










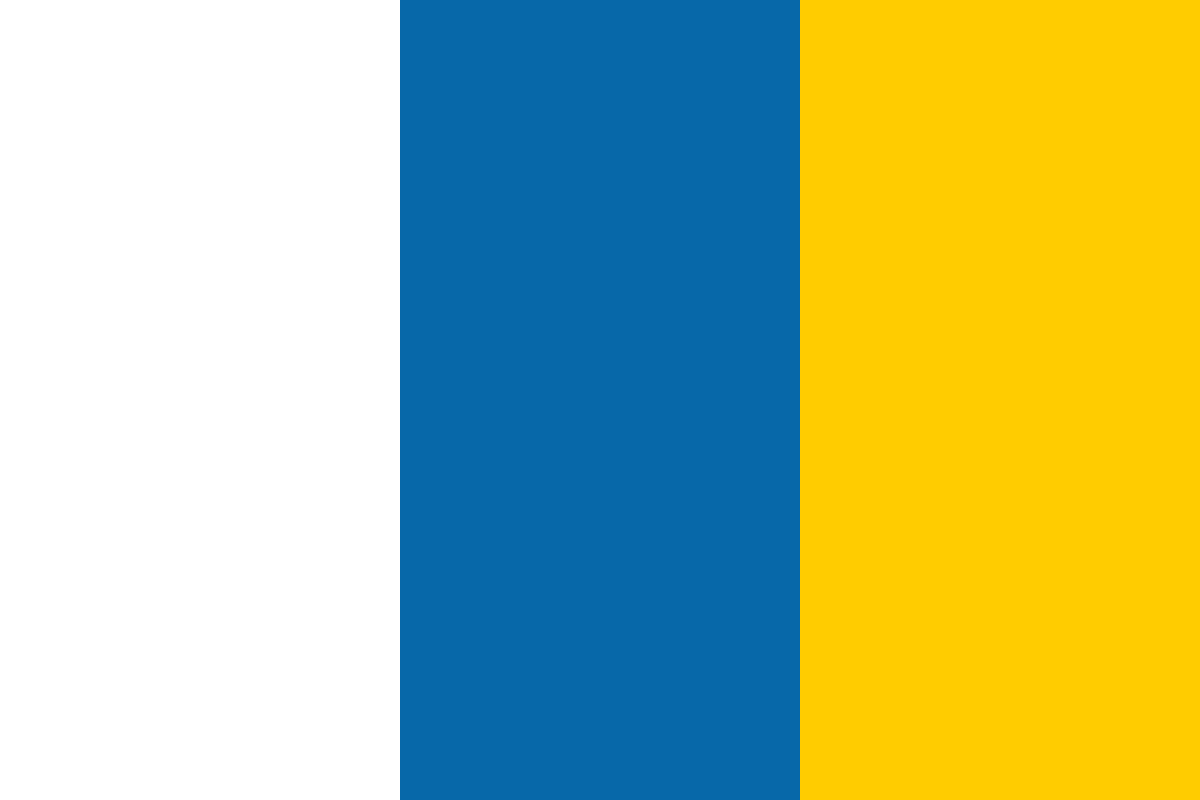

































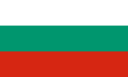








Read more about this book |
(For more resources on this subject, see here.)
In this article, we will look at some advanced techniques that can be used to enhance the functionality of web applications.
We will create a few examples where we will search for images the from Flickr and videos from YouTube using their respective APIs. We will parse a RSS feed XML using jQuery and learn to create an endless scrolling page like Google reader or the new interface of Twitter.
Besides this, you will also learn to create a jQuery plugin, which you can use independently in your applications.
Browsers do not allow scripts to send cross-domain requests due to security reasons. This means a script at domain http://www.abc.com cannot send AJAX requests to http://www.xyz.com.
This recipe will show how you can overcome this limitation by using a PHP script on the server side. We will create an example that will search Flickr for images. Flickr will return a JSON, which will be parsed by jQuery and images will be displayed on the page. The following screenshot shows a JSON response from Flickr:
Create a directory for this article and name it as Article9. In this directory, create a folder named Recipe1.
