










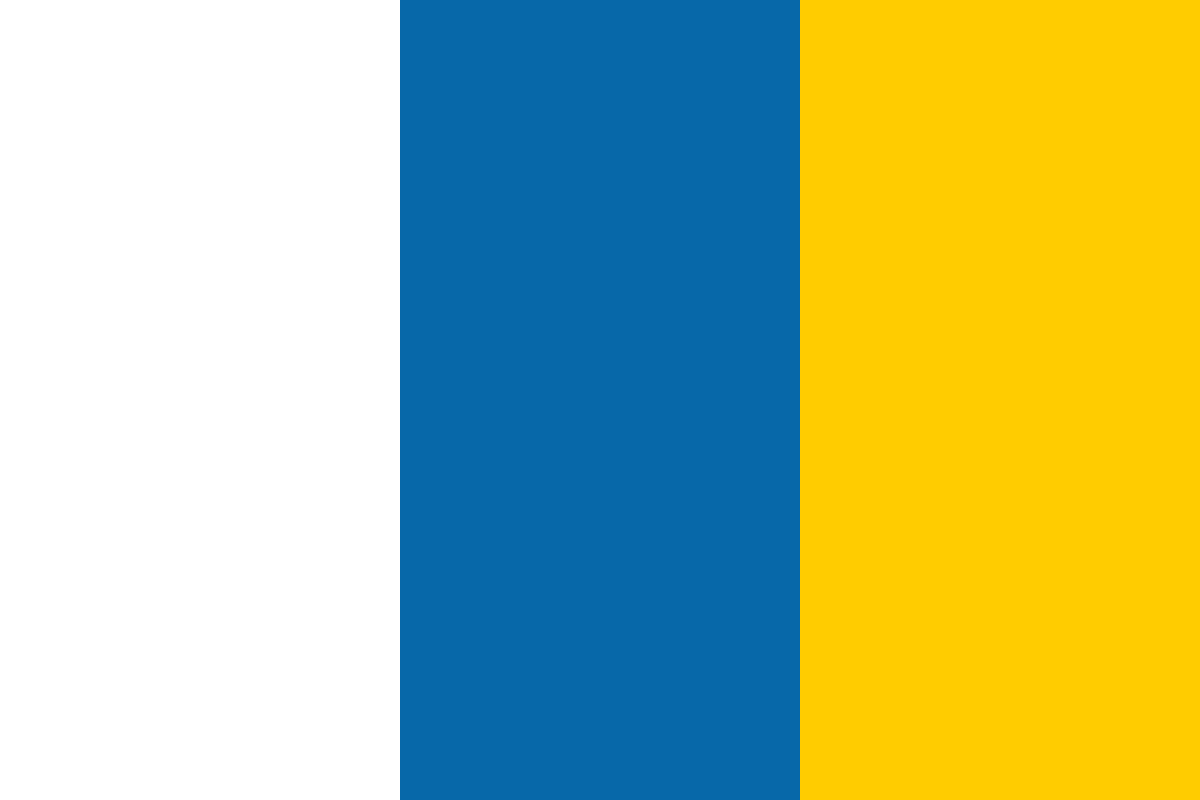

































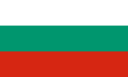








In this post we demonstrate how you can manipulate Lines and Markers in Matplotlib 2.0. It covers steps to plot, customize, and adjust line graphs and markers.
Lines and markers are key components found among various plots. Many times, we may want to customize their appearance to better distinguish different datasets or for better or more consistent styling. Whereas markers are mainly used to show data, such as line plots and scatter plots, lines are involved in various components, such as grids, axes, and box outlines. Like text properties, we can easily apply similar settings for different line or marker objects with the same method.
Most lines in Matplotlib are drawn with the lines class, including the ones that display the data and those setting area boundaries. Their style can be adjusted by altering parameters in lines.Line2D. We usually set color, linestyle, and linewidth as keyword arguments. These can be written in shorthand as c, ls, and lw respectively. In the case of simple line graphs, these parameters can be parsed to the
plt.plot()
function:
import numpy as np
import matplotlib.pyplot as plt
# Prepare a curve of square numbers
x = np.linspace(0,200,100) # Prepare 100 evenly spaced numbers from
# 0 to 200
y = x**2 # Prepare an array of y equals to x squared
# Plot a curve of square numbers
plt.plot(x,y,label = '$x^2$',c='burlywood',ls=('dashed'),lw=2)
plt.legend()
plt.show()
With the preceding keyword arguments for line color, style, and weight, you get a woody dashed curve:
Whether a line will appear solid or with dashes is set by the keyword argument linestyle. There are a few simple patterns that can be set by the linestyle name or the corresponding shorthand. We can also define our own dash pattern:
The cap of dashes can be rounded by setting the dash_capstyle
parameter if we want to create a softer image such as in promotion:
import numpy as np
import matplotlib.pyplot as plt
# Prepare 6 lines
x = np.linspace(0,200,100)
y1 = x*0.5
y2 = x
y3 = x*2
y4 = x*3
y5 = x*4
y6 = x*5
# Plot lines with different dash cap styles
plt.plot(x,y1,label = '0.5x', lw=5, ls=':',dash_capstyle='butt')
plt.plot(x,y2,label = 'x', lw=5, ls='--',dash_capstyle='butt')
plt.plot(x,y3,label = '2x', lw=5, ls=':',dash_capstyle='projecting')
plt.plot(x,y4,label = '3x', lw=5, ls='--',dash_capstyle='projecting')
plt.plot(x,y5,label = '4x', lw=5, ls=':',dash_capstyle='round')
plt.plot(x,y6,label = '5x', lw=5, ls='--',dash_capstyle='round')
plt.show()
Looking closely, you can see that the top two lines are made up of rounded dashes. The middle two lines with projecting capstyle have closer spaced dashes than the lower two with butt one, given the same default spacing:
A marker is another type of important component for illustrating data, for example, in scatter plots, swarm plots, and time series plots.
There are two groups of markers, unfilled markers and filled_markers.
The full set of available markers can be found by calling Line2D.markers
, which will output a dictionary of symbols and their corresponding marker style names. A subset of filled markers that gives more visual weight is under Line2D.filled_markers
.
Here are some of the most typical markers:
'o' : Circle
'x' : Cross
'+' : Plus sign
'P' : Filled plus sign
'D' : Filled diamond
'S' : Square
'^' : Triangle
Here is a scatter plot of random numbers to illustrate the various marker types:
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.lines import Line2D
# Prepare 100 random numbers to plot x = np.random.rand(100)
y = np.random.rand(100)
# Prepare 100 random numbers within the range of the number of
# available markers as index
# Each random number will serve as the choice of marker of the
# corresponding coordinates
markerindex = np.random.randint(0, len(Line2D.markers), 100)
# Plot all kinds of available markers at random coordinates
# for each type of marker, plot a point at the above generated
# random coordinates with the marker type
for k, m in enumerate(Line2D.markers):
i = (markerindex == k)
plt.scatter(x[i], y[i], marker=m)
plt.show()
The different markers suit different densities of data for better distinction of each point:
We often want to change the marker sizes so as to make them clearer to read from a slideshow. Sometimes we need to adjust the markers to have a different numerical value of marker size to:
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.ticker as ticker
# Prepare 5 lines
x = np.linspace(0,20,10)
y1 = x
y2 = x*2
y3 = x*3
y4 = x*4
y5 = x*5
# Plot lines with different marker sizes
plt.plot(x,y1,label = 'x', lw=2, marker='s', ms=10) # square size 10
plt.plot(x,y2,label = '2x', lw=2, marker='^', ms=12) # triangle size 12
plt.plot(x,y3,label = '3x', lw=2, marker='o', ms=10) # circle size 10
plt.plot(x,y4,label = '4x', lw=2, marker='D', ms=8) # diamond size 8
plt.plot(x,y5,label = '5x', lw=2, marker='P', ms=12) # filled plus sign
# size 12
# get current axes and store it to ax
ax = plt.gca()
plt.show()
After tuning the marker sizes, the different series look quite balanced:
If all markers are set to have the same markersize value, the diamonds and squares may look heavier:
Thus, we learned how to customize lines and markers in a Matplotlib plot for better visualization and styling. To know more about how to create and customize plots in Matplotlib, check out this book Matplotlib 2.x By Example.