










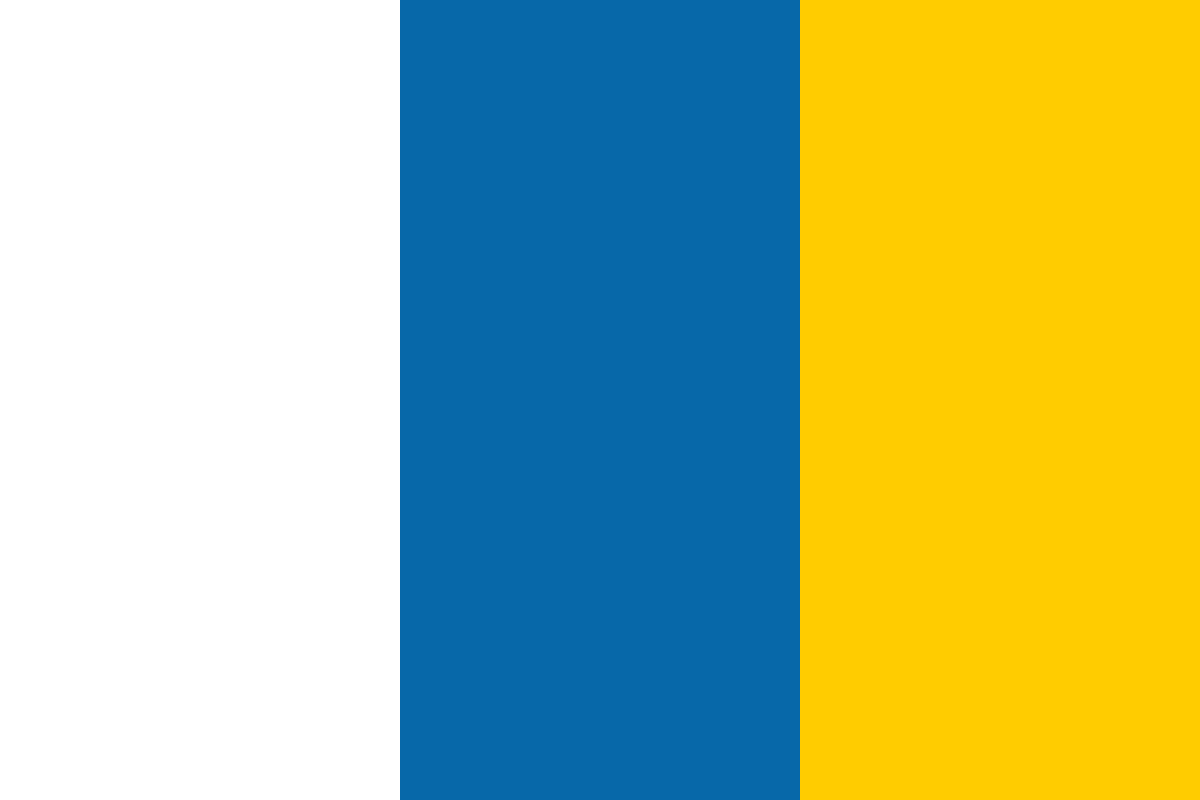

































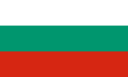








The dialog requires few methods in order to function. The full list of the methods we can call on a dialog is as follows:
Method |
Used to |
close |
Close or hide the dialog. |
destroy |
Permanently disable the dialog. The destroy method for a dialog works in a slightly different way than it does for the other widgets we've seen so far. Instead of just returning the underlying HTML to its original state, the dialog's destroy method also hides it. |
disable |
Temporarily disable the dialog. |
enable |
Enable the dialog if it has been disabled. |
isOpen |
Determine whether a dialog is open or not. |
moveToTop |
Move the specified dialog to the top of the stack. |
open |
Open the dialog. |
option |
Get or set any configurable option after the dialog has been initialized. |
We first take a look at opening the widget, which can be achieved with the simple use of the open method. Let's revisit dialog3.html in which the autoOpen option was set to false, so the dialog did not open when the page was loaded. Add the following < button> to the page:
<button id="toggle">Toggle dialog!</button>
Then add the following click-handler directly after the dialog's widget method:
$("#toggle").click(function() {
($("#myDialog").dialog("isOpen") == false) ?
$("#myDialog").dialog("open") : $("#myDialog").dialog("close") ;
});
Save this file as dialog14.html. To the page we've added a simple < button> that can be used to either open or close the dialog depending on its current state. In the < script> element, we've added a click handler for the < button> that uses the JavaScript ternary conditional to check the return value of the isOpen method. If it returns false, the dialog is not open so we call its open method else we call the close method instead.
The open and close methods both trigger any applicable events, for example, the close method fires first the beforeclose and then the close events. Calling the close method is analogous to clicking the close button on the dialog.
Because the widget is a part of the underlying page, passing data to and from it is simple. The dialog can be treated as any other standard element on the page. Let's look at a basic example.
We looked at an example earlier in the article which added some < button> elements to the dialog. The callback functions in that example didn't do anything, but this example gives us the opportunity to use them. Add the following code above the < script> tags in dialog14.html.
<p>Please answer the opinion poll:</p>
<div id="myDialog" title="Best Widget Library">
<p>Is jQuery UI the greatest JavaScript widget library?</p>
<label for="yes">Yes!</label><input type="radio" id="yes" value="yes" name="question"
checked="checked"><br>
<label for="no">No!</label><input type="radio" id="no" value="no" name="question">
</div>
<script type="text/javascript" src="development-bundle/jquery-1.3.2.js"></script>
Now change the final < script> element to as follows:
<script type="text/javascript">
$(function(){
var execute = function(){
var answer;
$("input").each(function(){
(this.checked == true) ? answer = $(this).val() : null;
});
$("<p>").text("Thanks for selecting " +answer).appendTo($("body"));
$("#myDialog").dialog("close");
}
var cancel = function() {
$("#myDialog").dialog("close");
}
var dialogOpts = {
buttons: {
"Ok": execute,
"Cancel": cancel
}
};
$("#myDialog").dialog(dialogOpts);
});
</script>
Save this as dialog15.html. Our dialog widget now contains a set of radio buttons, < label> elements, and some text. The purpose of the example is to get the result of which radio is selected, and then do something with it when the dialog closes.
We start the < script> by filling out the execute function that will be attached as the value of the Ok property in the buttons object later in the script. It will therefore be executed each time the Ok button is clicked.
In this function we use jQuery's each() method to look at both of the radio buttons and determine which one is selected. We set the value of the answer variable to the radio button's value and then created a short message along with appending it to the < body> of the page. The callback mapped to the Cancel button is simple, all we do is close the dialog using the close method.
The following screenshot shows how the page should appear once a radio button has been selected and the Ok button has been clicked:
The point of this trivial example was to see that getting data from the dialog is as simple as getting data from any other component on the page.
We can easily place other UI widgets into the dialog, such as the accordion widget. In a new file in your text editor create the following page:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
<html lang="en">
<head>
<link rel="stylesheet" type="text/css" href="development-bundle/themes/smoothness/ui.all.css">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>jQuery UI Dialog Example 17</title>
</head>
<body>
<div id="myDialog" title="An Accordion Dialog">
<div id="myAccordion">
<h2><a href="#">Header 1</a></h2><div>Lorem ipsum dolor sit amet,
consectetuer adipiscing elit.Aenean sollicitudin.</div>
<h2><a href="#">Header 2</a></h2><div>Etiam tincidunt est vitae est.
Ut posuere, mauris at sodales rutrum, turpis.</div>
<h2><a href="#">Header 3</a></h2><div>Donec at dolor ac metus pharetra aliquam.
Suspendisse purus.</div>
</div>
</div>
<script type="text/javascript" src="development-bundle/jquery-1.3.2.js"></script>
<script type="text/javascript" src="development-bundle/ui/ui.core.js"></script>
<script type="text/javascript" src="development-bundle/ui/ui.dialog.js"></script>
<script type="text/javascript" src="development-bundle/ui/ui.draggable.js"></script>
<script type="text/javascript" src="development-bundle/ui/ui.resizable.js"></script>
<script type="text/javascript" src="development-bundle/ui/ui.accordion.js"></script>
<script type="text/javascript">
$(function(){
$("#myDialog").dialog();
$("#myAccordion").accordion();
});
</script>
</body>
</html>
Save this file as dialog16.html. The underlying markup for the accordion widget is placed into the dialog's container element, and we just call each component's widget method in the < script>. The combined widget should appear like this: