










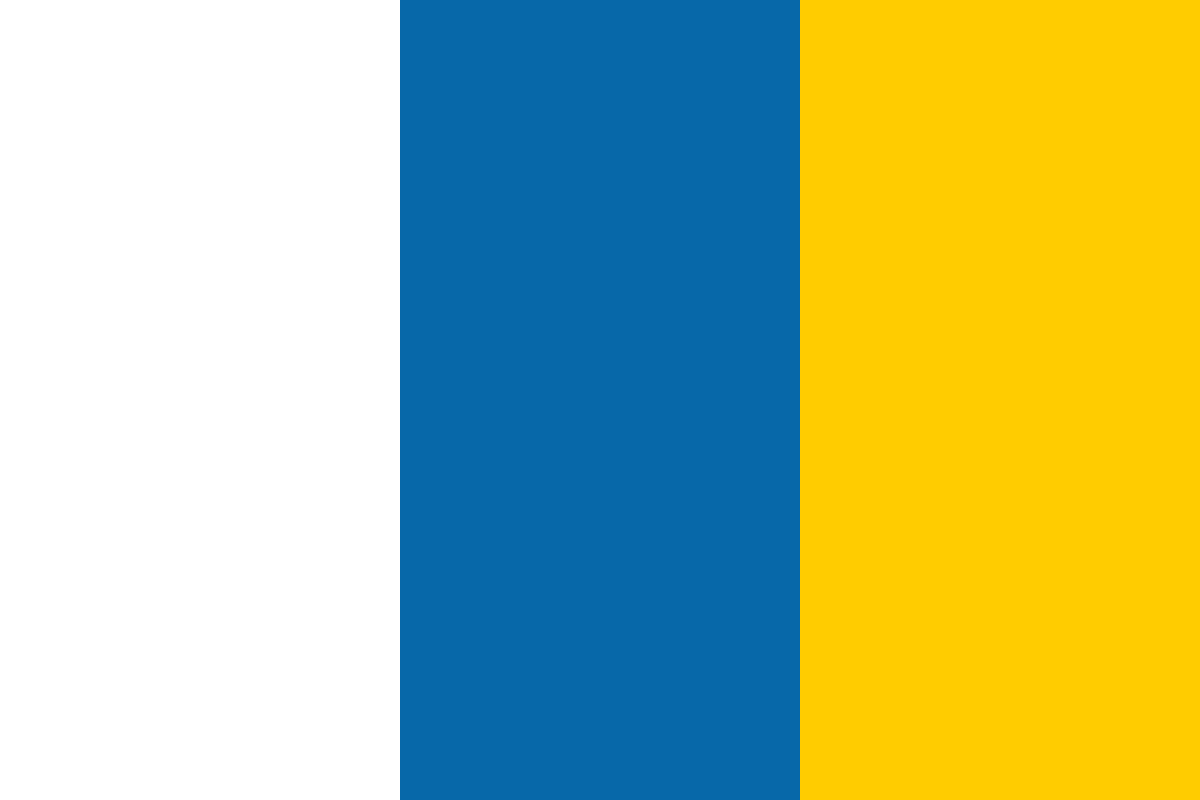

































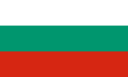








Let's get along.
The application folder will be made up of five files:
Addressbook.css will contain the css for the interface styling, addressbook.html will contain the html source, addressbook.js contains javascript codes, addressbook.php will mostly contain the server side code that will store the contacts to database, delete the contacts, provide updates and fetch the list of the contacts.
Let's look through the HTML
We include the scripts and the css file in the head tag in addressbook.html file.
<title>sample address book</title>
<link rel="stylesheet" type="text/css" href="addressbook.css">
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript" src="addressbook.js"></script>
The code above includes the css for styling the application, jquery library for cross browser javascript and easy DOM access, and the addressbook.js contains functions that help user actions translated via javascript and ajax calls.
The Body tag should contain this:
<div id="Layer1">
<h1>Simple Address Book</h1>
<div id="addContact">
<a href="#add-contact" id="add-contact-btn">Add Contact</a>
<table id="add-contact-form">
<tr>
<td>Names:</td><td><input type="text" name="names" id="names" /></td>
</tr>
<tr>
<td>Phone Number:</td><td><input type="text" name="phone" id="phone" /></td>
</tr>
<tr>
<td> </td><td>
<a href="#save-contact" id="save-contact-btn">Save Contact</a>
<a href="#cancel" id="cancel-btn">Cancel</a>
</td>
</tr>
</table>
</div>
<div id="notice">
notice box
</div>
<div id="list-title">My Contact List</div>
<ul id="contacts-lists">
<li>mambe nanje [+23777545907] - <a
href="#delete-id" class="deletebtn" contactid='1'> delete contact </a></li>
<li>mambe nanje [+23777545907] - <a
href="#delete-id" class="deletebtn" contactid='2'> delete contact</a></li>
<li>mambe nanje [+23777545907] - <a
href="#delete-id" class="deletebtn" contactid='3'> delete contact</a></li>
</ul>
</div>
The above code creates an html form that provides input fields to insert new address book entries. It also displays a button to make it appear via javascript functions. It also creates a notification div and goes to display the contact list with delete button on each entry.
With the above code, the application will now look like this:
/* CSS Document */
body {
background-color: #000000;
}
#Layer1 {
margin:auto;
width:484px;
height:308px;
z-index:1;
}
#add-contact-form{
color:#FF9900;
font-weight:bold;
font-family:Verdana, Arial, Helvetica, sans-serif;
background-color:#333333;
margin-top:5px;
padding:10px;
}
#add-contact-btn{
background-color:#FF9900;
font-weight:bold;
font-family:Verdana, Arial, Helvetica, sans-serif;
border:1px solid #666666;
color:#000;
text-decoration:none;
padding:2px;
font-weight:bold;
}
#save-contact-btn{
background-color:#FF9900;
font-weight:bold;
font-family:Verdana, Arial, Helvetica, sans-serif;
border:1px solid #666666;
color:#000;
text-decoration:none;
padding:2px;
font-weight:bold;
}
#cancel-btn{
background-color:#FF9900;
font-weight:bold;
font-family:Verdana, Arial, Helvetica, sans-serif;
border:1px solid #666666;
color:#000;
text-decoration:none;
padding:2px;
font-weight:bold;
}
h1{
color:#FFFFFF;
font-family:Arial, Helvetica, sans-serif;
}
#list-title{
color:#FFFFFF;
font-weight:bold;
font-size:14px;
font-family:Arial, Helvetica, sans-serif;
margin-top:10px;
}
#contacts-lists{
color:#FF6600;
font-weight:bold;
font-family:Verdana, Arial, Helvetica, sans-serif;
font-size:12px;
}
#contacts-lists a{
background-color:#FF9900;
text-decoration:none;
padding:2px;
color:#000;
margin-bottom:2px;
}
#contacts-lists li{
list-style:none;
border-bottom:1px dashed #666666;
margin-bottom:10px;
padding-bottom:5px;
}
#notice{
width:400px;
margin:auto;
background-color:#FFFF99;
border:1px solid #FFCC99;
font-weight:bold;
font-family:verdana;
margin-top:10px;
padding:4px;
}
The CSS code styles the HTML above and it ends up looking like this:
Now that we have our html and css perfectly working, we need to setup the database and the PHP server codes that will handle the AJAX requests from the jquery functions.
Create a MySQL database and executing the following SQL code will create the contacts table. This is the only table this application needs.
CREATE TABLE `contacts` (
`id` INT NOT NULL AUTO_INCREMENT PRIMARY KEY ,
`names` VARCHAR( 200 ) NOT NULL ,
`phone` VARCHAR( 100 ) NOT NULL
);
Let's analyse the php codes. Remember, this code will be located in addressbook.php.
The database connection code
# FileName="Connection_php_mysql.htm"
# Type="MYSQL"
# HTTP="true"
//configure the database paramaters
$hostname_packpub_addressbook = "YOUR-DATABASE-HOST";
$database_packpub_addressbook = "YOUR-DATABASE-NAME";
$username_packpub_addressbook = "YOUR-DATABASE-USERNAME";
$password_packpub_addressbook = "YOUR-DATABASE-PASSWORD";
//connect to the database server
$packpub_addressbook = mysql_pconnect($hostname_packpub_addressbook, $username_packpub_addressbook,
$password_packpub_addressbook) or trigger_error(mysql_error(),E_USER_ERROR);
//selete the database
mysql_select_db($database_packpub_addressbook);
the above code sets the parameters required for the database connection, then establishes a connection to the server and selects your database.
The PHP codes will then contain functions SAVECONTACTS, DELETECONTACTS, GETCONTACTS. These functions will do exactly as their name implies. Save the contact from AJAX call to the database, delete contact via AJAX request or get the contacts. The functions are as show below:
//function to save new contact
/**
* @param <string> $name //name of the contact
* @param <string> $phone //the telephone number of the contact
*/
function saveContact($name,$phone){
$sql="INSERT INTO contacts (names , phone ) VALUES ('".$name."','".$phone."');";
$result=mysql_query($sql)or die(mysql_error());
}
//lets write a function to delete contact
/**
* @param <int> id //the contact id in database we wish to delete
*/
function deleteContact($id){
$sql="DELETE FROM contacts where id=".$id;
$result=mysql_query($sql);
}
//lets get all the contacts
function getContacts(){
//execute the sql to get all the contacts in db
$sql="SELECT * FROM contacts";
$result=mysql_query($sql);
//store the contacts in an array of objects
$contacts=array();
while($record=mysql_fetch_object($result)){
array_push($contacts,$record);
}
//return the contacts
return $contacts;
}
The codes above creates the functions but the functions are not called till the following code executes:
//lets handle the Ajax calls now
$action=$_POST['action'];
//the action for now is either add or delete
if($action=="add"){
//get the post variables for the new contact
$name=$_POST['name'];
$phone=$_POST['phone'];
//save the new contact
saveContact($name,$phone);
$output['msg']=$name." has been saved successfully";
//reload the contacts
$output['contacts']=getContacts();
echo json_encode($output);
}else if($action=="delete"){
//collect the id we wish to delete
$id=$_POST['id'];
//delete the contact with that id
deleteContact($id);
$output['msg']="one entry has been deleted successfully";
//reload the contacts
$output['contacts']=getContacts();
echo json_encode($output);
}else{
$output['contacts']=getContacts();
$output['msg']="list of all contacts";
echo json_encode($output);
}
The above code is the heart of the addressbook.php codes. It gets the action from post variables sent via AJAX call in addressbook.js file, interprets the action and executes the appropriate function for either add, delete or nothing which will just get the list of contacts. json_encode() function is used to encode the data in to Javascript Object Notation format; it will be easily interpreted by the javascript codes.