










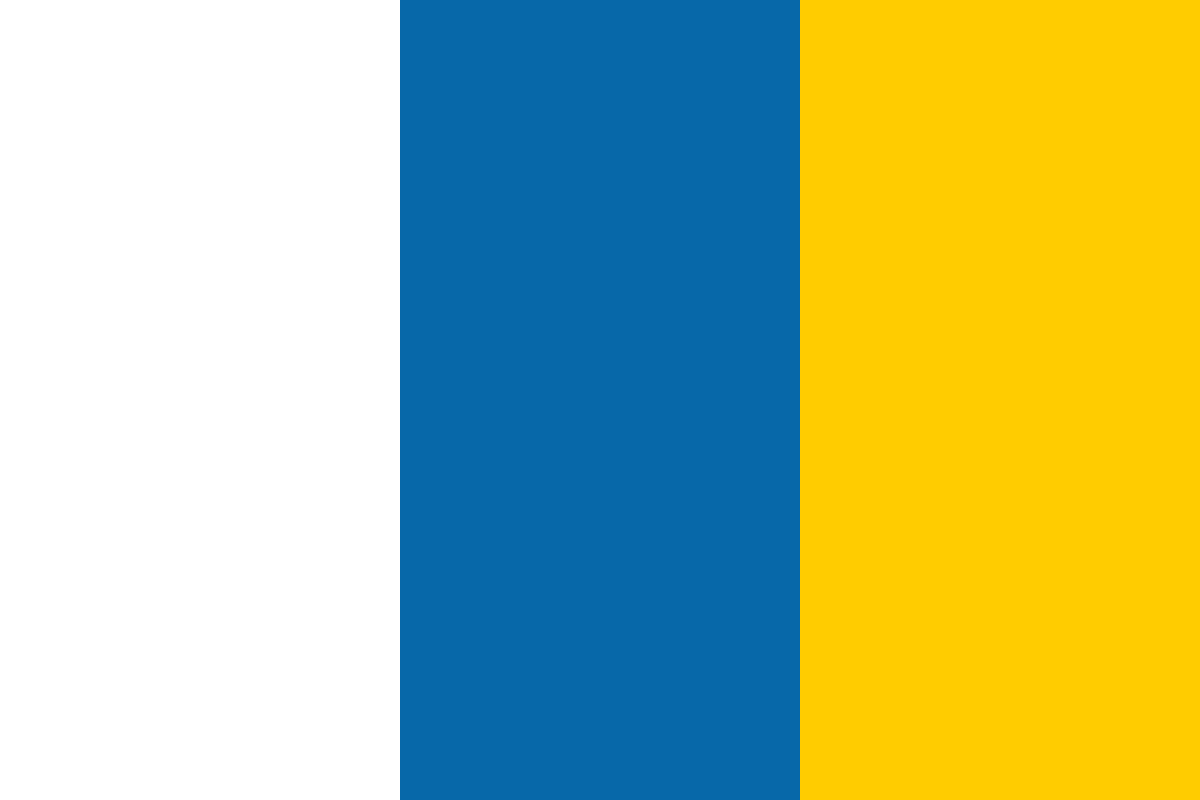

































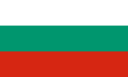








Hibernate provides a bridge between the database and the application by persisting application objects in the database, rather than requiring the developer to write and maintain lots of code to store and retrieve objects.
The main configuration file, hibernate.cfg.xml, specifies how Hibernate obtains database connections, either from a JNDI DataSource or from a JDBC connection pool. Additionally, the configuration file defines the persistent classes, which are backed by mapping definition files.
This is a sample hibernate.cfg.xml configuration file that is used to handle connections to a MySQL database, mapping the com.sample.MySample class.
<hibernate-configuration>
<session-factory>
<property name="connection.username">user</property>
<property name="connection.password">password</property>
<property name="connection.url">
jdbc:mysql://localhost/database
</property>
<property name="connection.driver_class">
com.mysql.jdbc.Driver
</property>
<property name="dialect">
org.hibernate.dialect.MySQLDialect
</property>
<mapping resource="com/sample/MyClass.hbm.xml"/>
</session-factory>
</hibernate-configuration>
From our point of view, it is important to know that Hibernate applications can coexist in both the managed environment and the non-managed environment. An application server is a typical example of a managed environment that provides services to hosting applications, such as connection pooling and transaction.
On the other hand, a non-managed application refers to standalone applications, such as Swing Java clients that typically lack any built-in service.
In this article, we will focus on managed environment applications, installed on JBoss Application Server. You will not need to download any library to your JBoss installation. As a matter of fact, JBoss persistence layer is designed around Hibernate API, so it already contains all the core libraries.
You can choose different strategies for building a Hibernate application. For example, you could start building Java classes and map files from scratch, and then let Hibernate generate the database schema accordingly. You can also start from a database schema and reverse engineer it into Java classes and Hibernate mapping files. We will choose the latter option, which is also the fastest. Here's an overview of our application.
In this example, we will design an employee agenda divided into departments. The persistence model will be developed with Hibernate, using the reverse engineering facet of JBoss tools. We will then need an interface for recording our employees and departments, and to query them as well.
The web interface will be developed using a simple Model-View-Controller (MVC) pattern and basic JSP 2.0 and servlet features.
The overall architecture of this system resembles the AppStore application that has been used to introduce JPA. As a matter of fact, this example can be used to compare the two persistence models and to decide which option best suits your project needs. We have added a short section at the end of this example to stress a few important points about this choice.
The overall architecture of this system resembles the AppStore application that has been used to introduce JPA. As a matter of fact, this example can be used to compare the two persistence models and to decide which option best suits your project needs. We have added a short section at the end of this example to stress a few important points about this choice.
CREATE schema hibernate;
GRANT ALL PRIVILEGES ON hibernate.* TO 'jboss'@'localhost' WITH GRANT
OPTION;
CREATE TABLE `hibernate`.`department` (
`department_id` INTEGER UNSIGNED NOT NULL AUTO_INCREMENT,
`department_name` VARCHAR(45) NOT NULL,
PRIMARY KEY (`department_id`)
)
ENGINE = InnoDB;
CREATE TABLE `hibernate`.`employee` (
`employee_id` INTEGER UNSIGNED NOT NULL AUTO_INCREMENT,
`employee_name` VARCHAR(45) NOT NULL,
`employee_salary` INTEGER UNSIGNED NOT NULL,
`employee_department_id` INTEGER UNSIGNED NOT NULL,
PRIMARY KEY (`employee_id`),
CONSTRAINT `FK_employee_1` FOREIGN KEY `FK_employee_1` (`employee_
department_id`)
REFERENCES `department` (`department_id`)
ON DELETE CASCADE
ON UPDATE CASCADE
)
ENGINE = InnoDB;
With the first Data Definition Language (DDL) command, we have created a schema named Hibernate that will be used to store our tables. Then, we have assigned the necessary privileges on the Hibernate schema to the user jboss.
Finally, we created a table named department that contains the list of company units, and another table named employee that contains the list of workers. The employee table references the department with a foreign key constraint.