










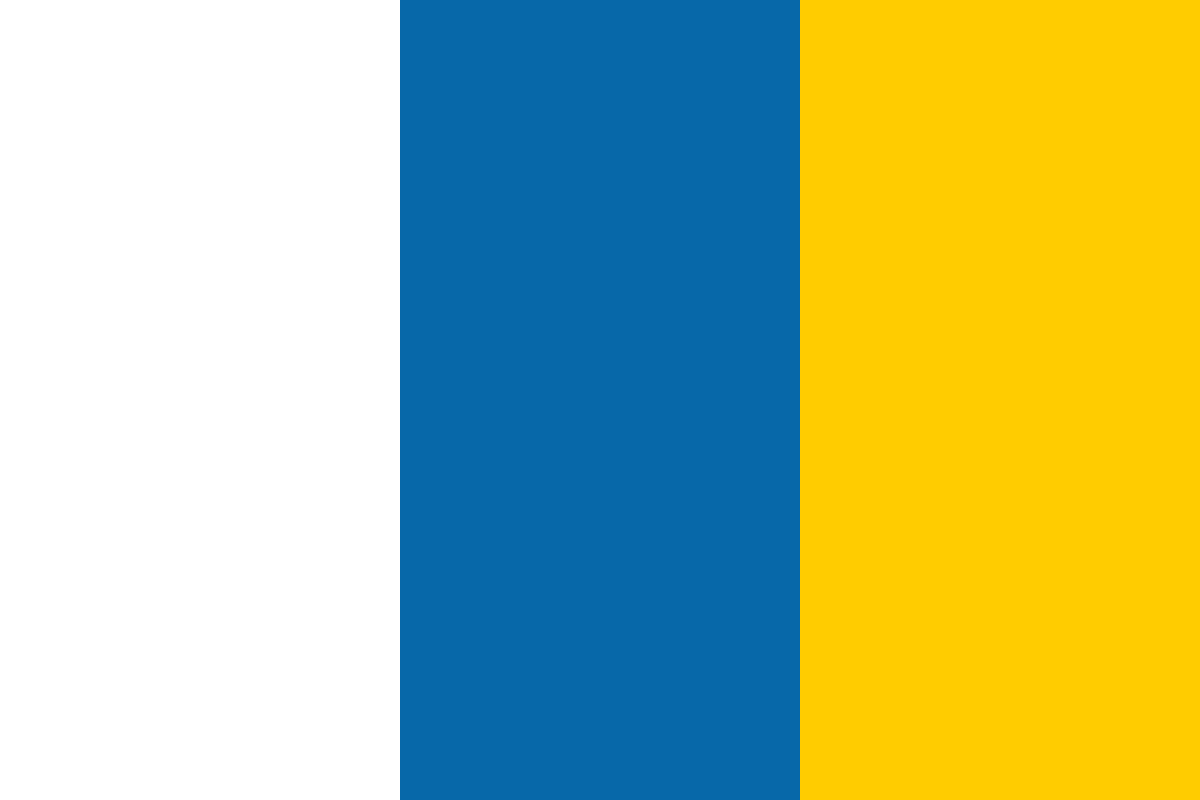

































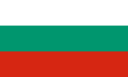








And you will do all of these by developing a Post Types plugin that provide pre-defined post templates to add a photo or a link quickly to your blog.
The concepts you will learn in this article will help you discover the not so obvious capabilities of the WordPress platform that allows you to transform it into software—capable of handling much more than just a blog.
Localization is an important part of WordPress development as not everyone using WordPress speaks English (WordPress comes in different languages too).
Localization involves just a small amount of the extra work on your side, since the translation itself is usually done by volunteers (people who like and use your plugin).
You only need to provide some base files for translation, and don't be surprised when you start getting translated files sent to your inbox.
WordPress uses the GNU gettext localization framework, which is a standardized method of managing translations, and we will make use of it in our plugin.
We will start by defining our plugin as usual, and then add localization support.
<?php
// pluginname Post Types
// shortname PostTypes
// dashname post-types
/*
Plugin Name: Post Types
Version: 0.1
Plugin URI:
http://www.prelovac.com/vladimir/wordpress-plugins/post-types
Author: Vladimir Prelovac
Author URI:http://www.prelovac.com/vladimir
Description: Provides pre-defined post templates to quickly add a
photo or a link to your blog
*/
// Avoid name collisions.
if ( !class_exists('PostTypes') ) :
class PostTypes
{
// localization domain
var $plugin_domain='PostTypes';
// Initialize the plugin
function PostTypes()
{
global $wp_version;
$exit_msg='Post Types requires WordPress 2.5 or newer.
<a href="http://codex.wordpress.org/Upgrading_WordPress">
Please update!</a>';
if (version_compare($wp_version,"2.5","<"))
{
exit ($exit_msg);
}
}
// Set up default values
function install()
{
}
}
endif;
if ( class_exists('PostTypes') ) :
$PostTypes = new PostTypes();
if (isset($PostTypes))
{
register_activation_hook( __FILE__, array(&$PostTypes,
'install') );
}
endif;
// Localization support
function handle_load_domain()
{
// get current language
$locale = get_locale();
// locate translation file
$mofile = WP_PLUGIN_DIR.'/'.plugin_basename(dirname
(__FILE__)).'/lang/' . $this->plugin_domain . '-' .
$locale . '.mo';
// load translation
load_textdomain($this->plugin_domain, $mofile);
}
// Initialize the plugin
function PostTypes()
{
global $wp_version, $pagenow;
// pages where our plugin needs translation
$local_pages=array('plugins.php');
if (in_array($pagenow, $local_pages))
$this->handle_load_domain();
$exit_msg='Post Types requires WordPress 2.5 or newer.
<a href="http://codex.wordpress.org/Upgrading_WordPress">
Please update!</a>';
$this->handle_load_domain();
$exit_msg=__('Post Types requires WordPress 2.5 or newer.
<a href="http://codex.wordpress.org/Upgrading_WordPress">
Please update!</a>', $this->plugin_domain);
if (version_compare($wp_version,"2.5","<"))
We have added localization support to our plugin by using the provided localization functions provided by WordPress.
Currently, we have only localized the error message for WordPress version checking:
$exit_msg=__('Post Types requires WordPress 2.5 or newer.
<a href="http://codex.wordpress.org/Upgrading_WordPress">
Please update!</a>', $this->plugin_domain);
We have done that by enclosing the text in the __() function, which takes the text as localized, and enclosing our unique localization domain or context within the WordPress localization files.
To load localization, we created a handle_load_domain function.
The way it works is to first get the current language in use by using the get_locale() function:
// Localization support
function handle_load_domain()
{
// get current language
$locale = get_locale();
Then it creates the language file name by adding together the plugin dir, plugin folder, and the lang folder where we will keep the translations. The file name is derived from the locale, and the *.mo language file extension:
// locate translation file
$mofile = WP_PLUGIN_DIR.'/'.plugin_basename
(dirname(__FILE__)).'/lang/' . $this->plugin_domain .
'-' . $locale . '.mo';
Finally, the localization file is loaded using the load_textdomain() function, taking our text domain and .mo file as parameters.
// load translation
load_textdomain($this->plugin_domain, $mofile);
The translation file needs to be loaded as the first thing in the plugin—before you output any messages. So we have placed it as the first thing in the plugin constructor.
Since loading the translation file occurs at the beginning of the constructor, which is executed every time, it is a good idea to select only the pages where the translation will be needed in order to preserve resources.
WordPress provides the global variable, $pagenow, which holds the name of the current page in use.
We can check this variable to find out if we are on a page of interest. In the case of plugin activation error message, we want to check if we are on the plugins page defined as plugins.php in WordPress:
// pages where our plugin needs translation
$local_pages=array('plugins.php');
if (in_array($pagenow, $local_pages))
$this->handle_load_domain();
You can optimize this further by querying the page parameter, if it exists, as this will—in most cases—point precisely to the usage of your page (plugins.php?page=photo):
if ($_GET['page']=='photo')
Optimizing the usage of the translation file is not required; it's just a matter of generally loading only what you need in order to speed up the whole system.
For localization to work, you need to provide .po and .mo files with your plugins. These files are created by using external tools such as PoEdit.
These tools output the compiled translation file, which can be then loaded by using the load_textdomain() function. This function accepts a language domain name and a path to the file.
In order to use translated messages, you can use the __($text, $domain) and _e($text, $domain) functions. The _e() function is just an equivalent of
echo __();
These functions accept two parameters, the first being the desired text, and the second, the language domain where the message will be looked for.
If no translation was found, the text is just printed out as it is. This means that you can always safely use these functions, even if you do not provide any translation files. This will prepare the plugin for future translation.
Quick reference
$pagenow: A global variable holding the name of the currently displayed page within WordPress.
get_locale(): A function which gets the currently selected language.
load_textdomain(domain, filepath): This function loads the localization file and adds it to the specified language domain identifier.
_(); _e(): These functions are used to find the output text using a given language domain.
More information about WordPress localization is available at: http://codex.wordpress.org/Translating_WordPress.