










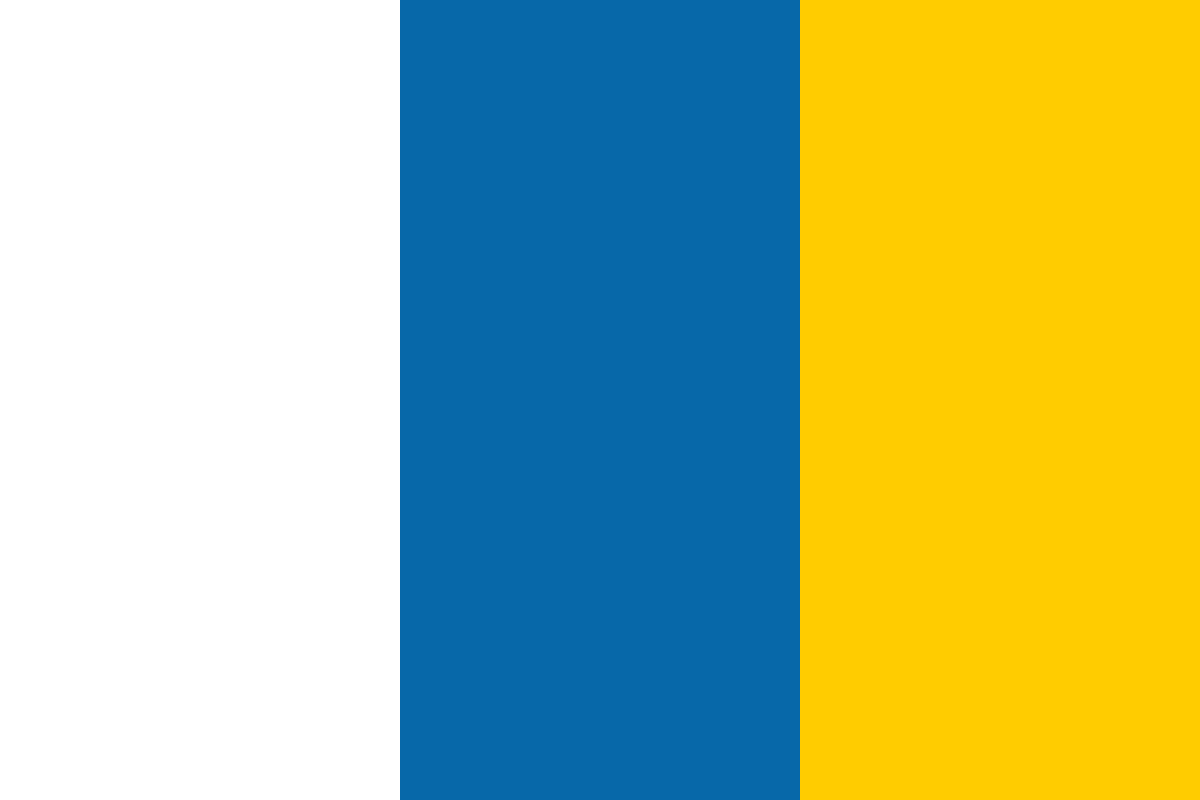

































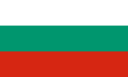








The main reason for the simplicity of our Hello World example was that it neither took in any information, nor passed any information out—the rule always fired, and said the same thing. In real life, we need to pass information between our rules and the rest of the system. You may remember that in our tour of the Guvnor, we came across models that solved this problem of 'How do we get information into and out of the rules?'.
Here is the spreadsheet that we will use as an example in this article:
If we want to duplicate this in our model/JavaBean, we would need places to hold four key bits of sales-related information.
We also need a description for this group of information that is useful when we have many spreadsheets/models in our system (similar to the way this spreadsheet tab is called Sales).
Note that one JavaBean (model) is equal to one line in the spreadsheet. Because we can have multiple copies of JavaBeans in memory, we are able to represent the many lines of information that we have in a spreadsheet. Later, we'll loop and add 10, 100, or 1000 lines (that is, JavaBeans) of information into Drools (for as many lines as we need). As we loop, adding them one at a time, the various rules will fire as a match is made.
We will now build this model in Java using the Eclipse editor. We're going to do it step-by-step.
When you've finished entering the details, click on Finish. You will be redirected to the main screen, with a new project (SalesModel) created. If you can't see the project, try opening either the Package or the Navigator tab.
When you can see the project name, right-click on it. From the menu, choose New | Package. The New Java Package dialog will be displayed, as shown below. Enter the details as per the screenshot to create a new package called org.sample, and then click on Finish.
If you are doing this via the navigator (or you can take a peek via Windows Explorer), you'll see that this creates a new folder org, and within it a subfolder called sample. Now that we've created a set of folders to organize our JavaBeans, let's create the JavaBean itself by creating a class.
To create a new Java class, expand/select the org.sample package (folder) that we created in the previous step. Right-click on it and select New Class. Fill in the dialog as shown in the following screenshot, and then click on Finish:
We will now be back in the main editor, with a newly created class called Sales.java (below). For the moment, there isn't much there—it's akin to two nested folders (a sample folder within one called org) and a new (but almost empty) file / spreadsheet called Sales.
package org.sample;
public class Sales {
}
By itself, this is not of much use. We need to tell Java about the information that we want our class (and hence the beans that it creates) to hold. This is similar to adding new columns to a spreadsheet.
Edit the Java class until it looks something like the code that follows (and take a quick look of the notes information box further down the page if you want to save a bit of typing). If you do it correctly, you should have no red marks on the editor (the red marks look a little like the spell checking in Microsoft Word).
package org.sample;
import java.util.Date;
public class Sales {
private String name;
private long sales;
private Date dateOfSale;
private boolean chocolateOnlyCustomer;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public long getSales() {
return sales;
}
public void setSales(long sales) {
this.sales = sales;
}
public Date getDateOfSale() {
return dateOfSale;
}
public void setDateOfSale(Date dateOfSale) {
this.dateOfSale = dateOfSale;
}
public boolean isChocolateOnlyCustomer() {
return chocolateOnlyCustomer;
}
public void setChocolateOnlyCustomer(boolean choclateOnlyCustomer) {
this.chocolateOnlyCustomer = chocolateOnlyCustomer;
}
}
Believe it or not, this piece of Java code is almost the same as the Excel Spreadsheet we saw at the beginning of the article. If you want the exact details, let's go through what it means line by line.
The get and set lines (in the previous code) are known as accessor methods. They control access to hidden or private fields. They're more complicated than may seem necessary for our simple example, as Java has a lot more power than we're using at the moment.
Luckily, Eclipse can auto-generate these for us. (Right-click on the word Sales in the editor, then select Source | Generate Getters and Setters from the context menu. You should be prompted for the Accessor methods that you wish to create.)
Once you have the text edited like the sample above, check again that there are no spelling mistakes. A quick way to do this is to check the Problems tab in Eclipse (which is normally at the bottom of the screen).
Now that we've created our model in Java, we need to export it so that we can use in the Guvnor.