










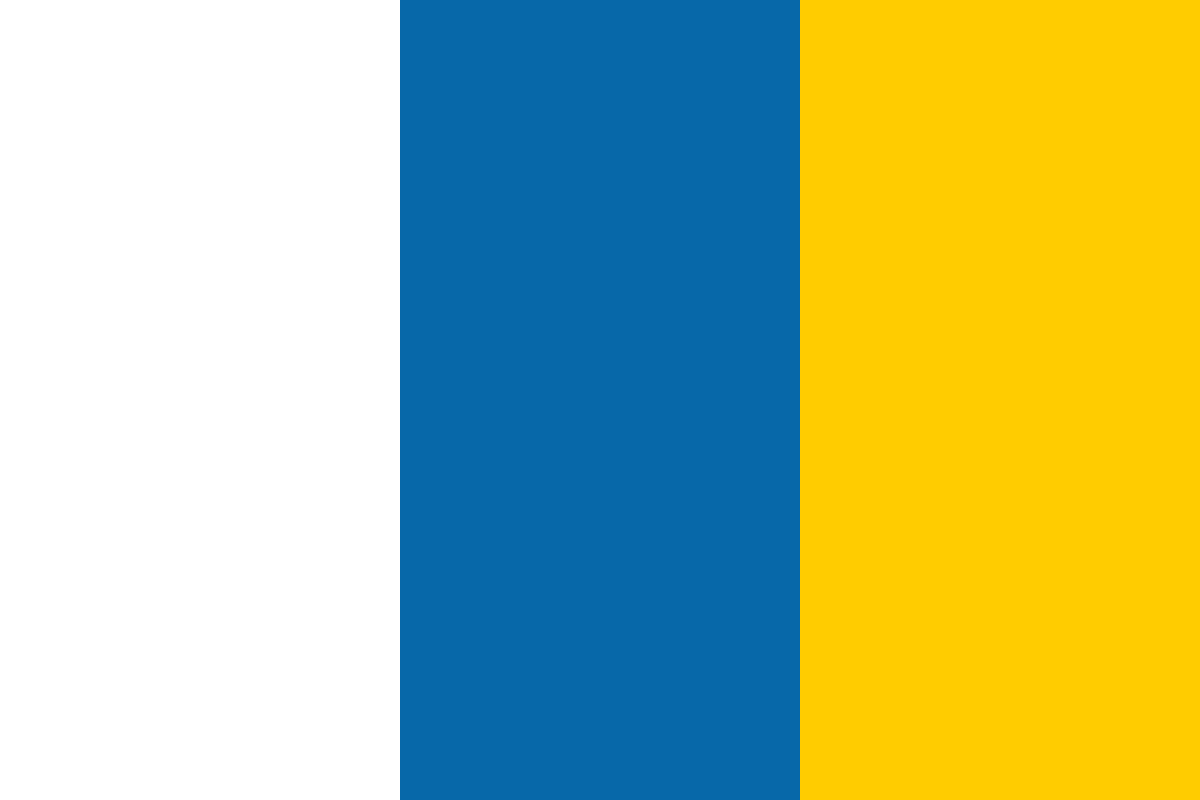

































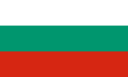








The Godot Engine is a piece of open source software designed to help you make any kind of game. It possesses a great number of features to ease the work flow of game development. This two-part article will cover some basic features of the engine—such as physics and scripting—by showing you how to make a game with the mechanics of the classic Breakout.
To install Godot, you can download it from the website and extract it in your place of preference. You can also install it through Steam. While the latter has a larger download, it has all the demos and export templates already installed.
When you first open Godot, you are presented with the Project Manager window. In here, you can create new or import existing projects. It's possible to run a project directly from here without opening the editor itself. The Templates tab shows the available projects in the online Asset Library where you can find and download community-made content. Note that there might also be a console window, which shows some messages of what's happening on the engine, like warnings and error messages. This window must remain open but it's also helpful for debugging. It will not show up in your final exported version of the game.
Project Manager
To start creating our game, let's click on the New Project button. Using the dialog interface, navigate to where you want to place it, and then create a new folder just for the project. Select it and choose a name for the project ("Breakout" may be a good choice). Once you do that, the new project will be shown at the top of the list. You can double-click to open it in the editor.
You will see first the main screen of the Godot editor. I rearranged the docks to suit my preferences, but you can leave the default one or change it to something you like. If you click on the Settings button at the top-right corner, you can save and load layouts. Godot uses a system in which every object is a Node. A Scene is just a tree of nodes and can also be used as a "prefab", like other engines call it. Every scene can be instanced as a part of another scene. This helps in dividing the project and reusing the work. This is all explained in the documentation and you can consult it if you have any doubt.
Main screen
Now we are going to create a scene for the paddle, which can later be instanced on the game scene. I like to start with an object that can be controlled by the player so that we can start to feel what the interactivity looks like.
On the Scene dock, click on the "+" (plus) button to add a new Node (pressing Ctrl + A will also work). You'll be presented with a large collection of Nodes to choose from, each with its own behavior. For the paddle, choose a StaticBody2D. The search field can help you find it easily. This will be root of the scene. Remember that a scene is a tree of nodes, so it needs a "root" to be its main anchor point.
You may wonder why we chose a static body for the paddle since it will move. The reason for using this kind of node is that we don't want it to be moved by physical interaction. When the ball hits the paddle, we want it to be kept in the same place. We will move it only through scripting.
Select the node you just added in the Scene dock and rename it to Paddle. Save the scene as paddle.tscn. Saving often is good to avoid losing your work. Add a new node of the type Sprite (not Sprite3D, since this is a 2D game). This is now a child of the root node in the tree. The sprite will serve as the image of the paddle. You can use the following image in it:
Paddle
Save the image in the project folder and use the FileSystem dock in the Godot editor to drag and drop into the Texture property on the Inspector dock. Any property that accepts a file can be set with drag and drop.
Now the Static Body needs a collision area so that it can tell where other physics objects (like the ball) will collide. To do this, select the Paddle root node in the Scene dock and add a child node of type CollisionShape2D to it. The warning icon is there because we didn't set a shape to it yet, so let's do that now. On the Inspector dock, set the Shape property to a New RectangleShape2D. You can set the shape extents visually using the editor. Or you can click on the ">" button just in front of the Shape property on the Inspector to edit its properties and set the extents to (100, 15) if you're using the provided image. This is the half-extent, so the rectangle will be doubled in each dimension based on what you set here.
While our paddle is mostly done, it still isn't controllable. Before we delve into the scripting world, let's set up the Input Map. This is a Godot functionality that allows us to abstract user input into named actions. You can later modify the keys needed to move the player later without changing the code. It also allows you to use multiple keys and joystick buttons for a single action, making the game work on keyboard and gamepad seamlessly.
Click on the Project Settings option under the Scene menu in the top left of the window. There you can see the Input Map tab. There are some predefined actions, which are needed by UI controls. Add the actions move_left and move_right that we will use to move the paddle. Then map the left and right arrow keys of the keyboard to them. You can also add a mapping to the D-pad left and right buttons if you want to use a joystick. Close this window when done.
Now we're ready to do some coding. Right-click on the Paddle root node in the Scene dock and select the option Add Script from the context menu. The "Create Node Script" dialog will appear and you can use the default settings, which will create a new script file with the same name as the scene (with a different extension). Godot has its own script language called "GDScript", which has a syntax a bit like Python and is quite easy to learn if you are familiar with programming. You can use the following code on the Paddle script:
extends StaticBody2D
# Paddle speed in pixels per second
export var speed = 150.0
# The "export" keyword allows you to edit this value from the Inspector
# Holds the limits off the screen
var left_limit = 0
var right_limit = 0
# This function is called when this node enters the game tree
# It is useful for initialization code
func _ready():
# Enable the processing function for this node
set_process(true)
# Set the limits to the paddle based on the screen size
left_limit = get_viewport_rect().pos.x + (get_node("Sprite").get_texture().get_width() / 2)
right_limit = get_viewport_rect().pos.x + get_viewport_rect().size.x - (get_node("Sprite").get_texture().get_width() / 2)
# The processing function
func _process(delta):
var direction = 0
if Input.is_action_pressed("move_left"):
# If the player is pressing the left arrow, move to the left
# which means going in the negative direction of the X axis
direction = -1
elif Input.is_action_pressed("move_right"):
# Same as above, but this time we go in the positive direction
direction = 1
# Create a movement vector
var movement = Vector2(direction * speed * delta, 0)
# Move the paddle using vector arithmetic
set_pos(get_pos() + movement)
# Here we clamp the paddle position to not go off the screen
if get_pos().x < left_limit:
set_pos(Vector2(left_limit, get_pos().y))
elif get_pos().x > right_limit:
set_pos(Vector2(right_limit, get_pos().y))
If you play the scene (using the top center bar or pressing F6), you can see the paddle and move it with the keyboard. You may find it too slow, but this will be covered in part two of this article when we set up the game scene.
You now have a project set up and a paddle on the screen that can be controlled by the player. You also have some understanding of how the Godot Engine operates with its nodes, scenes, and scripts. In part two, you will learn how to add the ball and the destroyable bricks.
George Marques is a Brazilian software developer who has been playing with programming in a variety of environments since he was a kid. He works as a freelancer programmer for web technologies based on open source solutions such as WordPress and Open Journal Systems. He's also one of the regular contributors of the Godot Engine, helping solving bugs and adding new features to the software, while also giving solutions to the community for the questions they have.