










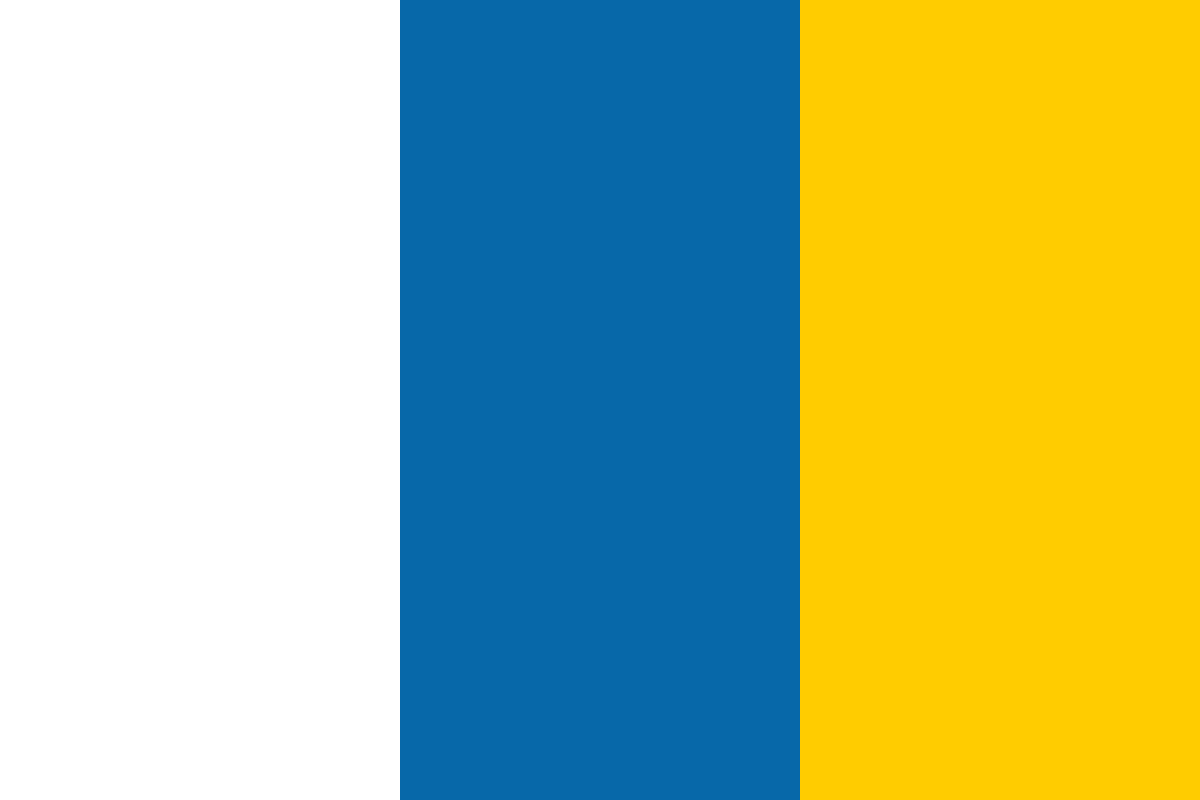

































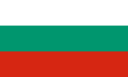








These days e-commerce platforms are widely available. However, as common as they might be, there are instances that after investing a significant amount of time learning how to use a specific tool you might realize that it can not fit your unique e-commerce needs as it promised. Hence, a great advantage of building your own application with an agile framework is that you can quickly meet your immediate and future needs with a system that you fully understand.
Adrian Mejia Rosario, the author of the book, Building an E-Commerce Application with MEAN, shows us how MEAN stack (MongoDB, ExpressJS, AngularJS and NodeJS) is a killer JavaScript and full-stack combination. It provides agile development without compromising on performance and scalability. It is ideal for the purpose of building responsive applications with a large user base such as e-commerce applications.
Let's have a look at a project using MEAN.
(For more resources related to this topic, see here.)
The applications built with the angular-fullstack generator have many files and directories. Some code goes in the client, other executes in the backend and another portion is just needed for development cases such as the tests suites. It’s important to understand the layout to keep the code organized.
The Yeoman generators are time savers! They are created and maintained by the community following the current best practices. It creates many directories and a lot of boilerplate code to get you started. The numbers of unknown files in there might be overwhelming at first.
On reviewing the directory structure created, we see that there are three main directories: client, e2e and server:
This is the overview of the file structure of this project:
meanshop
├── client
│ ├── app - App specific components
│ ├── assets - Custom assets: fonts, images, etc…
│ └── components - Non-app specific/reusable components
│
├── e2e - Protractor end to end tests
│
└── server
├── api - Apps server API
├── auth - Authentication handlers
├── components - App-wide/reusable components
├── config - App configuration
│ └── local.env.js - Environment variables
│ └── environment - Node environment configuration
└── views - Server rendered views
You might be already familiar with a number of tools used in this project. If that’s not the case, you can read the brief description here.
AngularJS comes with a default test runner called Karma and we are going going to leverage its default choices:
The following are some of the tools/libraries that we are going to use in order to increase our productivity:
We have package managers for our third-party backend and frontend modules. They are as follows:
If you have followed the exact steps to scaffold our app you will have the following frontend components installed:
Let’s take a pause from the terminal. In any project, before starting coding, we need to spend some time planning and visualizing what we are aiming for. That’s exactly what we are going to do, draw some wireframes that walk us through the app.
Our e-commerce app, MEANshop, will have three main sections:
The home page will contain featured products, navigation, menus, and basic information, as you can see in the following image:
Figure 2 - Wireframe of the homepage
This section will show all the products, categories, and search results.
Figure 3 - Wireframe of the products page
You need to be a registered user to access the back office section, as shown in the following figure:
Figure 4 - Wireframe of the login page
After you login, it will present you with different options depending on the role. If you are the seller, you can create new products, such as the following:
Figure 5 - Wireframe of the Product creation page
If you are an admin, you can do everything that a seller does (create products) plus you can manage all the users and delete/edit products.
There’s no better way than to learn new concepts and technologies while developing something useful with it. This is why we are building a real-time e-commerce application from scratch. However, there are many kinds of e-commerce apps. In the following sections we will delimit what we are going to do.
Even the largest applications that we see today started small and grew their way up. The minimum viable product (MVP) is strictly the minimum that an application needs to work on. In the e-commerce example, it will be:
This is strictly the minimum requirement to get an e-commerce site working. We are going to start with these but by no means will we stop there. We will keep adding features as we go and build a framework that will allow us to extend the functionality with high quality.
We are going to capture our requirements for the e-commerce application with user stories. A user story is a brief description of a feature told from the perspective of a user where he expresses his desire and benefit in the following format:
As a <role>, I want <desire> [so that <benefit>]
User stories and many other concepts were introduced with the Agile Manifesto. Learn more at https://en.wikipedia.org/wiki/Agile_software_development
Here are the features that we are planning to develop through this book that have been captured as user stories:
All these stories might seem verbose but they are useful in capturing requirements in a consistent way. They are also handy to develop test cases against it.
Now that we have a gist of an e-commerce app with MEAN, lets build a full-fledged e-commerce project with Building an E-Commerce Application with MEAN.
Further resources on this subject: