










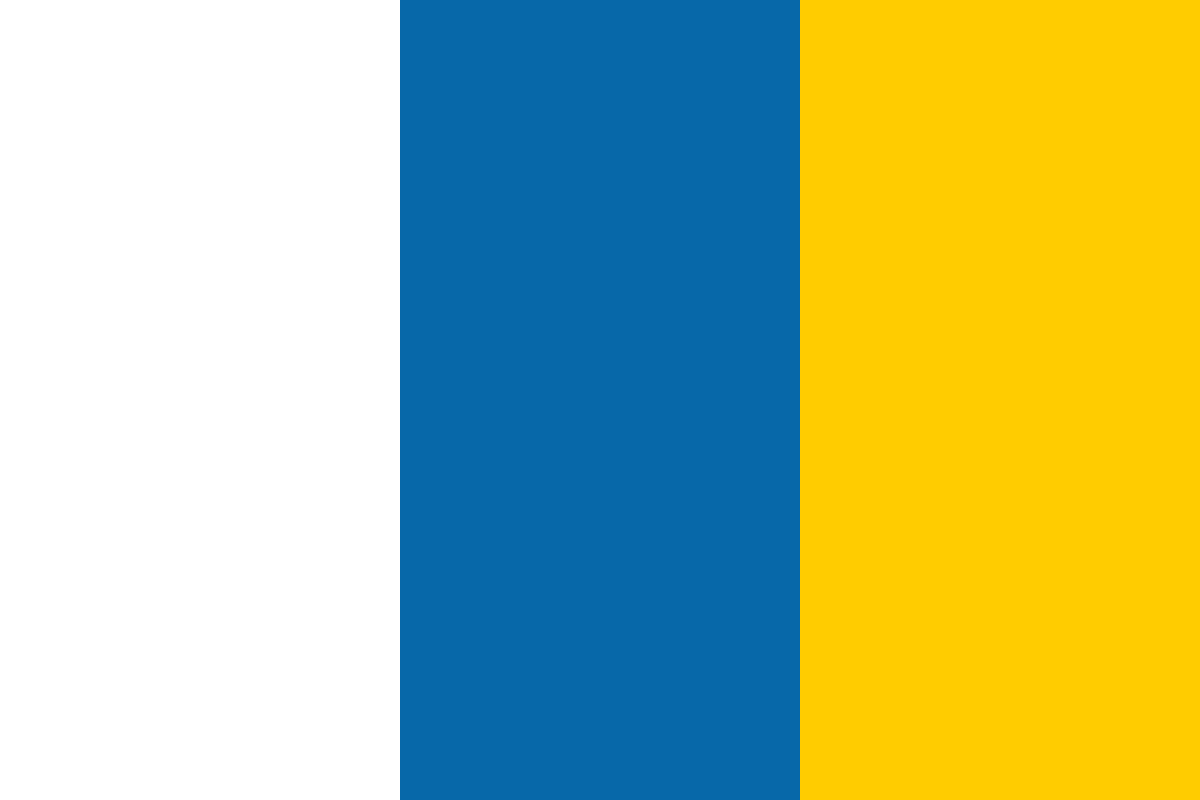

































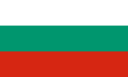








(For more resources related to this topic, see here.)
Collecting user data is a basic function of many websites and web applications, from simple data collection techniques such as registration or login information, to more complex scenarios such as payment or billing information. It is important that only relevant and complete information is collected from the user. To ensure this, the web developer must enforce validation on all data input. It is also important to provide a good user experience while enforcing this data integrity. This can be done by providing useful feedback to the user regarding any validation errors their data may have caused. This article will show you how to create an attractive web form that enforces data integrity while keeping a high-quality user experience.
A very important point to note is that any JavaScript or jQuery validation is open to manipulation by the user. JavaScript and jQuery resides within the web browser, so a user with little knowledge can easily modify the code to bypass any client-side validation techniques. This means that client-side validation cannot be totally relied on to prevent the user from submitting invalid data. Any validation done within the client side must be replicated on the server, which is not open for manipulation by the user.
We use client-side validation to improve the user experience. Because of this, the user does not need to wait for a server response.
At the most basic level of form validation, you will need to be able to prevent the user from submitting empty values. This recipe will provide the HTML and CSS code for a web form that will be used for recipes 1 through 8 of this article.
