










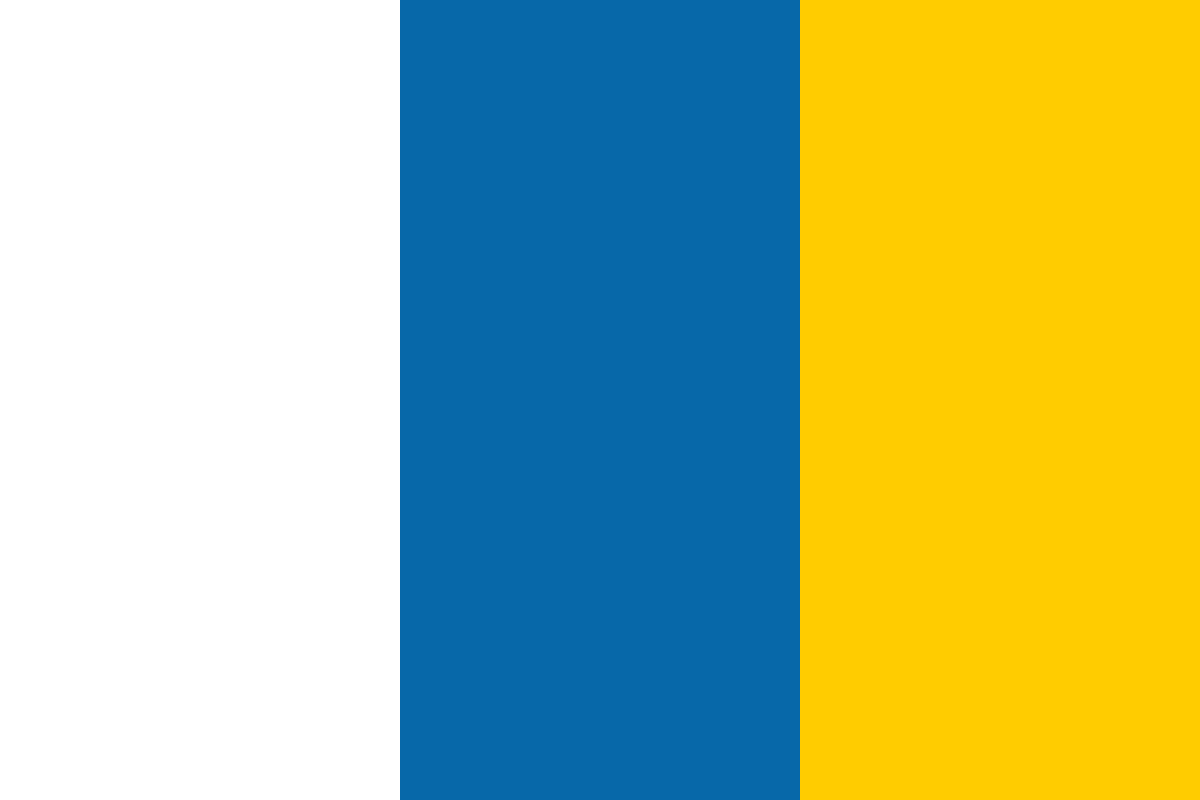

































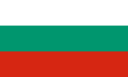








Dialogs: at least under the jQuery Mobile framework: are small windows that cover an existing page. They typically provide a short message or question for the user. They will also typically include a button that allows the user to dismiss the dialog and return back to the site. Creating a dialog in jQuery Mobile is done by simply adding a simple attribute to a link: data-rel="dialog". The following listing demonstrates an example:
Listing 7-1: test1.html <!DOCTYPE html> <html> <head> <title>Dialog Test</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="http://code.jquery.com/mobile/ latest/jquery.mobile.min.css" /> <script src="http://code.jquery.com/jquery-1.7.1.min.js"></script> <script src="http://code.jquery.com/mobile/latest/ jquery.mobile.min.js"></script> </head> <body> <div data-role="page" id="first"> <div data-role="header"> <h1>Dialog Test</h1> </div> <div data-role="content"> <p> <a href="#page2">Another Page (normal)</a> </p> <p> <a href="#page3" data-rel="dialog">A Dialog (dialog)</a> </p> </div> </div> <div data-role="page" id="page2"> <div data-role="header"> <h1>The Second</h1> </div> <div data-role="content"> <p> This is the Second </p> </div> </div> <div data-role="page" id="page3"> <div data-role="header"> <h1>The Third</h1> </div> <div data-role="content"> <p> This is the Third </p> </div> </div> </body> </html>
This is a simple, multi-page jQuery Mobile site. Notice how we link to the second and third page. The first link is typical. The second link, though, includes the data-rel attribute mentioned earlier. Notice that both the second and third page are defined in the usual manner. So the only change we have here is in the link. When that second link is clicked, the page is rendered completely differently:
Remember, that page wasn't defined differently. The change you see in the previous screenshot is driven by the change to the link itself. That's it! Clicking the little X button will hide the dialog and return the user back to the original page.
Any link within the page will handle closing the dialog as well. If you wish to add a cancel type button, or link, you can do so using data-rel="back" in the link. The target of the link should be to the page that launched the dialog. Listing 7-2 shows a modified version of the earlier template. In this one, we've simply added two buttons to the dialog. The first button will launch the second page, while the second one will act as a Cancel action.
Listing 7-2: test2.html <!DOCTYPE html> <html> <head> <title>Dialog Test (2)</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="http://code.jquery.com/mobile/ latest/jquery.mobile.min.css" /> <script src="http://code.jquery.com/jquery-1.7.1.min.js"></script> <script src="http://code.jquery.com/mobile/ latest/jquery.mobile.min.js"></script> </head> <body> <div data-role="page" id="first"> <div data-role="header"> <h1>Dialog Test</h1> </div> <div data-role="content"> <p> <a href="#page2">Another Page (normal)</a> </p> <p> <a href="#page3" data-rel="dialog">A Dialog (dialog)</a> </p> </div> </div> <div data-role="page" id="page2"> <div data-role="header"> <h1>The Second</h1> </div> <div data-role="content"> <p> This is the Second </p> </div> </div> <div data-role="page" id="page3"> <div data-role="header"> <h1>The Third</h1> </div> <div data-role="content"> <p> This is the Third </p> <a href="#page2" data-role="button">Page 2</a> <a href="#first" data-role="button" data-rel="back">Cancel</a> </div> </div> </body> </html>
The major change in this template is the addition of the buttons in the dialog, contained within page3 div. Notice the first link is turned into a button, but outside of that is a simple link. The second button includes the addition of the data-rel="back" attribute. This will handle simply dismissing the dialog. The following screenshot shows how the dialog looks with the buttons added:
Grids are one of the few features of jQuery Mobile that do not make use of particular data attributes. Instead, you work with grids simply by specifying CSS classes for your content.
Grids come in four flavors: Two column, Three column, Four column, and Five column. (You will probably not want to use the five column on a phone device. Save that for a tablet instead.)
You begin a grid with a divblockthat makes use of the class ui-grid-X, where X will be either a, b, c, or d. ui-grid-arepresents a two column grid. ui-grid-bis a three column grid. You can probably guess what cand dcreate.
So to begin a two column grid, you would wrap your content with the following:
<div class="ui-grid-a"> Content </div>
Within the divtag, you then use a divfor each "cell" of the content. The class for grid calls begins with ui-block-X, where Xgoes from a to d. ui-block-a would be used for the first cell, ui-block-b for the next, and so on. This works much like HTML tables.
Putting it together, the following code snippet demonstrates a simple two column grid with two cells of content:
<div class="ui-grid-a"> <div class="ui-block-a">Left</div> <div class="ui-block-b">Right</div> </div>
Text within a cell will automatically wrap. Listing 7-3 demonstrates a simple grid with a large amount of text in one of the columns:
Listing 7-3: test3.html <!DOCTYPE html> <html> <head> <title>Grid Test</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="http://code.jquery.com/mobile/ latest/jquery.mobile.min.css" /> <script src="http://code.jquery.com/jquery-1.7.1.min.js"></script> <script src="http://code.jquery.com/mobile/ latest/jquery.mobile.min.js"></script> </head> <body> <div data-role="page" id="first"> <div data-role="header"> <h1>Grid Test</h1> </div> <div data-role="content"> <div class="ui-grid-a"> <div class="ui-block-a"> <p> This is my left hand content. There won't be a lot of it. </p> </div> <div class="ui-block-b"> <p> This is my right hand content. I'm going to fill it with some dummy text. </p> <p> Bacon ipsum dolor sit amet andouille capicola spare ribs, short loin venison sausage prosciutto turducken turkey flank frankfurter pork belly short ribs. Venison frankfurter filet mignon, jowl meatball hamburger pastrami pork chop drumstick. Fatback pancetta boudin, ribeye shoulder capicola cow leberkäse bresaola spare ribs prosciutto venison ball tip jowl andouille. Beef ribs t-bone swine, tail capicola turkey pork belly leberkäse frankfurter jowl. Shankle ball tip sirloin frankfurter bacon beef ribs. Tenderloin beef ribs pork chop, pancetta turkey bacon short ribs ham flank chuck pork belly. Tongue strip steak short ribs tail swine. </p> </div> </div> </div> </div> </body> </html>
In the mobile browser, you can clearly see the two columns:
Working with other types of grids then is simply a matter of switching to the other classes. For example, a four column grid would be set up similar to the following code snippet:
<div class="ui-grid-c"> <div class="ui-block-a">1st cell</div> <div class="ui-block-b">2nd cell</div> <div class="ui-block-c">3rd cell</div> </div>