










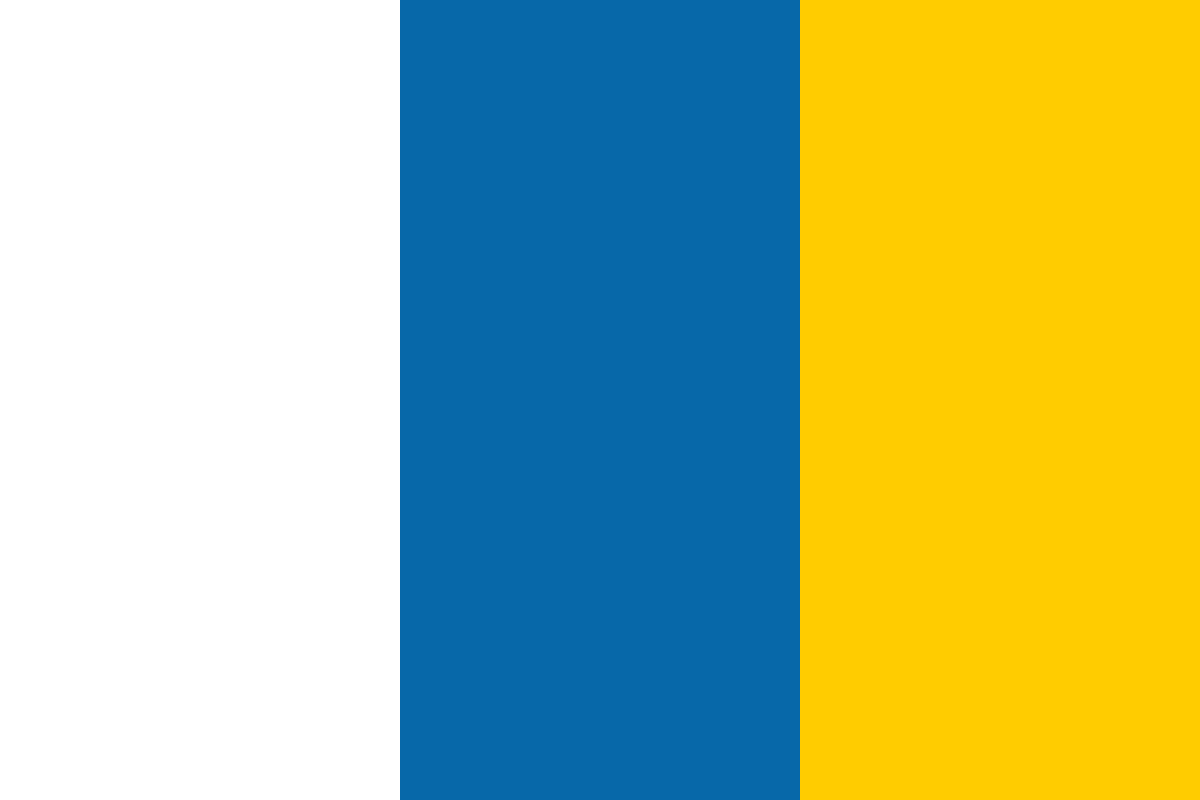

































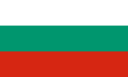








(For more resources related to this topic, see here.)
The DHTMLX grid component is one of the more widely used components of the library. It has a vast amount of settings and abilities that are so robust we could probably write an entire book on them. But since we have an application to build, we will touch on some of the main methods and get into utilizing it.
Some of the cool features that the grid supports is filtering, spanning rows and columns, multiple headers, dynamic scroll loading, paging, inline editing, cookie state, dragging/ordering columns, images, multi-selection, and events.
By the end of this article, we will have a functional grid where we will control the editing, viewing, adding, and removing of users.
When creating a DHTMLX grid, we first create the object; second we add all the settings and then call a method to initialize it. After the grid is initialized data can then be added.
The order of steps to create a grid is as follows:
Now we will go over initializing a grid.
We can initialize a DHTMLX grid in two ways, similar to the other DHTMLX objects. The first way is to attach it to a DOM element and the second way is to attach it to an existing DHTMLX layout cell or layout.
A grid can be constructed by either passing in a JavaScript object with all the settings or built through individual methods.
Let's attach the grid to a DOM element. First we must clear the page and add a div element using JavaScript.
Type and run the following code line in the developer tools console:
document.body.innerHTML = "<div id='myGridCont'></div>";
We just cleared all of the body tags content and replaced it with a div tag having the id attribute value of myGridCont.
Now, create a grid object to the div tag, add some settings and initialize it.
Type and run the following code in the developer tools console:
var myGrid = new dhtmlXGridObject("myGridCont"); myGrid.setImagePath(config.imagePath); myGrid.setHeader(["Column1", "Column2", "Column3"]); myGrid.init();
You should see the page with showing just the grid header with three columns.
Next, we will create a grid on an existing cell object.
Refresh the page and add a grid to the appLayout cell.
Type and run the following code in the developer tools console:
var myGrid = appLayout.cells("a").attachGrid(); myGrid.setImagePath(config.imagePath); myGrid.setHeader(["Column1","Column2","Column3"]); myGrid.init();
You will now see the grid columns just below the toolbar.
Now let's go over some available grid methods. Then we can add rows and call events on this grid.
For these exercises we will be using the global appLayout variable.
Refresh the page.
We will begin by creating a grid to a cell.
The attachGrid method creates and attaches a grid object to a cell. This is the first step in creating a grid.
Type and run the following code line in the console:
var myGrid = appLayout.cells("a").attachGrid();
The setImagePath method allows the grid to know where we have the images placed for referencing in the design. We have the application image path set in the config object.
Type and run the following code line in the console:
myGrid.setImagePath(config.imagePath);
The setHeader method sets the column headers and determines how many headers we will have. The argument is a JavaScript array.
Type and run the following code line in the console:
myGrid.setHeader(["Column1", "Column2", "Column3"]);
The setinitWidths method will set the initial widths of each of the columns. The asterisk mark (*) is used to set the width automatically.
Type and run the following code line in the console:
myGrid.setInitWidths("125,95,*");
The setColAlign method allows us to align the column's content position.
Type and run the following code line in the console:
myGrid.setColAlign("right,center,left");
Up until this point, we haven't seen much going on. It was all happening behind the scenes. To see these changes the grid must be initialized.
Type and run the following code line in the console:
myGrid.init();
Now you see the columns that we provided.
Now that we have a grid created let's add a couple rows and start interacting.
The addRow method adds a row to the grid. The parameters are ID and columns.
Type and run the following code in the console:
myGrid.addRow(1,["test1","test2","test3"]); myGrid.addRow(2,["test1","test2","test3"]);
We just created two rows inside the grid.
The setColTypes method sets what types of data a column will contain.
The available type options are:
Currently, the grid allows for inline editing if you were to double-click on grid cell. We do not want this for the application. So, we will set the column types to read-only.
Type and run the following code in the console:
myGrid.setColTypes("ro,ro,ro");
Now the cells are no longer editable inside the grid.
The getSelectedRowId method returns the ID of the selected row. If there is nothing selected it will return null.
Type and run the following code line in the console:
myGrid.getSelectedRowId();
The clearSelection method clears all selections in the grid.
Type and run the following code line in the console:
myGrid.clearSelection();
Now any previous selections are cleared.
The clearAll method removes all the grid rows. Prior to adding more data to the grid we first must clear it. If not we will have duplicated data.
Type and run the following code line in the console:
myGrid.clearAll();
Now the grid is empty.
The parse method allows the loading of data to a grid in the format of an XML string, CSV string, XML island, XML object, JSON object, and JavaScript array. We will use the parse method with a JSON object while creating a grid for the application.
Here is what the parse method syntax looks like (do not run this in console):
myGrid.parse(data, "json");
The DHTMLX grid component has a vast amount of events. You can view them in their entirety in the documentation. We will cover the onRowDblClicked and onRowSelect events.
The onRowDblClicked event is triggered when a grid row is double-clicked. The handler receives the argument of the row ID that was double-clicked. Type and run the following code in console:
myGrid.attachEvent("onRowDblClicked", function(rowId){ console.log(rowId); });
Double-click one of the rows and the console will log the ID of that row.
The onRowSelect event will trigger upon selection of a row.
Type and run the following code in console:
myGrid.attachEvent("onRowSelect", function(rowId){ console.log(rowId); });
Now, when you select a row the console will log the id of that row. This can be perceived as a single click.
In this article, we learned about the DHTMLX grid component. We also added the user grid to the application and tested it with the storage and callbacks methods.
Further resources on this subject: