










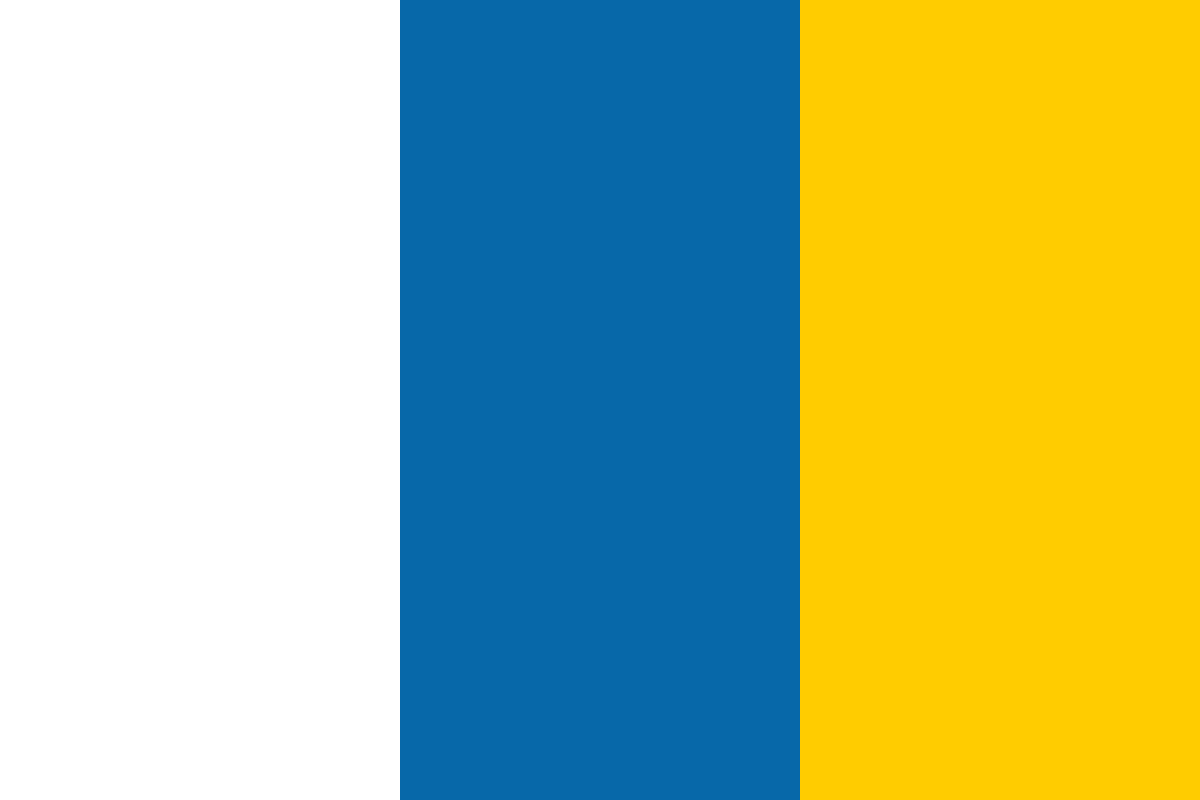

































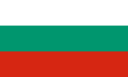








To start with we'll create a DataGrid which will contain a first name and a last name. We will add a Panel container to place our DataGrid into. Here is what our initial app looks like:
<?xml version="1.0" encoding="utf-8"?> <mx:Application layout="absolute"> <mx:Panel title="Pop Up Window" > <mx:DataGrid width="100%" height="100%"> <mx:columns> <mx:DataGridColumn headerText="First Name"/> <mx:DataGridColumn headerText="Last Name"/> </mx:columns> </mx:DataGrid> </mx:Panel> </mx:Application>
You'll notice the common Application tag along with the Panel. Immediately inside the Panel is our all important DataGrid. Note, if you are not familiar with the basics of Flex then stop here and head over to the Adobe site for an introduction. Although this tutorial is not complex, I wont be able to focus on the fundamentals.
I've set the DataGrid width and height attibutes to 100%. This will force it to expand to the size and width of the panel. You can make this application full screen by setting the same attributes on the Panel tag. Inside our DataGrid tag is the columns tag. Here we can describe what each column of our DataGrid will contain. In this case, one column for first name and one column for the last name. Here is a first look at our app:
The ease of Flex has allowed us to create this simple user interface with little effort. Now we come to a point where we need to add data to the DataGrid. The easiest way to do this is to use the dataProvider attribute. We will add an ArrayCollection Object to the script portion of our application to hold all the names which will appear in our DataGrid. The DataGrid and the accompanying ArrayCollection will look something like this:
<mx:DataGrid id="names" width="100%" height="100%" dataProvider="{namesDP}"> <mx:columns> <mx:DataGridColumn headerText="First Name" dataField="firstName"/> <mx:DataGridColumn headerText="Last Name" dataField="lastName"/> </mx:columns> </mx:DataGrid>
[Bindable]
public var namesDP:ArrayCollection =
new ArrayCollection ([
{firstName:'Keith',lastName:'Lee'},
{firstName:'Ira',lastName:'Glass'},
{firstName:'Christopher',lastName:'Rossin'},
{firstName:'Mary',lastName:'Little'},
{firstName:'Charlie',lastName:'Wagner'},
{firstName:'Cali',lastName:'Gonia'},
{firstName:'Molly',lastName:'Ivans'},
{firstName:'Amber',lastName:'Johnson'}]);
The dataProvider attribute binds the DataGrid to the nameDP ArrayCollection. The ArrayCollection constructor's argument is an array of objects. Each object has a firstName and lastName property. If you wanted to expand on this application, you can add more data to this Array. You should also notice that the DataGridColumn tags also have an addition. We've set the dataField equal to the property in the dataProvider. The dataField is the real key in populating the DataGrid. Here is what our populated DataGrid looks like:
We've now created the foundation of our application.
By default the DataGrid doubleclick event is turned off. This means when a user doubleclicks an entry nothing will happen. In order to tell the DataGrid to trigger the doublelcick event we need to set the doubleClick and doubleClickEnabled attributes of the DataGrid tag. It looks like this:
<mx:DataGrid width="100%" height="100%" doubleClick="showPopUp(event)" doubleClickEnabled="true">
I've set the doubleClick attribute to a method called showPopUp. We've not written this method yet, but it will be responsible for displaying the PopUp window which will allow editing of the DataGrid data. For now, let's add the Script tag and an empty showPopUp method:
<mx:Script> <![CDATA[
import mx.events.ItemClickEvent;
public function showPopUp(event:MouseEvent):void{
// show the popup
}
]]>
</mx:Script>
The Script tag is a child of the Application tag.