










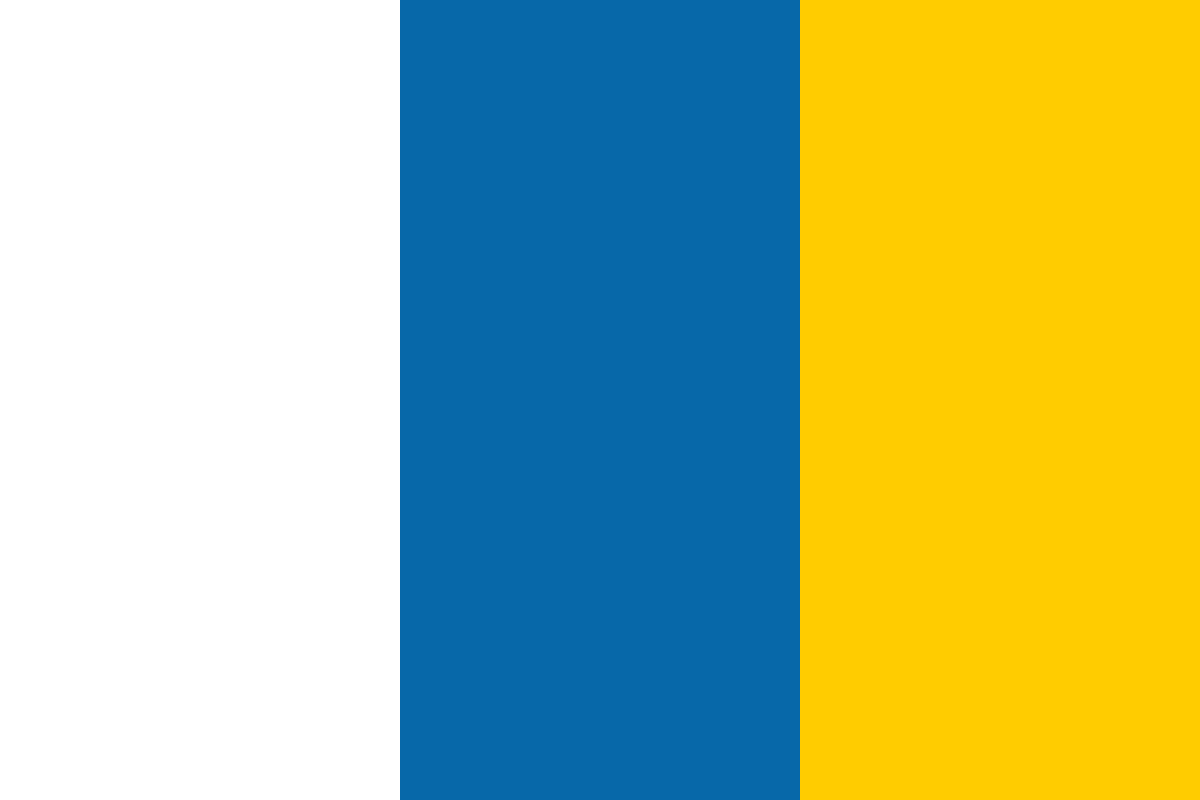

































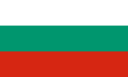








(For more resources on this topic, see here.)
This article is designed to demonstrate the fundamental capabilities of the HTML5 canvas by providing a series of progressively complex tasks. The HTML5 canvas API provides the basic tools necessary to draw and style different types of sub paths including lines, arcs, Quadratic curves, and Bezier curves, as well as a means for creating paths by connecting sub paths. The API also provides great support for text drawing with several styling properties. Let's get started!
When learning how to draw with the HTML5 canvas for the fi rst time, most people are interested in drawing the most basic and rudimentary element of the canvas. This recipe will show you how to do just that by drawing a simple straight line.
Follow these steps to draw a diagonal line:
window.onload = function(){
// get the canvas DOM element by its ID
var canvas = document.getElementById("myCanvas");
// declare a 2-d context using the getContext() method of the
// canvas object
var context = canvas.getContext("2d");
// set the line width to 10 pixels
context.lineWidth = 10;
// set the line color to blue
context.strokeStyle = "blue";
// position the drawing cursor
context.moveTo(50, canvas.height - 50);
// draw the line
context.lineTo(canvas.width - 50, 50);
// make the line visible with the stroke color
context.stroke();
};
<canvas id="myCanvas" width="600" height="250" style="border:1px
solid black;">
</canvas>
As you can see from the preceding code, we need to wait for the page to load before trying to access the canvas tag by its ID. We can accomplish this with the window.onload initializer. Once the page loads, we can access the canvas DOM element with document. getElementById() and we can define a 2D canvas context by passing 2d into the getContext() method of the canvas object. We can also define 3D contexts by passing in other contexts such as webgl, experimental-webgl, and others.
When drawing a particular element, such as a path, sub path, or shape, it's important to understand that styles can be set at any time, either before or after the element is drawn, but that the style must be applied immediately after the element is drawn for it to take effect, We can set the width of our line with the lineWidth property, and we can set the line color with the strokeStyle property. Think of this behavior like the steps that we would take if we were to draw something onto a piece of paper. Before we started to draw, we would choose a colored marker (strokeStyle) with a certain tip thickness (lineWidth).
Now that we have our marker in hand, so to speak, we can position it onto the canvas using the moveTo() method:
context.moveTo(x,y);
Think of the canvas context as a drawing cursor. The moveTo() method creates a new sub path for the given point. The coordinates in the top-left corner of the canvas are (0,0), and the coordinates in the bottom-right corner are (canvas width, canvas height).
Once we have positioned our drawing cursor, we can draw the line using the lineTo() method by defi ning the coordinates of the line's end point:
context.lineTo(x,y);
Finally, to make the line visible, we can use the stroke() method. Unless, otherwise specified, the default stroke color is black.
To summarize, here's the typical drawing procedure we should follow when drawing lines with the HTML5 canvas API:
HTML5 canvas lines can also have one of three varying line caps, including butt, round, and square. The line cap style can be set using the lineCap property of the canvas context. Unless otherwise specified, the line cap style is defaulted to butt. The following diagram shows three lines, each with varying line cap styles. The top line is using the default butt line cap, the middle line is using the round line cap, and the bottom line is using a square line cap:
Notice that the middle and bottom lines are slightly longer than the top line, even though all of the line widths are equal. This is because the round line cap and the square line cap increase the length of a line by an amount equal to the width of the line. For example, if our line is 200 px long and 10 px wide, and we use a round or square line cap style, the resulting line will be 210 px long because each cap adds 5 px to the line length.
When drawing with the HTML5 canvas, it's sometimes necessary to draw perfect arcs. If you're interested in drawing happy rainbows, smiley faces, or diagrams, this recipe would be a good start for your endeavor.
Follow these steps to draw a simple arc:
window.onload = function(){
var canvas = document.getElementById("myCanvas");
var context = canvas.getContext("2d");
context.lineWidth = 15;
context.strokeStyle = "black"; // line color
context.arc(canvas.width / 2, canvas.height / 2 + 40, 80, 1.1 *
Math.PI, 1.9 * Math.PI, false);
context.stroke();
};
<canvas id="myCanvas" width="600" height="250" style="border:1px
solid black;">
</canvas>
We can create an HTML5 arc with the arc() method which is defined by a section of the circumference of an imaginary circle. Take a look at the following diagram:
The imaginary circle is defi ned by a center point and a radius. The circumference section is defi ned by a starting angle, an ending angle, and whether or not the arc is drawn counter-clockwise:
context.arc(centerX,centerY, radius, startingAngle,
endingAngle,counterclockwise);
Notice that the angles start with 0p at the right of the circle and move clockwise to 3p/2, p, p/2, and then back to 0. For this recipe, we've used 1.1p as the starting angle and 1.9p as the ending angle. This means that the starting angle is just slightly above center on the left side of the imaginary circle, and the ending angle is just slightly above center on the right side of the imaginary circle.
The values for the starting angle and the ending angle do not necessarily have to lie within 0p and 2p. In fact, the starting angle and ending angle can be any real number because the angles can overlap themselves as they travel around the circle.
For example, let's say that we defi ne our starting angle as 3p. This is equivalent to one full revolution around the circle (2p) and another half revolution around the circle (1p). In other words, 3p is equivalent to 1p. As another example, - 3p is also equivalent to 1p because the angle travels one and a half revolutions counter-clockwise around the circle, ending up at 1p.
Another method for creating arcs with the HTML5 canvas is to make use of the arcTo() method. The resulting arc from the arcTo() method is defi ned by the context point, a control point, an ending point, and a radius:
context.arcTo(controlPointX1, controlPointY1, endingPointX,
endingPointY, radius);
Unlike the arc() method, which positions an arc by its center point, the arcTo() method is dependent on the context point, similar to the lineTo() method. The arcTo() method is most commonly used when creating rounded corners for paths or shapes.
In this recipe, we'll learn how to draw a Quadratic curve. Quadratic curves provide much more flexibility and natural curvatures compared to its cousin, the arc, and are an excellent tool for creating custom shapes.
Follow these steps to draw a Quadratic curve:
window.onload = function(){
var canvas = document.getElementById("myCanvas");
var context = canvas.getContext("2d");
context.lineWidth = 10;
context.strokeStyle = "black"; // line color
context.moveTo(100, canvas.height - 50);
context.quadraticCurveTo(canvas.width / 2, -50, canvas.width
- 100, canvas.height - 50);
context.stroke();
};
<canvas id="myCanvas" width="600" height="250" style="border:1px
solid black;">
</canvas>
HTML5 Quadratic curves are defined by the context point, a control point, and an ending point:
context.quadraticCurveTo(controlX, controlY, endingPointX,
endingPointY);
Take a look at the following diagram:
The curvature of a Quadratic curve is defined by three characteristic tangents. The first part of the curve is tangential to an imaginary line that starts with the context point and ends with the control point. The peak of the curve is tangential to an imaginary line that starts with midpoint 1 and ends with midpoint 2. Finally, the last part of the curve is tangential to an imaginary line that starts with the control point and ends with the ending point.
If Quadratic curves don't meet your needs, the Bezier curve might do the trick. Also known as cubic curves, the Bezier curve is the most advanced curvature available with the HTML5 canvas API.
Follow these steps to draw an arbitrary Bezier curve:
window.onload = function(){
var canvas = document.getElementById("myCanvas");
var context = canvas.getContext("2d");
context.lineWidth = 10;
context.strokeStyle = "black"; // line color
context.moveTo(180, 130);
context.bezierCurveTo(150, 10, 420, 10, 420, 180);
context.stroke();
};
<canvas id="myCanvas" width="600" height="250" style="border:1px
solid black;">
</canvas>
HTML5 canvas Bezier curves are defined by the context point, two control points, and an ending point. The additional control point gives us much more control over its curvature compared to Quadratic curves:
context.bezierCurveTo(controlPointX1, controlPointY1,
controlPointX2, controlPointY2,
endingPointX, endingPointY);
Take a look at the following diagram:
Unlike Quadratic curves, which are defined by three characteristic tangents, the Bezier curve is defined by five characteristic tangents. The first part of the curve is tangential to an imaginary line that starts with the context point and ends with the fi rst control point. The next part of the curve is tangential to the imaginary line that starts with midpoint 1 and ends with midpoint 3. The peak of the curve is tangential to the imaginary line that starts with midpoint 2 and ends with midpoint 4. The fourth part of the curve is tangential to the imaginary line that starts with midpoint 3 and ends with midpoint 5. Finally, the last part of the curve is tangential to the imaginary line that starts with the second control point and ends with the ending point.