










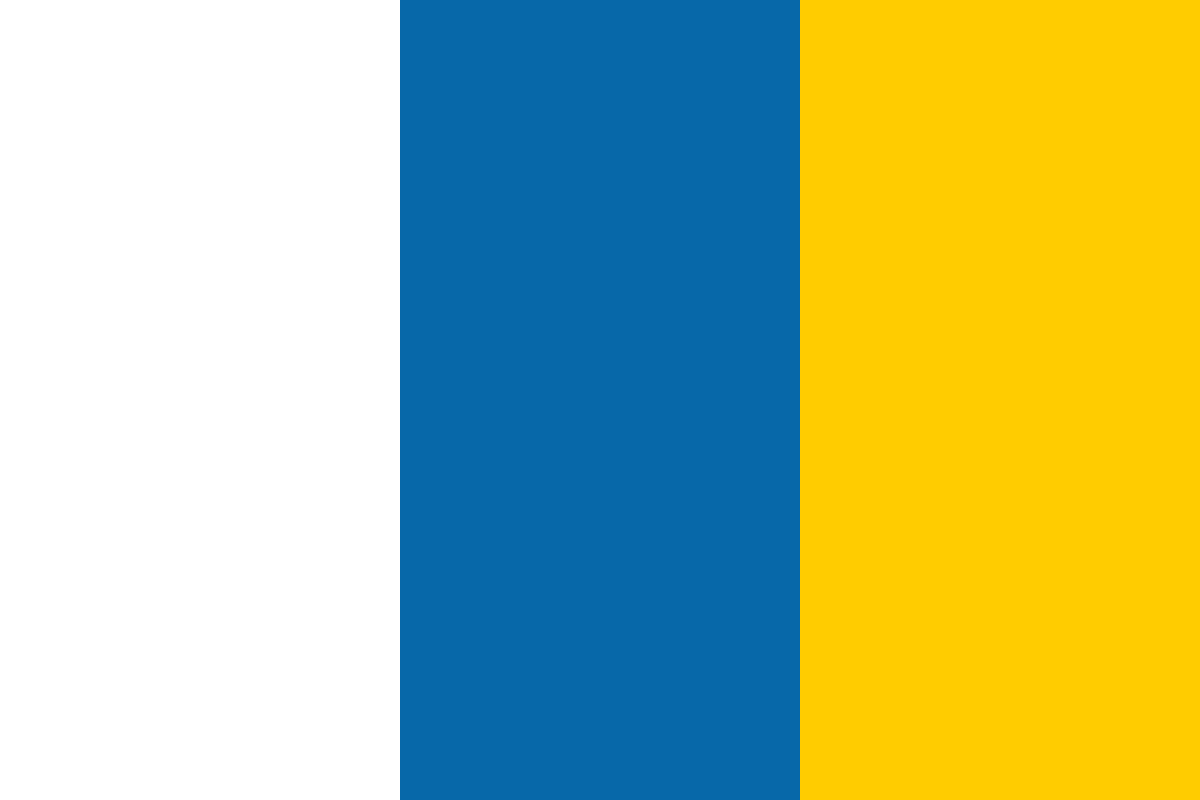

































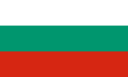








(For more resources on Liferay, see here.)
The portal has defined user management with a set of entities, such as, User, Contact, Address, EmailAddress, Phone, Website, and Ticket, and so on at /portal/service.xml. In the following section, we're going to address the User entity, its association, and relationship.
The following figure depicts these entities and their relationships. The entity User has a one-to-one association with the entity Contact, which may have many contacts as children. And the entity Contact has a one-to-one association with the entity Account, which may have many accounts as children. The entity Contact can have a many-to-many association with the entities Address, EmailAddress, Phone, Website, and Ticket. Logically, the entities Address, EmailAddress, Phone, Website, and Ticket may have a many-to-many association with the other entities, such as Group, Organization, and UserGroup as shown in the following image:
The following table shows user-related service interfaces, extensions, utilities, wrappers, and their main methods:
Interface | Extension | Utility/Wrapper | Main methods |
UserService, UserLocalService | PersistedModelLocalService | User(Local)ServiceUtil, User(Local)ServiceWrapper | add*, authenticate*, check*, decrypt*, delete*, get*, has*, search, unset*, update*, and so on. |
ContactService, ContactLocalService | persistedmodellocalservice> | Contact(Local)ServiceUtil, Contact(Local)ServiceWrapper | add*, create*, delete*, get*, update*, dynamicQuery, and so on. |
AccountService, AccountLocalService | Account(Local)ServiceUtil, Account(Local)ServiceWrapper | add*, create*, delete*, get*, update*, dynamicQuery, and so on. | |
AddressService, AddressLocalService | Address(Local)ServiceUtil, Address(Local)ServiceWrapper | add*, create*, delete*, get*, update*, dynamicQuery, and so on. | |
EmailAddressService, EmailAddressLocalService | PersistedModelLocalService | Address(Local)ServiceUtil, Address(Local)ServiceWrapper | add*, create*, delete*, get*, update*, dynamicQuery, and so on. |
PhoneService, PhoneLocalService | PersistedModelLocalService | Phone(Local)ServiceUtil, Phone(Local)ServiceWrapper | add*, create*, delete*, get*, update*, dynamicQuery, and so on. |
WebsiteService, WebsiteLocalService | PersistedModelLocalService | Website(Local)ServiceUtil, Website(Local)ServiceWrapper | add*, create*, delete*, get*, update*, dynamicQuery, and so on. |
TicketLocalService | PersistedModelLocalService | TicketLocalServiceUtil, TicketLocalServiceWrapper | add*, create*, delete*, get*, update*, dynamicQuery, and so on. |
The portal also defined many-to-many relationships between User and Group, User and Organization, User and Team, User and UserGroup, as shown in the following code:
<column name="groups" type="Collection"
entity="Group" mapping-table="Users_Groups" />
<column name="userGroups" type="Collection"
entity="UserGroup" mapping-table="Users_UserGroups" />
In particular, you will be able to find a similar definition at /portal/service.xml.
The portal provides a sample portal service plugin called sample-portal-service-portlet (refer to the plugin details at /portlets/sample-portal-service-portlet). The following is the code snippet:
List organizations =
OrganizationServiceUtil.getUserOrganizations(
themeDisplay.getUserId());
// add your logic
The previous code shows how to consume Liferay services through regular Java calls. These services include com.liferay.portal.service.OrganizationServiceUtil and the model involves com.liferay.portal.model.Organization. Similarly, you can use other services, for example, com.liferay.portal.service.UserServiceUtil and com.liferay.portal.service.GroupServiceUtil; and models, for example, com.liferay.portal.model.User, com.liferay.portal.model.Group. Of course, you can find other services and models—you will find services located at the com. liferay.portal.service package in the /portal-service/src folder. In the same way, you will find models located at the com.liferay.portal.model package in the /portal-service/src folder.
What's the difference between *LocalServiceUtil and *ServiceUtil? The sign * represents models, for example, Organization, User, Group, and so on. Generally speaking, *Service is the remote service interface that defines the service methods available to remote code. *ServiceUtil has an additional permission check, since this method might be called as a remote service. *ServiceUtil is a facade class that combines the service locator with the actual call to the service *Service. While *LocalService is the internal service interface,*LocalServiceUtil is a facade class that combines the service locator with the actual call to the service *LocalService. *Service has a PermissionChecker in each method, and *LocalService usually doesn't have the same.
Authorization is a process of finding out if the user, once identified, is permitted to have access to a resource. The portal implemented authorization by assigning permissions via roles and checking permissions, and this is called Role-Based Access Control (RBAC).
The following figure depicts an overview of authorization. A user can be a member of Group, UserGroup, Organization, or Team. And a user or a group of users, such as Group, UserGroup, or Organization can be a member of Role. And the entity Role can have many ResourcePermission entities associated with it, while the entity ResourcePermission may contain many ResourceAction entities, as shown in the following diagram:
The following table shows the entities Role, ResourcePermission, and ResourceAction:
Interface | Extension | Wrapper/SOAP | Main methods |
Role | RoleModel, PersistedModel | RoleWrapper, RoleSoap | clone, compareTo, get*, set*, toCacheModel, toEscapedModel, and so on. |
ResourceAction | ResourceActionModel, PersistedModel | ResourceActionWrapper, ResourceActionSoap | clone, compareTo, get*, set*, toCacheModel, toEscapedModel, and so on. |
ResourcePermission | ResourcePermissionModel, PersistedModel | ResourcePermissionWrapper, ResourcePermissionSoap | clone, compareTo, get*, set*, toCacheModel, toEscapedModel, and so on. |
In addition, the portal specifies role constants in the class RoleConstants.
The entity ResourceAction gets specified with the columns name, actionId, and bitwiseValue as follows:
<column name="name" type="String" />
<column name="actionId" type="String" />
<column name="bitwiseValue" type="long" />
The entity ResourcePermission gets specified with the columns name, scope, primKey, roleId, and actionIds as follows:
<column name="name" type="String" />
<column name="scope" type="int" />
<column name="primKey" type="String" />
<column name="roleId" type="long" />
<column name="ownerId" type="long" />
<column name="actionIds" type="long" />
In addition, the portal specified resource permission constants in the class ResourcePermissionConstants
The portal implements enterprise password policies and user account lockout using the entities PasswordPolicy and PasswordPolicyRel, as shown in the following table:
Interface | Extension | Wrapper/Soap | Description |
PasswordPolicy | PasswordPolicyModel, PersistedModel | PasswordPolicyWrapper, PasswordPolicySoap | Columns: name, description, minAge, minAlphanumeric, minLength, minLowerCase, minNumbers, minSymbols, minUpperCase, lockout, maxFailure, lockoutDuration, and so on. |
PasswordPolicyRel | PasswordPolicyRelModel, PersistedModel | PasswordPolicyRelWrapper, PasswordPolicyRelSoap | Columns: passwordPolicyId, classNameId, and classPK.
Ability to associate the entity PasswordPolicy with other entities. |
The portal has defined the following properties related to the passwords toolkit in portal.properties:
passwords.toolkit=
com.liferay.portal.security.pwd.PasswordPolicyToolkit
passwords.passwordpolicytoolkit.generator=dynamic
passwords.passwordpolicytoolkit.static=iheartliferay
The property passwords.toolkit defines a class name that extends com.liferay.portal.security.pwd.BasicToolkit, which is called to generate and validate passwords.
If you choose to use com.liferay.portal.security.pwd.PasswordPolicyToolkit as your password toolkit, you can choose either static or dynamic password generation. Static is set through the property passwords.passwordpolicytoolkit.static and dynamic uses the class com.liferay.util.PwdGenerator to generate the password. If you are using LDAP password syntax checking, you will also have to use the static generator, so that you can guarantee that passwords obey their rules.
The passwords' toolkits get addressed in detail in the following table:
Class | Interface | Utility | Property | Main methods |
DigesterImpl | Digester | DigesterUtil | passwords.digest.encoding | digest, digestBase64, digestHex, digestRaw, and so on. |
Base64 | None | None | None | decode, encode, fromURLSafe, objectToString, stringToObject, toURLSafe, and so on. |
PwdEncryptor | None | None | passwords.encryption.algorithm | encrypt, default types: MD2, MD5, NONE, SHA, SHA-256, SHA-384, SSHA, UFC-CRYPT, and so on . |
Authentication is the process of determining whether someone or something is, in fact, who or what it is declared to be. The portal defines the class called PwdAuthenticator for authentication, as shown in the following code:
public static boolean authenticate(
String login, String clearTextPassword,
String currentEncryptedPassword)
{
String encryptedPassword = PwdEncryptor.encrypt(
clearTextPassword, currentEncryptedPassword);
if (currentEncryptedPassword.equals(encryptedPassword))
{
return true;
}
}
As you can see, it encrypts the clear text password first into the variable encryptedPassword. It then tests whether the variable currentEncryptedPassword has the same value as that of the variable encryptedPassword or not. The classes UserLocalServiceImpl (the method authenticate) and EditUserAction (the method updateUser) call the class PwdAuthenticator for authentication.
A Message Authentication Code (MAC) is a short piece of information used to authenticate a message. The portal supports MAC through the following properties:
auth.mac.allow=false
auth.mac.algorithm=MD5
auth.mac.shared.key=
To use authentication with MAC, simply post to a URL as follows:
It passes the MAC in the password field. Make sure that the MAC gets URL encoded, since it might contain characters not allowed in a URL. Authentication with MAC also requires that you set the following property in system-ext.properties:
com.liferay.util.servlet.SessionParameters=false
As shown in the previous code, it encrypts session parameters, so that browsers can't remember them.
The portal provides the authentication pipeline framework for authentication, as shown in the following code:
auth.pipeline.pre=com.liferay.portal.security.auth.LDAPAuth
auth.pipeline.post=
auth.pipeline.enable.liferay.check=true
As you can see, the property auth.pipeline.enable.liferay.check is set to true to enable password checking by the internal portal authentication. If it is set to false, essentially, password checking is delegated to the authenticators configured in the auth.pipeline.pre and auth.pipeline.post settings.
The interface com.liferay.portal.security.auth.Authenticator defines the constant values that should be used as return code from the classes implementing the interface. If authentication is successful, it returns SUCCESS; if the user exists but the passwords doesn't match, then it returns FAILURE. If the user doesn't exist in the system, it returns DNE. Constants get defined in the interface Authenticator.
As shown in the following table, the available authenticator is com.liferay.portal.security.auth.LDAPAuth:
Class | Extension | Involved properties | Main Methods |
PasswordPolicyToolkit | BasicToolkit | passwords.passwordpolicytoolkit.charset.lowercase, passwords.passwordpolicytoolkit.charset.numbers, passwords.passwordpolicytoolkit.charset.symbols, passwords.passwordpolicytoolkit.charset.uppercase, passwords.passwordpolicytoolkit.generator, passwords.passwordpolicytoolkit.static | generate, validate |
RegExpToolkit | BasicToolkit | passwords.regexptoolkit.pattern, passwords.regexptoolkit.charset, passwords.regexptoolkit.length | generate, validate |
PwdToolkitUtil | None | passwords.toolkit | Generate, validate |
PwdGenerator | None | None | getPassword. getPinNumber |
The portal provides the interface com.liferay.portal.security.auth.AuthToken for the authentication token as follows:
auth.token.check.enabled=true
auth.token.impl=
com.liferay.portal.security.auth.SessionAuthToken
As shown in the previous code, the property auth.token.check.enabled is set to true to enable authentication token security checks. The checks can be disabled for specific actions via the property auth.token.ignore.actions or for specific portlets via the init parameter check-auth-token in portlet.xml.
The property auth.token.impl is set to the authentication token class. This class must implement the interface AuthToken. The class SessionAuthToken is used to prevent CSRF (Cross-Site Request Forgery) attacks.
The following table shows the interface AuthToken and its implementation:
Class | Interface | Properties | Main Methods |
LDAPAuth | Authenticator | ldap.auth.method, ldap.referral, ldap.auth.password.encryption.algorithm, ldap.base.dn, ldap.error.user.lockout, ldap.error.password.expired, ldap.import.user.password.enabled, ldap.base.provider.url, auth.pipeline.enable.liferay.check, ldap.auth.required | authenticateByEmailAddress, authenticateByScreenName, authenticateByUserId |
Java Authentication and Authorization Service (JAAS) is a Java security framework for user-centric security to augment the Java code-based security. The portal has specified a set of properties for JAAS as follows:
portal.jaas.enable=false
portal.jaas.auth.type=userId
portal.impersonation.enable=true
The property portal.jaas.enable is set to false to disable JAAS security checks. Disabling JAAS would speed up login. Note that JAAS must be disabled, if administrators are able to impersonate other users. JAAS can authenticate users based on their e-mail address, screen name, user ID, or login, as determined by the property company.security.auth.type. By default, the class com.liferay.portal.security.jaas.PortalLoginModule loads the correct JAAS login module, based on what application server or servlet container the portal is deployed on. You can set a JAAS implementation class to override this behavior.
The following table shows this class and its associations:
Class | Interface | Properties | Main methods |
AuthTokenImpl | AuthToken | auth.token.impl | check, getToken |
AuthTokenWrapper | AuthToken | None | check, getToken |
AuthTokenUtil | None | None | check, getToken |
SessionAuthToken | AuthToken | auth.token.shared.secret | check, getToken |
As you have noticed, the classes com.liferay.portal.kernel.security.jaas, PortalLoginModule, and com.liferay.portal.security.jaas.PortalLoginModule, implement the interface LoginModule, configured by the property portal.jaas.impl.
As shown in the following table, the portal has provided different login module implementation for different application servers or servlet containers:
Class | Interface/Extension | Package | Main methods |
ProtectedPrincipal | Principal | com.liferay.portal.kernel.servlet | getName, equals, hasCode, toString |
PortalPrincipal | ProtectedPrincipal | com.liferay.portal.kernel.security.jaas | PortalPrincipal |
PortalRole | PortalPrincipal | com.liferay.portal.kernel.security.jaas | PortalRole |
PortalGroup | PortalPrincipal, java.security.acl.Group | com.liferay.portal.kernel.security.jaas | addMember, isMember, members, removeMember |
PortalLoginModule | javax.security.auth.spi.LoginModule | com.liferay.portal.kernel.security.jaas, com.liferay.portal.security.jaas | abort, commit, initialize, login, logout |