










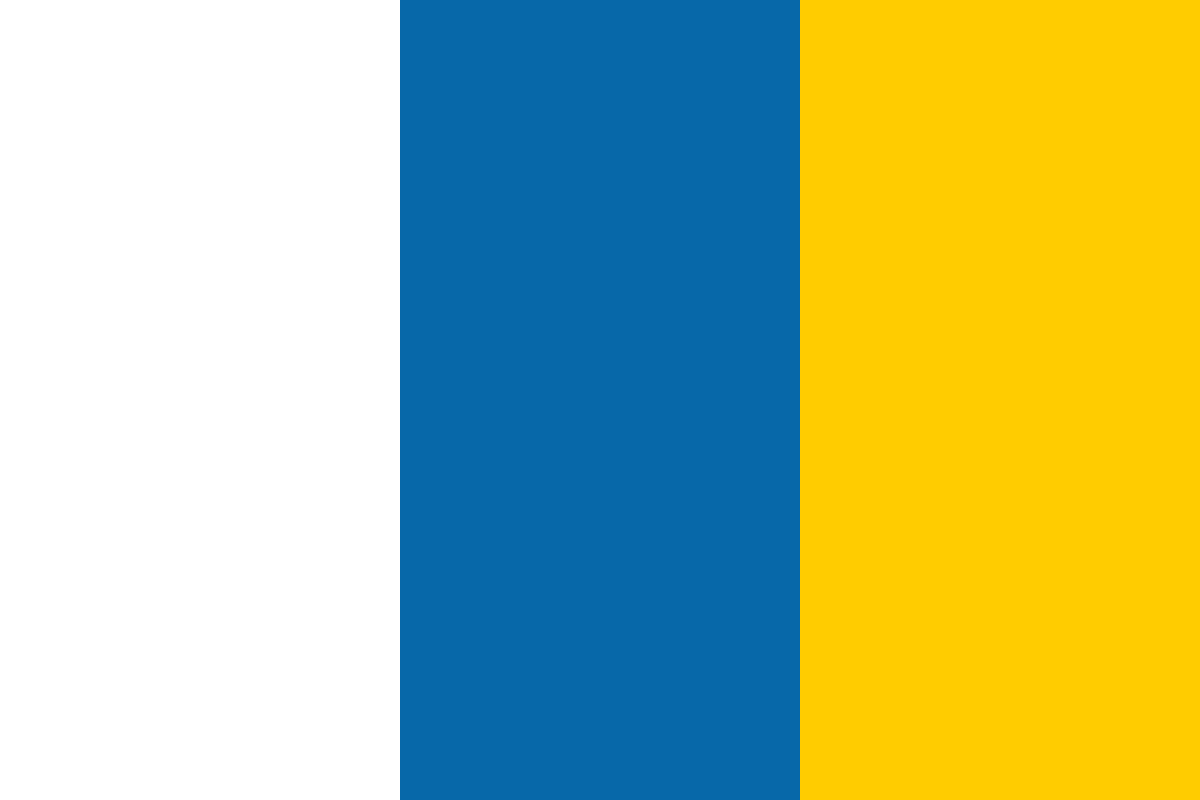

































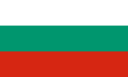








If you are not familiar with the great MelonJS game framework, please go to their official page and read about the great things you can do with this awesome tool.
In this post I will teach you how to create a simple plugin to use in your MelonJS game.
First, you need to understand the plugin structure:
(function($) {
myPlugin = me.plugin.Base.extend({
// minimum melonJS version expected
version : "1.0.0",
init : function() {
// call the parent constructor
this.parent();
this.myVar = null;
},
});
})(window);
As you can see, there are no real difficulties in creating new plugins. You just have to create a me class inheriting from the me.plugin.Base class, passing the minimum melonJS version this plugin will use. If you need to persist some variables, you can override the init method just like the code from the start.
For this plugin I will create a Clay.io leaderboard integration for a game. The code for the plugin is as follows:
/*
* MelonJS Game Engine
* Copyright (C) 2011 - 2013, Olivier Biot, Jason Oster
* http://www.melonjs.org
*
* Clay.io API plugin
*
*/
(function($) {
/**
* @class
* @public
* @extends me.plugin.Base
* @memberOf me
* @constructor
*/
Clayio = me.plugin.Base.extend({
// minimum melonJS version expected
version : "1.0.0",
gameKey: null,
_leaderboard: null,
init : function(gameKey, options) {
// call the parent constructor
this.parent();
this.gameKey = gameKey;
Clay = {};
Clay.gameKey = this.gameKey;
Clay.readyFunctions = [];
Clay.ready = function( fn ) {
Clay.readyFunctions.push( fn );
};
if (options === undefined) {
options = {
debug: false,
hideUI: false
}
}
Clay.options = {
debug: options.debug === undefined ? false: options.debug,
hideUI: options.hideUI === undefined ? false: options.hideUI,
fail: options.fail
}
window.onload = function() {
var clay = document.createElement("script");
clay.async = true;
clay.src = ( "https:" == document.location.protocol ? "https://" : "http://" ) + "cdn.clay.io/api.js";
var tag = document.getElementsByTagName("script")[0];
tag.parentNode.insertBefore(clay, tag);
}
},
leaderboard: function(id, score, callback) {
if (!id) {
throw "You must pass a leaderboard id";
}
// we can get the score directly from game.data.score
if (!score){
score = game.data.score;
}
var leaderboard = new Clay.Leaderboard({id: id});
this._leaderboard = leaderboard;
if (!callback) {
this._leaderboard.post({score: score}, callback);
}else{
this._leaderboard.post({score: score});
}
},
showLeaderBoard: function(id, options, callback) {
if (!options){
options = {};
}
if (options.limit === undefined){
options.limit = 10;
}
if (!this._leaderboard) {
if (id === undefined) {
throw "The leaderboard was not defined before. You must pass a leaderboard id";
}
var leaderboard = new Clay.Leaderboard({id: id});
this._leaderboard = leaderboard;
}
this._leaderboard.show(options, callback);
}
});
})(window);
Let me explain how all of this works:
To use this plugin in your game, first register the plugin in your game.js file. On the game.onload method add the following code:
window.onload(function() {
me.plugin.register.defer(this, Clayio, "clay");
});
Due to a Clay.io bug you need to add the socket.io.js script into the index.html file manually. Place the following code into the file <head>:
<script src='http://api.clay.io/socket.io/socket.io.js'></script>
Now, if you want to call the leaderboard method, just add the following code into your scene:
me.plugin.clay.leaderboard(leaderboardId);
And that's it! I hope I’ve shown you how easy it is to create plugins for MelonJS.
Ellison Leão (@ellisonleao) is a passionate software engineer with more than 6 years of experience in web projects and a contributor to the MelonJS framework and other open source projects. When he is not writing games, he loves to play drums.