










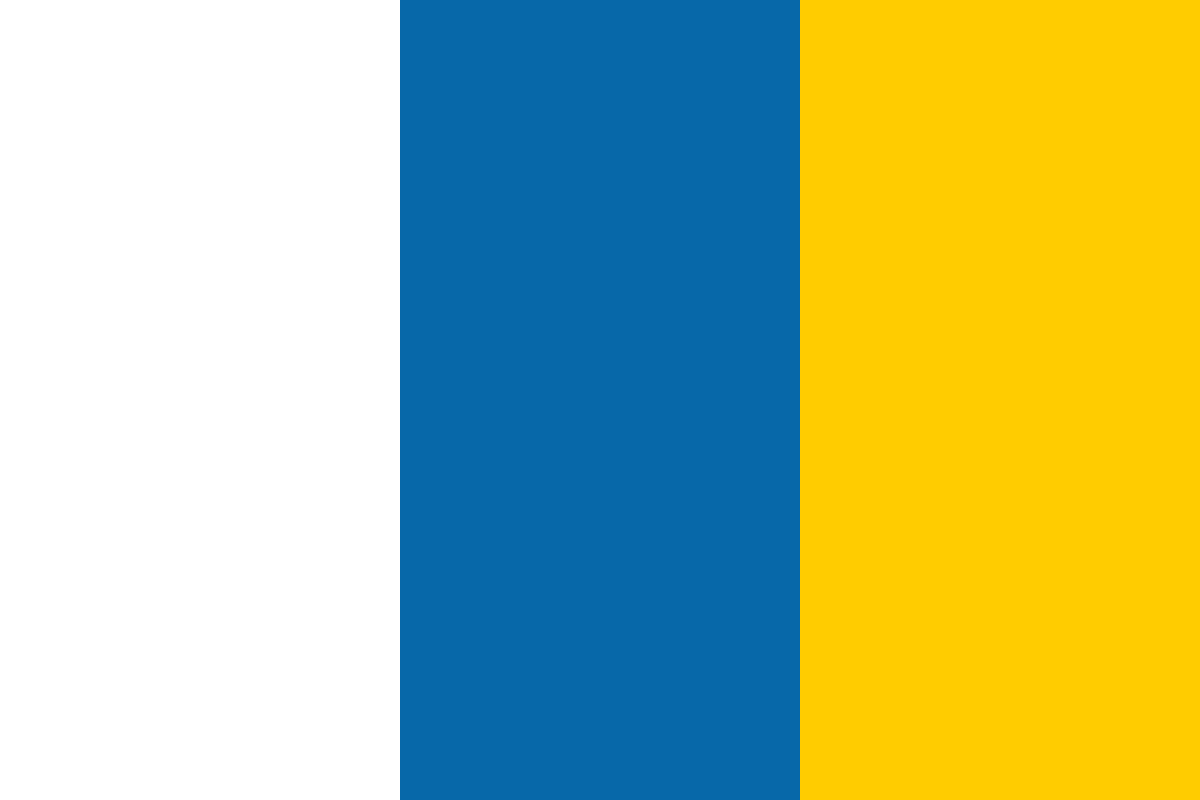

































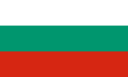








This recipe will teach you how to create a basic HTML5 presentation using reveal.js. We will cover the initial markup and setup. The presentation created here will be used throughout the rest of the book.
Before we start, we need to download the reveal.js script and assets. To do that, simply visit https://github.com/hakimel/reveal.js/downloads and get the latest version of reveal.
Now open your favorite text editor and let's work on the presentation markup.
To create our initial presentation perform the following steps:
reveal.js is a powerful JavaScript library that allows us to create presentations using a web browser. It was created by Hakim El Hattab, a web developer known for his experiments with CSS3 transitions and JavaScript animations.
By performing the preceding steps we created a regular HTML5 presentation. We have used reveal.js default themes and some minor configurations just to get started.
We started with a basic HTML5 structure. It is important to bear in mind that our presentation is, before anything else, an HTML and it should be rendered normally in the browser.
The presentation must follow a structure expected by the reveal.js script. First, our HTML must have one div with reveal as a class attribute. That div must contain another div with slides as a class attribute.
The slides div must contain one or more section elements, each one representing a slide. As we will see in the Creating vertical/nested slides (Simple) recipe, it is possible to nest slides, creating a vertical navigation.
Each slide can contain any HTML or markdown content. We started with just a few elements but we will enrich our presentation through each recipe.
reveal.js comes with multiple themes, available under the css/theme directory. For our initial presentation, we are using the default.css theme. Other options would be beige, night, serif, simple, and sky. Theming will be covered in the Theming (Medium) recipe.
With all the markup and styles set, we initialized the reveal.js JavaScript object by using the initialize method. We used only four options, as follows:
Reveal.initialize({
controls: true,
keyboard: true,
center: true,
transition: 'default'
});
First we enabled the navigation controls at the user interface, setting the controls option to true. We activated keyboard navigation using the arrow keys and also centered the slides' content. The last option sets the transition between slides to default. The next recipe will cover the full list of available options.
reveal.js will work on any browser, but modern browsers are preferred due to animations and 3D effects. We will also need to use external plugins in order to extend our presentation features.
As previously mentioned, reveal.js uses the HeadJS library to manage plugins and dependencies. You can use only reveal.js if you want, but some useful resources (such as speaker notes and zoom) are only enabled using plugins.
The plugins can be loaded through the initialize method and we will see more about them in the Plugins and extensions (Medium) recipe.
While on our presentation, pressing the Esc key will switch to overview mode where you can interact with every slide.
Another useful resource is the blackout mode. If you want to pause and draw attention from your audience, you can press the B key to alternate between normal and blackout modes, and hide your current slide data.
reveal.js will work better on browsers with full support for CSS 3D animations (Chrome, Safari, Firefox, and Internet Explorer 10), but fallbacks (such as 2D transitions) are available for older browsers.
Presentations will not work on Internet Explorer 7 or lower.
In this recipe we have created our initial HTML5 presentation using reveal.js default options and theme.
reveal.js provides an extended API to control our presentation behavior. With reveal.js API methods we can move to previous and next slides, get the current index, toggle overview mode, and more.
To use reveal.js JavaScript API perform the following steps:
reveal.js JavaScript API allows us to control our presentation behavior using JavaScript code. In the preceding example we have used almost all methods available. Let's take a closer look at them:
Option | Description |
Reveal.slide(indexh, indexv,
indexf) |
Slides to the specified index (the indexh
parameter). The first slide will have 0 as index, the second has 1, and so on. You can also specify the vertical index (the indexv parameter) in case of nested slides and the fragment index (the indexf parameter) inside the slide. |
Reveal.left() | Goes to the previous slide in the horizontal line of
the presentation. |
Reveal.right() | Goes to the next slide in the horizontal line of the
presentation. |
Reveal.up() | Changes to the following nested slide. |
Reveal.down() | Returns to the previous nested slide. |
Reveal.prev() | Goes to the previous slide, horizontal or vertical. |
Reveal.next() | Goes to the next slide, horizontal or vertical. |
Reveal.prevFragment() | Switches to the previous fragment inside the
current slide. |
Reveal.nextFragment() | Switches to the next fragment inside the current
slide. |
Reveal.toggleOverview() | Toggles overview mode. |
Reveal.getPreviousSlide() | Returns the DOM element of the previous slide. |
Reveal.getCurrentSlide() | Returns the DOM element of the next slide. |
Reveal.getIndices() | Returns an object with the current indices, for
example {h: 2, v: 1}. |
The preceding methods are available to use in any JavaScript code after the reveal.js object has been initialized. You can either use it directly on elements' attributes (such as onclick) or call it directly from JavaScript functions and listeners.
In this article, the Creating our initial presentation recipe explained how we can create a basic HTML5 presentation and the Using reveal.js JavaScript API recipe explored Reveal.js JavaScript API by creating a custom navigation bar.