










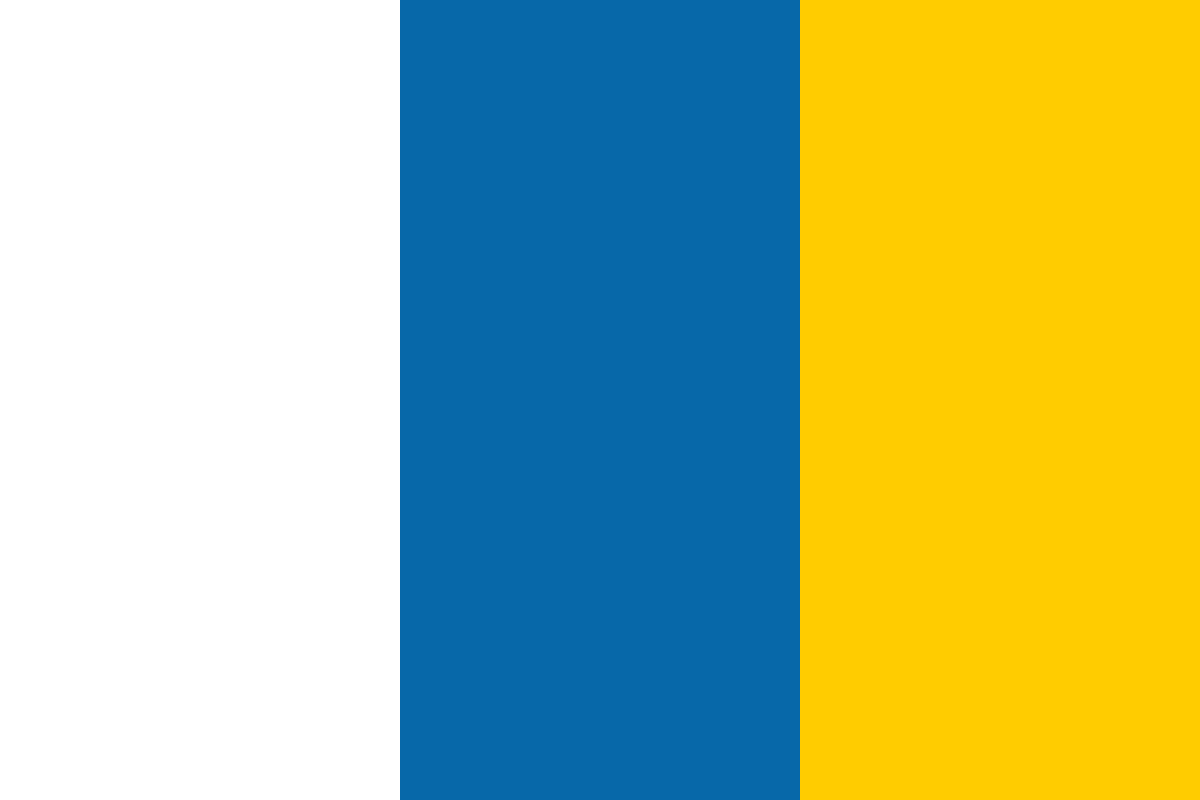

































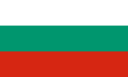








In Part 1 of this series, we got everything in place for our Koa app using Jade and Mongel. In this post, we will cover Jade templates and how to use listing and viewing pages. Please note that this series requires that you use Node.js version 0.11+.
Rendering HTML is always an important part of any web application. Luckily, when using Node.js there are many great choices, and for this article we’ve chosen Jade. Keep in mind though that we will only touch on a tiny fraction of the Jade functionality. Let’s create our first Jade template. Create a file called create.jade and put in the following:
create.jade
doctype html
html(lang='en')
head
title Create Page
body
h1 Create Page
form(method='POST', action='/create')
input(type='text', name='title', placeholder='Title')
input(type='text', name='contents', placeholder='Contents')
input(type='submit')
For all the Jade questions you have that we won’t answer in this series, I refer you to the excellent official Jade website at http://jade-lang.com .
If you add the following statement app.listen(3000); to the end of index.js, then you should be able to run the program from your terminal using the following command and by visiting http://localhost:3000 in your browser.
$ node --harmony index.js
The --harmony flag just tells the node program that we need support for generators in our program:
Now that we can create a page in our MongoDB database, it is time to actually list and view these pages. For this purpose we need to add another middleware to our index.js file after the first middleware:
app.use(function* () {
if (this.method != 'GET') {
this.status = 405;
this.body = 'Method Not Allowed';
return
}
…
});
As you can probably already tell, this new middleware is very similar to the first one we added that handled the creation of pages. At first we make sure that the method of the request is GET, and if not, we respond appropriately and return the following:
var params = this.path.split('/').slice(1);
var id = params[0];
if (id.length == 0) {
var pages = yield Page.find();
var html = jade.renderFile('list.jade', { pages: pages });
this.body = html;
return
}
Then, we proceed to inspect the path attribute of the Koa context, looking for an ID that represents the page in the database. Remember how we redirected using the ID in the previous middleware. We inspect the path by splitting it into an array of strings separated by the forward slashes of a URL; this way the path /1234 becomes an array of ‘’ and ‘1234.’ Because the path starts with a forward slash, the first item in the array will always be the empty string, so we just discard that by default.
Then we check the length of the ID parameter, and if it’s zero we know that there is in fact no ID in the path, and we should just look for the pages in the database and render our list.jade template with those pages made available to the template as the variable pages. Making data available in templates is also known as providing locals to the template.
list.jade
doctype html
html(lang="en")
head
title Your Web Application
body
h1 Your Web Application
ul
- each page in pages
li
a(href='/#{page._id}')= page.title
But if the length of id was not zero, we assume that it’s an id and we try to load that specific page from the database instead of all the pages, and we proceed to render our view.jade template with the:
var page = yield Page.findById(id);
var html = jade.renderFile('view.jade', page);
this.body = html;
view.jade
doctype html
html(lang="en")
head
title= title
body
h1= title
p= contents
You should now be able to run the app as previously described and create a page, list all of your pages, and view them. If you want to, you can continue and build a simple CMS system.
Koa is very simple to use and doesn’t enforce a lot of functionality on you, allowing you to pick and choose between libraries that you need and want to use. There are many possibilities and that is one of Koa’s biggest strengths.
Find even more Node.js content on our Node.js page. Featuring our latest titles and most popular tutorials, it's the perfect place to learn more about Node.js.
Christoffer Hallas is a software developer and entrepreneur from Copenhagen, Denmark. He is a computer polyglot and contributes to and maintains a number of open source projects. When not contemplating his next grand idea (which remains an idea), he enjoys music, sports, and design of all kinds. Christoffer can be found on GitHub as hallas and at Twitter as @hamderhallas.