










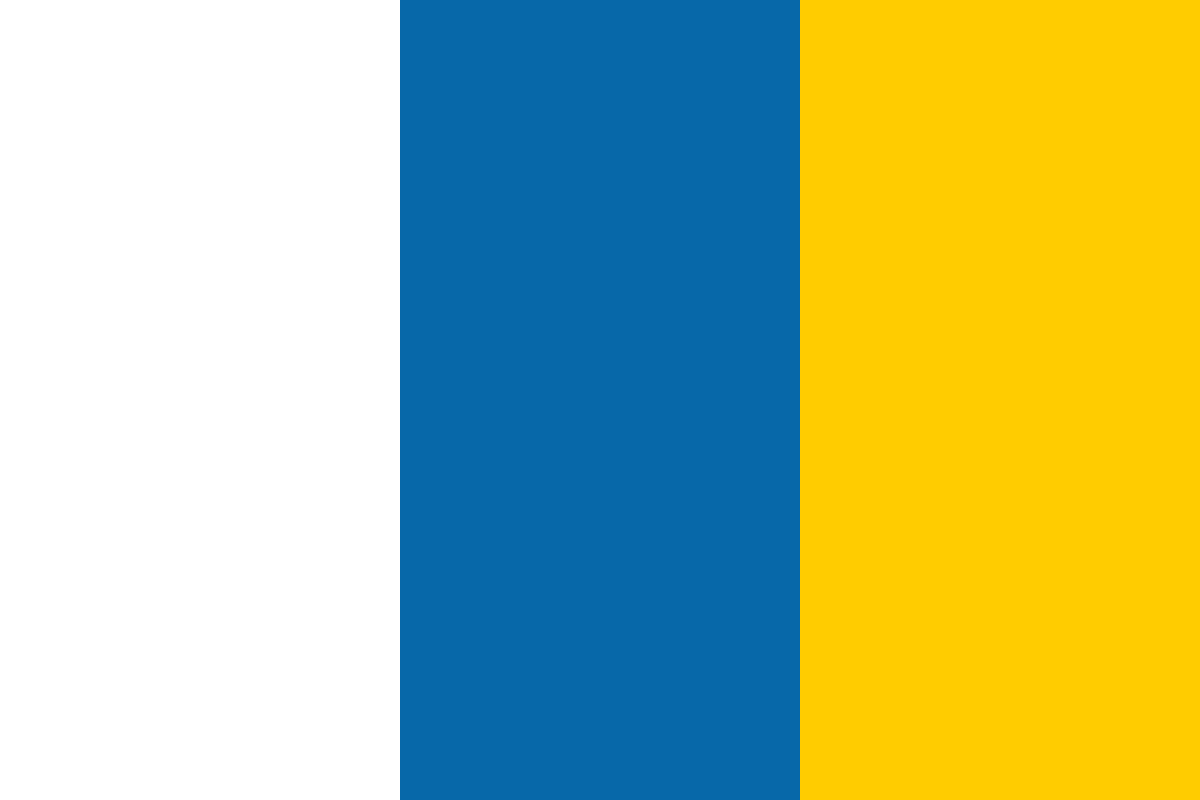

































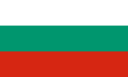








(For more resources related to this topic, see here.)
QuickTest Object property for a Web object allows us to get a reference to the DOM object, and can perform any operation on a DOM object. For example, the following code shows that the object on the page allows retrieving the element by name username, that is, the textbox in the next step assigns the value as ashish.
Set Obj = Browser("Tours").Page("Tours").Object.getElementsByName("userName) 'Get the length of the objects Print obj.length ' obj(0).value="ashish"
The following code snippet shows various operations that can be performed using the Object property of the web object:
'Retrieve all the link elements in a web page Set links = Browser ("Mercury Tours").Page("Mercury Tours").Object.links 'Length property provide total number of links For i =0 to links.length -1 Print links(i).toString() 'Print the value of the links Next Get the web edit object and set the focus on it Set MyWebEdit = Browser("Tours").Page("Tours").WebEdit("username"). Object MyWebEdit.focus 'Retrieve the html element by its name obj = Browser("Tours").Page("Tours").Object. getElementsByName("userName" ) 'set the value to the retrieved object obj.value ="ashish" 'Retrieve the total number of the images in the web pages set images = Browser("Mercury Tours").Page("Mercury Tours").Object.images print images.length 'Clicking on the Image obj = Browser("Tours").Page("Mercury Tours").Object.getElementsByName("login") obj.click 'Selecting the value from the drop down using selectedIndex method Browser("Flight").Page("Flight).WebList("pass.0.meal").Object. selectedIndex =1 'Click on the check box Browser("Flight").Page("Flight").WebCheckBox("ticketLess").Object. click
QTP allows firing of the events on the web objects:
Browser("The Fishing Website fishing").Page("The Fishing Website fishing").Link("Link").FireEvent "onmouseover"
The following example uses the FireEvent method to trigger the onpropertychange event on a form:
Browser("New Page").Page("New Page").WebElement("html tag:=Form").FireEvent "onpropertychange"
QTP allows executing JavaScript code. There are two JavaScript functions that allow us to interact with web pages. We can retrieve objects and perform the actions on them or we can retrieve the properties from the element on the pages:
RunScript executes the JavaScript code, passed as an argument to this function.
The following example shows how the RunScript method calls the ImgCount method, which returns the number of images in the page:
length = Browser("Mercury Tours").Page("Mercury Tours").RunScript("ImgCount(); function ImageCount() {var list = document.getElementsByTagName('img'); return list.length;}") print "The total number of images on the page is " & length
RunScriptsFormFile uses the full path of the JavaScript files to execute it. The location can be an absolute or relative filesystem path or a quality center path.
The following is a sample JavaScript file (logo.js):
var myNode = document.getElementById("lga"); myNode.innerHTML = '';
Use the logo.js file, as shown in the following code:
Browser("Browser").Page("page").RunScriptFromFile "c:logo.js" 'Check that the Web page behaves correctly If Browser("Browser").Page("page").Image("Image").Exist Then Reporter.ReportEvent micFail, "Failed to remove logo" End If
The above example uses the RunScriptFromFile method to remove a DOM element from a web page and checks if the page still behaves correctly when the DOM element has been removed.
XPath allows navigating and finding elements and attributes in an HTML document. XPath uses path expressions to navigate in HTML documents. QTP allows XPath to create the object description; for example:
xpath:=//input[@type='image' and contains(@name,'findFlights')
In the following section, we will learn the various XPath terminologies and methodologies to find the objects using XPath.
XPath uses various terms to define elements and their relationships among HTML elements, as shown in the following table:
Atomic values |
Atomic values are nodes with no children or parent |
Ancestors |
A node's parent, parent's parent, and so on |
Descendants |
A node's children, children's children, and so on |
Parent |
Each element and attribute has one parent |
Children |
Element nodes may have zero, one, or more children |
Siblings |
Nodes that have the same parent |
A path expression allows selecting nodes in a document. The commonly used path expressions are shown in the following table:
Symbol |
Meaning |
/(slash) |
Select elements relative to the root node |
//(double slash) |
Select nodes in the document from the current node that match the selection irrespective of its position |
.(dot) |
Represents the current node |
.. |
Represents the parent of the current node |
@ |
Represents an attribute |
nodename |
Selects all nodes with the name "nodename" |
Slash (/) is used in the beginning and it defines an absolute path; for example, /html/head/title returns the title tag. It defines ancestor and descendant relationships if used in the middle; for example, //div/table that returns the div containing a table.
Double slash (//) is used to find a node in any location; for example, //table returns all the tables. It defines a descendant relationship if used in the middle; for example, /html//title returns the title tag, which is descendant of the html tag.
Refer to the following table to see a few more examples with their meanings:
Expression |
Meaning |
//a |
Find all anchor tags |
//a//img |
List the images that are inside a link |
//img/@alt |
Show all the alt tags |
//a/@href |
Show the href attribute for every link |
//a[@*] |
Anchor tab with any attribute |
//title/text() or /html/head/title/text() |
Get the title of a page |
//img[@alt] |
List the images that have alt tags |
//img[not(@alt)] |
List the images that don't have alt tags |
//*[@id='mainContent'] |
Get an element with a particular CSS ID |
//div [not(@id="div1")] |
Make an array element from the XPath |
//p/.. |
Selects the parent element of p (paragraph) child |
XXX[@att] |
Selects all the child elements of XXX with an attribute named att |
./@* for example, //script/./@* |
Finds all attribute values of current element |
A predicate is embedded in square brackets and is used to find out specific node(s) or a node that contains a specific value:
//p[@align]: This allows finding all the tags that have align attribute value as center
//img[@alt]: This allows finding all the img (image) tags that contain the alt tag
//table[@border]: This allows finding all the table tags that contain border attributes
//table[@border >1]: This finds the table with border value greater than 1
Retrieve the table row using the complete path:
//body/div/table/tbody/tr[1]
Get the name of the parent of //body/div/table/.. (parent of the table tag)
//body/div/table/..[name()]
Path expression |
Result |
//div/p[1] |
Selects the first paragraph element that is the child of the div element |
//div/p [last()] |
Selects the last paragraph element that is the child of the div element |
//div/p[last()-1] |
Selects the second last paragraph element that is the child of the div element |
//div/p[position()<3] |
Selects the first two paragraph elements that are children of the div element |
//script[@language] |
Selects all script element(s) with an attribute named as language |
//script[@language='javascript'] |
Selects all the script elements that have an attribute named language with a value of JavaScript |
//div/p[text()>45.00] |
Selects all the paragraph elements of the div element that have a text element with a value greater than 45.00 |
Apart from selecting the specific nodes in XPath, XPath allows us to select the group of HTML elements using *, @, and node() functions.
* represents an element node
@ represents the attribute
node() represents any node
The previous mentioned elements allow selecting the unknown nodes; for example:
/div/* selects all the child nodes of a div element
//* selects all the elements in a document
//script[@*] selects all the title elements which contain attributes