










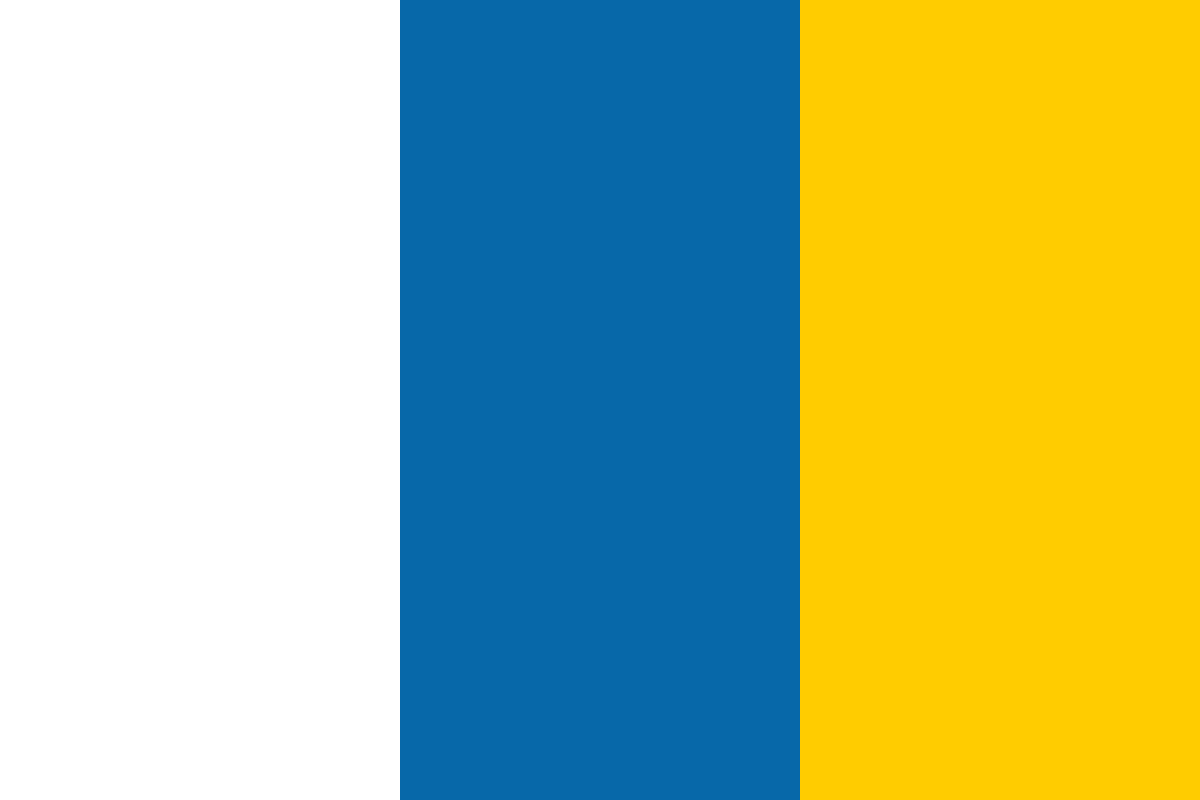

































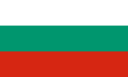








In this article by Rakhitha Nimesh Ratnayake, author of the book WordPress Web Application Development - Second Edition, we will see how to implement frontend registration and how to create a login form in the frontend.
(For more resources related to this topic, see here.)
Fortunately, we can make use of the existing functionalities to implement registration from the frontend. We can use a regular HTTP request or AJAX-based technique to implement this feature. In this article, I will focus on a normal process instead of using AJAX. Our first task is to create the registration form in the frontend.
There are various ways to implement such forms in the frontend. Let's look at some of the possibilities as described in the following section:
Now, let's look at the implementation of each of these techniques.
Shortcodes are the quickest way to add dynamic content to your pages. In this situation, we need to create a page for registration. Therefore, we need to create a shortcode that generates the registration form, as shown in the following code:
add_shortcode( "register_form", "display_register_form" );
function display_register_form(){
$html = "HTML for registration form";
return $html;
}
Then, you can add the shortcode inside the created page using the following code snippet to display the registration form:
[register_form]
Following are the pros and cons of using shortcodes:
Page templates are a widely used technique in modern WordPress themes. We can create a page template to embed the registration form. Consider the following code for a sample page template:
/*
* Template Name : Registration
*/
HTML code for registration form
Next, we have to copy the template inside the theme folder. Finally, we can create a page and assign the page template to display the registration form. Now, let's look at the pros and cons of this technique.
Following are the pros and cons of page templates:
Experienced web application developers will always look to separate business logic from view templates. This will be the perfect technique for such people. In this technique, we will create our own independent templates by intercepting the WordPress default routing process. An implementation of this technique starts from the next section on routing.
Routing is one of the important aspects in advanced application development. We need to figure out ways of building custom routes for specific functionalities. In this scenario, we will create a custom router to handle all the user-related functionalities of our application.
Let's list the requirements for building a router:
Make sure to set up your permalinks structure to post name for the examples in this article. If you prefer a different permalinks structure, you will have to update the URLs and routing rules accordingly.
As you can see, the user section is common for all the functionalities. The second URL segment changes dynamically based on the functionality. In MVC terms, user acts as the controller and the next URL segment (register, login, and activate) acts as the action. Now, let's see how we can implement a custom router for the given requirements.
There are various ways and action hooks used to create custom rewrite rules. We will choose the init action to define our custom routes for the user section, as shown in the following code:
public function manage_user_routes() {
add_rewrite_rule( '^user/([^/]+)/?',
'index.php?control_action=$matches[1]', 'top' );
}
Based on the discussed requirements, all the URLs for the user section will follow the /user/custom action pattern. Therefore, we will define the regular expression for matching all the routes in the user section. Redirection is made to the index.php file with a query variable called control_action. This variable will contain the URL segment after the /user segment. The third parameter of the add_rewrite_rule function will decide whether to check this rewrite rule before the existing rules or after them. The value of top will give a higher precedence, while the value of bottom will give a lower precedence.
We need to complete two other tasks to get these rewriting rules to take effect:
WordPress doesn't allow you to use any type of variable in the query string. It will check for query variables within the existing list and all other variables will be ignored. Whenever we want to use a new query variable, make sure to add it to the existing list. First, we need to update our constructor with the following filter to customize query variables:
add_filter( 'query_vars', array( $this, 'manage_user_routes_query_vars' ) );
This filter on query_vars will allow us to customize the list of existing variables by adding or removing entries from an array. Now, consider the implementation to add a new query variable:
public function manage_user_routes_query_vars( $query_vars ) {
$query_vars[] = 'control_action';
return $query_vars;
}
As this is a filter, the existing query_vars variable will be passed as an array. We will modify the array by adding a new query variable called control_action and return the list. Now, we have the ability to access this variable from the URL.
Once rewrite rules are modified, it's a must to flush the rules in order to prevent 404 page generation. Flushing existing rules is a time consuming task, which impacts the performance of the application and hence should be avoided in repetitive actions such as init. It's recommended that you perform such tasks in plugin activation or installation as we did earlier in user roles and capabilities. So, let's implement the function for flushing rewrite rules on plugin activation:
public function flush_application_rewrite_rules() {
flush_rewrite_rules();
}
As usual, we need to update the constructor to include the following action to call the flush_application_rewrite_rules function:
register_activation_hook( __FILE__, array( $this,
'flush_application_rewrite_rules' ) );
Now, go to the admin panel, deactivate the plugin, and activate the plugin again. Then, go to the URL http://www.example.com/user/login and check whether it works. Unfortunately, you will still get the 404 error for the request.
You might be wondering what went wrong. Let's go back and think about the process in order to understand the issue. We flushed the rules on plugin activation. So, the new rules should persist successfully. However, we will define the rules on the init action, which is only executed after the plugin is activated. Therefore, new rules will not be available at the time of flushing.
Consider the updated version of the flush_application_rewrite_rules function for a quick fix to our problem:
public function flush_application_rewrite_rules() {
$this->manage_user_routes();
flush_rewrite_rules();
}
We call the manage_user_routes function on plugin activation, followed by the call to flush_rewrite_rules. So, the new rules are generated before flushing is executed. Now, follow the previous process once again; you won't get a 404 page since all the rules have taken effect.
You can get 404 errors due to the modification in rewriting rules and not flushing it properly. In such situations, go to the Permalinks section on the Settings page and click on the Save Changes button to flush the rewrite rules manually.
Now, we are ready with our routing rules for user functionalities. It's important to know the existing routing rules of your application. Even though we can have a look at the routing rules from the database, it's difficult to decode the serialized array, as we encountered in the previous section.
So, I recommend that you use the free plugin called Rewrite Rules Inspector. You can grab a copy at http://wordpress.org/plugins/rewrite-rules-inspector/. Once installed, this plugin allows you to view all the existing routing rules as well as offers a button to flush the rules, as shown in the following screen:
We have a custom router, which handles the URLs of the user section of our application. Next, we need a controller to handle the requests and generate the template for the user. This works similar to the controllers in the MVC pattern. Even though we have changed the default routing, WordPress will look for an existing template to be sent back to the user. Therefore, we need to intercept this process and create our own templates. WordPress offers an action hook called template_redirect for intercepting requests. So, let's implement our frontend controller based on template_redirect. First, we need to update the constructor with the template_redirect action, as shown in the following code:
add_action( 'template_redirect', array( $this, 'front_controller' ) );
Now, let's take a look at the implementation of the front_controller function using the following code:
public function front_controller() {
global $wp_query;
$control_action = isset ( $wp_query-
>query_vars['control_action'] ) ? $wp_query-
>query_vars['control_action'] : ''; ;
switch ( $control_action ) {
case 'register':
do_action( 'wpwa_register_user' );
break;
}
}
We will be handling custom routes based on the value of the control_action query variable assigned in the previous section. The value of this variable can be grabbed through the global query_vars array of the $wp_query object. Then, we can use a simple switch statement to handle the controlling based on the action.
The first action to consider will be to register as we are in the registration process. Once the control_action query variable is matched with registration, we will call a handler function using do_action. You might be confused why we use do_action in this scenario. So, let's consider the same implementation in a normal PHP application, where we don't have the do_action hook:
switch ( $control_action ) {
case 'register':
$this->register_user();
break;
}
This is the typical scenario where we call a function within the class or in an external class to implement the registration. In the previous code, we called a function within the class, but with the do_action hook instead of the usual function call.
WordPress action hooks define specific points in the execution process, where we can develop custom functions to modify existing behavior. In this scenario, we are calling the wpwa_register_user function within the class using do_action.
Unlike websites or blogs, web applications need to be extendable with future requirements. Think of a situation where we only allow Gmail addresses for user registration. This Gmail validation is not implemented in the original code. Therefore, we need to change the existing code to implement the necessary validations. Changing a working component is considered bad practice in application development. Let's see why it's considered as a bad practice by looking at the definition of the open/closed principle on Wikipedia.
"Open/closed principle states "software entities (classes, modules, functions, and so on) should be open for extension, but closed for modification"; that is, such an entity can allow its behavior to be modified without altering its source code. This is especially valuable in a production environment, where changes to the source code may necessitate code reviews, unit tests, and other such procedures to qualify it for use in a product: the code obeying the principle doesn't change when it is extended, and therefore, needs no such effort."
WordPress action hooks come to our rescue in this scenario. We can define an action for registration using the add_action function, as shown in the following code:
add_action( 'wpwa_register_user', array( $this, 'register_user' ) );
Now, you can implement this action multiple times using different functions. In this scenario, register_user will be our primary registration handler. For Gmail validation, we can define another function using the following code:
add_action( 'wpwa_register_user', array( $this, 'validate_gmail_registration') );
Inside this function, we can make the necessary validations, as shown in the following code:
public function validate_user(){
// Code to validate user
// remove registration function if validation fails
remove_action( 'wpwa_register_user', array( $this,
'register_user' ) );
}
Now, the validate_user function is executed before the primary function. So, we can remove the primary registration function if something goes wrong in validation. With this technique, we have the capability of adding new functionalities as well as changing existing functionalities without affecting the already written code.
We have implemented a simple controller, which can be quite effective in developing web application functionalities. In the following sections, we will continue the process of implementing registration on the frontend with custom templates.
Themes provide a default set of templates to cater to the existing behavior of WordPress. Here, we are trying to implement a custom template system to suit web applications. So, our first option is to include the template files directly inside the theme. Personally, I don't like this option due to two possible reasons:
As a solution to these concerns, we will implement the custom templates inside the plugin. First, create a folder inside the current plugin folder and name it as templates to get things started.
We need to design a custom form for frontend registration containing the default header and footer. The whole content area will be used for the registration and the default sidebar will be omitted for this screen. Create a PHP file called register-template.php inside the templates folder with the following code:
<?php get_header(); ?>
<div id="wpwa_custom_panel">
<?php
if( isset($errors) && count( $errors ) > 0) {
foreach( $errors as $error ){
echo '<p class="wpwa_frm_error">'. $error .'</p>';
}
}
?>
HTML Code for Form
</div>
<?php get_footer(); ?>
We can include the default header and footer using the get_header and get_footer functions, respectively. After the header, we will include a display area for the error messages generated in registration. Then, we have the HTML form, as shown in the following code:
<form id='registration-form' method='post' action='<?php echo
get_site_url() . '/user/register'; ?>'>
<ul>
<li>
<label class='wpwa_frm_label'><?php echo
__('Username','wpwa'); ?></label>
<input class='wpwa_frm_field' type='text'
id='wpwa_user' name='wpwa_user' value='' />
</li>
<li>
<label class='wpwa_frm_label'><?php echo __('Email','
wpwa'); ?></label>
<input class='wpwa_frm_field' type='text'
id='wpwa_email' name='wpwa_email' value='' />
</li>
<li>
<label class='wpwa_frm_label'><?php echo __('User
Type','wpwa'); ?></label>
<select class='wpwa_frm_field' name='wpwa_user_type'>
<option <?php echo __('Follower','wpwa');
?></option>
<option <?php echo __('Developer','wpwa');
?></option>
<option <?php echo __('Member','wpwa');
?></option>
</select>
</li>
<li>
<label class='wpwa_frm_label' for=''> </label>
<input type='submit' value='<?php echo
__('Register','wpwa'); ?>' />
</li>
</ul>
</form>
As you can see, the form action is set to a custom route called user/register to be handled through the front controller. Also, we have added an extra field called user type to choose the preferred user type on registration.
You might have noticed that we used wpwa as the prefix for form element names, element IDs, as well as CSS classes. Even though it's not a must to use a prefix, it can be highly effective when working with multiple third-party plugins. A unique plugin-specific prefix avoids or limits conflicts with other plugins and themes.
We will get a screen similar to the following one, once we access the /user/register link in the browser:
Once the form is submitted, we have to create the user based on the application requirements.
In this application, we have opted to build a complex registration process in order to understand the typical requirements of web applications. So, it's better to plan it upfront before moving into the implementation. Let's build a list of requirements for registration:
So, let's begin the task of registering users by displaying the registration form as given in the following code:
public function register_user() {
if ( !is_user_logged_in() ) {
include dirname(__FILE__) . '/templates/registertemplate.
php';
exit;
}
}
Once user requests /user/register, our controller will call the register_user function using the do_action call. In the initial request, we need to check whether a user is already logged in using the is_user_logged_in function. If not, we can directly include the registration template located inside the templates folder to display the registration form.
WordPress templates can be included using the get_template_part function. However, it doesn't work like a typical template library, as we cannot pass data to the template. In this technique, we are including the template directly inside the function. Therefore, we have access to the data inside this function.
Once the user fills the data and clicks the submit button, we have to execute quite a few tasks in order to register a user in WordPress database. Let's figure out the main tasks for registering a user:
In the registration form, we specified the action as /user/register, and hence the same register_user function will be used to handle form submission. Validating user data is one of the main tasks in form submission handling. So, let's take a look at the register_user function with the updated code:
public function register_user() {
if ( $_POST ) {
$errors = array();
$user_login = ( isset ( $_POST['wpwa_user'] ) ?
$_POST['wpwa_user'] : '' );
$user_email = ( isset ( $_POST['wpwa_email'] ) ?
$_POST['wpwa_email'] : '' );
$user_type = ( isset ( $_POST['wpwa_user_type'] ) ?
$_POST['wpwa_user_type'] : '' );
// Validating user data
if ( empty( $user_login ) )
array_push($errors, __('Please enter a username.','wpwa') );
if ( empty( $user_email ) )
array_push( $errors, __('Please enter e-mail.','wpwa') );
if ( empty( $user_type ) )
array_push( $errors, __('Please enter user type.','wpwa') );
}
// Including the template
}
The following steps are to be performed:
Now, we can move into more advanced validations inside the register_user function, as shown in the following code:
$sanitized_user_login = sanitize_user( $user_login );
if ( !empty($user_email) && !is_email( $user_email ) )
array_push( $errors, __('Please enter valid email.','wpwa'));
elseif ( email_exists( $user_email ) )
array_push( $errors, __('User with this email already
registered.','wpwa'));
if ( empty( $sanitized_user_login ) || !validate_username(
$user_login ) )
array_push( $errors, __('Invalid username.','wpwa') );
elseif ( username_exists( $sanitized_user_login ) )
array_push( $errors, __('Username already exists.','wpwa') );
The steps to perform are as follows:
In this scenario, we choose to go with the most essential validations for the registration form. You can add more advanced validation in your implementations in order to minimize potential security threats.
In case we get validation errors in the form, we can directly print the contents of the error array on top of the form as it's visible to the registration template. Here is a preview of our registration screen with generated error messages:
Also, it's important to repopulate the form values once errors are generated. We are using the same function for loading the registration form and handling form submission. Therefore, we can directly access the POST variables inside the template to echo the values, as shown in the updated registration form:
<form id='registration-form' method='post' action='<?php echo
get_site_url() . '/user/register'; ?>'>
<ul>
<li>
<label class='wpwa_frm_label'><?php echo
__('Username','wpwa'); ?></label>
<input class='wpwa_frm_field' type='text'
id='wpwa_user' name='wpwa_user' value='<?php echo isset(
$user_login ) ? $user_login : ''; ?>' />
</li>
<li>
<label class='wpwa_frm_label'><?php echo __('Email','
wpwa'); ?></label>
<input class='wpwa_frm_field' type='text'
id='wpwa_email' name='wpwa_email' value='<?php echo isset(
$user_email ) ? $user_email : ''; ?>' />
</li>
<li>
<label class='wpwa_frm_label'><?php echo __('User
"Type','wpwa'); ?></label>
<select class='wpwa_frm_field' name='wpwa_user_type'>
<option <?php echo (isset( $user_type ) &&
$user_type == 'follower') ? 'selected' : ''; ?> value='follower'><?php
echo __('Follower','wpwa'); ?></option>
<option <?php echo (isset( $user_type ) &&
$user_type == 'developer') ? 'selected' : ''; ?>
value='developer'><?php echo __('Developer','wpwa'); ?></option>
<option <?php echo (isset( $user_type ) && $user_type ==
'member') ? 'selected' : ''; ?> value='member'><?php
echo __('Member','wpwa'); ?></option>
</select>
</li>
<li>
<label class='wpwa_frm_label' for=''> </label>
<input type='submit' value='<?php echo
__('Register','wpwa'); ?>' />
</li>
</ul>
</form>
Now, let's look at the success path, where we don't have any errors by looking at the remaining sections of the register_user function:
if ( empty( $errors ) ) {
$user_pass = wp_generate_password();
$user_id = wp_insert_user( array('user_login' =>
$sanitized_user_login,
'user_email' => $user_email,
'role' => $user_type,
'user_pass' => $user_pass)
);
if ( !$user_id ) {
array_push( $errors, __('Registration failed.','wpwa') );
} else {
$activation_code = $this->random_string();
update_user_meta( $user_id, 'wpwa_activation_code',
$activation_code );
update_user_meta( $user_id, 'wpwa_activation_status', 'inactive'
);
wp_new_user_notification( $user_id, $user_pass, $activation_code
);
$success_message = __('Registration completed successfully.
Please check your email for activation link.','wpwa');
}
if ( !is_user_logged_in() ) {
include dirname(__FILE__) . '/templates/login-template.php';
exit;
}
}
We can generate the default password using the wp_generate_password function. Then, we can use the wp_insert_user function with respective parameters generated from the form to save the user in the database.
The wp_insert_user function will be used to update the current user or add new users to the application. Make sure you are not logged in while executing this function; otherwise, your admin will suddenly change into another user type after using this function.
If the system fails to save the user, we can create a registration fail message and assign it to the $errors variable as we did earlier. Once the registration is successful, we will generate a random string as the activation code. You can use any function here to generate a random string.
Then, we update the user with activation code and set the activation status as inactive for the moment. Finally, we will use the wp_new_user_notification function to send an e-mail containing the registration details. By default, this function takes the user ID and password and sends the login details. In this scenario, we have a problem as we need to send an activation link with the e-mail.
This is a pluggable function and hence we can create our own implementation of this function to override the default behavior. Since this is a built-in WordPress function, we cannot declare it inside our plugin class. So, we will implement it as a standalone function inside our main plugin file. The full source code for this function will not be included here as it is quite extensive. I'll explain the modified code from the original function and you can have a look at the source code for the complete code:
$activate_link = site_url() .
"/user/activate/?wpwa_activation_code=$activate_code";
$message = __('Hi there,') . 'rnrn';
$message .= sprintf(__('Welcome to %s! Please activate your
account using the link:','wpwa'), get_option('blogname')) .
'rnrn';
$message .= sprintf(__('<a href="%s">%s</a>','wpwa'),
$activate_link, $activate_link) . 'rn';
$message .= sprintf(__('Username: %s','wpwa'), $user_login) .
'rn';
$message .= sprintf(__('Password: %s','wpwa'), $plaintext_pass) .
'rnrn';
We create a custom activation link using the third parameter passed to this function. Then, we modify the existing message to include the activation link. That's about all we need to change from the original function. Finally, we set the success message to be passed into the login screen.
Now, let's move back to the register_user function. Once the notification is sent, the registration process is completed and the user will be redirected to the login screen. Once the user has the e-mail in their inbox, they can use the activation link to activate the account.
In general, most web applications uses e-mail confirmations before allowing users to log in to the system. However, there can be certain scenarios where we need to automatically authenticate the user into the application. A social network sign in is a great example for such a scenario. When using social network logins, the system checks whether the user is already registered. If not, the application automatically registers the user and authenticates them. We can easily modify our code to implement an automatic login after registration. Consider the following code:
if ( !is_user_logged_in() ) {
wp_set_auth_cookie($user_id, false, is_ssl());
include dirname(__FILE__) . '/templates/login-template.php';
exit;
}
The registration code is updated to use the wp_set_auth_cookie function. Once it's used, the user authentication cookie will be created and hence the user will be considered as automatically signed in. Then, we will redirect to the login page as usual. Since the user is already logged in using the authentication cookie, they will be redirected back to the home page with access to the backend. This is an easy way of automatically authenticating users into WordPress.
Once the user clicks on the activate link, redirection will be made to the /user/activate URL of the application. So, we need to modify our controller with a new case for activation, as shown in the following code:
case 'activate':
do_action( 'wpwa_activate_user' );
As usual, the definition of add_action goes in the constructor, as shown in the following code:
add_action( 'wpwa_activate_user', array( $this,'activate_user') );
Next, we can have a look at the actual implementation of the activate_user function:
public function activate_user() {
$activation_code = isset( $_GET['wpwa_activation_code'] ) ?
$_GET['wpwa_activation_code'] : '';
$message = '';
// Get activation record for the user
$user_query = new WP_User_Query(
array(
'meta_key' => ' wpwa_activation_code',
'meta_value' => $activation_code
)
);
$users = $user_query->get_results();
// Check and update activation status
if ( !empty($users) ) {
$user_id = $users[0]->ID;
update_user_meta( $user_id, ' wpwa_activation_status',
'active' );
$message = __('Account activated successfully.','wpwa');
} else {
$message = __('Invalid Activation Code','wpwa');
}
include dirname(__FILE__) . '/templates/info-template.php';
exit;
}
We will get the activation code from the link and query the database for finding a matching entry. If no records are found, we set the message as activation failed or else, we can update the activation status of the matching user to activate the account. Upon activation, the user will be given a message using the info-template.php template, which consists of a very basic template like the following one:
<?php get_header(); ?>
<div id='wpwa_info_message'>
<?php echo $message; ?>
</div>
<?php get_footer(); ?>
Once the user visits the activation page on the /user/activation URL, information will be given to the user, as illustrated in the following screen:
We successfully created and activated a new user. The final task of this process is to authenticate and log the user into the system. Let's see how we can create the login functionality.
The frontend login can be found in many WordPress websites, including small blogs. Usually, we place the login form in the sidebar of the website. In web applications, user interfaces are complex and different, compared to normal websites. Hence, we will implement a full page login screen as we did with registration. First, we need to update our controller with another case for login, as shown in the following code:
switch ( $control_action ) {
// Other cases
case 'login':
do_action( 'wpwa_login_user' );
break;
}
This action will be executed once the user enters /user/login in the browser URL to display the login form. The design form for login will be located in the templates directory as a separate template called login-template.php. Here is the implementation of the login form design with the necessary error messages:
<?php get_header(); ?>
<div id=' wpwa_custom_panel'>
<?php
if (isset($errors) && count($errors) > 0) {
foreach ($errors as $error) {
echo '<p class="wpwa_frm_error">' .$error. '</p>';
}
}
if( isset( $success_message ) && $success_message != ""){
echo '<p class="wpwa_frm_success">' .$success_message.
'</p>';
}
?>
<form method='post' action='<?php echo site_url();
?>/user/login' id='wpwa_login_form' name='wpwa_login_form'>
<ul>
<li>
<label class='wpwa_frm_label' for='username'><?php
echo __('Username','wpwa'); ?></label>
<input class='wpwa_frm_field' type='text'
name='wpwa_username' value='<?php echo isset( $username ) ?
$username : ''; ?>' />
</li>
<li>
<label class='wpwa_frm_label' for='password'><?php
echo __('Password','wpwa'); ?></label>
<input class='wpwa_frm_field' type='password'
name='wpwa_password' value="" />
</li>
<li>
<label class='wpwa_frm_label' > </label>
<input type='submit' name='submit' value='<?php echo
__('Login','wpwa'); ?>' />
</li>
</ul>
</form>
</div>
<?php get_footer(); ?>
Similar to the registration template, we have a header, error messages, the HTML form, and the footer in this template. We have to point the action of this form to /user/login. The remaining code is self-explanatory and hence I am not going to make detailed explanations. You can take a look at the preview of our login screen in the following screenshot:
Next, we need to implement the form submission handler for the login functionality. Before this, we need to update our plugin constructor with the following code to define another custom action for login:
add_action( 'wpwa_login_user', array( $this, 'login_user' ) );
Once the user requests /user/login from the browser, the controller will execute the do_action( 'wpwa_login_user' ) function to load the login form in the frontend.
We will use the same function to handle both template inclusion and form submission for login, as we did earlier with registration. So, let's look at the initial code of the login_user function for including the template:
public function login_user() {
if ( !is_user_logged_in() ) {
include dirname(__FILE__) . '/templates/login-template.php';
} else {
wp_redirect(home_url());
}
exit;
}
First, we need to check whether the user has already logged in to the system. Based on the result, we will redirect the user to the login template or home page for the moment. Once the whole system is implemented, we will be redirecting the logged in users to their own admin area.
Now, we can take a look at the implementation of the login to finalize our process. Let's take a look at the form submission handling part of the login_user function:
if ( $_POST ) {
$errors = array();
$username = isset ( $_POST['wpwa_username'] ) ?
$_POST['wpwa_username'] : '';
$password = isset ( $_POST['wpwa_password'] ) ?
$_POST['wpwa_password'] : '';
if ( empty( $username ) )
array_push( $errors, __('Please enter a username.','wpwa') );
if ( empty( $password ) )
array_push( $errors, __('Please enter password.','wpwa') );
if(count($errors) > 0){
include dirname(__FILE__) . '/templates/login-template.php';
exit;
}
$credentials = array();
$credentials['user_login'] = $username;
$credentials['user_login'] = sanitize_user(
$credentials['user_login'] );
$credentials['user_password'] = $password;
$credentials['remember'] = false;
// Rest of the code
}
As usual, we need to validate the post data and generate the necessary errors to be shown in the frontend. Once validations are successfully completed, we assign all the form data to an array after sanitizing the values. The username and password are contained in the credentials array with the user_login and user_password keys. The remember key defines whether to remember the password or not. Since we don't have a remember checkbox in our form, it will be set to false. Next, we need to execute the WordPress login function in order to log the user into the system, as shown in the following code:
$user = wp_signon( $credentials, false );
if ( is_wp_error( $user ) )
array_push( $errors, $user->get_error_message() );
else
wp_redirect( home_url() );
WordPress handles user authentication through the wp_signon function. We have to pass all the credentials generated in the previous code with an additional second parameter of true or false to define whether to use a secure cookie. We can set it to false for this example. The wp_signon function will return an object of the WP_User or the WP_Error class based on the result.
Internally, this function sets an authentication cookie. Users will not be logged in if it is not set. If you are using any other process for authenticating users, you have to set this authentication cookie manually.
Once a user is successfully authenticated, a redirection will be made to the home page of the site. Now, we should have the ability to authenticate users from the login form in the frontend.
Take a moment to think carefully about our requirements and try to figure out what we have missed.
Actually, we didn't check the activation status on log in. Therefore, any user will be able to log in to the system without activating their account. Now, let's fix this issue by intercepting the authentication process with another built-in action called authenticate, as shown in the following code:
public function authenticate_user( $user, $username, $password ) {
if(! empty($username) && !is_wp_error($user)){
$user = get_user_by('login', $username );
if (!in_array( 'administrator', (array) $user->roles ) ) {
$active_status = '';
$active_status = get_user_meta( $user->data->ID, 'wpwa_
activation_status', true );
if ( 'inactive' == $active_status ) {
$user = new WP_Error( 'denied', __('<strong>ERROR</strong>:
Please activate your account.','wpwa'
) );
}
}
}
return $user;
}
This function will be called in the authentication action by passing the user, username, and password variables as default parameters. All the user types of our application need to be activated, except for the administrator accounts. Therefore, we check the roles of the authenticated user to figure out whether they are admin. Then, we can check the activation status of other user types before authenticating. If an authenticated user is in inactive status, we can return the WP_Error object and prevent authentication from being successful.
Last but not least, we have to include the authenticate action in the controller, to make it work as shown in the following code:
add_filter( 'authenticate', array( $this, 'authenticate_user' ), 30, 3 );
This filter is also executed when the user logs out of the application. Therefore, we need to consider the following validation to prevent any errors in the logout process:
if(! empty($username) && !is_wp_error($user))
Now, we have a simple and useful user registration and login system, ready to be implemented in the frontend of web applications. Make sure to check login- and registration-related plugins from the official repository to gain knowledge of complex requirements in real-world scenarios.
In this article, we implemented a simple registration and login functionality from the frontend. Before we have a complete user creation and authentication system, there are plenty of other tasks to be completed. So, I would recommend you to try out the following tasks in order to be comfortable with implementing such functionalities for web applications:
Make sure to try out these exercises and validate your answers against the implementations provided on the website for this book.
In this article, we looked at how we can customize the built-in registration and login process in the frontend to cater to advanced requirements in web application development.
By now, you should be capable of creating custom routers for common modules, implement custom controllers with custom template systems, and customize the existing user registration and authentication process.
Further resources on this subject: