










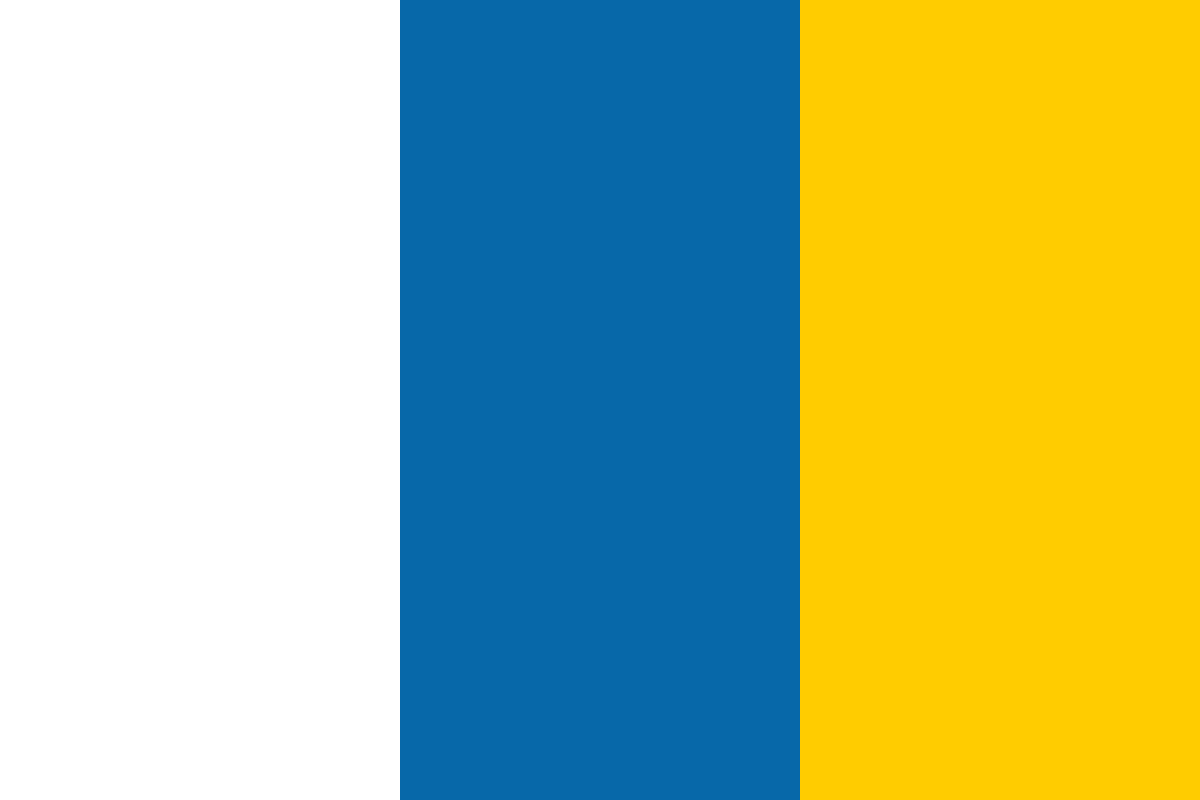

































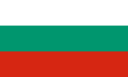








The tab widget defines a series of useful options that allow you to add callback functions to perform different actions when certain events exposed by the widget are detected. The following table lists the configuration options that are able to accept executable functions on an event:
Property |
Usage |
add |
Execute a function when a new tab is added. |
disable |
Execute a function when a tab is disabled. |
enable |
Execute a function when a tab is enabled. |
load |
Execute a function when a tab's remote data has loaded. |
remove |
Execute a function when a tab is removed. |
select |
Execute a function when a tab is selected. |
show |
Execute a function when the content section of a tab is shown. |
Each component of the library has callback options (such as those in the previous table), which are tuned to look for key moments in any visitor interaction. Any function we use with these callbacks are usually executed before the change happens. Therefore, you can return false from your callback and prevent the action from occurring.
In our next example, we will look at how easy it is to react to a particular tab being selected using the standard non-bind technique. Change the final <script> element in tabs7.html so that it appears as follows:
<script type="text/javascript">
$(function(){
function handleSelect(event, tab) {
$("<p>").text("The tab at index " + tab.index +
" was selected").addClass("status-message ui-corner-all")
.appendTo($(".ui-tabs-nav","#myTabs")).fadeOut(5000);
}
var tabOpts = {
select:handleSelect
};
$("#myTabs").tabs(tabOpts);
});
</script>
Save this file as tabs8.html. We also need a little CSS to complete this example, in the <head> of the page we just created add the following <link> element:
<link rel="stylesheet" type="text/css" href="css/tabSelect.css">
Then in a new page in your text editor add the following code:
.status-message {
position:absolute; right:3px; top:4px; margin:0;
padding:11px 8px 10px; font-size:11px;
background-color:#ffffff; border:1px solid #aaaaaa;
}
Save this file as tabSelect.css in the css folder.
We made use of the select callback in this example, although the principle is the same for any of the other custom events fired by tabs. The name of our callback function is provided as the value of the select property in our configuration object.
Two arguments will be passed automatically to the function we define by the widget when it is executed. These are the original event object and a custom object containing useful properties from the tab which is in the function's execution context.
To find out which of the tabs was clicked, we can look at the index property of the second object (remember these are zero-based indices). This is added, along with a little explanatory text, to a paragraph element that we create on the fly and append to the widget header.
In this example, the callback function was defined outside the configuration object, and was instead referenced by the object. We can also define these callback functions inside our configuration object to make our code more efficient. For example, our function and configuration object from the previous example could have been defined like this:
var tabOpts = {
select: function(event, tab) {
$("<p>").text("The tab at index " + tab.index + " was selected")
.addClass("status-message ui-corner-all").appendTo($(".ui-tabs-nav",
"#myTabs")).fadeOut(5000);
}
}
Check tabs8inline.html in the code download for further clarification on this way of using event callbacks. Whenever a tab is selected, you should see the paragraph before it fades away. Note that the event is fired before the change occurs.
Using the event callbacks exposed by each component is the standard way of handling interactions. However, in addition to the callbacks listed in the previous table we can also hook into another set of events fired by each component at different times.
We can use the standard jQuery bind() method to bind an event handler to a custom event fired by the tabs widget in the same way that we could bind to a standard DOM event, such as a click.
The following table lists the tab widget's custom binding events and their triggers:
Event |
Trigger |
tabsselect |
A tab is selected. |
tabsload |
A remote tab has loaded. |
tabsshow |
A tab is shown. |
tabsadd |
A tab has been added to the interface. |
tabsremove |
A tab has been removed from the interface. |
tabsdisable |
A tab has been disabled. |
tabsenable |
A tab has been enabled. |