










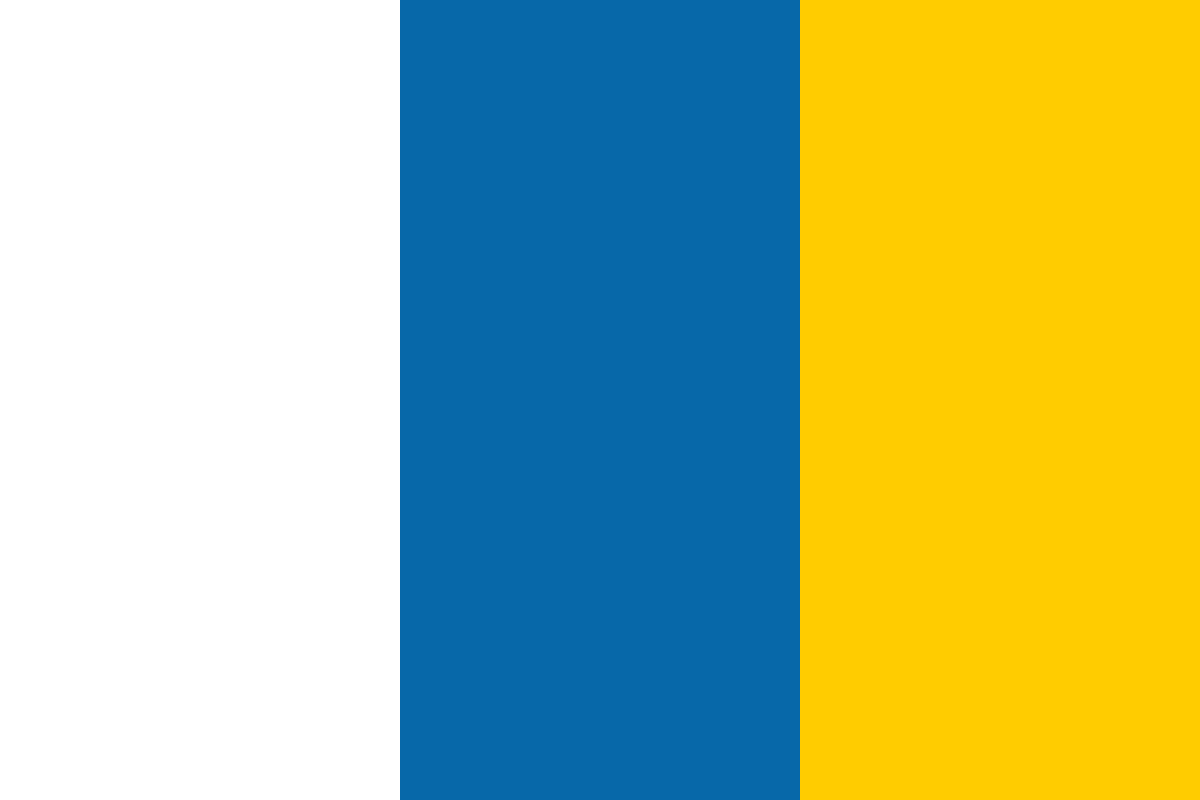

































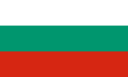








The public class fields syntax aims to simplify the class definition. This will also eliminate the need of constructor just to define some fields. Here’s how you can implement a class as per this syntax:
class IncreasingCounter {
_count = 0;
get value() {
console.log('Getting the current value!');
return this._count;
}
increment() {
this._count++;
}
}
A field in JavaScript is marked as private using _. This makes the field hidden by convention, but in fact, it is fully public. To make the fields private we can mark them with #, which means that they will not be accessible outside of the class body.
class IncreasingCounter {
#count = 0;
get value() {
console.log('Getting the current value!');
return this.#count;
}
increment() {
this.#count++;
}
}
Though in JavaScript, there is a need for some kind of mechanism to provide private or controlled access beyond closures, this proposal seems very sloppy as some developers believe. One of the Hacker News users said, “This syntax is...odd. It is not expressive and comes off as a "language hack." The only people who would understand it are those who happen to stumble on this article.“
Read more in detail on Google Developers and also check out the proposal on GitHub.
4 key findings from The State of JavaScript 2018 developer survey
Why use JavaScript for machine learning?
V8 JavaScript Engine releases version 6.9!