










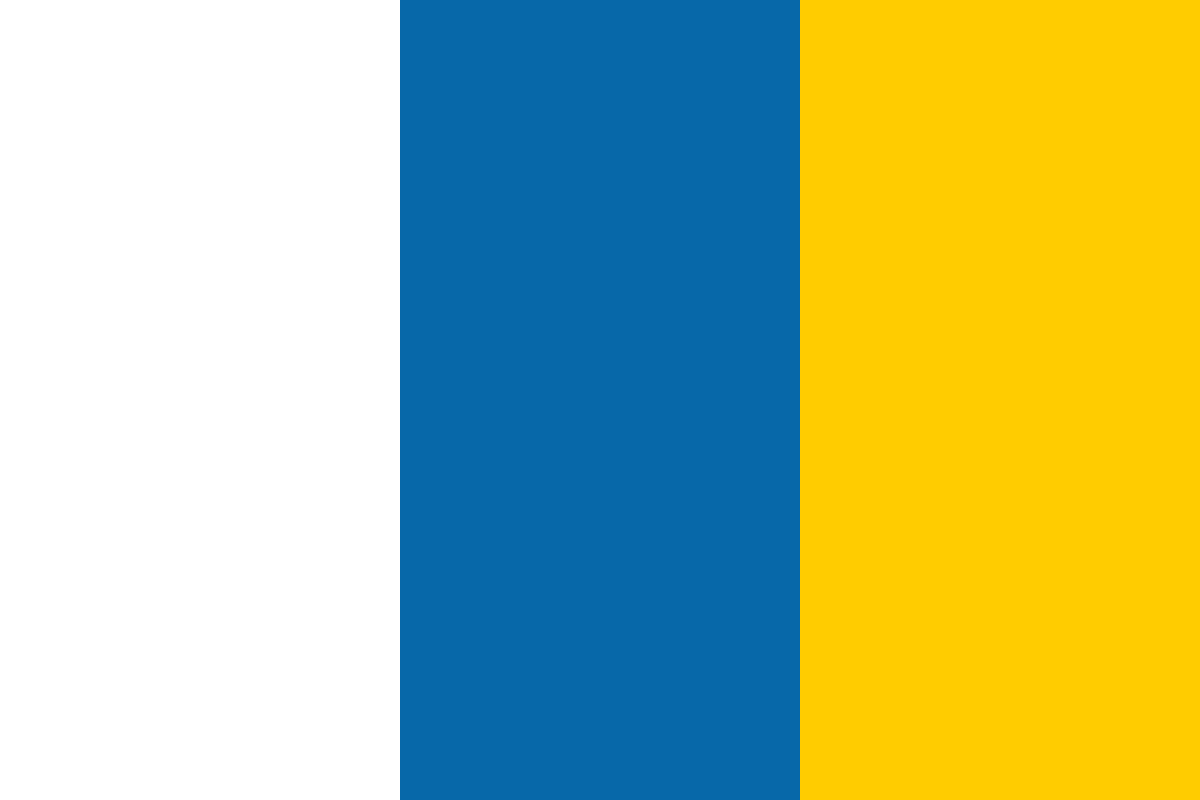

































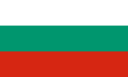








In this article written by Sanjeev Jaiswal and Ratan Kumar, authors of the book Learning Django Web Development, this article will cover all the basic topics which you would require to follow, such as coding practices for better Django web development, which IDE to use, version control, and so on.
We will learn the following topics in this article:
This article is based on the important fact that code is read much more often than it is written. Thus, before you actually start building your projects, we suggest that you familiarize yourself with all the standard practices adopted by the Django community for web development.
Most of Django's important practices are based on Python. Though chances are you already know them, we will still take a break and write all the documented practices so that you know these concepts even before you begin.
To mainstream standard practices, Python enhancement proposals are made, and one such widely adopted standard practice for development is PEP8, the style guide for Python code–the best way to style the Python code authored by Guido van Rossum.
The documentation says, "PEP8 deals with semantics and conventions associated with Python docstrings." For further reading, please visit http://legacy.python.org/dev/peps/pep-0008/.
When you are writing Python code, indentation plays a very important role. It acts as a block like in other languages, such as C or Perl. But it's always a matter of discussion amongst programmers whether we should use tabs or spaces, and, if space, how many–two or four or eight. Using four spaces for indentation is better than eight, and if there are a few more nested blocks, using eight spaces for each indentation may take up more characters than can be shown in single line. But, again, this is the programmer's choice.
The following is what incorrect indentation practices lead to:
>>> def a(): ... print "foo" ... print "bar" IndentationError: unexpected indent
So, which one we should use: tabs or spaces?
Choose any one of them, but never mix up tabs and spaces in the same project or else it will be a nightmare for maintenance. The most popular way of indention in Python is with spaces; tabs come in second. If any code you have encountered has a mixture of tabs and spaces, you should convert it to using spaces exclusively.
There has been a lot of confusion about it, as of course, Python's syntax is all about indentation. Let's be honest: in most cases, it is. So, what is highly recommended is to use four spaces per indentation level, and if you have been following the two-space method, stop using it. There is nothing wrong with it, but when you deal with multiple third party libraries, you might end up having a spaghetti of different versions, which will ultimately become hard to debug.
Now for indentation. When your code is in a continuation line, you should wrap it vertically aligned, or you can go in for a hanging indent. When you are using a hanging indent, the first line should not contain any argument and further indentation should be used to clearly distinguish it as a continuation line.
A hanging indent (also known as a negative indent) is a style of indentation in which all lines are indented except for the first line of the paragraph. The preceding paragraph is the example of hanging indent.
The following example illustrates how you should use a proper indentation method while writing the code:
bar = some_function_name(var_first, var_second, var_third, var_fourth) # Here indentation of arguments makes them grouped, and stand clear from others. def some_function_name( var_first, var_second, var_third, var_fourth): print(var_first) # This example shows the hanging intent.
We do not encourage the following coding style, and it will not work in Python anyway:
# When vertical alignment is not used, Arguments on the first line are forbidden foo = some_function_name(var_first, var_second, var_third, var_fourth) # Further indentation is required as indentation is not
distinguishable between arguments and source code. def some_function_name( var_first, var_second, var_third, var_fourth): print(var_first)
Although extra indentation is not required, if you want to use extra indentation to ensure that the code will work, you can use the following coding style:
# Extra indentation is not necessary. if (this and that): do_something()
Ideally, you should limit each line to a maximum of 79 characters. It allows for a + or – character used for viewing difference using version control. It is even better to limit lines to 79 characters for uniformity across editors. You can use the rest of the space for other purposes.
The importance of two blank lines and single blank lines are as follows:
Importing a package is a direct implication of code reusability. Therefore, always place imports at the top of your source file, just after any module comments and document strings, and before the module's global and constants as variables. Each import should usually be on separate lines.
The best way to import packages is as follows:
import os import sys
It is not advisable to import more than one package in the same line, for example:
import sys, os
You may import packages in the following fashion, although it is optional:
from django.http import Http404, HttpResponse
If your import gets longer, you can use the following method to declare them:
from django.http import ( Http404, HttpResponse, HttpResponsePermanentRedirect )
Package imports can be grouped in the following ways:
import re
import simplejson
from decimal import *
from models import ModelFoo
from models import ModelBar
Every programming language and framework has its own naming convention. The naming convention in Python/Django is more or less the same, but it is worth mentioning it here. You will need to follow this while creating a variable name or global variable name and when naming a class, package, modules, and so on.
This is the common naming convention that we should follow:
There are many options on the market when it comes to source code editors. Some people prefer full-fledged IDEs, whereas others like simple text editors. The choice is totally yours; pick up whatever feels more comfortable. If you already use a certain program to work with Python source files, I suggest that you stick to it as it will work just fine with Django. Otherwise, I can make a couple of recommendations, such as these:
Most of the examples that we will show you in this book will be written using Sublime text editor. In this section, we will show how to install and set up the Django project.
After successful installation, it will look like this:
Most important of all is Package Control, which is the manager for installing additional plugins directly from within Sublime. This will be your only manual installation of the package. It will take care of the rest of the package installation ahead.
Some of the recommendations for Python development using Sublime are as follows:
You can also explore other plugins for Sublime to increase your productivity.
You can use any of your favorite IDEs for Django project development. We will use pycharm IDE for this book. This IDE is recommended as it will help you at the time of debugging, using breakpoints that will save you a lot of time figuring out what actually went wrong.
Here is how to install and set up pycharm IDE for Django:
Download and installation: You can check the features and download the pycharm IDE from the following link:
http://www.jetbrains.com/pycharm/
The Django project structure has been changed in the 1.6 release version. Django (django-admin.py) also has a startapp command to create an application, so it is high time to tell you the difference between an application and a project in Django.
A project is a complete website or application, whereas an application is a small, self-contained Django application. An application is based on the principle that it should do one thing and do it right.
To ease out the pain of building a Django project right from scratch, Django gives you an advantage by auto-generating the basic project structure files from which any project can be taken forward for its development and feature addition.
Thus, to conclude, we can say that a project is a collection of applications, and an application can be written as a separate entity and can be easily exported to other applications for reusability.
To create your first Django project, open a terminal (or Command Prompt for Windows users), type the following command, and hit Enter:
$ django-admin.py startproject django_mytweets
This command will make a folder named django_mytweets in the current directory and create the initial directory structure inside it. Let's see what kind of files are created.
The new structure is as follows:
django_mytweets/// django_mytweets/ manage.py
This is the content of django_mytweets/:
django_mytweets/ __init__.py settings.py urls.py wsgi.py
Here is a quick explanation of what these files are:
This means that you can import other third party applications on the same level as the Django project. This folder also contains the manage.py file, which include all the project management settings.
Further clarification about the settings will be provided when are going to tweak the changes.
Let's have a look at the manage.py file:
#!/usr/bin/env python import os import sys if __name__ == "__main__": os.environ.setdefault("DJANGO_SETTINGS_MODULE", "django_mytweets.settings") from django.core.management import execute_from_command_line execute_from_command_line(sys.argv)
The source code of the manage.py file will be self-explanatory once you read the following code explanation.
#!/usr/bin/env python
The first line is just the declaration that the following file is a Python file, followed by the import section in which os and sys modules are imported. These modules mainly contain system-related operations.
import os import sys
The next piece of code checks whether the file is executed by the main function, which is the first function to be executed, and then loads the Django setting module to the current path. As you are already running a virtual environment, this will set the path for all the modules to the path of the current running virtual environment.
if __name__ == "__main__": os.environ.setdefault("DJANGO_SETTINGS_MODULE", "django_mytweets.settings") django_mytweets/ ( Inner folder) __init__.py
Django projects are Python packages, and this file is required to tell Python that this folder is to be treated as a package. A package in Python's terminology is a collection of modules, and they are used to group similar files together and prevent naming conflicts.
By default, the database is configured to use SQLite Database, which is advisable to use for testing purposes. Here, we will only see how to enter the database in the settings file; it also contains the basic setting configuration, and with slight modification in the manage.py file, it can be moved to another folder, such as config or conf.
To make every other third-party application a part of the project, we need to register it in the settings.py file. INSTALLED_APPS is a variable that contains all the entries about the installed application. As the project grows, it becomes difficult to manage; therefore, there are three logical partitions for the INSTALLED_APPS variable, as follows:
When we start writing code for our application, we will create new files inside the project's folder. So, the folder also serves as a container for our code.
Now that you have a general idea of the structure of a Django project, let's configure our database system.
We prepared our development environment in this article, created our first project, set up the database, and learned how to launch the Django development server. We learned the best way to write code for our Django project and saw the default Django project structure.
Further resources on this subject: