










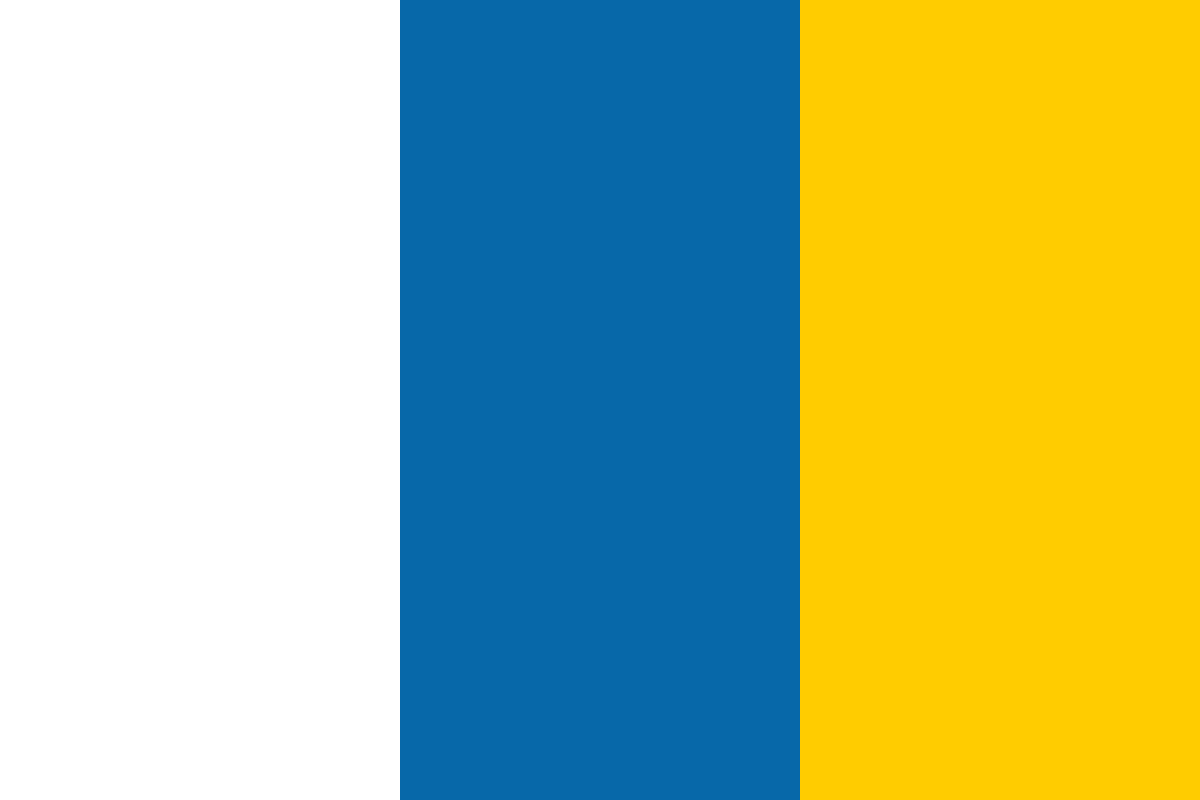

































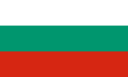








In this section, we will develop our very own plugin, the World Clock plugin. This is a very simple plugin that provides the time in different locales. We will go through all of the steps required to develop it from scratch. These steps are as follows:
There are many ways in which you can develop plugins. You can manually create all of the plugin artifacts and package them. We will use the easiest method, that is, by using Maven's geronimo-plugin-archetype. This will generate the plugin project with all of the artifacts with the default values filled in.
To generate the plugin project, run the following command:
mvn archetype:create -DarchetypeGroupId=org.apache.geronimo.buildsupport
-DarchetypeArtifactId=geronimo-plugin-archetype -DarchetypeVersion=2.1.4
-DgroupId=com.packt.plugins -DartifactId=WorldClock
This will create a plugin project called WorldClock. A directory called WorldClock will be created, with the following artifacts in it:
In the same directory in which the WorldClock directory is created, you will need to create a java project that will contain the source code of the plugin. We can create this by using the following command:
mvn archetype:create -DgroupId=com.packt.plugins -DartifactId=WorldClock
Module
This will create a java project with the same groupId and artifactId in a directory called WorldClockModule. This directory will contain the following artifacts:
You can safely remove the second and third artifacts, as they are just sample stubs generated by the archetype.
In this project, we will need to modify the pom.xml to have a dependency on the Geronimo kernel, so that we can compile the GBean that we are going to create and include in this module. The modified pom.xml is shown below:
<project
xsi_schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.packt.plugins</groupId>
<artifactId>WorldClockModule</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>WorldClockModule</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.apache.geronimo.framework</groupId>
<artifactId>geronimo-kernel</artifactId>
<version>2.1.4</version>
</dependency>
</dependencies>
</project>
For simplicity, we have only one GBean in our sample. In a real world scenario, there may be many GBeans that you will need to create. Now we need to create the GBean that forms the core functionality of our plugin. Therefore, we will create two classes, namely, Clock and ClockGBean. These classes are shown below:
package com.packt.plugins;
import java.util.Date;
import java.util.Locale;
public interface Clock {
public void setTimeZone(String timeZone);
public String getTime();
}
and
package com.packt.plugins;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.Locale;
import java.util.TimeZone;
import org.apache.geronimo.gbean.GBeanInfo;
import org.apache.geronimo.gbean.GBeanInfoBuilder;
import org.apache.geronimo.gbean.GBeanLifecycle;
import sun.util.calendar.CalendarDate;
public class ClockGBean implements GBeanLifecycle, Clock{
public static final GBeanInfo GBEAN_INFO;
private String name;
private String timeZone;
public String getTime() {
GregorianCalendar cal = new GregorianCalendar(TimeZone.
getTimeZone(timeZone));
int hour12 = cal.get(Calendar.HOUR); // 0..11
int minutes = cal.get(Calendar.MINUTE); // 0..59
int seconds = cal.get(Calendar.SECOND); // 0..59
boolean am = cal.get(Calendar.AM_PM) == Calendar.AM;
return (timeZone +":"+hour12+":"+minutes+":"+seconds+":"+((am)?
"AM":"PM"));
}
public void setTimeZone(String timeZone) {
this.timeZone = timeZone;
}
public ClockGBean(String name){
this.name = name;
timeZone = TimeZone.getDefault().getID();
}
public void doFail() {
System.out.println("Failed.............");
}
public void doStart() throws Exception {
System.out.println("Started............"+name+" "+getTime());
}
public void doStop() throws Exception {
System.out.println("Stopped............"+name);
}
static {
GBeanInfoBuilder infoFactory = GBeanInfoBuilder.createStatic
("ClockGBean",ClockGBean.class);
infoFactory.addAttribute("name", String.class, true);
infoFactory.addInterface(Clock.class);
infoFactory.setConstructor(new String[] {"name"});
GBEAN_INFO = infoFactory.getBeanInfo();
}
public static GBeanInfo getGBeanInfo() {
return GBEAN_INFO;
}
}
As you can see, Clock is an interface and ClockGBean is a GBean that implements this interface. The Clock interface exposes the functionality that is provided by the ClockGBean. The doStart(), doStop(), and doFail() methods are provided by the GBeanLifeCycle interface, and provide lifecycle callback functionality. The next step is to run Maven to build this module. Go to the command prompt, and change the directory to the WorldClockModule directory. To build the module, run the following command:
mvn clean install
Once the build completes, you will find a WorldClockModule-1.0-SNAPSHOT.jar in the WorldClockModule/target directory.
Now change the directory to WorldClock, and open the generated pom.xml file. You will need to uncomment the deploymentConfigs for the gbeanDeployer, and add the following module that you want to include in the plugin:
<module>
<groupId>com.packt.plugins</groupId>
<artifactId>WorldClockModule</artifactId>
<version>1.0</version>
<type>jar</type>
</module>
You will notice that we are using the car-maven-plugin in the pom.xml file. The car-maven-plugin is used to build Apache Geronimo configuration archives without starting the server.
The final step is to create the deployment plan in order to deploy the module that we just created into the Apache Geronimo server. This deployment plan will be used by the car-maven-plugin to actually create the artifacts that will be created during deployment to Apache Geronimo. The deployment plan is shown below:
<module >
<environment>
<moduleId>
<groupId>com.packt.plugins</groupId>
<artifactId>WorldClock</artifactId>
<version>1.0</version>
<type>car</type>
</moduleId>
<dependencies/>
<hidden-classes/>
<non-overridable-classes/>
<private-classes/>
</environment>
<gbean name="ClockGBean" class="com.packt.clock.ClockGBean">
<attribute name="name">ClockGBean</attribute>
</gbean>
</module>
Once the plan is ready, go to the command prompt and change the directory to the WorldClock directory. Run the following command to build the plugin:
mvn clean install
You will notice that the car-maven-plugin is invoked and a WorldClock-1.0-SNAPSHOT.car file is created in the WorldClock/target directory. We have now completed the steps required to create an Apache Geronimo plugin. In the next section, we will see how we can install the plugin in Apache Geronimo.
We can install a plugin in three different ways. One way is to use the deploy.bat or deploy.sh script, another way is to use the install-plugin command in GShell, and the third way is to use the Administration Console to install a plugin from a plugin repository. We will discuss each of these methods:
The deploy.bat or deploy.sh script is found in the <GERONIMO_HOME>/bin directory. It has an option install-plugin, which can be used to install plugins onto the server. The command syntax is shown below:
deploy install-plugin <path to the plugin car file>
Running this command, and passing the path to the plugin .car archive on the disk, will result in the plugin being installed onto the Geronimo server. Once the installation has finished, an Installation Complete message will be displayed, and the command will exit.
Invoke the gsh command from the command prompt, after changing the current directory to <GERONIMO_HOME>/bin. This will bring up the GShell prompt. In the GShell prompt, type the following command to install the plugin:
deploy/install-plugin <path to the plugin car file>
Please note that, you should escape special characters in the path by using a leading "" (back slash) before the character.
Another way to install plugins that are available in remote plugin repository is by using the list-plugins command. The syntax of this command is as given below:
deploy/list-plugins <URI of the remote repository>
If a remote repository is not specified, then the one configured in Geronimo will be used instead. Once this command has been invoked, the list of available plugins in the remote repository is shown, along with their serial numbers, and you will be prompted to enter a comma separated list of the serial numbers of the plugins that you want to install.
The Administration Console has a Plugins portlet that can be used to list the plugins available in a repository specified by the user. You can use the Administration Console to select and install the plugins that you want from this list. This portlet also has the capability to export applications or services in your server instance as Geronimo plugins, so that they can be installed on other server instances. See the Plugin portlet section for details of the usage of this portlet.
The web site http://geronimoplugins.com/ hosts Apache Geronimo plugins. It has many plugins listed for Apache Geronimo. There are plugins for Quartz, Apache Directory Server, and many other popular software packages. However, they are not always available for the latest versions of Apache Geronimo. A couple of fairly up-to-date plugins that are available for Apache Geronimo are the Windows Service Wrapper plugin and the Apache Tuscany plugin for Apache Geronimo. The Windows Service Wrapper provides the ability for Apache Geronimo to be registered as a windows service. The Tuscany plugin is an implementation of the SCA Java EE Integration specification by integrating Apache Tuscany as an Apache Geronimo plugin. Both of these plugins are available from the Apache Geronimo web site.
Older versions of Apache Geronimo came with a monolithic Administration Console. However, the server was extendable through plugins. This introduced a problem: How to administer the new plugins that were added to the server? To resolve this problem, the Apache Geronimo developers rewrote the Administration Console to be extensible through console plugins called Administration Console Extensions. In this section, we will look into how to create an Administration Console portlet for the World Clock plugin that we developed in the previous section.
The pluggable Administration Console functionality is based on the support provided by the Apache Pluto portlet container for dynamically adding and removing portlets and pages without requiring a restart. Apache Geronimo exposes this functionality through two GBeans, namely, the Administration Console Extension (ACE) GBean (org.apache.geronimo.pluto.AdminConsoleExtensionGBean) and the Portal Container Services GBean (org.apache.geronimo.pluto. PortalContainerServicesGBean). The PortalContainerServicesGBean exposes the features of the Pluto container in order to add and remove portlets and pages at runtime. The ACE GBean invokes these APIs to add and remove the portlets or pages. The ACE GBean should be specified in the Geronimo-specific deployment plan of your web application or plugin, that is, geronimo-web.xml. The architecture is shown in the following figure:
We will now go through the steps to develop an Administration Console Extension for the World Clock plugin that we created in the previous section.
We will use Maven WAR archetype to create a web application project. To create the project, run the following command from the command-line console:
mvn archetype:create -DgroupId=com.packt.plugins -
DartifactId=ClockWebApp -DarchetypeArtifactId=maven-archetype-
webapp
This will result in the Maven web project being created, named ClockWebApp.
A default pom.xml will be created. This will need to be edited to add dependencies to the two modules, as shown in the following code snippet:
<dependency>
<groupId>org.apache.geronimo.framework</groupId>
<artifactId>geronimo-kernel</artifactId>
<version>2.1.4</version>
</dependency>
<dependency>
<groupId>com.packt.plugins</groupId>
<artifactId>WorldClockModule</artifactId>
<version>1.0</version>
</dependency>
We add these dependencies because the portlet that we are going to write will use the classes mentioned in the above two modules.