Using your favorite text editor or IDE, create a blank HTML page in an easily accessible location and save this file as recipe-1.html. Ensure that you have the latest version of jQuery downloaded to the same location as this HTML file.
This HTML page will form the basis of most of this article, so remember to keep it after you have completed this recipe.
Learn how to implement basic form validation with jQuery by performing the following steps:
<!DOCTYPE html> <html > <head> <title>Chapter 5 :: Recipe 1</title> <link type="text/css" media="screen" rel="stylesheet" href="styles.css" /> <script src = "jquery.min.js"></script> <script src = "validation.js"></script> </head> <body> <form id="webForm" method="POST"> <div class="header"> <h1>Register</h1> </div> <div class="input-frame"> <label for="firstName">First Name:</label> <input name="firstName" id="firstName" type="text" class="required" /> </div> <div class="input-frame"> <label for="lastName">Last Name:</label> <input name="lastName" id="lastName" type="text" class="required" /> </div> <div class="input-frame"> <label for="email">Email:</label> <input name="email" id="email" type="text" class="required email" /> </div> <div class="input-frame"> <label for="number">Telephone:</label> <input name="number" id="number" type="text" class="number" /> </div> <div class="input-frame"> <label for="dob">Date of Birth:</label> <input name="dob" id="dob" type="text" class="required date" placeholder="DD/MM/YYYY"/> </div> <div class="input-frame"> <label for="creditCard">Credit Card #:</label> <input name="creditCard" id="creditCard" type="text" class="required credit-card" /> </div> <div class="input-frame"> <label for="password">Password:</label> <input name="password" id="password" type="password" class="required" /> </div> <div class="input-frame"> <label for="confirmPassword">Confirm Password:</label> <input name="confirmPassword" id="confirmPassword" type="password" class="required" /> </div> <div class="actions"> <button class="submit-btn">Submit</button> </div> </form> </body> </html>
@import url(http: //fonts.googleapis.com/css?family=Ubuntu); body { background-color: #FFF; font-family: 'Ubuntu', sans-serif; } form { width: 500px; padding: 20px; background-color: #333; border-radius: 5px; margin: 10px auto auto auto; color: #747474; border: solid 2px #000; } form label { font-size: 14px; line-height: 30px; width: 27%; display: inline-block; text-align: right; } .input-frame { clear: both; margin-bottom: 25px; position: relative; } form input { height: 30px; width: 330px; margin-left: 10px; background-color: #191919; border: solid 1px #404040; padding-left: 10px; color: #DB7400; } form input:hover { background-color: #262626; } form input:focus { border-color: #DB7400; } form .header { margin: -20px -20px 25px -20px; padding: 10px 10px 10px 20px; position: relative; background-color: #DB7400; border-top-left-radius: 4px; border-top-right-radius: 4px; } form .header h1 { line-height: 50px; margin: 0px; padding: 0px; color: #FFF; font-weight: normal; } .actions { text-align: right; } .submit-btn { background-color: #DB7400; border: solid 1px #000; border-radius: 5px; color: #FFF; padding: 10px 20px 10px 20px; text-decoration: none; cursor: pointer; } .error input { border-color: red; } .error-data { color: red; font-size: 11px; position: absolute; bottom: -15px; left: 30%; }
$(function(){ $('.submit-btn').click(function(event){ //Prevent form submission event.preventDefault(); var inputs = $('input'); var isError = false; //Remove old errors $('.input-frame').removeClass('error'); $('.error-data').remove(); for (var i = 0; i < inputs.length; i++) { var input = inputs[i]; if ($(input).hasClass('required') && !validateRequired($(input).val())) { addErrorData($(input), "This is a required field"); isError = true; } } if (isError === false) { //No errors, submit the form $('#webForm').submit(); } }); }); function validateRequired(value) { if (value == "") return false; return true; } function addErrorData(element, error) { element.parent().addClass("error"); element.after("<div class='error-data'>" + error + "</div>"); }
Now, let us understand the steps performed previously in detail.
The HTML creates a web form with various fields that will take a range of data inputs, including text, date of birth, and credit card number. This page forms the basis for most of this article. Each of the input elements has been given different classes depending on what type of validation they require. For this recipe, our JavaScript will only look at the required class, which indicates a required field and therefore cannot be blank. Other classes have been added to the input fields, such as date and number, which will be used in the later recipes in this article.
Basic CSS has been added to create an attractive web form. The CSS code styles the input fields so they blend in with the form itself and adds a hover effect. The Google Web Font Ubuntu has also been used to improve the look of the form.
The first part of the jQuery code is wrapped within $(function(){});, which will ensure the code is executed on page load. Inside this wrapper, we attach a click event handler to the form submit button, shown as follows:
$(function(){ $('.submit-btn').click(function(event){ //Prevent form submission event.preventDefault(); }); });
As we want to handle the form submission based on whether valid data has been provided, we use event.preventDefault(); to initially stop the form from submitting, allowing us to perform the validation first, shown as follows:
var inputs = $('input'); var isError = false;
After the preventDefault code, an inputs variable is declared to hold all the input elements within the page, using $('input') to select them. Additionally, we create an isError variable, setting it to false. This will be a flag to determine if our validation code has discovered an error within the form. These variable declarations are shown previously. Using the length of the inputs variable, we are able to loop through all of the inputs on the page. We create an input variable for each input that is iterated over, which can be used to perform actions on the current input element using jQuery. This is done with the following code:
for (var i = 0; i < inputs.length; i++) { var input = inputs[i]; }
After the input variable has been declared and assigned the current input, any previous error classes or data is removed from the element using the following code:
$(input).parent().removeClass('error'); $(input).next('.error-data').remove();
The first line removes the error class from the input's parent (.input-frame), which adds the red border to the input element. The second line removes the error information that is displayed under the input if the validation check has determined that this input has invalid data.
Next, jQuery's hasClass() function is used to determine if the current input element has the required class. If the current element does have this class, we need to perform the required validation to make sure this field contains data. We call the validateRequired() function within the if statement and pass through the value of the current input, shown as follows:
if ($(input).hasClass('required') && !validateRequired($(input).val())) { addErrorData($(input), "This is a required field"); isError = true; }
We call the validateRequired() function prepended with an exclamation mark to check to determine if this function's results are equal to false; therefore, if the current input has the required class and validateRequired() returns false, the value of the current input is invalid. If this is the case, we call the addErrorData() function inside the if statement with the current input and the error message, which will be displayed under the input. We also set the isError variable to true, so that later on in the code, we will know a validation error occurred.
The JavaScript's for loop will repeat these steps for each of the selected input elements on the page. After the for loop has completed, we check if the isError flag is still set to false. If so, we use jQuery to manually submit the form, shown as follows:
if (isError === false) { //No errors, submit the form $('#webForm').submit(); }
Note that the operator === is used to compare the variable type of isError (that is, Boolean) as well as its value. At the bottom of the JavaScript file, we declare our two functions that have been called earlier in the script. The first function, validateRequired(), simply takes the input value and checks to see if it is blank or not. If the value is blank, the function returns false, meaning validation failed; otherwise, the function returns true. This can be coded as follows:
function validateRequired(value) { if (value == "") return false; return true; }
The second function used is the addErrorData() function, which takes the current input and an error message. It uses jQuery's addClass() function to add the error class to the input's parent, which will display the red border on the input element using CSS. It then uses jQuery's after() function to insert a division element into the DOM, which will display the specified error message under the current input field, shown as follows:
function validateRequired(value) { if (value == "") return false; return true; } function addErrorData(element, error) { element.parent().addClass("error"); element.after("<div class='error-data'>" + error + "</div>"); }
This structure allows us to easily add additional validation to our web form. Because the JavaScript is iterating over all of the input fields in the form, we can easily check for additional classes, such as date, number, and credit-card, and call extra functions to provide the alternative validation. The other recipes in this article will look in detail at the additional validation types and add these functions to the current validation.js file.
When collecting data from a user, there are many situations when you will want to only allow numbers in a form field. Examples of this could be telephone numbers, PIN codes, or ZIP codes, to name a few. This recipe will show you how to validate the telephone number field within the form we created in the previous recipe.
Ensure that you have completed the previous recipe and have the same files available. Open validation.js in your text editor or IDE of choice.
Add number validation to the form you created in the previous recipe by performing the following steps:
$(function(){ $('.submit-btn').click(function(event){ //Prevent form submission event.preventDefault(); var inputs = $('input'); var isError = false; //Remove old errors $('.input-frame').removeClass('error'); $('.error-data').remove(); for (var i = 0; i < inputs.length; i++) { var input = inputs[i]; if ($(input).hasClass('required') && !validateRequired($(input).val())) { addErrorData($(input), "This is a required field"); isError = true; } /* Code for this recipe */ if ($(input).hasClass('number') && !validateNumber($(input).val())) { addErrorData($(input), "This field can only contain numbers"); isError = true; } /* --- */ } if (isError === false) { //No errors, submit the form $('#webForm').submit(); } }); }); function validateRequired(value) { if (value == "") return false; return true; } /* Code for this recipe */ function validateNumber(value) { if (value != "") { return !isNaN(parseInt(value, 10)) && isFinite(value); //isFinite, in case letter is on the end } return true; } /* --- */ function addErrorData(element, error) { element.parent().addClass("error"); element.after("<div class='error-data'>" + error + "</div>"); }
First, we add an additional if statement to the main for loop of validation.js to check to see if the current input field has the class number, as follows:
if ($(input).hasClass('number') && !validateNumber($(input).val())) { addErrorData($(input), "This field can only contain numbers"); isError = true; }
If it does, this input value needs to be validated for a number. To do this, we call the validateNumber function inline within the if statement:
function validateNumber(value) { if (value != "") { return !isNaN(parseInt(value, 10)) && isFinite(value); //isFinite, in case letter is on the end } return true; }
This function takes the value of the current input field as an argument. It first checks to see if the value is blank. If it is, we do not need to perform any validation here because this is handled by the validateRequired() function from the first recipe of this article.
If there is a value to validate, a range of actions are performed on the return statement. First, the value is parsed as an integer and passed to the isNaN() function. The JavaScript isNaN() function simply checks to see if the provided value is NaN (Not a Number). In JavaScript, if you try to parse a value as an integer and that value is not actually an integer, you will get the NaN value. The first part of the return statement is to ensure that the provided value is a valid integer. However, this does not prevent the user from inputting invalid characters. If the user was to input 12345ABCD, the parseInt function would ignore ABCD and just parse 12345, and therefore the validation would pass. To prevent this situation, we also use the isFinite function, which returns false if provided with 12345ABCD.
Number validation could be enough validation for a credit card number; however, using regular expressions, it is possible to check for number combinations to match credit card numbers from Visa, MasterCard, American Express, and more.
Make sure that you have validation.js from the previous two recipes in this article open and ready for modification.
Use jQuery to provide form input validation for credit card numbers by performing the following step-by-step instructions:
$(function(){ $('.submit-btn').click(function(event){ //Prevent form submission event.preventDefault(); var inputs = $('input'); var isError = false; for (var i = 0; i < inputs.length; i++) { // -- JavaScript from previous two recipes hidden if ($(input).hasClass('credit-card') && !validateCreditCard($(input).val())) { addErrorData($(input), "Invalid credit card number"); isError = true; } } // -- JavaScript from previous two recipes hidden }); }); // -- JavaScript from previous two recipes hidden function validateCreditCard(value) { if (value != "") { return /^(?:4[0-9]{12}(?:[0-9]{3})?|5[1-5][0-9]{14}|6(?:011|5[0-9][0-9]) [0-9]{12}|3[47][0-9]{13}|3(?:0[0-5]|[68][0-9])[0-9]{11}| (?:2131|1800|35d{3})d{11})$/.test(value); } return true; } // -- JavaScript from previous two recipes hidden }
To add credit card validation, as with the previous two recipes, we added an additional check in the main for loop to look for the credit-card class on the input elements, as follows:
if ($(input).hasClass('credit-card') &&
!validateCreditCard($(input).val())) { addErrorData($(input), "Invalid credit card number"); isError = true; }
The validateCreditCard function is also added, which uses a regular expression to validate the input value, as follows:
function validateCreditCard(value) { if (value != "") { return /^(?:4[0-9]{12}(?:[0-9]{3})?|5[1-5][0- 9]{14}|6(?:011|5[0-9][0-9])[0-9]{12}|3[47][0- 9]{13}|3(?:0[0-5]|[68][0-9])[0- 9]{11}|(?:2131|1800|35d{3})d{11})$/.test(value); } return true; }
The first part of this function determines if the provided value is blank. If it isn't, the function will perform further validation; otherwise, it will return true. Most credit card numbers start with a prefix, which allows us to add additional validation to the inputted value on top of numeric validation. The regular expression used in this function will allow for Visa, MasterCard, American Express, Diners Club, Discover, and JCB cards.