










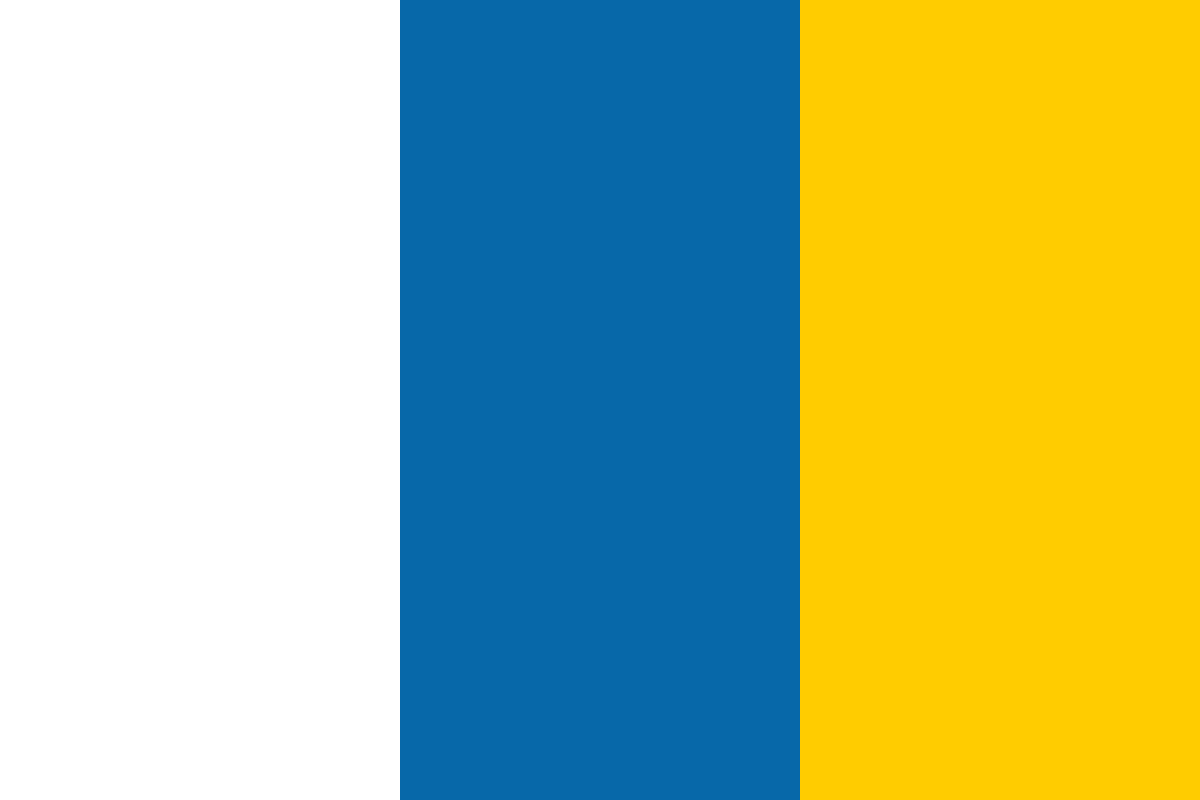

































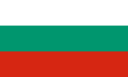








Firebase is a real-time database for your web or mobile app. Data in your Firebase is stored as JSON and synchronized in real time to every connected client. This makes it really easy to share a state between clients. Firebase is a great fit for cross-platform applications and especially collaborative applications. However, you can use it for almost anything.
Firebase is a NoSQL database. This is a great fit for many web and mobile applications, but as with any NoSQL store, we need to be mindful when structuring related data.
Firebase is also SSL by default, which is great!
In this post, we will take a look at building a basic web application using Firebase as our backend.
Our app will be an SPA (Single Page Application) and we will leverage modern JavaScript tooling. We will be using npm as a package manager, babel for ES2015+ transpilation, and browserify for bundling together our scripts.
Let's get started. Use the following command to create a folder:
$ mkdir fbapp && cd fbapp
$ npm init
$ npm install --save-dev budo
$ npm install --save-dev babelify
We have now made a folder for our app fbapp. Inside there we have created a new project using npm init (defaults are fine) and we have installed some packages. I didn't mention budo before, but it is a great browserify development server.
Before we can start talking with our Firebase, we need to head over to https://www.firebase.com/ and sign up for an account. No credit card required. Firebases have quite a bit of free storage and data transfer before they start charging, so it's great for getting started.
Once you are logged in, create a new Firebase.
Notice the APP URL https://<name>.firebaseio.com/ depending on what you named your Firebase. We are going to use that url in a second.
Click your newly created firebase will navigate to the administration UI for this Firebase.
Now that we have created a Firebase, it's time to start talking to it. First, we need to install the firebase library using npm:
$ npm install --save firebase
$ touch index.js
I also created a file named index.js. This will be the entrypoint for our app. Open index.js in your favorite editor. It's time to hack:
import Firebase from 'firebase/lib/firebase-web'
let ref = new Firebase('https://<name>.firebaseio.com/')
ref.set({ counter : 0 })
console.log('wuhu')
The firebase npm package includes both node.js and browser libraries for Firebase. Since we are going to use our app in the browser, we need to import those libraries.
Now we can run that application in a browser using budo (browserify under the hood).
budo index.js --live -- -t babelify
Navigate to http://localhost:9966/ and verify that you can see wuhu printed in the console (developer tools).
Notice the --live parameter we pass to budo. It automatically enables livereload for the bundle. If you're not familiar with it, get familiar with it!
Next, try and navigate to the Firebase admin UI and verify that it now has a property counter set to 0.
Now, let's try to read this data back. Since Firebase is a real-time database it pushes data to us. So, we need to set up listeners.
Also, remember to remove the ref.set line from your code as it would reset this property every time someone loads our page and we do not want that.
import Firebase from 'firebase/lib/firebase-web'
let ref = new Firebase('https://<name>.firebaseio.com/')
ref.child('counter').on('value', (snap) => {
console.log(snap.val())
})
Navigate to http://localhost:9966 and verify that 0 is printed in the console.
Now, let's see if we can make this counter "useful":
import Firebase from 'firebase/lib/firebase-web'
let ref = new Firebase('https://getting-started.firebaseio.com/')
let counter = 0
ref.child('counter').on('value', (snap) => {
counter = snap.val()
render(counter)
})
let render = (counter) => {
if (!button) createNodes()
else buttonText.nodeValue = "Click Me ("+counter+")"
}
let increaseCounter = () => {
ref.child('counter').set(counter+1)
}
let button, buttonText;
let createNodes = () => {
button = document.createElement('button')
buttonText = document.createTextNode("Click Me")
button.appendChild(buttonText)
button.addEventListener('click', increaseCounter)
document.body.appendChild(button)
}
render()
Notice the render and increaseCounter functions. Whenever the counter property gets updated on Firebase, the render function is called. Whenever we click on the button increaseCounter is called, setting the value on Firebase which in turn triggers render. This is a typical way of working with Firebase. You use it both as a database and as an event source.
Navigate your browser to http://localhost:9966 again. Click the button a few times and watch the counter increase. Then, open another browser window and navigate to the same URL. Have the browser windows side-by-side so you can see them both at the same time, then click one of the buttons. It's a little bit like magic isn't it?
Once you get familiar with the way Firebase works it is a really great store for your data. It encourages event driven design and functional code. It frees you from manually coordinating state between different parts of your application. Firebase holds your state, so all you have to do is listen.
Asbjørn Enge is a software enthusiast living in Sandes, Norway. He is passionate about free software and the web. His interests includes the engineering and designing of web clients, APIs, and server side services, as well as cloud architecture and devops. He cares about modular design, simplicity and readability. He can be found on Twitter @asbjornenge.