










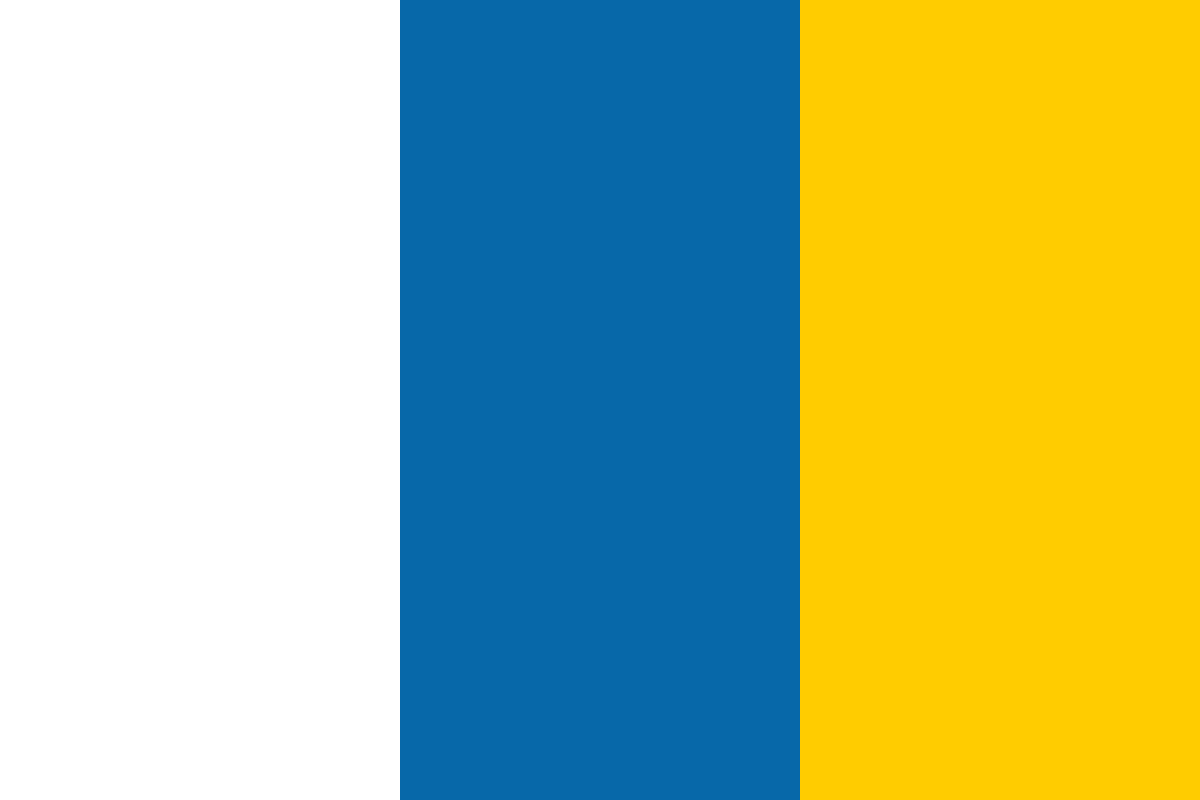

































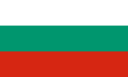








In this article by Chendrayan Venkatesan, the author of the book Windows PowerShell for .NET Developers, we will cover the following topics:
(For more resources related to this topic, see here.)
Windows PowerShell 5.0 has many significant benefits, to know more features about its features refer to the following link:
http://go.microsoft.com/fwlink/?LinkID=512808
A few highlights of Windows PowerShell 5.0 are as follows:
Desired State Configuration also known as DSC is a new management platform in Windows PowerShell. Using DSC, we can deploy and manage configuration data for software servicing and manage the environment. DSC can be used to streamline datacenters and this was introduced along with Windows Management Framework 4.0 and it heavily extended into Windows Management Framework 5.0.
Few highlights of DSC in April 2015 Preview are as follows:
It's not mandatory to know PowerShell to learn DSC but it's a great added advantage.
Similar to function we can also use configuration keyword but it has a huge difference because in DSC everything is declarative, which is a cool thing in Desired State Configuration. So before beginning this exercise, I created a DSCDemo lab machine in Azure cloud with Windows Server 2012 and it's available out of the box. So, the default PowerShell version is 4.0.
For now let's create and define a simple configuration, which creates a file in the local host. Yeah! A simple New-Item command can do that but it's an imperative cmdlet and we need to write a program to tell the computer to create it, if it does not exist.
Structure of the DSC configuration is as follows:
Configuration Name
{
Node ComputerName
{
ResourceName <String>
{
}
}
}
To create a simple text file with contents, we use the following code:
Configuration FileDemo
{
Node $env:COMPUTERNAME
{
File FileDemo
{
Ensure = 'Present'
DestinationPath = 'C:TempDemo.txt'
Contents = 'PowerShell DSC Rocks!'
Force = $true
}
}
}
Look at the following screenshot:
Following are the steps represented in the preceding figure:
Look at the MOF file structure in the following image:
We can manually edit the MOF and use it on another machine that has PS 4.0 installed on it. It's not mandatory to use PowerShell for generating MOF, if you are comfortable with PowerShell, you can directly write the MOF file.
To explore the available DSC resources you can execute the following command:
Get-DscResource
The output is illustrated in the following image:
Following are the steps represented in the preceding figure:
Module name the resource belongs to.
Properties of the resource.
To know the Syntax of a particular DSC resource we can try the following code:
Get-DscResource -Name Service -Syntax
The output is illustrated in the following figure ,which shows the resource syntax in detail:
Now, let's see how DSC works and its three different phases:
The "Make it so" phase.
In this phase we will create a DSC Configuration using PowerShell and this outputs a MOF file. We saw a FileDemo example to create a configuration is considered to be an authoring phase.
In this phase the declarative MOF will be staged and it's as per node. DSC has a push and pull model, where push is simply pushing the configuration to target nodes. The custom providers need to be manually placed in target machines whereas in pull mode, we need to build an IIS Server that will have MOF for target nodes and this is well defined by the OData interface. In pull mode, the custom providers are downloaded to target system.
This is the phase for enacting the configuration, that is applying the configuration on the target nodes.
Before we summarize the basics of DSC, let's see a few more DSC Commands. We can do this by executing the following command:
Get-Command -Noun DSC*
The output is as follows:
We are using a PowerShell 4.0 stable release and not 5.0, so the version will not be available.
Local Configuration Manager (LCM) is the engine for DSC and it runs on all nodes. LCM is responsible to call the configuration resources that are included in a DSC configuration script. Try executing Get-DscLocalConfigurationManager cmdlet to explore its properties. To Apply the LCM settings on target nodes we can use Set-DscLocalConfigurationManager cmdlet.
Using classes in PowerShell makes IT professionals, system administrators, and system engineers to start learning development in WMF.
It's time for us to switch back to Windows PowerShell 5.0 because the Class keyword is supported from version 5.0 onwards. Why do we need to write class in PowerShell? Is there any special need? May be we will answer this in this section but this is one reason why I prefer to say that, PowerShell is far more than a scripting language.
When the Class keyword was introduced, it mainly focused on creating DSC resources. But using class we can create objects like in any other object oriented programming language. The documentation that reads New-Object is not supported. But it's revised now. Indeed it supports the New-Object. The class we create in Windows PowerShell is a .NET framework type.
How to create a PowerShell Class? It's easy, just use the Class keyword! The following steps will help you to create a PowerShell class.
Create a class named ClassName {}—this is an empty class.
Define properties in the class as Class ClassName {$Prop1 , $prop2}
Instantiate the class as $var = [ClassName]::New()
Now check the output of $var:
Class ClassName {
$Prop1
$Prop2
}
$var = [ClassName]::new()
$var
Let's now have a look at how to create a class and its advantages.
Let us define the properties in class:
Class Catalog
{
#Properties
$Model = 'Fujitsu'
$Manufacturer = 'Life Book S Series'
}
$var = New-Object Catalog
$var
The following image shows the output of class, its members, and setting the property value:
Now, by changing the property value, we get the following output:
Now let's create a method with overloads. In the following example we have created a method name SetInformation that accepts two arguments $mdl and $mfgr and these are of string type.
Using $var.SetInformation command with no parenthesis will show the overload definitions of the method.
The code is as follows:
Class Catalog
{
#Properties
$Model = 'Fujitsu'
$Manufacturer = 'Life Book S Series'
SetInformation([String]$mdl,[String]$mfgr)
{
$this.Manufacturer = $mfgr
$this.Model = $mdl
}
}
$var = New-Object -TypeName Catalog
$var.SetInformation
#Output
OverloadDefinitions
-------------------
void SetInformation(string mdl, string mfgr)
Let's set the model and manufacturer using set information, as follows:
Class Catalog
{
#Properties
$Model = 'Fujitsu'
$Manufacturer = 'Life Book S Series'
SetInformation([String]$mdl,[String]$mfgr)
{
$this.Manufacturer = $mfgr
$this.Model = $mdl
}
}
$var = New-Object -TypeName Catalog
$var.SetInformation('Surface' , 'Microsoft')
$var
The output is illustrated in following image:
Inside the PowerShell class we can use PowerShell cmdlets as well. The following code is just to give a demo of using PowerShell cmdlet.
Class allows us to validate the parameters as well. Let's have a look at the following example:
Class Order
{
[ValidateSet("Red" , "Blue" , "Green")]
$color
[ValidateSet("Audi")]
$Manufacturer
Book($Manufacturer , $color)
{
$this.color = $color
$this.Manufacturer = $Manufacturer
}
}
The parameter $Color and $Manufacturer has ValidateSet property and has a set of values. Now let's use New-Object and set the property with an argument which doesn't belong to this set, shown as follows:
$var = New-Object Order
$var.color = 'Orange'
Now, we get the following error:
Exception setting "color": "The argument "Orange" does not belong to the set "Red,Blue,Green" specified by the ValidateSet attribute. Supply an argument that is in the set and then try the command again."
Let's set the argument values correctly to get the result using Book method, as follows:
$var = New-Object Order
$var.Book('Audi' , 'Red')
$var
The output is illustrated in the following figure:
A constructor is a special type of method that creates new objects. It has the same name as the class and the return type is void. Multiple constructors are supported, but each one takes different numbers and types of parameters. In the following code, let's see the steps to create a simple constructor in PowerShell that simply creates a user in the active directory.
Class ADUser {
$identity
$Name
ADUser($Idenity , $Name) {
New-ADUser -SamAccountName $Idenity -Name $Name
$this.identity = $Idenity
$this.Name = $Name
}
}
$var = [ADUser]::new('Dummy' , 'Test Case User')
$var
We can also hide the properties in PowerShell class, for example let's create two properties and hide one. In theory, it just hides the property but we can use the property as follows:
Class Hide {
[String]$Name
Hidden $ID
}
$var = [Hide]::new()
$var
The preceding code is illustrated in the following figure:
Additionally, we can carry out operations, such as Get and Set, as shown in the following code:
Class Hide {
[String]$Name
Hidden $ID
}
$var = [Hide]::new()
$var.Id = '23'
$var.Id
This returns output as 23.
To explore more about class use help about_Classes -Detailed.
In Windows PowerShell 5.0 a new cmdlet ConvertFrom-String has been introduced and it's available in Microsoft.PowerShell.Utility.
Using this command, we can parse the structured objects from any given string content. To see information, use help command with ConvertFrom-String -Detailed command.
The help has an incorrect parameter as PropertyName. Copy paste will not work, so use help ConvertFrom-String –Parameter * and read the parameter—it's actually PropertyNames.
Now, let's see an example of using ConvertFrom-String.
Let us examine a scenario where a team has a custom code which generates log files for daily health check-up reports of their environment. Unfortunately, the tool delivered by the vendor is an EXE file and no source code is available. The log file format is as follows:
"Error 4356 Lync" , "Warning 6781 SharePoint" , "Information 5436 Exchange",
"Error 3432 Lync" , "Warning 4356 SharePoint" , "Information 5432 Exchange"
There are many ways to manipulate this record but let's see how PowerShell cmdlet ConvertFrom-String helps us. Using the following code, we will simply extract the Type, EventID, and Server:
"Error 4356 Lync" , "Warning 6781 SharePoint" , "Information 5436 Exchange",
"Error 3432 Lync" , "Warning 4356 SharePoint" , "Information 5432 Exchange" |
ConvertFrom-String -PropertyNames Type , EventID, Server
Following figure shows the output of the code we just saw:
Okay, what's interesting in this? It's cool because now your output is a PSCustom object and you can manipulate it as required.
"Error 4356 Lync" , "Warning 6781 SharePoint" , "Information 5436 Exchange",
"Error 3432 SharePoint" , "Warning 4356 SharePoint" , "Information 5432 Exchange" |
ConvertFrom-String -PropertyNames Type , EventID, Server | ? {$_.Type -eq 'Error'}
An output in Lync and SharePoint has some error logs that needs to be taken care of on priority. Since, requirement varies you can use this cmdlet as required.
ConvertFrom-String has a delimiter parameter, which helps us to manipulate the strings as well. In the following example let's use the –Delimiter parameter that removes white space and returns properties, as follows:
"Chen V" | ConvertFrom-String -Delimiter "s" -PropertyNames "FirstName" , "SurName"
This results FirstName and SurName – FirstName as Chen and SurName as V
In the preceding example, we walked you through using template file to manipulate the string as we need. To do this we need to use the parameter –Template Content. Use help ConvertFrom-String –Parameter Template Content
Before we begin we need to create a template file. To do this let's ping a web site.
Ping www.microsoft.com and the output returned is, as shown:
Pinging e10088.dspb.akamaiedge.net [2.21.47.138] with 32 bytes of data:
Reply from 2.21.47.138: bytes=32 time=37ms TTL=51
Reply from 2.21.47.138: bytes=32 time=35ms TTL=51
Reply from 2.21.47.138: bytes=32 time=35ms TTL=51
Reply from 2.21.47.138: bytes=32 time=36ms TTL=51
Ping statistics for 2.21.47.138:
Packets: Sent = 4, Received = 4, Lost = 0 (0% loss),
Approximate round trip times in milli-seconds:
Minimum = 35ms, Maximum = 37ms, Average = 35ms
Now, we have the information in some structure. Let's extract IP and bytes; to do this I replaced the IP and Bytes as {IP*:2.21.47.138}
Pinging e10088.dspb.akamaiedge.net [2.21.47.138] with 32 bytes of data:
Reply from {IP*:2.21.47.138}: bytes={[int32]Bytes:32} time=37ms TTL=51
Reply from {IP*:2.21.47.138}: bytes={[int32]Bytes:32} time=35ms TTL=51
Reply from {IP*:2.21.47.138}: bytes={[int32]Bytes:32} time=36ms TTL=51
Reply from {IP*:2.21.47.138}: bytes={[int32]Bytes:32} time=35ms TTL=51
Ping statistics for 2.21.47.138:
Packets: Sent = 4, Received = 4, Lost = 0 (0% loss),
Approximate round trip times in milli-seconds:
Minimum = 35ms, Maximum = 37ms, Average = 35ms
ConvertFrom-String has a debug parameter using which we can debug our template file. In the following example let's see the debugging output:
ping www.microsoft.com | ConvertFrom-String -TemplateFile C:TempTemplate.txt -Debug
As we mentioned earlier PowerShell 5.0 is a Preview release and has few bugs. Let's ignore those for now and focus on the features, which works fine and can be utilized in environment.
In this topic, we will walk you through the features of package management, which is another great feature of Windows Management Framework 5.0. This was introduced in Windows 10 and was formerly known as OneGet.
Using package management we can automate software discovery, installation of software, and inventorying. Do not think about Software Inventory Logging (SIL) for now.
As we know, in Windows Software Installation, technology has its own way of doing installations, for example MSI type, MSU type, and so on. This is a real challenge for IT professionals and developers, to think about the unique automation of software installation or deployment. Now, we can do it using package management module.
To begin with, let's see the package management Module using the following code:
Get-Module -Name PackageManagement
The output is illustrated as follows:
Yeah, well we got an output that is a binary module. Okay, how to know the available cmdlets and their usage? PowerShell has the simplest way to do things, as shown in the following code:
Get-Module -Name PackageManagement
The available cmdlets are shown in the following image:
Package Providers are the providers connected to package management (OneGet) and package sources are registered for providers. To view the list of providers and sources we use the following cmdlets:
Now, let's have a look at the available packages—in the following example I am selecting the first 20 packages, for easy viewing:
Okay, we have 20 packages so using Install-Package cmdlet, let us now install WindowsAzurePowerShell on our Windows 2012 Server.
We need to ensure that the source are available prior to any installation. To do this just execute the cmdlet Get-PackageSource. If the chocolatey source didn't come up in the output, simply execute the following code—do not change any values. This code will install chocolatey package manager on your machine. Once the installation is done we need to restart the PowerShell:
Invoke-Expression ((New-Object System.Net.WebClient).DownloadString('https://chocolatey.org/install.ps1'))
Find-Package -Name WindowsAzurePowerShell | Install-Package -Verbose
The command we just saw shows the confirmation dialog for chocolatey, which is the package source, as shown in the following figure:
Click on Yes and install the package.
Following are the steps represented in the figure that we just saw:
Installation successful.
Windows Server 2012 has .NET 4.5 in the box by default, so the verbose turned up as False for .NET 4.5, which says PowerShell not installed but WindowsAzurePowerShell is installed successfully.
If you are trying to install the same package and the same version that is available on your system – the cmdlet will skip the installation.
Find-Package -Name PowerShell Here | Install-Package -Verbose
VERBOSE: Skipping installed package PowerShellHere 0.0.3
Explore all the package management cmdlets and automate your software deployments.
PowerShell Get-Module is a module available in Windows PowerShell 5.0 preview. Following are few more modules:
Add your own custom repository with Register-PSRepository
The following screenshot shows the additional cmdlets that are available:
This will allow us to find a module from PowerShell gallery and install it in our environment. PS gallery is a repository of modules. Using Find-Module cmdlet we get a list of module available in the PS gallery. Pipe and install the required module, alternatively we can save the module and examine it before installation, to do this use Save-Module cmdlet.
The following screenshot illustrates the installation and deletion of the xJEA module:
We can also publish module in the PS gallery, which will be available over the internet to others.
This is not a great module. All it does is get user-information from an active directory for the same account name—creates a function and saves it as PSM1 in module folder. In order to publish the module in PS gallery, we need to ensure that the module has manifest. Following are the steps to publish your module:
Publish your module using the Publish-PSModule cmdlet.
Following figure shows modules that are currently published:
Following figure shows the commands to publish modules:
In this article, we saw that Windows PowerShell 5.0 preview has got a lot more significant features, such as enhancement in PowerShell DSC, cmdlets improvements and new cmdlets, ISE support transcriptions, support class, and using class. We can create Custom DSC resources with easy string manipulations, A new Network Switch module is introduced using which we can automate and manage Microsoft signed network switches.
Further resources on this subject: