










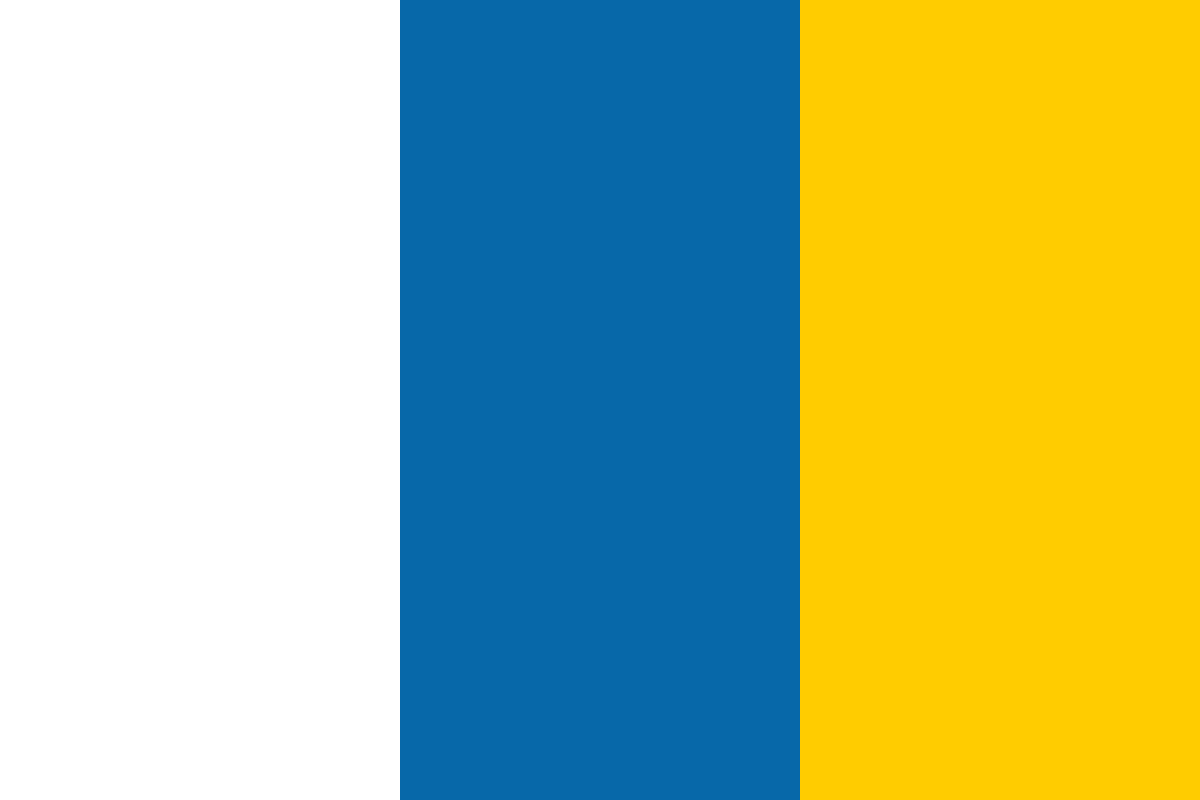

































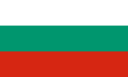








In this article by Jorge R. Castro, author of Building a Home Security System with Arduino, you will learn to read a technical specification sheet, the ports on the Uno and how they work, the necessary elements for a proof of concept. Finally, we will extend the capabilities of our script in Python and rely on NFC technology.
We will cover the following points:
(For more resources related to this topic, see here.)
There are many ways of working on and testing electrical components, one of the ways developers use is using ProtoBoards (also known as breadboards). Breadboards help eliminate the need to use soldering while testing out components and prototype circuit schematics, it lets us reuse components, enables rapid development, and allows us to correct mistakes and even improve the circuit design. It is a rectangle case composed of internally interconnected rows and columns; it is possible to couple several together. The holes on the face of the board are designed to in way that you can insert the pins of components to mount it.
ProtoBoards may be the intermediate step before making our final PCB design, helping eliminate errors and reduce the associated cost. For more information visit http://en.wikipedia.org/wiki/Breadboard.
We know have to know more about the wrong wiring techniques when using a breadboard. There are rules that have always been respected, and if not followed, it might lead to shorting out elements that can irreversibly damage our circuit.
A ProtoBoard and an Arduino Uno
The ProtoBoard is shown in the preceding image; we can see there are mainly two areas - the vertical columns (lines that begin with the + and - polarity sign), and the central rows (with a coordinate system of letters and numbers). Both + and - usually connect to the electrical supply of our circuit and to the ground. It is advised not to connect components directly in the vertical columns, instead to use a wire to connect to the central rows of the breadboard. The central row number corresponds to a unique track. If we connected one of the leads of a resistor in the first pin of the first row then other lead should be connected to any other pin in another row, and not in the same row.
There is an imaginary axis that divides the breadboard vertically in symmetrical halves, which implies that they are electrically independent. We will use this feature to use certain Integrated Circuits (IC), and they will be mounted astride the division.
Protoboard image obtained using Fritzing.
Carefully note that in the preceding picture, the top half of the breadboard shows the components mounted incorrectly, while the lower half shows the components mounted the correct way. A chip is set correctly between the two areas, this usage is common for IC's.
Fritzing is a tool that helps us create a quick circuit design of our project. Visit http://fritzing.org/download/ for more details on Fritzing.
Now that we know about the correct usage of a breadboard, we now have another very important concept - ports. Our board will be composed of various inputs and outputs, the number of which varies with the kind of model we have.
But what are ports? The Arduino UNO board has ports on its sides. They are connections that allow it to interact with sensors, actuators, and other devices (even with another Arduino board). The board also has ports that support digital and analog signals. The advantage is that these ports are bidirectional, and the programmers are able to define the behavior of these ports.
In the following code, the first part shows that we set the status of the ports that we are going to use. This is the setup function:
//CODE
void setup(){ // Will run once, and use it to
// "prepare" I/0 pins of Arduino
pinMode(10, OUTPUT); // put the pin 10 as an output
pinMode(11, INPUT); // put the pin 11 as an input
}
Lets take a look at what the digital and analog ports are on the Arduino UNO.
Analog signals are signals that continuously vary with time These can be explained as voltages that have continuously varying intermediate values. For example, it may vary from 2V to 3.3V to 3.0V, or 3.333V, which means that the voltages varied progressively with time, and are all different values from each other; the figures between the two values are infinite (theoretical value). This is an interesting property, and that is what we want. For example, if we want to measure the temperature of a room, the temperature measure takes values with decimals, need an analog signal. Otherwise, we will lose data, for example, in decimal values since we are not able to store infinite decimal numbers we perform a mathematical rounding (truncating the number). There is a process called discretization, and it is used to convert analog signals to digital.
In reality, in the world of microcontrollers, the difference between two values is not infinite. The Arduino UNO's ports have a range of values between 0 and 1023 to describe an analog input signal. Certain ports, marked as PWM or by a ~, can create output signals that vary between values 0 and 255.
A digital signal consists of just two values - 0s and 1s. Many electronic devices internally have a range currently established, where voltage values from 3.5 to 5V are considered as a logic 1, and voltages from 0 to 2.5V are considered as a logic 0.
To better understand this point, let's see an example of a button that triggers an alarm. The only useful cases for an alarm are when the button is pressed to ring the alarm and when it's not pressed; there are only two states observed. So unlike the case of the temperature sensor, where we can have a range of linear values, here only two values exist. Logically, we use these properties for different purposes.
The Arduino IDE has some very illustrative examples. You can load them onto your board to study the concepts of analog and digital signals by navigating to File | Examples | Basic | Blink.
There is a wide range of sensors, and without pretending to be an accurate guide of all possible sensors, we will try to establish some small differences.
All physical properties or sets of them has an associated sensor, so we can measure the force (presence of people walking on the floor), movement (physical displacement even in the absence of light), smoke, gas, pictures (yes, the cameras also are sensors) noise (sound recording), antennas (radio waves, WiFi, and NFC), and an incredible list that would need a whole book to explain all its fantastic properties.
It is also interesting to note that the sensors can also be specific and concrete; however, they only measure very accurate or nonspecific properties, being able to perceive a set of values but more accurately. If you go to an electronics store or a sales portal buy a humidity sensor (and generally any other electronic item), you will see a sensitive price range. In general, expensive sensors indicate that they are more reliable than their cheaper counterparts, the price also indicates the kinds of conditions that it can work in, and also the duration of operation. Expensive sensors can last more than the cheaper variants.
When we started looking for a component, we looked to the datasheets of a particular component (the next point will explain what this document is), you will not be surprised to find two very similar models mentioned in the datasheets. It contains operating characteristics and different prices. Some components are professionally deployed (for instance in the technological and military sectors), while others are designed for general consumer electronics.
Here, we will focus on those with average quality and foremost an economic price. We proceed with an example that will serve as a liaison with the following paragraph. To do this, we will use a temperature sensor (TMP-36) and an analog port on the Arduino UNO. The following image shows the circuit schematic:
Our design - designed using Fritzing
The following is the code for the preceding circuit schematic:
//CODE
//###########################
//Author : Jorge Reyes Castro
//Arduino Home Security Book
//###########################
// A3 <- Input
// Sensor TMP36
//###########################
//Global Variable
int outPut = 0;
float outPutToVol = 0.0;
float volToTemp = 0.0;
void setup(){
Serial.begin(9600); // Start the SerialPort 9600 bauds
}
void loop(){
outPut = analogRead(A3);
// Take the value from the A3 incoming port
outPutToVol = 5.0 *(outPut/1024.0);
// This is calculated with the values
volToTemp = 100.0 *(outPutToVol - 0.5);
// obtained from the datasheet
Serial.print("_____________________n");
mprint("Output of the sensor ->", outPut);
mprint("Conversion to voltage ->", outPutToVol);
mprint("Conversion to temperature ->", volToTemp);
delay(1000); // Wait 1 Sec.
}
// Create our print function
// smarter than repeat the code and it will be reusable
void mprint(char* text, double value){
// receives a pointer to the text and a numeric value of type double
Serial.print(text);
Serial.print("t"); // tabulation
Serial.print(value);
Serial.print("n"); // new line
}
Now, open a serial port from the IDE or just execute the previous Python script. We can see the output from the Arduino. Enter the following command to run the code as python script:
$ python SerialPython.py
The output will look like this:
____________________
Output of the sensor 145.00
Conversion to voltage 0.71
Conversion to temperature 20.80
_____________________
By using Python script, which is not simple, we managed to extract some data from a sensor after the signal is processed by the Arduino UNO. It is then extracted and read by the serial interface (script in Python) from the Arduino UNO. At this point, we will be able to do what we want with the retrieved data, we can store them in a database, represent them in a function, create a HTML document, and more.
As you may have noticed we make a mathematical calculation. However, from where do we get this data? The answer is in the data sheet.
Whenever we need to work with or handle an electrical component, we have to study their properties. For this, there are official documents, of variable length, in which the manufacturer carefully describes the characteristics of that component.
First of all, we, have to identify that a component can either use the unique ID or model name that it was given when it was manufactured.
TMP 36GZ
We have very carefully studied the component surface and thus extracted the model. Then, using an Internet browser, we can quickly find the datasheet. Go to http://www.google.com and search for: TMP36GZ + datasheet, or visit http://dlnmh9ip6v2uc.cloudfront.net/datasheets/Sensors/Temp/TMP35_36_37.pdf to get the datasheet.
Once you have the datasheet, you can see that it has various components which look similar, but with very different presentations (this can be crucial for a project, as an error in the choice of encapsulated (coating/presentation) can lead to a bitter surprise). Just as an example, if we confuse the presentation of our circuit, we might end up buying components used in cellphones, and those can hardly be soldered by a standard user as they smaller than your pinkie fingernail). Therefore, an important property to which you must pay attention is the coating/presentation of the components.
In the initial pages of the datasheet you see that it shows you the physical aspects of the component. It then show you the technical characteristics, such as the working current, the output voltage, and other characteristics which we must study carefully in order to know whether they will be consistent with our setup. In this case, we are working with a range of input voltage (V) of between 2.7 V to 5.5 V is perfect. Our Arduino has an output of 5V. Furthermore, the temperature range seems appropriate. We do not wish to measure anything below 0V and 5V. Let's study the optimal behavior of the components in temperature ranges in more detail.
The behavior of the components is stable only between the desired ranges. This is a very important aspect because although the measurement can be done at the ends supported by the sensor, the error will be much higher and enormously increases the deviation to small variations in ambient temperature. Therefore, always choose or build a circuit with components having the best performance as shown in the optimal region of the graph of the datasheet. Also, always respect and observe that the input voltages do not exceed the specified limit, otherwise it will be irreversibly damaged.
To know more about ADC visit http://en.wikipedia.org/wiki/Analog-to-digital_converter.
Once we have the temperature sensor, we have to extract the data you need. Therefore, we make a simple calculation supported in the datasheet. As we can see, we feed 5V to the sensor, and it return values between 0 and 1023. So, we use a simple formula that allows us to convert the values obtained in voltage (analog value):
Voltage = (Value from the sensor) x (5/1024)
The value 1024 is correct, as we have said that the data can range from 0 (including zero as a value) to 1023. This data can be strange to some, but in the world of computer and electronics, zero is seen as a very important value. Therefore, be careful when making calculations.
Once we obtain a value in volts, we proceed to convert this measurement in a data in degrees. For this, by using the formula from the datasheet, we can perform the conversion quickly. We use a variable that can store data with decimal point (double or float), otherwise we will be truncating the result and losing valuable information (this may seem strange, but this bug very common).
The formula for conversion is Cº = (Voltage -0.5) x 100.0.
We now have all the data we need and there is a better implementation of this sensor, in which we can eliminate noise and disturbance from the data. You can dive deeper into these issues if desired. With the preceding explanation, it will not be difficult to achieve.
Near Field Communication (NFC) technology is based on the RFID technology. Basically, the principle consists of two devices fused onto one board, one acting as an antenna to transmit signals and the other that acts as a reader/reciever. It exchanges information using electromagnetic fields. They deal with small pieces of information. It is enough for us to make this extraordinary property almost magical.
For more information on RFID and NFC, visit the following links:
http://en.wikipedia.org/wiki/Near_field_communication
http://en.wikipedia.org/wiki/Radio-frequency_identification
Today, we find this technology in identification and access cards (a pioneering example is the Document ID used in countries like Spain), tickets for public transport, credit cards, and even mobile phones (with ability to make payment via NFC).
For this project, we will use a popular module PN532 Adafruit RFID/NFC board. Feel free to use any module that suits your needs. I selected this for its value, price, and above all, for its chip. The popular PN532 is largely supported by the community, and there are vast number of publications, tools, and libraries that allow us to integrate it in many projects.
For instance, you can use it in conjunction with an Arduino a Raspberry Pi, or directly with your computer (via a USB to serial adapter). Also, the PN532 board comes with a free Mifare 1k card, which we can use for our project.
You can also buy tag cards or even key rings that house a small circuit inside, on which information is stored, even encrypted information. One of the benefits, besides the low price of a set of labels, is that we can block access to certain areas of information or even all the information. This allows us in maintaining our valuable data safely or avoid the cloned/duplicate of this security tag.
There are a number of standards that allow us to classify the different types of existing cards, one of which is the Mifare 1K card (You can use the example presented here and make small changes to adapt it to other NFC cards).
For more information on MIFARE cards, visit http://en.wikipedia.org/wiki/MIFARE.
As the name suggests, this model can store up to 1KB (Kilobyte) information, and is of the EEPROM type (can be rewritten). Furthermore, it possesses a serial number unique to each card (this is very interesting because it is a perfect fingerprint that will allow us to distinguish between two cards with the same information).
Internally, it is divided into 16 sectors (0-15), and is subdivided into 4 blocks (0-3) with at least 16 bytes of information. For each block, we can set a password that prevents reading your content if it does not pose. (At this point, we are not going to manipulate the key because the user can accidentally, encrypt a card, leaving it unusable). By default, all cards usually come with a default password (ffffffffffff).
The reader should consult the link http://www.adafruit.com/product/789, to continue with the examples, or if you select another antenna, search the internet for the same features. The link also has tutorials to make the board compatible with other devices (Raspberry Pi), the datasheet, and the library can be downloaded from Github to use this module.
We will have a look at this last point more closely later; just download the libraries for now and follow my steps. It is one of the best advantages of open hardware, that the designs can be changed and improved by anyone.
Remember, when handling electrical components, we have to be careful and avoid electrostatic sources.
As you have already seen, the module is inserted directly on the Arduino. If soldered pins do not engage directly above then you have to use Dupont male-female connectors to be accessible other unused ports. Once we have the mounted module, we will use the library that is used to control this device (top link) and install it. It is very important that you rename the library to download, eliminate possible blanks or the like. However, the import process may throw an error.
Once we have this ready and have our Mifare 1k card close, let's look at a small example that will help us to better understand all this technology and get the UID (Unique Identifier) of our tag:
// ###########################
// Author : Jorge Reyes Castro
// Arduino Home Security Book
// ###########################
#include <Wire.h>
#include <Adafruit_NFCShield_I2C.h>
// This is the Adafruit Library
//MACROS
#define IRQ (2)
#define RESET (3)
Adafruit_NFCShield_I2C nfc(IRQ, RESET); //Prepare NFC MODULE
//SETUP
void setup(void){
Serial.begin(115200); //Open Serial Port
Serial.print("###### Serial Port Ready ######n");
nfc.begin(); //Start NFC
nfc.SAMConfig();
if(!Serial){ //If the serial port don´ work wait
delay(500);
}
}
//LOOP
void loop(void) {
uint8_t success;
uint8_t uid[] = { 0, 0, 0, 0}; //Buffer to save the read value
//uint8_t ok[] = { X, X, X, X }; //Put your serial Number. * 1
uint8_t uidLength;
success = nfc.readPassiveTargetID(PN532_MIFARE_ISO14443A, uid,
&uidLength); // READ
// If TAG PRESENT
if (success) {
Serial.println("Found cardn");
Serial.print(" UID Value: t");
nfc.PrintHex(uid, uidLength); //Convert Bytes to Hex.
int n = 0;
Serial.print("Your Serial Number: t");
// This function show the real value
// 4 position runs extracting data
while (n < 4){
// Copy and remenber to use in the next example * 1
Serial.print(uid[n]);
Serial.print("t");
n++;
}
Serial.println("");
}
delay(1500); //wait 1'5 sec
}
Now open a serial port from the IDE or just execute the previous Python script. We can see the output from the Arduino. Enter the following command to run the Python code:
$ python SerialPython.py
The output will look like this:
###### Serial Port Ready ######
Found card
UID Value: 0xED 0x05 0xED 0x9A
Your Serial Number: 237 5 237 154
So we have our own identification number, but what are those letters that appear above our number? Well, it is hexadecimal, another widely used way to represent numbers and information in the computer. It represents a small set of characters and more numbers in decimal notation than we usually use. (Remember our card with little space. We use hexadecimal to be more useful to save memory). An example is the number 15, we need two characters in tenth, while in decimal just one character is needed f (0xf, this is how the hexadecimal code, preceded by 0x, this is a thing to remember as this would be of great help later on).
Open a console and the Python screen. Run the following code to obtain hexadecimal numbers, and will see transformation to decimal (you can replace them with the values previously put in the code):
For more information about numbering systems, see http://en.wikipedia.org/wiki/Hexadecimal.
$ python
>>> 0xED
237
>>> 0x05
5
>>> 0x9A
154
You can see that they are same numbers.
RFID/NFC module and tag
Once we are already familiar with the use of this technology, we proceed to increase the difficultly and create our little access control. Be sure to record the serial number of your access card (in decimal).
We will make this little montage focus on the use of NFC cards, no authentication key. As mentioned earlier, there is a variant of this exercise and I suggest that the reader complete this on their part, thus increasing the robustness of our assembly. In addition, we add a LED and buzzer to help us improve the usability.
The objective is simple: we just have to let people in who have a specific card with a specific serial number. If you want to use encrypted keys, you can change the ID of an encrypted message inside the card with a key known only to the creator of the card. When a successful identification occurs, a green flash lead us to report that someone has had authorized access to the system. In addition, the user is notified of the access through a pleasant sound, for example, will invite you to push the door to open it.
Otherwise, an alarm is sounded repeatedly, alerting us of the offense and activating an alert command center (a red flash that is repeated and consistent, which captures the attention of the watchman.)
Feel free to add more elements. The diagram shall be as follows:
Our scheme – Image obtained using Fritzing
The following is a much clearer representation of the preceding wiring diagram as it avoids the part of the antenna for easy reading:
Our design - Image obtained using Fritzing
Once we clear the way to take our design, you can begin to connect all elements and then create our code for the project. The following is the code for the NFC access:
//###########################
//Author : Jorge Reyes Castro
//Arduino Home Security Book
//###########################
#include <Wire.h>
#include <Adafruit_NFCShield_I2C.h> // this is the Adafruit
Library
//MACROS
#define IRQ (2)
#define RESET (3)
Adafruit_NFCShield_I2C nfc(IRQ, RESET); //Prepare NFC MODULE
void setup(void) {
pinMode(5, OUTPUT); // PIEZO
pinMode(9, OUTPUT); // LED RED
pinMode(10, OUTPUT); // LED GREEN
okHAL();
Serial.begin(115200); //Open Serial Port
Serial.print("###### Serial Port Ready ######n");
nfc.begin(); //Start NFC
nfc.SAMConfig();
if(!Serial){ // If the Serial Port don't work wait
delay(500);
}
}
void loop(void) {
uint8_t success;
uint8_t uid[] = { 0, 0, 0, 0};
//Buffer to storage the ID from the read tag
uint8_t ok[] = { 237, 5, 237, 154};
//Put your serial Number.
uint8_t uidLength;
success = nfc.readPassiveTargetID(PN532_MIFARE_ISO14443A, uid,
&uidLength); //READ
if (success) {
okHAL(); // Sound "OK"
Serial.println("Found cardn");
Serial.print(" UID Value: t");
nfc.PrintHex(uid, uidLength);
// The Value from the UID in HEX
int n = 0;
Serial.print("Your Serial Number: t");
// The Value from the UID in DEC
while (n < 4){
Serial.print(uid[n]);
Serial.print("t");
n++;
}
Serial.println("");
Serial.println(" Wait..n"); // Verification
int m = 0, l = 0;
while (m < 5){
if(uid[m] == ok[l]){
// Compare the elements one by one from the obtained UID
card that was stored previously by us.
}
else if(m == 4){
Serial.println("###### Authorized ######n"); // ALL OK
authOk();
okHAL();
}
else{
Serial.println("###### Unauthorized ######n");
// NOT EQUALS ALARM !!!
authError();
errorHAL();
m = 6;
}
m++;
l++;
}
}
delay(1500);
}
//create a function that allows us to quickly create sounds
// alarm ( "time to wait" in ms, "tone/power")
void alarm(unsigned char wait, unsigned char power ){
analogWrite(5, power);
delay(wait);
analogWrite(5, 0);
}
// HAL OK
void okHAL(){
alarm(200, 250);
alarm(100, 50);
alarm(300, 250);
}
// HAL ERROR
void errorHAL(){
int n = 0 ;
while(n< 3){
alarm(200, 50);
alarm(100, 250);
alarm(300, 50);
n++;
}
}
// These functions activated led when we called.
// (Look at the code of the upper part where they are used)
// RED - FAST
void authError(){
int n = 0 ;
while(n< 20){
digitalWrite(9, HIGH);
delay(500);
digitalWrite(9, LOW);
delay(500);
n++;
}
}
// GREEN - SLOW
void authOk(){
int n = 0 ;
while(n< 5){
digitalWrite(10, HIGH);
delay(2000);
digitalWrite(10, LOW);
delay(500);
n++;
}
}
// This code can be reduced to increase efficiency and speed of
execution,
// but has been created with didactic purposes and therefore
increased readability
Once you have the copy, compile it, and throw it on your board. Ensure that our creation now has a voice, whenever we start communicating or when someone tries to authenticate. In addition, we have added two simple LEDs that give us much information about our design.
Finally, we have our whole system, perhaps it will be time to improve our Python script and try the serial port settings, creating a red window or alarm sound on the computer that is receiving the data. It is the turn of reader to assimilate all of the concepts seen in this day and modify them to delve deeper and deeper into the wonderful world of programming.
This has been an intense article, full of new elements. We learned to handle technical documentation fluently, covered the different type of signals and their main differences, found the perfect component for our needs, and finally applied it to a real project, without forgetting the NFC. This has just been introduced, laying the foundation for the reader to be able to modify and study it deeper it in the future.
Further resources on this subject: