










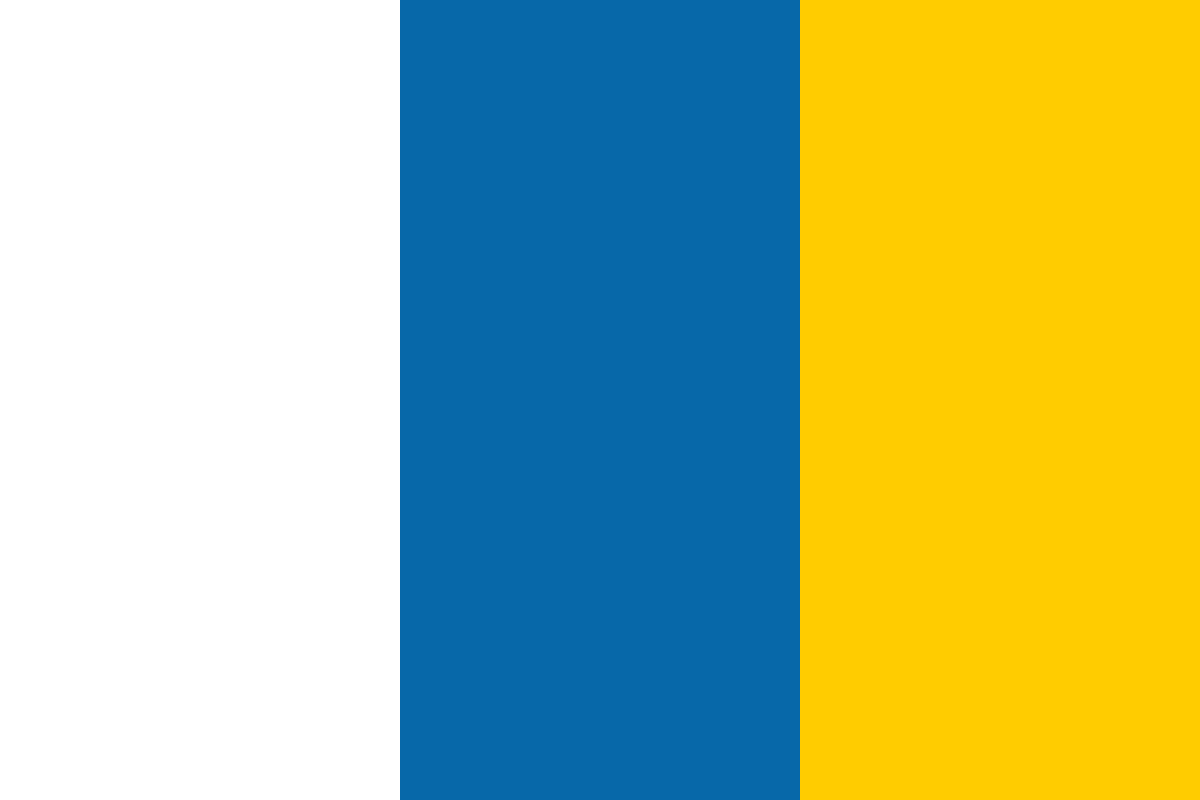

































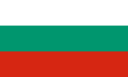








This article by Yogesh Dhanapal and Jayakrishnan Vijayaraghavan, authors of the book ArcGIS for JavaScript developers by Example, will develop a custom widget.
(For more resources related to this topic, see here.)
Let's create a custom widget in the app, which will do the following:
Let's list the modules required to define class and their corresponding intended callback function decoration
The modules for Class declaration and OOPS are illustrated in the following table:
Modules |
Callback functions |
dojo/_base/declare |
declare |
dijit/_WidgetBase |
_WidgetBase |
dojo/_base/lang |
lang |
The modules for using HTML templates are illustrated in the following table:
Modules |
Callback functions |
dijit/_TemplatedMixin |
_TemplatedMixin |
dojo/text! |
dijitTemplate |
The modules for using Event is illustrated in the following table:
Modules |
Callback functions |
dojo/on |
on |
dijit/a11yclick |
a11yclick |
The modules for manipulating dom elements and their style are illustrated in the following table:
Modules |
Callback functions |
dojo/dom-style |
domStyle |
dojo/dom-class |
domClass |
dojo/domReady! |
- |
Modules |
Callback functions |
esri/toolbars/draw |
Draw |
esri/symbols/SimpleFillSymbol |
SimpleFillSymbol |
esri/symbols/SimpleLineSymbol |
SimpleLineSymbol |
esri/graphic |
Graphic |
dojo/_base/Color |
Color |
Modules |
Callback functions |
esri/tasks/query |
Query |
esri/tasks/QueryTask |
QueryTask |
Module |
Callback functions |
dojo/i18n! |
nls |
Draw toolbar enables us to draw graphics on the map. Draw toolbar has events associated with it. When a draw operation is completed, it returns the object drawn on the map as geometry. Perform the following steps to create a graphic using the draw toolbar:
The draw toolbar is provided by the module esri/toolbars/draw. The draw toolbar accepts the map object as an argument. Instantiate the draw toolbar within the postCreate function. The draw toolbar also accepts an additional optional argument named options. One of the properties in the options object is named showTooltips. This can be set to true so that we can see a tooltip associated while drawing. The text in the tooltip can be customized. Else, a default tooltip associated with draw geometry is displayed:
return declare([_WidgetBase, _TemplatedMixin], {
//assigning html template to template string
templateString: dijitTemplate,
isDrawActive: false,
map: null,
tbDraw: null,
constructor: function (options, srcRefNode) {
this.map = options.map;
},
startup: function () {},
postCreate: function () {
this.inherited(arguments);
this.tbDraw = new Draw(this.map, {showTooltips : true});
}
The Draw toolbar can be activated on the click event or touch event (in case of smartphones or tablets) of a button, which is intended to indicate the start of a draw event. Dojo provides a module that takes care of touch as well as click events. The module is named dijit/a11yclick.
To activate the draw toolbar, we need to provide the type of symbol to draw. The draw toolbar provides a list of constants, which corresponds to the type of draw symbol. These constants are POINT, POLYGON, LINE, POLYLINE, FREEHAND_POLYGON, FREEHAND_POLYLINE, MULTI_POINT, RECTANGLE, TRIANGLE, CIRCLE, ELLIPSE, ARROW, UP_ARROW, DOWN_ARROW, LEFT_ARROW, and RIGHT_ARROW.
While activating the draw toolbar, these constants must be used to define the type of Draw operation required. Our objective is to draw a polygon on the click of a draw button. The code is shown in the following screenshot:
Once the draw tool bar is activated, the draw operation will begin. For point geometry, the draw operation is just a single click. For a polyline and a polygon, the single click adds a vertex to the polyline and a double-click ends the sketch. For freehand polyline or polygon, the click-and-drag operation draws the geometry and a mouse-up operation ends the drawing.
When the draw operation is complete, we need an event handler to do something with the shape that was drawn by the draw toolbar. The API provides a draw-end event, which is fired once the draw operation is complete. This event handler must be connected to the draw toolbar. This event handler shall be defined within the this.own() function inside the postCreate() method of the widget. The event result can be passed to a named function or an anonymous function:
postCreate: function () {
...
this.tbDraw.on("draw-end", lang.hitch(this,
this.querybyGeometry));
},
...
querybyGeometry: function (evt) {
this.isBusy(true);
//Get the Drawn geometry
var geometryInput = evt.geometry;
...
}
In the draw-end event call back function, we will get the geometry of the drawn shape as the result object. To add this geometry back to the map, we need to symbolize it. A symbol is associated with the geometry it symbolizes. Also, the styling of the symbol is defined by the colors or picture used to fill up the symbol and the size of the symbol. Just to symbolize a polygon, we need to use the SimpleFillSymbol and the SimpleLineSymbol modules. We may also need the esri/color module to define the fill colors.
Let's review a snippet to understand this better. This is a simple snippet to construct a symbol for a polygon with semitransparent solid red color fill and a yellow dash-dot line.
In the preceding snippet, SimpleFillSymbol.STYLE_SOLID and SimpleLineSymbol.STYLE_DASHDOT are the constants provided by the SimpleFIllSymbol and the SimpleLineSymbol modules, respectively. These constants are used for styling the polygon and the line.
Two colors are defined in the construction of the symbol—one for filling up the polygon and the other for coloring the outline. A color can be defined by four components. They are as follows:
Red, Green, and Blue components take values from 0 to 255 and the Opacity takes values from 0 to 1. A combination of Red, Green, and Blue components can be used to produce any color according to the RGB color theory. So, to create a yellow color, we are using the maximum of Red component (255) and the maximum of Green Component (255); we don't want the Blue component to contribute to our color, so we will use 0. An Opacity value of 0 means 100% transparency and an opacity value of 1 means 100% opaqueness. We have used 0.2 for the fill color. This means that we need our polygon to be 20% opaque or 80% transparent. The default value for this component is 1.
Symbol is just a generic object. It means that any polygon geometry can use the symbol to render itself. Now, we need a container object to display the drawn geometry with the previously defined symbol on the map. A Graphic object provided by the esri/Graphic module acts as a container object, which can accept a geometry and a symbol. The graphic object can be added to the map's graphic layer.
A graphic layer is always present in the map object, which can be accessed by using the graphics property of the map (this.map.graphics).
In this article, we learned how to create classes and customized widget and its required modules, and how to use a draw toolbar.
Further resources on this subject: