










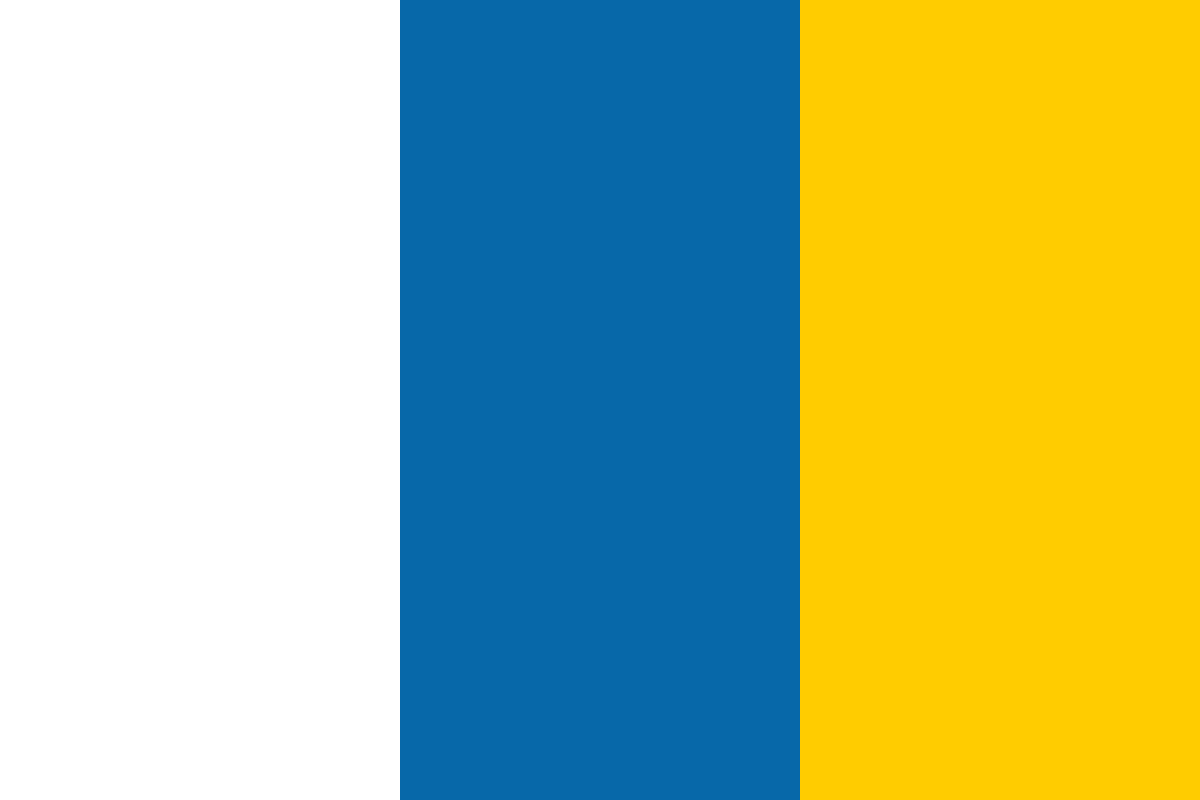

































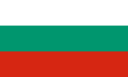








Some of the problems and concerns that this architecture attempts to solve are:
A REST based service should implement four basic constraints: identification of resources, manipulation or resources through, representations, self-descriptive messages, and HATEOAS (hypermedia as the engine of application state).
Basically, any information that can be named can be a resource. A resource could be a representation of a code Class, a file on the file system, a simple block of text, a byte array of an image, or the image itself. Think of a resource as a conceptual representation of entity - a conceptual representation of a person, building, order, so on.
Given the non-virtual resource representing person, a conceptual representation of that resource might be:
<?xml version="1.0" encoding="utf-8"?> <person id="" uri=""> <firstName/> <middleName/> <lastName/> </person>
Given the non-virtual resource "John Adams", a representation of that resource might be:
<?xml version="1.0" encoding="utf-8"?> <person id="123" uri="http://domain/people/123"> <firstName>John</firstName> <middleName></middleName> <lastame>Adams</lastame> </person>
There are a few things that should be noted regarding resources:
http://domain/People/123
, Dynamic: http://domain/People/Search?status=new
Some of the most basic constructs of HTTP 1.1 are some of the Web's most powerful tools. Users of the Web have been shielded from the specifics of protocols such as HTTP 1.1 (as described in RFC2616) via various user-agents such as Firefox and Google Chrome. It was no mistake that REST was being conceptualized at the same time HTTP 1.1 was being formulated. Both became instrumental to the success of Web based applications on the internet. To illustrate, consider the 7 basic URI routes for a Ruby on Rails application using the person representation from above:
HTTP Method | URI | Action |
GET | people | Index |
GET | people/123 | Show |
POST | people | Create |
PUT | people/123 | Update |
DELETE | people/123 | Delete |
GET | people/123/new | New |
GET | people/123/edit | Edit |
A patternized approach to URI schemes makes implementation and consumption of a REST service drastically more simplistic. The URI schemes above are examples of what the manipulation of resources through the use of HTTP verbs might look like. The point is that a RESTful service should provide the client with enough information to manipulate the resource.
Given the following: GET people/123
HTTP/1.1 200 OK
Cache-Control: private
Content-Type: application/xml; charset=utf-8
Content-Location: http://domain/people/123
Date: Fri, 01 May 2009 05:36:38 GMT
Content-Length: 102
<?xml version="1.0" encoding="utf-8"?>
<person id="123" uri="http://domain/people/123">
<firstName>John</firstName>
<middleName></middleName>
<lastame>Adams</lastame>
</person>
The consumer of the REST service now has all they need to know to update the "middleName" node with the value of "Quincy":
PUT people/123
HTTP/1.1 200 OK Content-Type: application/xml; charset=utf-8 Date: Fri, 01 May 2009 05:36:38 GMT Content-Length: 102
<?xml version="1.0" encoding="utf-8"?>
<person id="123" uri="http://domain/people/123">
<firstName>John</firstName>
<middleName>Quincy</middleName>
<lastame>Adams</lastame>
</person>
Server responds:
HTTP/1.1 200 OK
Content-Type: application/xml; charset=utf-8
Content-Location: http://domain/people/123
Date: Fri, 01 May 2009 05:36:40 GMT
Content-Length: 102
<?xml version="1.0" encoding="utf-8"?>
<person id="123" uri="http://domain/people/123">
<firstName>John</firstName>
<middleName>Quincy</middleName>
<lastame>Adams</lastame>
</person>
There are a few things to note regarding manipulation of resources via representations:
This rule of REST implies that the server must provide enough information in each message, to the consumer, so that they can properly process the message. The message must somehow be self-descriptive so that the consumer can use it without having to look into the body of the message. Headers are probably the best example of how to inform the user without inspection. Using HOST headers, content-type, content-location, and even resource extensions the server implementation quickly enables successful consumption. Caching, chunking are also some of the other enabling mechanisms of HTTP 1.1.
There are a few things to note regarding self-descriptive messages: