










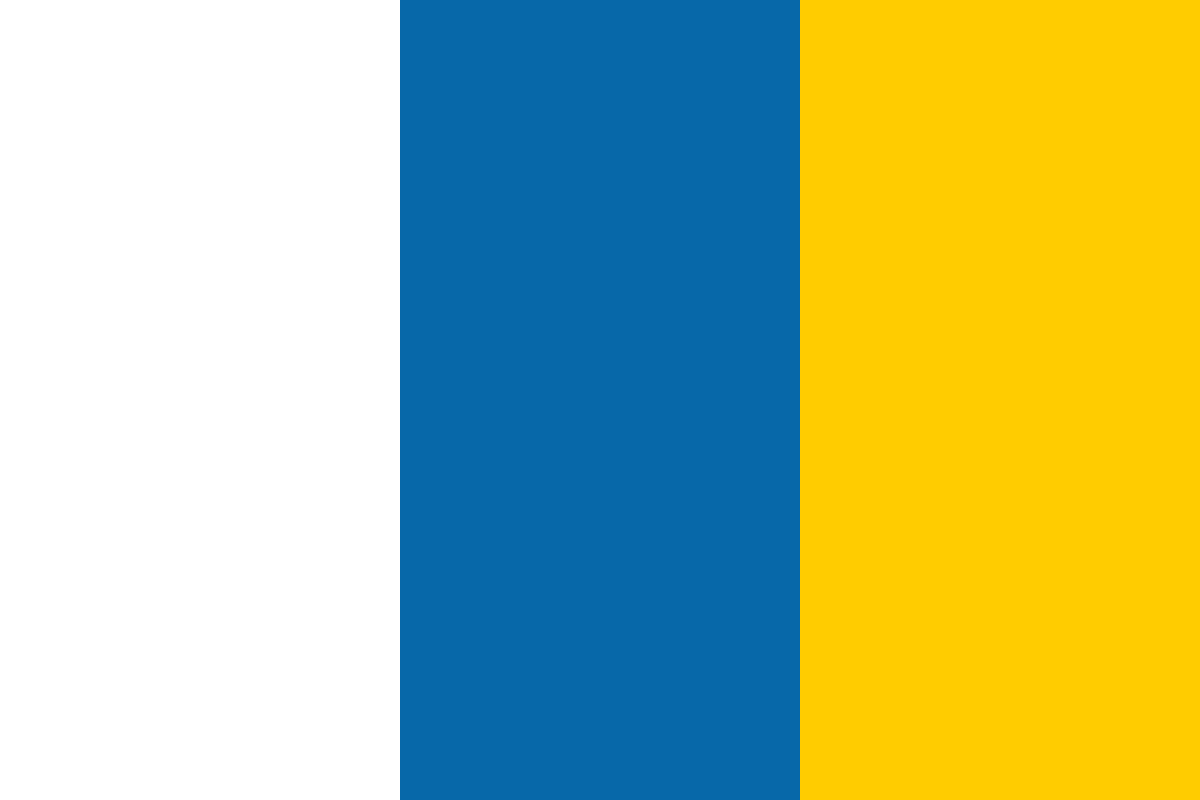

































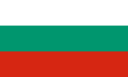








Kotlin has been gaining more and more attention recently. With its 1.1 release, the language has proved both stable and usable.
In this article we’re going to cover a few different things: what is Kotlin and why it’s become popular, how to get started, where it’s most effective, and where to go next if you want to learn more.
Kotlin is a programing language. Much like Scala or Groovy.It is a language that targets the JVM. It is developed by JetBrains, who make the IDEs that you know and love so much. The same diligence and finesse that is put into IntelliJ, PyCharm, Resharper, and their many other tools also shines through with Kotlin.
There are two secret ingredients that make Kotlin such a joy to use. First, since Kotlin compiles to Java bytecode, it is 100% interoperable with your existing Java code. Second, since JetBrains has control over both language and IDE, the tooling support is beyond excellent.
Here’s a quick example of some interoperability between Java and Kotlin:
Person.kt
data class Person(
val title: String?, // String type ends with “?”, so it is nullable.
val name: String, // val's are immutable. “String” field, so non-null.
var age: Int// var's are mutable.
)
PersonDemo.java
Person person = new Person("Mr.", "John Doe", 23);
person.getAge(); // data classes provide getter and setters automatically.
person.toString(); // ... in addition to toString, equals, hashCode, and copy methods.
The above example shows a “data class” (think Value Object / Data Transfer Object) in Kotlin being used by a Java class. Not only does the code work seamlessly, but also the JetBrains IDE allows you to navigate, auto-complete, debug, and refactor these together without skipping a beat. Continuing on with the above example, we’ll show how you might want to filter a list of Person objects using Kotlin.
Filters.kt
fun demoFilter(people: List<Person>) : List<String> {
return people
.filter { it.age>35 }
.map { it.name}
}
FiltersApplied.java
List<String> names = new Filters().demoFilter(people);
The simple addition of higher order functions (such as map, filter, reduce, sum, zip, and so on) in Kotlin greatly reduces the boilerplate code you usually have to write in Java programs when iterating through collections. The above filtering code in Java would require you to create a temporary list of results, iterate through people, perform an if check on age, add name to the list, then finally return the list. The above Kotlin version is not only 1 line, but it can actually be reduced even further since Kotlin supports Single Expression Functions:
fun demoFilter(people: List<Person>) = people.filter{ it.age>35 }.map { it.name } // return type and return keyword replaced with “=”.
Another Kotlin feature that greatly reduces the boilerplate found in Java code comes from its more advanced type system that treats nullable types differently from non-null types.
The Java version of:
if (people != null && !people.isEmpty() &&people.get(0).getAddress() != null &&people.get(0).getAddress().getStreet() != null) {
return people.get(0).getAddress().getStreet();
}
Using Kotlin’s “?” operator that checks for null before continuing to evaluate an expression, we can simplify this statement drastically:
return people?.firstOrNull()?.address?.street
Not only does this reduce the verbosity inherent in Java, but it also helps to eliminate the “Billion dollar mistake” in Java: NullPointerExceptions. The ability to mark a type as either nullable or not-null (Address? vs Address) means that the compiler can ensure null checks are properly done at compile time, not at runtime in production.
These are just a couple of examples of how Kotlin helps to both reduce the number of lines in your code and also reduce the often unneeded complexity. The more you use Kotlin, the more of these idioms you will find hard to start living without.
More than any other industry, Kotlin has gained the most ground with Android developers. Since Kotlin compiles to Java 6 bytecode, it can be used to build Android applications. Since the interoperability between Kotlin and Java is so simple, it can be slowly added into a larger Java Android project over time (or any Java project for that matter). Given that Android developers do not yet have access to the nice features of Java 8, Kotlin provides these and gives Android developers many of the new language features they otherwise can only read about. The Kotlin team realized this early on and has provided many libraries and tools targeted at Android devs. Here are a couple short examples of how Kotlin can simplify working with the Android SDK:
context.runOnUiThread{
...
}
button?.setOnClickListener{
Toast.makeText(...)
}
Android developers will immediately understand the reduced boilerplate here. If you are not an Android developer, trust me, this is much better.
If you are an Android developer I would suggest to you take a look at both Kotlin Android Extensions and Anko.
The last feature of Kotlin we will look at today is one of the most useful features. This is the ability to write Extension Functions. Think of these as the ability to add your own method to any existing class. Here is a quick example of extending Java’s String class to add a prepend method:
fun String.prepend(str: String) = str + this
Once imported, you can use this from any Kotlin code as though it were a method on String.
Consider the ability to extend any system or framework class. Suddenly all of your utility methods become extension methods and your code starts to look intentionally designed instead of patched together.
Maybe you’d like to add a dpToPx() method on your Android Context class. Or, maybe you’d like to add a subscribeOnNewObserveOnMain() method on your RxJava Observable class. Well, now you can.
If you’re interested in trying Kotlin, grab a copy of the IntelliJ IDEA IDE or Android Studio and install the Kotlin plugin to get started. There is also a very well built online IDE maintained by JetBrains along with a series of exercises called Kotin Koans. These can be found at http://try.kotlinlang.org/.
For more information on Kotlin, check out https://kotlinlang.org/ .
Brent Watson is an Android engineer in NYC. He is a developer, entrepreneur, author, TEDx speaker, and Kotlin advocate. He can be found at http://brentwatson.com/.