Also get an API key from Flickr by signing up at http://www.flickr.com/services/api/keys/.
<html>
<head>
<title>Flickr Image Search</title>
<style type="text/css">
body { font-family:"Trebuchet MS",verdana,arial;width:900px;
}
fieldset { width:333px; }
ul{ margin:0;padding:0;list-style:none; }
li{ padding:5px; }
span{ display:block;float:left;width:150px; }
#results li{ float:left; }
.error{ font-weight:bold; color:#ff0000; }
</style>
</head>
<body>
<form id="searchForm">
<fieldset>
<legend>Search Criteria</legend>
<ul>
<li>
<span>Tag</span>
<input type="text" name="tag" id="tag"/>
</li>
<li>
<span>Number of images</span>
<select name="numImages" id="numImages">
<option value="20">20</option>
<option value="30">30</option>
<option value="40">40</option>
<option value="50">50</option>
</select>
</li>
<li>
<span>Select a size</span>
<select id="size">
<option value="s">Small</option>
<option value="t">Thumbnail</option>
<option value="-">Medium</option>
<option value="b">Large</option>
<option value="o">Original</option>
</select>
</li>
<li>
<input type="button" value="Search" id="search"/>
</li>
</ul>
</fieldset>
</form>
<ul id="results">
</ul>
</body>
</html>
The following screenshot shows the form created:
<script type="text/javascript" src="../jquery.js"></script>
<script type="text/javascript">
$(document).ready(function()
{
$('#search').click(function()
{
if($.trim($('#tag').val()) == '')
{
$('#results').html('<li class="error">Please provide
search criteria</li>');
return;
}
$.post(
'search.php',
$('#searchForm').serialize(),
showImages,
'json'
);
});
function showImages(response)
{
if(response['stat'] == 'ok')
{
var photos = response.photos.photo;
var str= '';
$.each(photos, function(index,value)
{
var farmId = value.farm;
var serverId = value.server;
var id = value.id;
var secret = value.secret;
var size = $('#size').val();
var title = value.title;
var imageUrl = 'http://farm' + farmId +
'.static.flickr.com/' + serverId + '/' + id + '_' +
secret + '_' + size + '.jpg';
str+= '<li>';
str+= '<img src="' + imageUrl + '" alt="'
+ title + '" />';
str+= '</li>';
});
$('#results').html(str);
}
else
{
$('#results').html('<li class="error">an error
occured</li>');
}
}
});
</script>
<?php
define('API_KEY', 'your-API-key-here');
$url = 'http://api.flickr.com/services/rest/?method=flickr.
photos.search';
$url.= '&api_key='.API_KEY;
$url.= '&tags='.$_POST['tag'];
$url.= '&per_page='.$_POST['numImages'];
$url.= '&format=json';
$url.= '&nojsoncallback=1';
header('Content-Type:text/json;');
echo file_get_contents($url);
?>
<html>
<head>
<title>Youtube Video Search</title>
<style type="text/css">
body { font-family:"Trebuchet MS",verdana,arial;width:900px;
}
fieldset { width:333px; }
ul{ margin:0;padding:0;list-style:none; }
li{ padding:5px; }
span{ display:block;float:left;width:150px; }
#results ul li{ float:left; background-color:#483D8B;
color:#fff;margin:5px; width:120px; }
.error{ font-weight:bold; color:#ff0000; }
img{ border:0}
</style>
</head>
<body>
<form id="searchForm">
<fieldset>
<legend>Search Criteria</legend>
<ul>
<li>
<span>Enter query</span>
<input type="text" id="query"/>
</li>
<li>
<input type="button" value="Search" id="search"/>
</li>
</ul>
</fieldset>
</form>
<div id="results">
</div>
</body>
</html>
On clicking the Search button, form values are sent to the PHP file search.php. Now, we have to contact Flickr and search for images. Flickr API provides several methods for accessing images. We will use the method flickr.photos.search to search by tag name. Along with method name we will have to send the following parameters in the URL:
http://www.flickr.com/services/api/keys/.
Once the URL is complete we can contact Flickr to get results. To get the results' we will use the PHP function file_get_contents, which will get the results JSON from the specified URL. This JSON will be echoed to the browser.
jQuery will receive the JSON in callback function showImages. This function first checks the status of the response. If the response is OK, we get the photo elements from the response and we can iterate over them using jQuery's $.each method. To display an image, we will have to get its URL first, which will be created by combining different values of the photo object. According to Flickr API specification, an image URL can be constructed in the following manner:
http://farm{farm-id}.static.flickr.com/{server-id}/{id}_{secret}_[size].jpg
So we get the farmId, serverId, id, and secret from the photo element. The size can be one of the following:
We have already selected the image size from the select box in the form. By combining all these values, we now have the Flickr image URL. We wrap it in a li element and repeat the process for all images. Finally, we insert the constructed images into the results li.
The previous recipe demonstrated the use of a PHP file as a proxy for querying cross-domain URLs. This recipe will show the use of JSONP to query cross-domain URLs from jQuery itself.
We will create an example that will search for the videos from YouTube and will display them in a list. Clicking on a video thumbnail will open a new window that will take the user to the YouTube website to show that video.
The following screenshot shows a sample JSON response from YouTube:
Create a folder named Recipe2 inside the Article9 directory.
<script type="text/javascript" src="../jquery.js"></script>
<script type="text/javascript">
$(document).ready(function()
{
$('#search').click(function()
{
var query = $.trim($('#query').val());
if(query == '')
{
$('#results').html('<li class="error">Please enter
a query.</li>');
return;
}
$.get(
'http://gdata.youtube.com/feeds/api/videos?q=' + query +
'&alt=json-in-script',
{},
showVideoList,
'jsonp'
);
});
});
function showVideoList(response)
{
var totalResults =
response['feed']['openSearch$totalResults']['$t'];
if(parseInt(totalResults,10) > 0)
{
var entries = response.feed.entry;
var str = '<ul>';
for(var i=1; i< entries.length; i++)
{
var value = entries[i];
var title = value['title']['$t'];
var mediaGroup = value['media$group'];
var videoURL = mediaGroup['media$player'][0]['url'];
var thumbnail = mediaGroup['media$thumbnail'][0]['url'];
var thumbnailWidth =
mediaGroup['media$thumbnail'][0]['width'];
var thumbnailHeight =
mediaGroup['media$thumbnail'][0]['height'];
var numComments =
value['gd$comments']['gd$feedLink']['countHint'];
var rating =
parseFloat(value['gd$rating']['average']).toFixed(2);
str+= '<li>';
str+= '<a href="' + videoURL + '" target="_blank">';
str+= '<img src="'+thumbNail+'" width="'+thumbNailWidth+'"
height="'+thumbNailWidth+'" title="' + title + '" />';
str+= '</a>';
str+= '<hr>';
str+= '<p style="width: 120px; font-size: 12px;">Comments:
' + numComments + '';
str+= '<br/>';
str+= 'Rating: ' + rating;
str+= '</p>';
str+= '</li>';
}
str+= '</ul>';
$('#results').html(str);
}
else
{
$('#results').html('<li class="error">No results.</li>');
}
}
</script>
http://gdata.youtube.com/feeds/api/videos?q=' + query +
'&alt=json-in-script
script tags are an exception to cross-browser origin policy. We can take advantage of this by requesting the URL from the src attribute of a script tag and by wrapping the raw response in a callback function. In this way the response becomes JavaScript code instead of data. This code can now be executed on the browser.
The URL for YouTube video search is as follows:
http://gdata.youtube.com/feeds/api/videos?q=' + query + '&alt=json-in-script
Parameter q is the query that we entered in the textbox and alt is the type of response we want. Since we are using JSONP instead of JSON, the value for alt is defined as json-in-script as per YouTube API specification. On getting the response, the callback function showVideoList executes. It checks whether any results are available or not. If none are found, an error message is displayed. Otherwise, we get all the entry elements and iterate over them using a for loop. For each video entry, we get the videoURL, thumbnail, thumbnailWidth, thumbnailHeight, numComments, and rating. Then we create the HTML from these variables with a list item for each video. For each video an anchor is created with href set to videoURL. The video thumbnail is put inside the anchor and a p tag is created where we display the number of comments and rating for a particular video. After the HTML has been created, it is inserted in the DIV with ID results.
About JSONP
You can read more about JSONP at the following websites: