










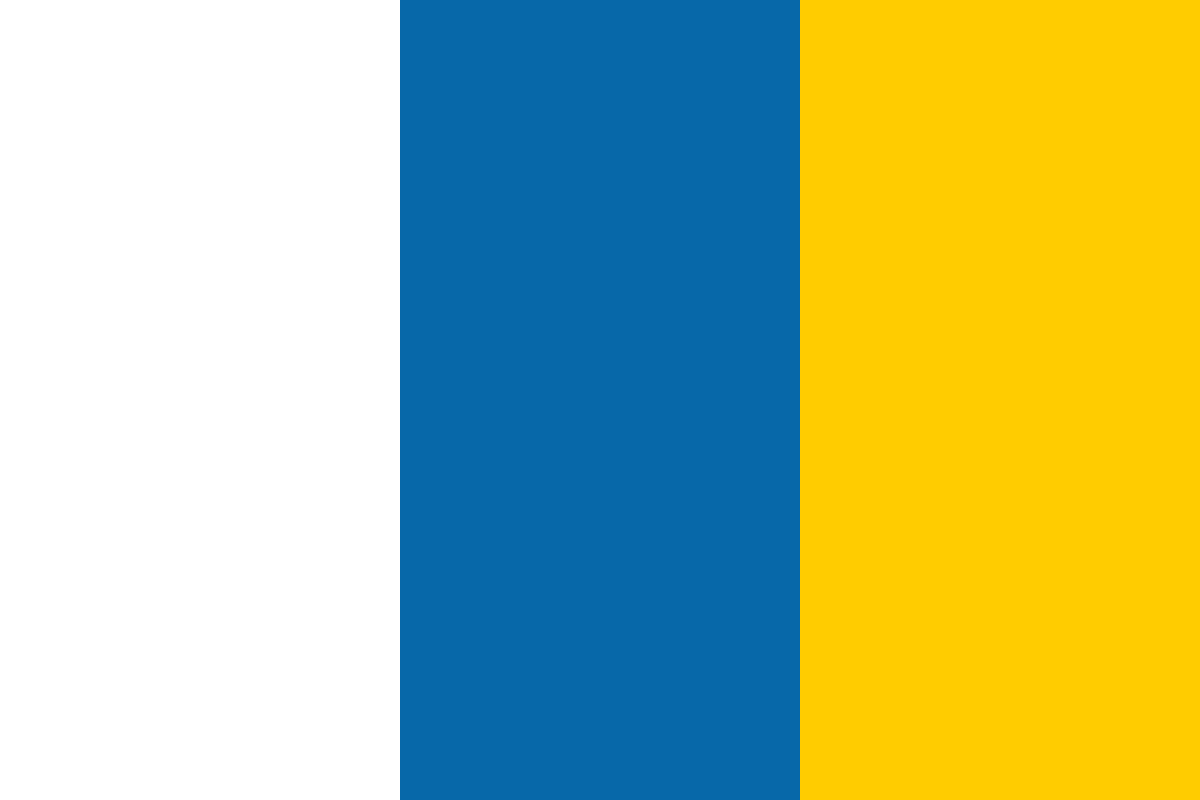

































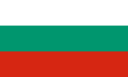








(For more resources related to this topic, see here.)
Before you get started with setting up your first ZF2 Project, make sure that you have the following software installed and configured in your development environment:
The following commands will be useful for installing the necessary tools to setup a ZF2 Project:
To install PHP Command Line Interface:
$ sudo apt-get install php5-cli
To install Git:
$ sudo apt-get install git
To install Composer:
$ curl -s https://getcomposer.org/installer | php
ZendSkeletonApplication provides a sample skeleton application that can be used by developers as a starting point to get started with Zend Framework 2.0. The skeleton application makes use of ZF2 MVC, including a new module system.
ZendSkeletonApplication can be downloaded from GitHub (https://github.com/zendframework/ZendSkeletonApplication).
To set up a new Zend Framework project, we will need to download the latest version of ZendSkeletonApplication and set up a virtual host to point to the newly created Zend Framework project. The steps are given as follows:
$ cd /var/www/
$ git clone git://github.com/zendframework/ ZendSkeletonApplication.git CommunicationApp
In some Linux configurations, necessary permissions may not be available to the current user for writing to /var/www. In such cases, you can use any folder that is writable and make necessary changes to the virtual host configuration.
$ cd CommunicationApp/ $ php composer.phar self-update $ php composer.phar install
The following screenshot shows how Composer downloads and installs the necessary dependencies:
$ sudo vim /etc/hosts
In Windows, this file can be accessed at %SystemRoot%system32driversetchosts.
127.0.0.1 comm-app.local
The final hosts file should look like the following:
$ sudo vim /usr/local/zend/etc/sites.d/vhost_comm-app-80.conf
This new virtual host filename could be different for you depending upon the web server that you use; please check out your web server documentation for setting up new virtual hosts.
For example, if you have Apache2 running on Linux, you will need to create the new virtual host file in /etc/apache2/sites-available and enable the site using the command a2ensite comm-app.local.
<VirtualHost *:80> ServerName comm-app.local DocumentRoot /var/www/CommunicationApp/public SetEnv APPLICATION_ENV "development" <Directory /var/www/CommunicationApp/public> DirectoryIndex index.php AllowOverride All Order allow,deny Allow from all </Directory> </VirtualHost>
If you are using a different path for checking out the ZendSkeletonApplication project make sure that you include that path for both DocumentRoot and Directory directives.
$ sudo service zend-server restart
Test rewrite rules
In some cases, mod_rewrite may not have been enabled in your web server by default; to check if the URL redirects are working properly, try to navigate to an invalid URL such as http://comm-app.local/12345; if you get an Apache 404 page, then the .htaccess rewrite rules are not working; they will need to be fixed, otherwise if you get a page like the following one, you can be sure of the URL working as expected.
We have successfully created a new ZF2 project by checking out ZendSkeletonApplication from GitHub and have used Composer to download the necessary dependencies including Zend Framework 2.0. We have also created a virtual host configuration that points to the project's public folder and tested the project in a web browser.
Alternate installation options
We have seen just one of the methods of installing ZendSkeletonApplication; there are other ways of doing this.
You can use Composer to directly download the skeleton application and create the project using the following command:
$ php composer.phar create-project --repositoryurl="
http://packages.zendframework.com" zendframework/
skeleton-application path/to/install
You can also use a recursive Git clone to create the same project:
$ git clone git://github.com/zendframework/
ZendSkeletonApplication.git --recursive
Refer to:
http://framework.zend.com/downloads/skeleton-app
In Zend Framework, a module can be defined as a unit of software that is portable and reusable and can be interconnected to other modules to construct a larger, complex application.
Modules are not new in Zend Framework, but with ZF2, there is a complete overhaul in the way modules are used in Zend Framework. With ZF2, modules can be shared across various systems, and they can be repackaged and distributed with relative ease. One of the other major changes coming into ZF2 is that even the main application is now converted into a module; that is, the application module.
Some of the key advantages of Zend Framework 2.0 modules are listed as follows:
The folder layout of a ZF2 project is shown as follows:
Folder name
|
Description
|
config
|
Used for managing application configuration.
|
data
|
Used as a temporary storage location for storing application
data including cache files, session files, logs, and indexes.
|
module
|
Used to manage all application code.
|
module/Application
|
This is the default application module that is provided with
ZendSkeletonApplication.
|
public
|
This is the default application module that is provided with
ZendSkeletonApplication.
|
vendor
|
Used to manage common libraries that are used by the
application. Zend Framework is also installed in this folder.
|
vendor/zendframework
|
endor/zendframework Zend Framework 2.0 is installed here.
|
Our next activity will be about creating a new Users module in Zend Framework 2.0. The Users module will be used for managing users including user registration, authentication, and so on. We will be making use of ZendSkeletonModule provided by Zend, shown as follows:
$ cd /var/www/CommunicationApp/ $ cd module/
$ git clone git://github.com/zendframework/ZendSkeletonModule.git Users
* Users/src/ZendSkeletonModule
* Users/view/zend-skeleton-module
We have installed a skeleton module for Zend Framework; this is just an empty module, and we will need to extend this by creating custom controllers and views. In our next activity, we will focus on creating new controllers and views for this module.
Creating a module using ZFTool
ZFTool is a utility for managing Zend Framework applications/projects, and it can also be used for creating new modules; in order to do that, you will need to install ZFTool and use the create module command to create the module using ZFTool:
$ php composer.phar require zendframework/zftool:dev-master $ cd vendor/zendframework/zftool/ $ php zf.php create module Users2 /var/www/CommunicationApp
Read more about ZFTool at the following link:
http://framework.zend.com/manual/2.0/en/modules/zendtool.introduction.html
The fundamental goal of any MVC Framework is to enable easier segregation of three layers of the MVC, namely, model, view, and controller. Before we get to the details of creating modules, let's quickly try to understand how these three layers work in an MVC Framework:
The folder structure of Zend Framework 2.0 module has three vital components—the configurations, the module logic, and the views. The following table describes how contents in a module are organized:
Folder name
|
Description
|
config
|
Used for managing module configuration
|
src
|
Contains all module source code, including all controllers and models
|
view
|
Used to store all the views used in the module
|
Now that we have created the module, our next step would be having our own controllers and views defined. In this section, we will create two simple views and will write a controller to switch between them:
$ cd /var/www/CommunicationApp/module/Users
$ mkdir -p src/Users/Controller/
$ cd src/Users/Controller/ $ vim IndexController.php
<?php namespace UsersController; use ZendMvcControllerAbstractActionController; use ZendViewModelViewModel; class IndexController extends AbstractActionController { public function indexAction() { $view = new ViewModel(); return $view; } public function registerAction() { $view = new ViewModel(); $view->setTemplate('users/index/new-user'); return $view; } public function loginAction() { $view = new ViewModel(); $view->setTemplate('users/index/login'); return $view; } }
$ cd /var/www/CommunicationApp/module/Users $ mkdir -p view/users/index/
$ cd view/users/index/
<h1>Welcome to Users Module</h1> <a href="/users/index/login">Login</a> | <a
href = "/users/index/register">New User Registration</a>
<h2> Login </h2> <p> This page will hold the content for the login form </p> <a href="/users"><< Back to Home</a>
<h2> New User Registration </h2> <p> This page will hold the content for the registration form </p> <a href="/users"><< Back to Home</a>
We have now created a new controller and views for our new Zend Framework module; the module is still not in a shape to be tested. To make the module fully functional we will need to make changes to the module's configuration, and also enable the module in the application's configuration.
Zend Framework 2.0 module configuration is spread across a series of files which can be found in the skeleton module. Some of the configuration files are described as follows:
In this section will make configuration changes to the Users module to enable it to work with the newly created controller and views using the following steps:
<?php return array();
'controllers' => array( 'invokables' => array( 'UsersControllerIndex' =>
'UsersControllerIndexController', ), ),
'view_manager' => array( 'template_path_stack' => array( 'users' => __DIR__ . '/../view', ), ),
'router' => array( 'routes' => array( 'users' => array( 'type' => 'Literal', 'options' => array( 'route' => '/users', 'defaults' => array( '__NAMESPACE__' => 'UsersController', 'controller' => 'Index', 'action' => 'index', ), ),
<?php return array( 'controllers' => array( 'invokables' => array( 'UsersControllerIndex' => 'UsersControllerIndexController', ), ), 'router' => array( 'routes' => array( 'users' => array( 'type' => 'Literal', 'options' => array( // Change this to something specific to your module 'route' => '/users', 'defaults' => array( //Change this value to reflect the namespace in which // the controllers for your module are found '__NAMESPACE__' => 'UsersController', 'controller' => 'Index', 'action' => 'index', ), ), 'may_terminate' => true, 'child_routes' => array( // This route is a sane default when developing a module; // as you solidify the routes for your module, however, // you may want to remove it and replace it with more // specific routes. 'default' => array( 'type' => 'Segment', 'options' => array( 'route' => '/[:controller[/:action]]', 'constraints' => array( 'controller' => '[a-zA-Z][a-zA-Z0-9_-]*', 'action' => '[a-zA-Z][a-zA-Z0-9_-]*', ), 'defaults' => array( ), ), ), ), ), ), ), 'view_manager' => array( 'template_path_stack' => array( 'users' => __DIR__ . '/../view', ), ), );
'modules' => array( 'Application', 'Users', ),
The module home page is shown as follows:
The registration page is shown as follows:
We have modified the configuration of ZendSkeletonModule to work with the new controller and views created for the Users module. Now we have a fully-functional module up and running using the new ZF module system.
Now that we have the knowledge to create and configure own modules, your next task would be to set up a new CurrentTime module. The requirement for this module is to render the current time and date in the following format:
Time: 14:00:00 GMT Date: 12-Oct-2012
We have now learned about setting up a new Zend Framework project using Zend's skeleton application and module. In our next chapters, we will be focusing on further development on this module and extending it into a fully-fledged application.
Further resources on this subject: