










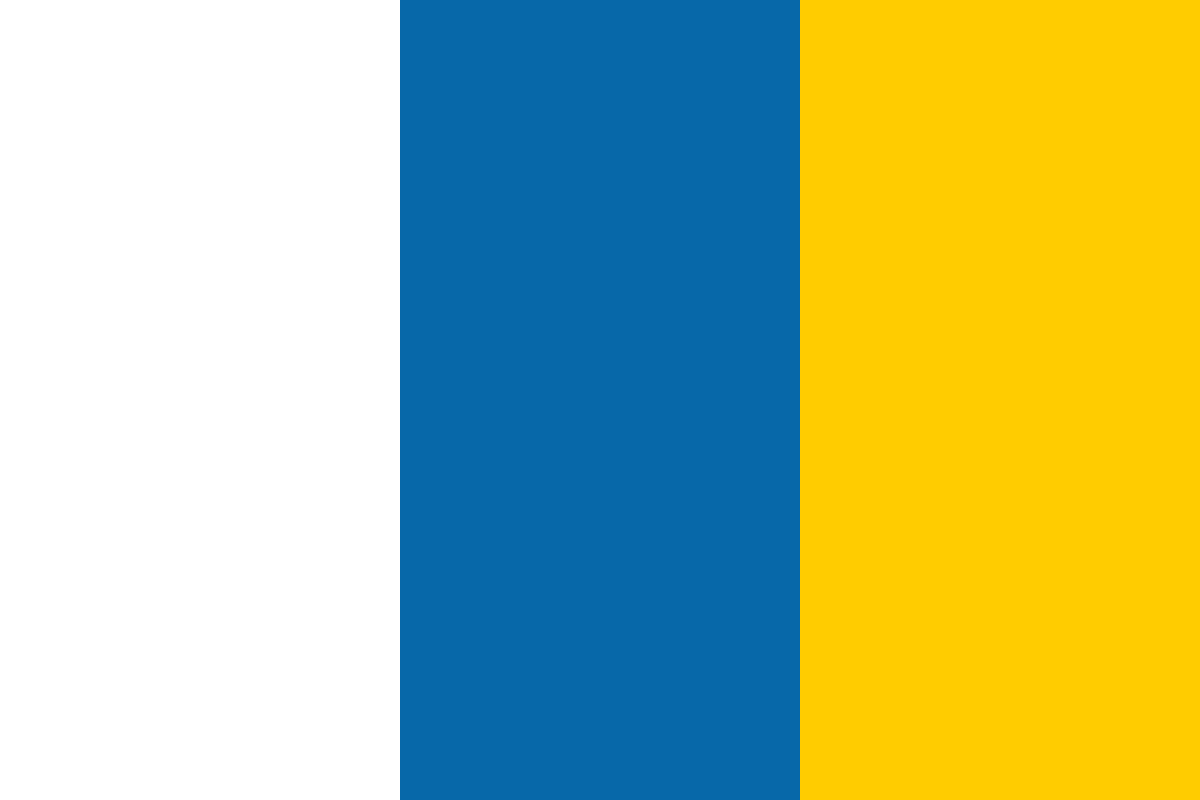

































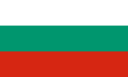








(For more resources related to this topic, see here.)
Please refer to the WinRTCalculator project for the full working code to create a WinRT component and consume it in Javascript.
Perform the following steps to create a WinRT component and consume it in Javascript:
Open Class1.h and add the following method declarations:
double ComputeAddition(double num1, double num2);
double ComputeSubstraction(double num1, double num2);
double ComputeMultiplication(double num1, double num2);
double ComputeDivision(double num1, double num2);
Open Class1.cpp and add the following method implementations:
double Class1::ComputeAddition(double num1, double num2)
{
return num1+num2;
}
double Class1::ComputeSubstraction(double num1, double num2)
{
if(num1>num2)
return num1-num2;
else return num2-num1;
}
double Class1::ComputeMultiplication(double num1, double num2)
{
return num1*num2;
}
double Class1::ComputeDivision(double num1, double num2)
{
if (num2 !=0)
{ return num1/num2; } else return 0; }
Now we need to create a Javascript project where the preceding WinRTCalculator component will be consumed. To create the Javascript project, follow these steps:
Go to Solution | Projects from the left pane of the References Manager dialog box.
Select WinRTCalculator from the center pane and then click on the OK button.
<p>Calculator from javascript</p> <div id="inputDiv"> <br /><br /> <span id="inputNum1Div">Input Number - 1 : </span> <input id="num1" /> <br /><br /> <span id="inputNum2Div">Input Number - 2 : </span> <input id="num2" /> <br /><br /> <p id="status"></p> </div> <br /><br /> <div id="addButtonDiv"> <button id="addButton" onclick= "AdditionButton_Click()">Addition of Two Numbers </button> </div> <div id="addResultDiv"> <p id="addResult"></p> </div> <br /><br /> <div id="subButtonDiv"> <button id= "subButton" onclick="SubsctractionButton_Click()"> Substraction of two numbers</button> </div> <div id="subResultDiv"> <p id="subResult"></p> </div> <br /><br /> <div id="mulButtonDiv"> <button id= "mulButton" onclick="MultiplicationButton_Click()"> Multiplcation of two numbers</button> </div> <div id="mulResultDiv"> <p id="mulResult"></p> </div> <br /><br /> <div id="divButtonDiv"> <button id= "divButton" onclick="DivisionButton_Click()"> Division of two numbers</button> </div> <div id="divResultDiv"> <p id="divResult"></p> </div>
var nativeObject = new WinRTCalculator.Class1(); function AdditionButton_Click() { var num1 = document.getElementById('num1').value; var num2 = document.getElementById('num2').value; if (num1 == '' || num2 == '') { document.getElementById('status').innerHTML = 'Enter input numbers to continue'; } else { var result = nativeObject.computeAddition(num1, num2); document.getElementById('status').innerHTML = ''; document.getElementById('addResult').innerHTML = result; } } function SubsctractionButton_Click() { var num1 = document.getElementById('num1').value; var num2 = document.getElementById('num2').value; if (num1 == '' || num2 == '') { document.getElementById('status').innerHTML = 'Enter input numbers to continue'; } else { var result = nativeObject.computeSubstraction (num1, num2); document.getElementById('status').innerHTML = ''; document.getElementById('subResult').innerHTML = result; } } function MultiplicationButton_Click() { var num1 = document.getElementById('num1').value; var num2 = document.getElementById('num2').value; if (num1 == '' || num2 == '') { document.getElementById('status').innerHTML = 'Enter input numbers to continue'; } else { var result = nativeObject.computeMultiplication (num1, num2); document.getElementById('status').innerHTML = ''; document.getElementById('mulResult').innerHTML = result; } }
The Class1.h and Class1.cpp files have a public ref class. It's an Activatable class that JavaScript can create by using a new expression. JavaScript activates the C++ class Class1 and then calls its methods and the returned values are populated to the HTML Div.
While debugging a JavaScript project that has a reference to a WinRT component DLL, the debugger is set to enable either stepping through the script or through the component native code. To change this setting, right-click on the JavaScript project and go to Properties | Debugging | Debugger Type.
If a C++ Windows Runtime component project is removed from a solution, the corresponding project reference from the JavaScript project must also be removed manually.
In this article, we learned how to reate a WinRT component and call it from JavaScript.
Further resources on this subject: