










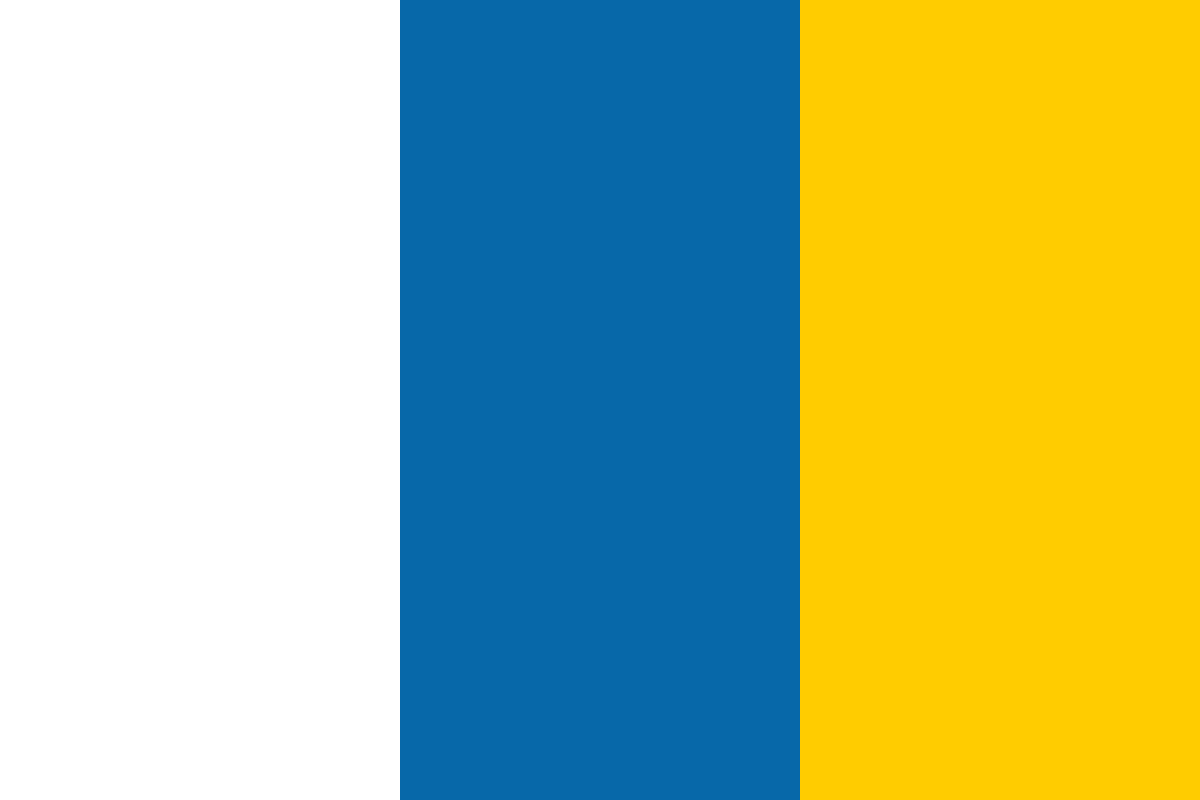

































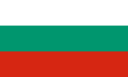








In this article by, Francesco Malatesta author of the book, Learning Laravel’s Eloquent, we will learn everything about Eloquent, starting from the very basics and going through models, relationships, and other topics. You probably started to like it and think about implementing it in your next project.
In fact, creating an application without a single SQL query is tempting. Maybe you also showed it to your boss and convinced him/her to use it in your next production project.
However, there is a little problem. Yeah, the next project isn't so new. It already exists, and, despite everything, it doesn't use Laravel! You start to shiver. This is so sad because you passed the last week studying this new ORM, a really cool one, and then moving forward.
There is always a solution! You are a developer! Also, the solution is not so hard to find. If you want, you can use Eloquent without Laravel.
Actually, Laravel is not a monolithic framework. It is made up of several, separate parts, which are combined together to build something greater. However, nothing prevents you from using only selected packages in another application.
(For more resources related to this topic, see here.)
So, what are we going to see in this article?
First of all, we will explore the structure of the database package and see what is inside it. Then, you will learn how to install the illuminate/database package separately for your project and how to configure it for the first use.
Then, you will encounter some examples. First of all, we will look at the Eloquent ORM. You will learn how to define models and use them.
Having done this, as a little extra, I will show you how to use the Query Builder (remember that the "illuminate/database" package isn't just Eloquent). Maybe you would also enjoy the Schema Builder class. I will cover it, don't worry!
We will cover the following:
As I mentioned before, the key step in order to use Eloquent in your application without Laravel is to use the "illuminate/database" package.
So, before we install it, let's examine it a little.
You can see the package contents here: https://github.com/illuminate/database.
So, this is what you will probably see:
Folder |
Description |
Capsule |
The capsule manager is a fundamental component. It instantiates the service container and loads some dependencies. |
Connectors |
The database package can communicate with various DB systems. For instance, SQLite, MySQL, or PostgreSQL. Every type of database has its own connector. This is the folder in which you will find them. |
Console |
The database package isn't just Eloquent with a bunch of connectors. In this specific folder, you will find everything related to console commands, such as artisan db:seed or artisan migrate. |
Eloquent |
Every single Eloquent class is placed here. |
Migrations |
Don't confuse this with the Console folder. Every class related to migrations is stored here. When you type artisan migrate in your terminal, you are calling a class that is placed here. |
Query |
The Query Builder is placed here. |
Schema |
Everything related to the Schema Builder is placed here. |
In the main folder, you will also find some other files. However, don't worry as you don't need to know what they are.
If you open the composer.json file, take a look at the following "require" section:
"require": { "php": ">=5.4.0", "illuminate/container": "5.1.*", "illuminate/contracts": "5.1.*", "illuminate/support": "5.1.*", "nesbot/carbon": "~1.0" },
As you can see, the database package has some prerequisites that you can't avoid. However, the container is quite small, and it is the same for contracts (just some interfaces) and "illuminate/support".
Eloquent uses Carbon (https://github.com/briannesbitt/Carbon) to deal with dates in a smarter way. So, if you are seeing this for the first time and you are confused, don't worry! Everything is all right.
Now that you know what you can find in this package, let's see how to install it and configure it for the first time.
Let's start with the setup. First of all, we will install the package using composer as usual. After that, we will configure the capsule manager in order to get started.
Installing the "illuminate/database" package is really easy.
All you have to do is to add "illuminate/database" to the "require" section of your composer.json file, like this:
"require": { "illuminate/database": "5.0.*", },
Then type composer update in to your terminal, and wait for a few seconds.
Another way is to include it with the shortcut in your project folder, obviously from the terminal:
composer require illuminate/database
No matter which method you chose, you just installed the package.
Time to use the capsule manager! In your project, you will use something like this to get started:
use Illuminate\Database\Capsule\Manager as Capsule; $capsule = new Capsule; $capsule->addConnection([ 'driver' => 'mysql', 'host' => 'localhost', 'database' => 'database', 'username' => 'root', 'password' => 'password', 'charset' => 'utf8', 'collation' => 'utf8_unicode_ci', 'prefix' => '', ]); // Set the event dispatcher used by Eloquent models... (optional) use Illuminate\Events\Dispatcher; use Illuminate\Container\Container; $capsule->setEventDispatcher(new Dispatcher(new Container));
The config syntax I used is exactly the same you can find in the config/database.php configuration file. The only difference is that this time you are explicitly using an instance of the capsule manager in order to do everything.
In the second part of the code, I am setting up the event dispatcher. You must do this if events are required from your project.
However, events are not included by default in this package, so you will have to manually add the "illuminate/events" dependency to your composer.json file.
Now, the final step!
Add this code to your setup file:
// Make this Capsule instance available globally via static methods... (optional) $capsule->setAsGlobal(); // Setup the Eloquent ORM... (optional; unless you've used setEventDispatcher()) $capsule->bootEloquent();
With setAsGlobal() called on the capsule manager, you can set it as a global component in order to use it with static methods. You may like it or not; the choice is yours. The final line starts up Eloquent, so you will need it.
However, this is also an optional instruction. In some situations you may need the Query Builder only.
Then there is nothing else to do! Your application is now configured with the database package (and Eloquent)!
Using the Eloquent ORM in a non-Laravel application is not a big change. All you have to do is to declare your model as you are used to doing. Then, you need to call it and use it as you are used to.
Here is a perfect example of what I am talking about:
use Illuminate\Database\Eloquent\Model; class Book extends Model { ... // some attributes here… protected $table = 'my_books_table'; // some scopes here... public function scopeNewest() { // query here... } ... }
Exactly as you did with Laravel, the package you are using is the same. So, no worries! If you want to use the model you just created, then use the following:
$books = Book::newest()->take(5)->get();
This also applies for relationships, observers, and so on. Everything is the same.
In order to use the database package and ORM exactly, you would do the same thing you did in Laravel; remember to set up the project structure in a way that follows the PSR-4 autoloading convention.
It's not just about the ORM; with the database package, you can also use the Query and the Schema Builders. Let's discover how!
The Query Builder is also very easy to use. The only difference, this time, is that you are passing through the capsule manager object, like this:
$books = Capsule::table('books') ->where('title', '=', "Michael Strogoff") ->first();
However, the result is still the same.
Also, if you like the DB facade in Laravel, you can use the capsule manager class in the same way:
$book = Capsule::select('select title, pages_count from books where id = ?', array(12));
Now, without Laravel, you don't have migrations.
However, you can still use the Schema Builder without Laravel. Like this:
Capsule::schema()->create('books', function($table)
{
$table->increments(''id');
$table->string(''title'', 30);
$table->integer(''pages_count'');
$table->decimal(''price'', 5, 2);.
$table->text(''description'');
$table->timestamps();
});
Previously, you used to call the create() method of the Schema facade. This time is a little different: you will use the create() method, chained to the schema() method of the Capsule class.
Obviously, you can use any Schema class method in this way. For instance, you could call something like following:
Capsule::schema()->table('books', function($table){ $table->string('title', 50)->change(); $table->decimal('special_price', 5, 2); });
And you are good to go!
Remember that if you want to unlock some Schema Builder-specific features you will need to install other dependencies.
For example, do you want to rename a column? You will need the doctrine/dbal dependency package.
I decided to add this article because many people ask me how to use Eloquent without Laravel. Mostly because they like the framework, but they can't migrate an already started project in its entirety.
Also, I think that it's cool to know, in a certain sense, what you can find under the hood.
It is always just about curiosity. Curiosity opens new paths, and you have a choice to solve a problem in a new and more elegant way.
In these few pages, I just scratched the surface. I want to give you some advice: explore the code. The best way to write good code is to read good code.
Further resources on this subject: