










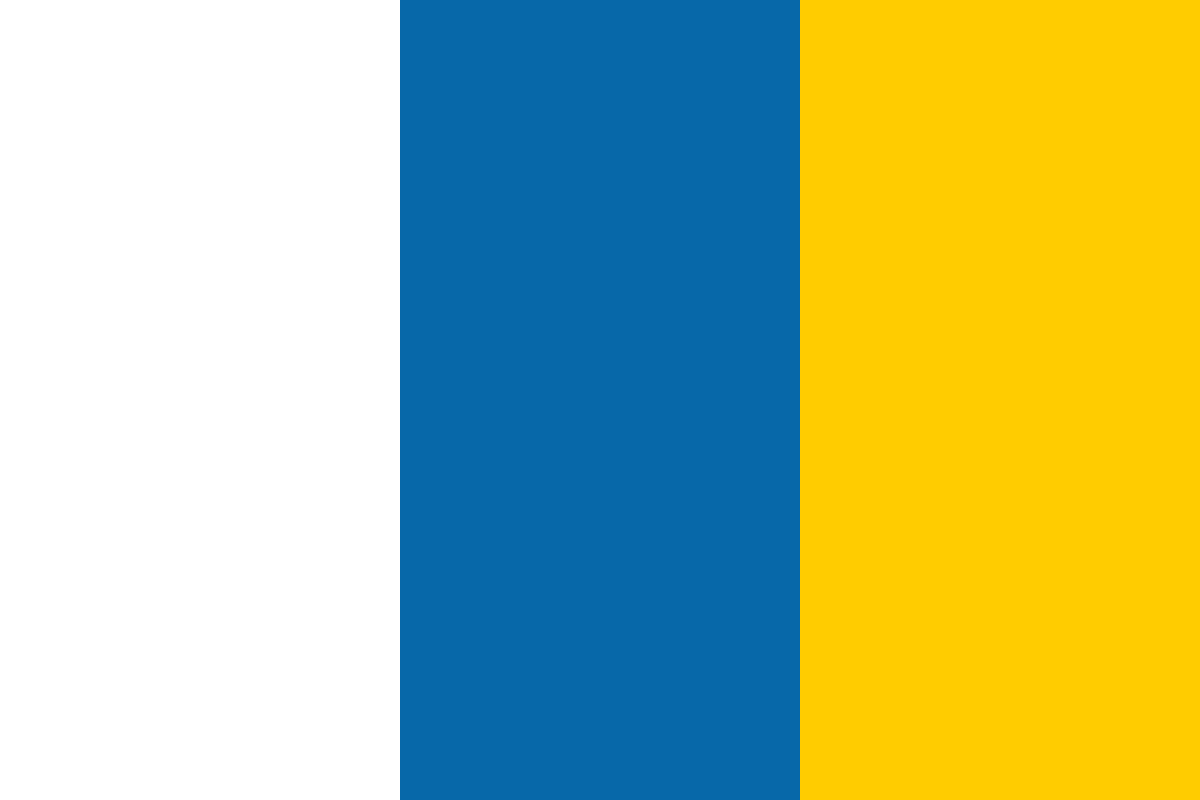

































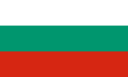








NHibernate is an open source object-relational mapper, or simply put, a way to rapidly retrieve data from your database into standard .NET objects. This article teaches you how to create NHibernate sessions, which use database sessions to retrieve and store data into the database.
In this article by Aaron B. Cure, author of Nhibernate 2 Beginner's Guide we'll talk about:
(Read more interesting articles on Nhibernate 2 Beginner's Guide here.)
Think of an NHibernate session as an abstract or virtual conduit to the database. Gone are the days when you have to create a Connection, open the Connection, pass the Connection to a Command object, create a DataReader from the Command object, and so on.
With NHibernate, we ask the SessionFactory for a Session object, and that's it. NHibernate handles all of the "real" sessions to the database, connections, pooling, and so on. We reap all the benefits without having to know the underlying intricacies of all of the database backends we are trying to connect to.
Before we actually connect to the database, we need to do a little "housekeeping". Just a note, if you run into trouble (that is, your code doesn't work like the walkthrough), then don't panic. See the troubleshooting section at the end of this Time for action section.
using System;
using System.Collections.Generic;
using System.Text;
namespace Ordering.Console
{
class Program
{
static void Main(string[] args)
{
}
}
}
Or, if you are using VB.NET, your Module1.vb file will look as follows:
Module Module1
Sub Main()
End Sub
End Module
using NHibernate;
using NHibernate.Cfg;
using Ordering.Data;
In VB.NET you need to use the Imports keyword as follows:
Imports NHibernate
Imports NHibernate.Cfg
Imports Ordering.Data
Configuration cfg = new Configuration();
cfg.Properties.Add(NHibernate.Cfg.Environment.ConnectionProvider,
typeof(NHibernate.Connection.DriverConnectionProvider)
.AssemblyQualifiedName);
cfg.Properties.Add(NHibernate.Cfg.Environment.Dialect,
typeof(NHibernate.Dialect.MsSql2008Dialect)
.AssemblyQualifiedName);
cfg.Properties.Add(NHibernate.Cfg.Environment.ConnectionDriver,
typeof(NHibernate.Driver.SqlClientDriver)
.AssemblyQualifiedName);
cfg.Properties.Add(NHibernate.Cfg.Environment.ConnectionString,
"Server= (local)SQLExpress;Database=
Ordering;Trusted_Connection=true;");
cfg.Properties.Add(NHibernate.Cfg.Environment.
ProxyFactoryFactoryClass, typeof
(NHibernate.ByteCode.LinFu.ProxyFactoryFactory)
.AssemblyQualifiedName);
cfg.AddAssembly(typeof(Address).AssemblyQualifiedName);
For a VB.NET project, add the following code:
Dim cfg As New Configuration()
cfg.Properties.Add(NHibernate.Cfg.Environment. _
ConnectionProvider, GetType(NHibernate.Connection. _
DriverConnectionProvider).AssemblyQualifiedName)
cfg.Properties.Add(NHibernate.Cfg.Environment.Dialect, _
GetType(NHibernate.Dialect.MsSql2008Dialect). _
AssemblyQualifiedName)
cfg.Properties.Add(NHibernate.Cfg.Environment.ConnectionDriver, _
GetType(NHibernate.Driver.SqlClientDriver). _
AssemblyQualifiedName)
cfg.Properties.Add(NHibernate.Cfg.Environment.ConnectionString, _
"Server= (local)SQLExpress;Database=Ordering; _
Trusted_Connection=true;")
cfg.Properties.Add(NHibernate.Cfg.Environment. _
ProxyFactoryFactoryClass, GetType _
(NHibernate.ByteCode.LinFu.ProxyFactoryFactory). _
AssemblyQualifiedName)
cfg.AddAssembly(GetType(Address).AssemblyQualifiedName)
The most common error that will show up is MappingException was unhandled. If you get a mapping exception, then see the next step for troubleshooting tips.
The second InnerException says "class Ordering.Data.Address, Ordering.Data, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null not found while looking for property: Id".
Now we are getting closer. It has something to do with the ID property. But wait, there is another InnerException. This InnerException says "Could not find a getter for property 'Id' in class 'Ordering.Data.Address'". How could that be? Looking at my Address.cs class, I see:
using System;
using System.Collections.Generic;
using System.Text;
namespace Ordering.Data
{
public class Address
{
}
}
Oops! Apparently I stubbed out the class, but forgot to add the actual properties. I need to put the rest of the properties into the file, which looks as follows:
using System;
using System.Collections.Generic;
using System.Text;
namespace Ordering.Data
{
public class Address
{
#region Constructors
public Address() { }
public Address(string Address1, string Address2, string
City, string State, string Zip)
: this()
{
this.Address1 = Address1;
this.Address2 = Address2;
this.City = City;
this.State = State;
this.Zip = Zip;
}
#endregion
#region Properties
private int _id;
public virtual int Id
{
get { return _id; }
set { _id = value; }
}
private string _address1;
public virtual string Address1
{
get { return _address1; }
set { _address1 = value; }
}
private string _address2;
public virtual string Address2
{
get { return _address2; }
set { _address2 = value; }
}
private string _city;
public virtual string City
{
get { return _city; }
set { _city = value; }
}
private string _state;
public virtual string State
{
get { return _state; }
set { _state = value; }
}
private string _zip;
public virtual string Zip
{
get { return _zip; }
set { _zip = value; }
}
private Contact _contact;
public virtual Contact Contact
{
get { return _contact; }
set { _contact = value; }
}
#endregion
}
}
We have successfully created a project to test out our database connectivity, and an NHibernate Configuration object which will allow us to create sessions, session factories, and a whole litany of NHibernate goodness!