










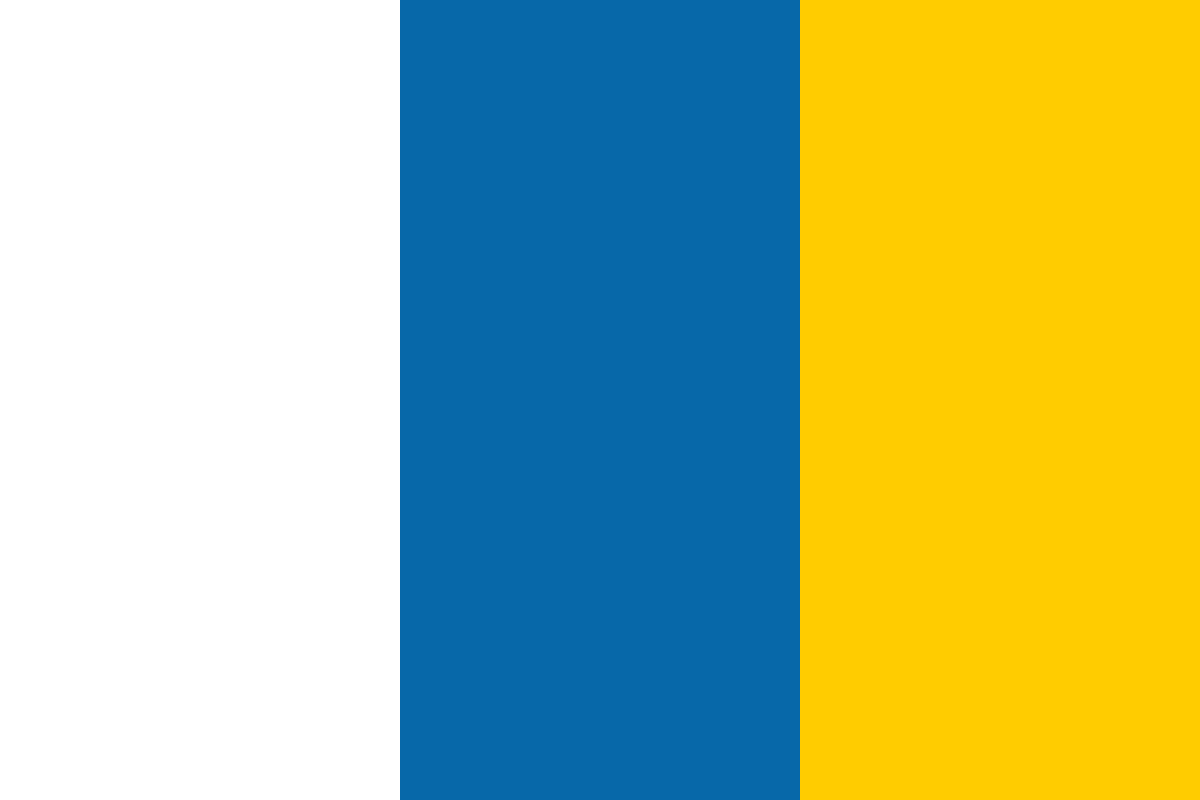

































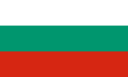








A step-by-step guide for web application development and quick tips to enhance applications using Lotus Domino
Simple views intended to provide information (for example, a table of values) or links to a limited number of documents can stand alone quite nicely, embedded on a page or a view template. But if more than a handful of documents display in the view, you should provide users a way to move forward and backward through the view. If you use the View Applet, enable the scroll bars; otherwise add some navigational buttons to the view templates to enable users to move around in it.
If you set the line count for a view, only that number of rows is sent to the browser. You need to add Action buttons or hotspots on the view template to enable users to advance the view to the next set of documents or to return to the previous set of documents—essentially paging backward and forward through the view.
Code a Next button with this formula:
@DbCommand("Domino"; "ViewNextPage")
Code a Previous button with this formula:
@DbCommand("Domino"; "ViewPreviousPage")
Buttons can be included on the view template to page to the first and last documents in the view. Code an @Formula in a First button's Click event to compute and open a relative URL. The link reopens the current view and positions it at the first document:
@URLOpen("/"+@WebDbName+"/"+@Subset(@ViewTitle;-1) +
"?OpenView&Start=1")
For a Last button, add a Computed for Display field to the view template with this @Formula:
@Elements(@DbColumn("":"NoCache"; "" ; @ViewTitle; 1))
The value for the field (vwRows in this example) is the current number of documents in the view. This information is used in the @Formula for the Last button's Click event:
url := "/" + @WebDbName + "/" + @Subset(@ViewTitle;-1) ;
@URLOpen(url + "?OpenView&Start=" + @Text(vwRows))
When Last is clicked, the view reopens, positioned at the last document.
Please note that for very large views, the @Formula for field vwRows may fail because of limitations in the amount of data that can be returned by @DbColumn.
As computer monitors today come in a wide range of sizes and resolutions, it may be difficult to determine the right number of documents to display in a view to accommodate all users. On some monitors the view may seem too short, on others too long.
Here is a strategy you might adapt to your application, that enables users to specify how many lines to display. The solution relies on several components working together:
The technique uses the Start and Count parameters, which can be used when you open a view with a URL. The Start parameter, used in a previous example, specifies the row or document within a view that should display at the top of the view window on a page. The Count parameter specifies how many rows or documents should display on the page. The Count parameter overrides the line count setting that you may have set on an embedded view element.
Here are the Computed for display fields to be created on the view template. The Query_String_Decoded field (a CGI variable) must be named as such, but all the other field names in this list are arbitrary. Following each field name is the @Formula that computes its value:
Query_String_Decoded
@Right(@LowerCase(Query_String_Decoded); "&")
@If(@Contains(vwParms; "start="); @Middle(vwParms; "start=";
"&"); "1")
@If(@Contains(vwParms; "count="); @Middle(vwParms; "count=";
"&"); "10")
"/" + @WebDbName + "/"+ @Subset(@ViewTitle;1) + "?OpenView"
@Elements(@DbColumn("":"NoCache"; ""; @ViewTitle; 1))
"n"
"1"
Add several buttons to the view template. Code JavaScript in each button's onClick event. You may want to code these scripts inline for testing, and then move them to a JavaScript library when you know they are working the way you want them to.
The Set Rows button's onClick event is coded with JavaScript that receives a line count from the user. If the user-entered line count is not good, then the current line count is retained. A flag is set indicating that the line count may have been changed:
var f = document.forms[0] ;
var rows = parseInt(f.vwRows.value) ;
var count = prompt("Number of Rows?","10") ;
if ( isNaN(count) | count < 1 | count >= rows ) {
count = f.vwCount.value ;
}
f.newCount.value = count ;
f.countFlag.value = "y" ;
The Previous button's onClick event is coded to page backward through the view using the user-entered line count:
var f = document.forms[0] ;
var URL = f.vwURL.value ;
var ctFlag = f.countFlag.value ;
var oCT = parseInt(f.vwCount.value) ;
var nCT = parseInt(f.newCount.value) ;
var oST = parseInt(f.vwStart.value) ;
var count ;
var start ;
if ( ctFlag == "n" ) {
count = oCT ;
start = oST - oCT ;
}
else {
count = nCT ;
start = oST - nCT ;
}
if (start < 1 ) { start = 1 ; }
location.href = URL + "&Start=" + start + "&Count=" + count ;
The Next button pages forward through the view using the user-entered line count:
var f = document.forms[0] ;
var URL = f.vwURL.value ;
var ctFlag = f.countFlag.value ;
var oCT = parseInt(f.vwCount.value) ;
var nCT = parseInt(f.newCount.value) ;
var start = parseInt(f.vwStart.value) + oCT ;
if ( ctFlag == "n" ) {
location.href = URL + "&Start=" + start + "&Count=" + oCT ;
}
else {
location.href = URL + "&Start=" + start + "&Count=" + nCT ;
}
Finally, if First and Last buttons are included with this scheme, they need to be recoded as well to work with a user-specified line count.
The @formula in the First button's Click event now looks like this:
count := @If(@IsAvailable(vwCount); vwCount; "10") ;
parms := "?OpenView&Start=1&Count=" + count ;
@URLOpen("/" + @WebDbName + "/" + @Subset(@ViewTitle;-1) + parms)
;7
The @formula in the Last button's Click event is also a little more complicated. Note that if the field vwRows is not available, then the Start value is set to 1,000. This is really more for debugging since the Start parameter should always be set to the value of vwRows:
start := @If(@IsAvailable(vwRows); @Text(vwRows); "1000") ;
count := @If(@IsAvailable(vwCount); vwCount; "10") ;
parms := "?OpenView&Start=" + start + "&Count=" + count ;
url := "/" + @WebDbName + "/" + @Subset(@ViewTitle;-1) ;
@URLOpen(url + parms) ;
Two other navigational buttons should be included on the view template for categorized views or views that include document hierarchies. These buttons expand all categories and collapse all categories respectively:
@Command([ViewExpandAll])
@Command([ViewCollapseAll])
Action Bar buttons can be added to a view template as well as to a view. If Action buttons appear on both design elements, then Domino places all the buttons together on the same top row. In the following image, the first button is from the view template, and the last three are from the view itself:
If it makes more sense for the buttons to be arranged in a different order, then take control of their placement by co-locating them all either on the view template or on the view.
As mentioned previously, Action Bar buttons are rendered in a table placed at the top of a form. But on typical Web pages, buttons and hotspots are located below a banner, or in a menu at the left or the right. Buttons along the top of a form look dated and may not comply with your organization's web development standards.
You can replace the view template and view Action buttons with hotspot buttons placed elsewhere on the view template: