










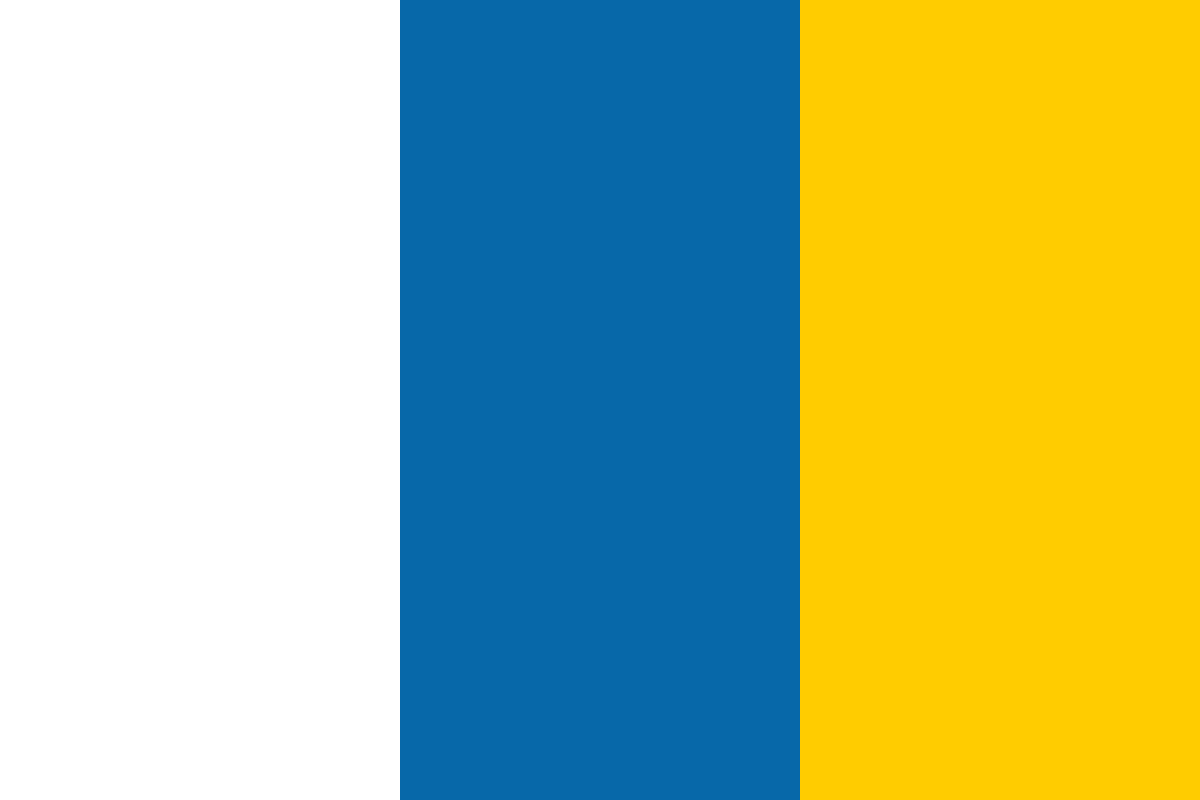

































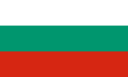








(For more resources related to this topic, see here.)
This section will parse the content of the page at, http://jsoup.org.
The index.html file in the project is provided if you want to have a fi le as input, instead of connecting to the URL.
The following screenshot shows the page that is going to be parsed:
By viewing the source code for this HTML page, we know the site structure.
The jsoup library is quite supportive of the DOM navigation method; it provides ways to find elements and extract their contents efficiently.
Document doc = Jsoup.connect("http://jsoup.org").get();
Elements navDivTag = doc.getElementsByClass("nav-sections");
Elements list = navDivTag.get(0).getElementsByTag("a");
for(Element menu: list) {
System.out.print(String.format("[%s]", menu.html()));
}
The output should look like the following screenshot after running the code:
The complete example source code for this section is placed at sourceSection02.
The API reference for this section is available at:
http://jsoup.org/apidocs/org/jsoup/nodes/Element.html
Let's have a look at the navigation structure:
html > body.n1-home > div.wrap > div.header > div.nav-sections > ul >
li.n1-news > a
The div class="nav-sections" tag is the parent of the navigation section, so by using getElementsByClass("nav-sections"), it will move to this tag. Since there is only one tag with this class value in this example, we only need to extract the first found element; we will get it at index 0 (first item of results).
Elements navDivTag = doc.getElementsByClass("nav-sections");
The Elements object in jsoup represents a collection ( Collection<>) or a list (List<>); therefore, you can easily iterate through this object to get each element, which is known as an Element object.
When at a parent tag, there are several ways to get to the children. Navigate from subtag <ul>, and deeper to each <li> tag, and then to the <a> tag. Or, you can directly make a query to find all the <a> tags. That's how we retrieved the list that we found, as shown in the following code:
Elements list = navDivTag.get(0).getElementsByTag("a");
The final part is to print the extracted HTML content of each <a> tag.
Beware of the list value; even if the navigation fails to find any element, it is always not null, and therefore, it is good practice to check the size of the list before doing any other task.
Additionally, the Element.html() method is used to return the HTML content of a tag.
jsoup is quite a powerful library for DOM navigation. Besides the following mentioned methods, the other navigation types to find and extract elements are also supported in the Element class. The following are the common methods for DOM navigation:
Methods
|
Descriptions
|
getElementById(String id)
|
Finds an element by ID, including its children.
|
getElementsByTag(String c)
|
Finds elements, including and recursively under the element that calls this method, with the specified tag name (in this case, c).
|
getElementsByClass(String className)
|
Finds elements that have this class, including or under the element that calls this method. Case insensitive.
|
getElementsByAttribute(String key)
|
Find elements that have a named attribute set. Case insensitive. This method has several relatives, such as:
|
getElementsMatchingText(Pattern pattern)
|
Finds elements whose text matches the supplied regular expression.
|
getAllElements()
|
Finds all elements under the specified element (including self and children of children).
|
There is a need to mention all methods that are used to extract content from an HTML element. The following table shows the common methods for extracting elements:
Methods
|
Descriptions
|
id()
|
This retrieves the ID value of an element.
|
className()
|
This retrieves the class name value of an element.
|
attr(String key)
|
This gets the value of a specific attribute.
|
attributes()
|
This is used to retrieve all the attributes.
|
html()
|
This is used to retrieve the inner HTML value of an element.
|
data()
|
This is used to retrieve the data content, usually applied for getting content from the <script> and <style> tags.
|
text()
|
This is used to retrieve the text content. This method will return the combined text of all inner children and removes all HTML tags, while the html() method returns everything between its open and closed tags.
|
tag()
|
This retrieves the tag of the element.
|
In this article we saw to extract data using DOM from an HTML page. It was seen that jsoup is quite a powerful library for DOM navigation.
Further resources on this subject: