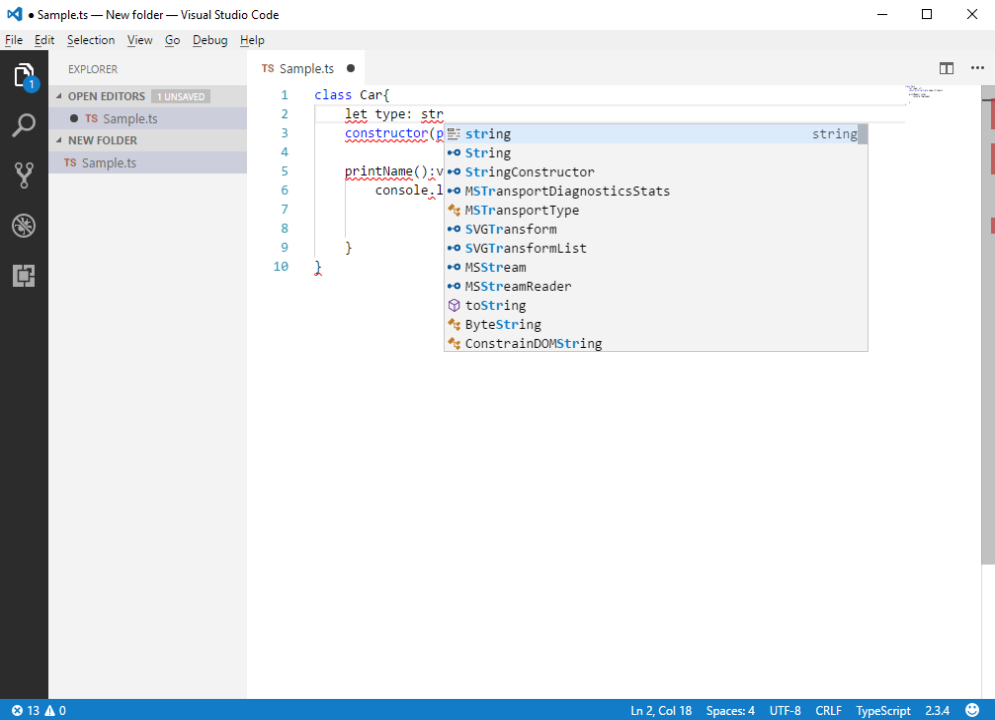
To run the project in VS Code, we need a task runner. VS Code includes multiple task runners which can be configured for the project, such as Gulp, Grunt, and TypeScript. We will be using the TypeScript task runner for our build.
VS Code has a Command Palette which allows you to access various different features, such as Build Task, Themes, Debug options, and so on. To open the Command Palette, use Ctrl + Shift + P on a Windows machine or Cmd + Shift + P on a macOS. In the Command Palette, type Build, as shown in the following screenshot, which will show the command to build the project:
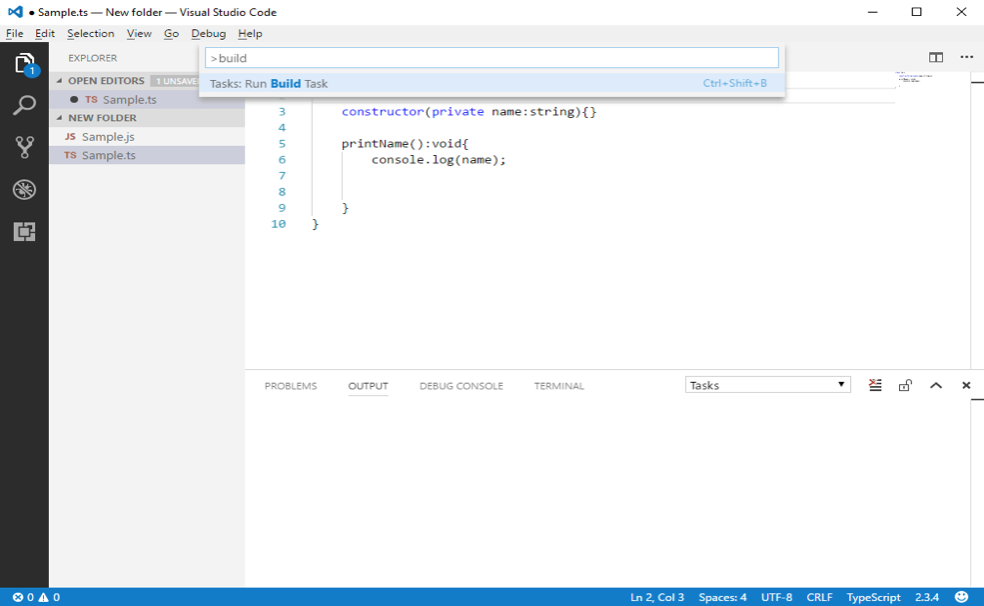
When the command is selected, VS Code shows an alert, No built task defined…, as follows:
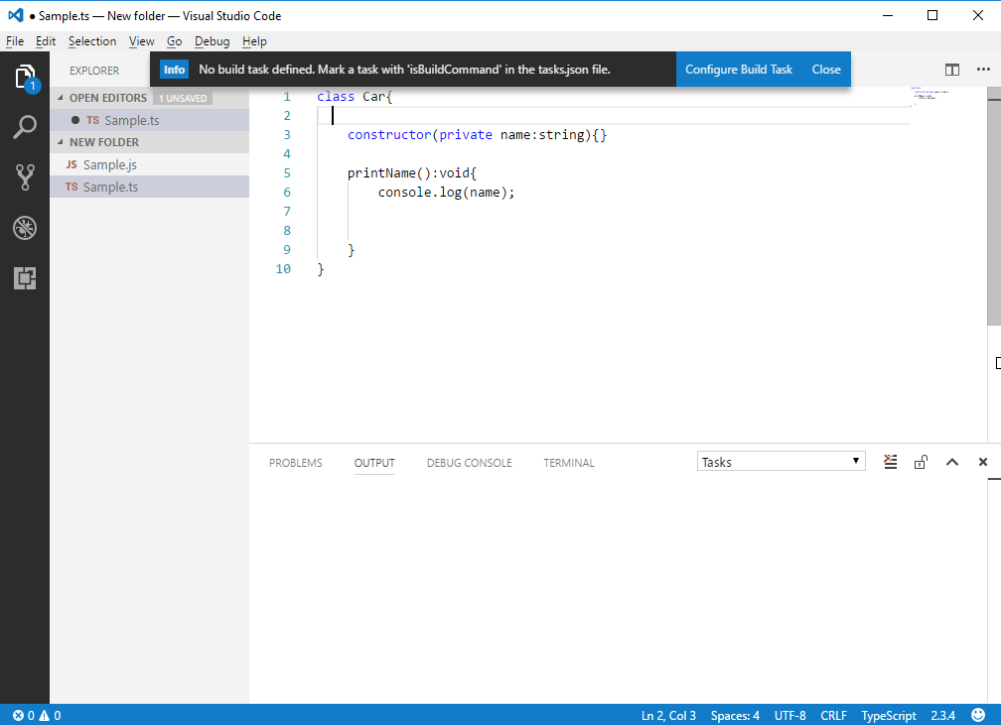
We select Configure Build Task and, from all the available options as shown in the following screenshot, choose TypeScript build:
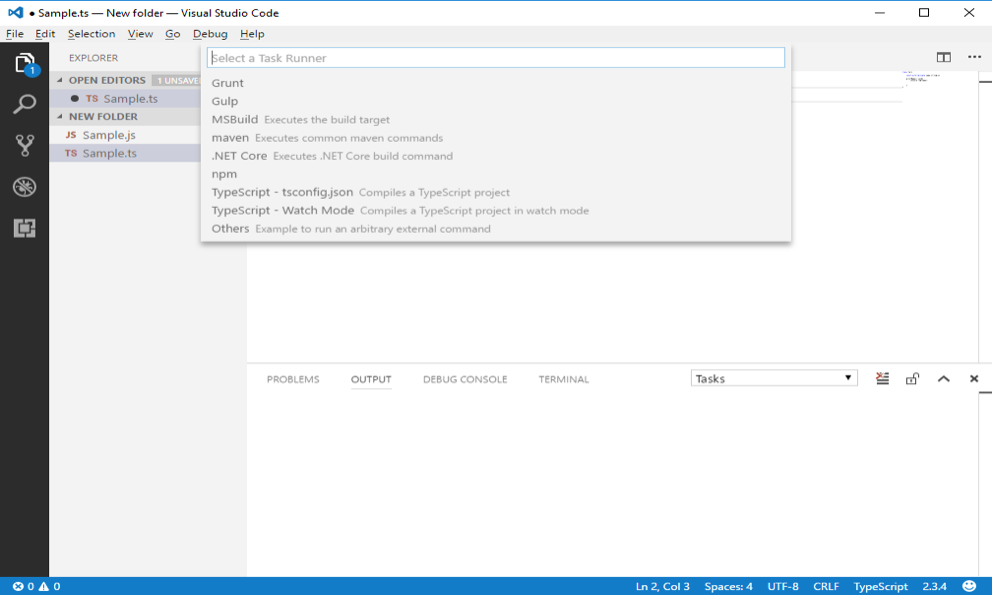
This creates a new folder in your project, .vscode and a new file, task.json. This JSON file is used to create the task that will be responsible for compiling TypeScript code in VS Code.
TypeScript needs another JSON file (tsconfig.json) to be able to configure compiler options. Every time we run the code, tsc will look for a file with this name and use this file to configure itself. TypeScript is extremely flexible in transpiling the code to JavaScript as per developer requirements, and this is achieved by configuring the compiler options of TypeScript.
TypeScript compiler
The TypeScript compiler is called tsc and is responsible for transpiling the TypeScript code to JavaScript. The TypeScript compiler is also cross-platform and supported on Windows, macOS, and Linux.
To run the TypeScript compiler, there are a couple of options. One is to integrate the compiler in your editor of choice, which we explained in the previous section. In the previous section, we also integrated the TypeScript compiler with VS Code, which allowed us to build our code from the editor itself. All the compiler configurations that we would want to use are added to the tsconfig.json file.
Another option is to use tsc directly from the command line / Terminal window. TypeScript’s tsc command takes compiler configuration options as parameters and compiles code into JavaScript. For example, create a simple TypeScript file in Notepad and add the following lines of code to it. To create a file as a TypeScript file, we just need to make sure we have the file extension as *.ts:
class Editor { constructor(public name: string,public isTypeScriptCompatible : Boolean) {} details() { console.log('Editor: ' + this.name + ', TypeScript installed: ' + this.isTypeScriptCompatible); } }
class VisualStudioCode extends Editor{
public OSType: string
constructor(name: string,isTypeScriptCompatible : Boolean,
OSType: string) {
super(name,isTypeScriptCompatible);
this.OSType = OSType;
}
}
let VS = new VisualStudioCode('VSCode', true, 'all');
VS.details();
This is the same code example we used in the TypeScript features section of this chapter. Save this file as app.ts (you can give it any name you want, as long as the extension of the file is *.ts). In the command line / Terminal window, navigate to the path where you have saved this file and run the following command:
tsc app.ts
This command will build the code and the transpile it into JavaScript. The JavaScript file is also saved in the same location where we had TypeScript. If there is any build issue, tsc will show these messages on the command line only.
As you can imagine, running the tsc command manually for medium- to large-scale projects is not a productive approach. Hence, we prefer to use an editor that has TypeScript integrated.
The following table shows the most commonly used TypeScript compiler configurations. We will be discussing these in detail in upcoming chapters:
Compiler option | Type | Description |
allowUnusedLabels | boolean | By default, this flag is false. This option tells the compiler to flag unused labels. |
alwaysStrict | boolean | By default, this flag is false. When turned on, this will cause the compiler to compile in strict mode and emit use strict in the source file. |
module | string | Specify module code generation: None, CommonJS, AMD, System, UMD, ES6, or ES2015. |
moduleResolution | string | Determines how the module is resolved. |
noImplicitAny | boolean | This property allows an error to be raised if there is any code which implies data type as any. This flag is recommended to be turned off if you are migrating a JavaScript project to TypeScript in an incremental manner. |
noImplicitReturn | boolean | Default value is false; raises an error if not all code paths return a value. |
noUnusedLocals | boolean | Reports an error if there are any unused locals in the code. |
noUnusedParameter | boolean | Reports an error if there are any unused parameters in the code. |
outDir | string | Redirects output structure to the directory. |
outFile | string | Concatenates and emits output to a single file. The order of concatenation is determined by the list of files passed to the compiler on the command line along with triple-slash references and imports. See the output file order documentation for more details. |
removeComments | boolean | Remove all comments except copyright header comments beginning with /*!. |
sourcemap | boolean | Generates corresponding .map file. |
Target | string | Specifies ECMAScript target version: ES3(default), ES5, ES6/ES2015, ES2016, ES2017, or ESNext. |
Watch | Runs the compiler in watch mode. Watches input files and triggers recompilation on changes. |
We saw it is quite easy to set up and configure TypeScript, and we are now ready to get started with our first application!
To learn more about writing and compiling your first TypeScript application, make sure you check out the book TypeScript 2.x By Example.
Can you tell what are the primary files to be installed (via npm or Nuget) to start the request and other dB connection related code of typescript ? Every time I install KendoUI for typescript or @type/Request entire project files through 1000 like errors in .ts files like (Severity Code Description Project File Line Suppression State
Error TS2322 (TS) Type ‘string | number | string[]’ is not assignable to type ‘string’.
Type ‘number’ is not assignable to type ‘string’. VuMaxDRWebTS E:\Web VuMaxDR\VuMaxDRTS\VuMaxDRWebTS\VuMaxDRWebTS\Scripts\LoginTS.ts 69 Active)