










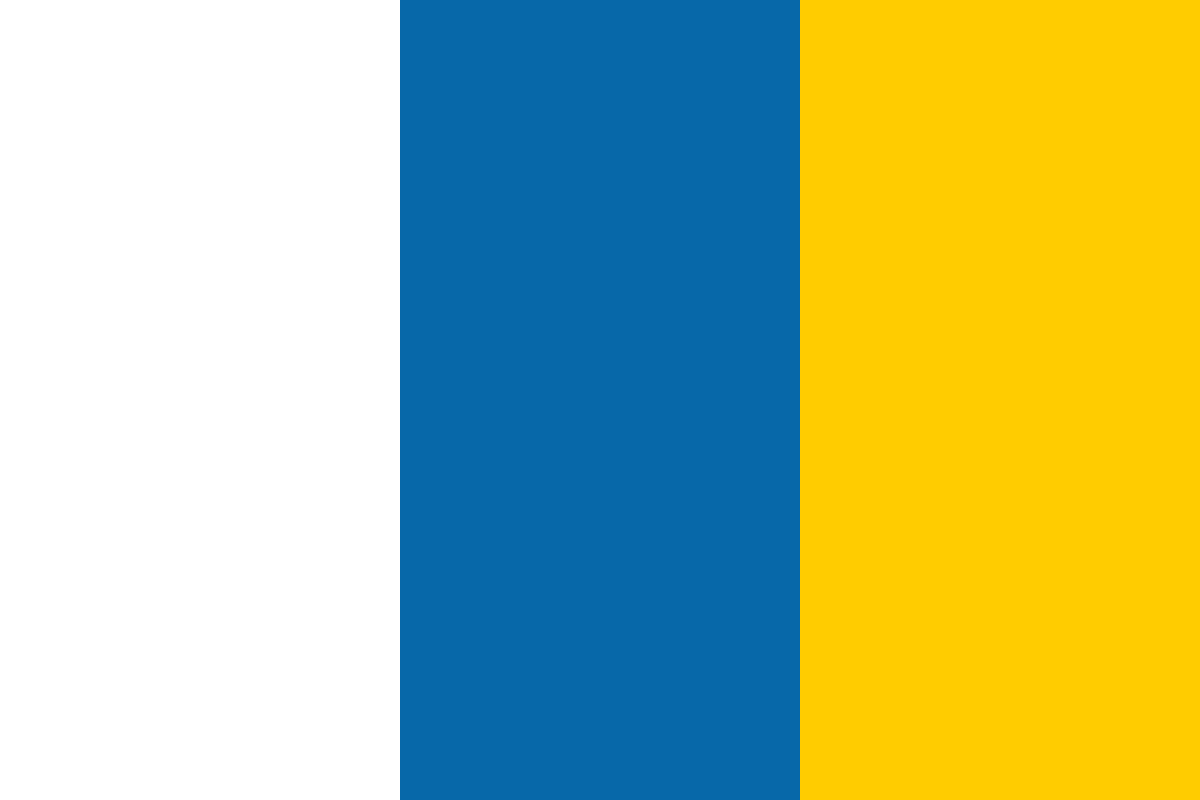

































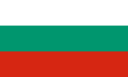








In the 3rd part of this article series, we learnt about:
All those things, we learnt in the third part of the article series were a big improvement for our Twitter client, but wouldn’t it be cool if you could click on the URL links from your friends’ time line and then a web browser window would open automatically to show you the related webpage? Well, after reading this part of the article series, you’ll be able to integrate this functionality into your own Twitter client among other things.
Here are the links to the earlier articles of this article series:
Read Build your own Application to access Twitter using Java and NetBeans: Part 1
Read Build your own Application to access Twitter using Java and NetBeans: Part 2
Read Build your own Application to access Twitter using Java and NetBeans: Part 3
Till now, we’ve been working with JPanel objects to show your Twitter information inside the JTabbedPane component. But as you can see from your friends’ tweets, the URL links that show up aren’t clickable. And how can we make them clickable? Well, fortunately for us there’s a Swing component called JEditorPane that will let us use HTML markup, so the URL hyperlinks will show up as if you were on a web page. Cool, huh? Now let’s start with the dirty job…
String paneContent = new String();
statusPane = new JEditorPane();
statusPane.setContentType("text/html");
statusPane.setEditable(false);
statusPane.setText(paneContent);
jTabbedPane1.add("Friends - Enhanced", statusPane);
JScrollPane editorScrollPane = new JScrollPane(statusPane,
JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED, // vertical bar policy
JScrollPane.HORIZONTAL_SCROLLBAR_NEVER ); // horizontal bar policy
jTabbedPane1.add("Friends - Enhanced", editorScrollPane);
Let’s stop for a while to review our progress so far. In the first step above the exercise, you added an import declaration to tell the Java compiler that we need to use an object from the JEditorPane class. In step 3, you added a JEditorPane object called statusPane to your application. This object acts as a container for your friends’ tweets.
And in case you’re wondering why we didn’t use a regular JPanel object, just remember that we want to make the URL links in your friends’ tweets clickable, so when you click on one of them, a web browser window will pop up to show you the web page associated to that hyperlink.
Now let’s get back to our exercise. In step 4, you added four lines to your application’s code. The first line:
String paneContent = new String();
creates a String variable called paneContent to store the username and text of each individual tweet from your friends’ timeline. The next three lines:
statusPane = new JEditorPane();
statusPane.setContentType("text/html");
statusPane.setEditable(false);
create a JEditorPane object called statusPane, set its content type to text/html so we can include HTML markup and make the statusPane non-editable, so nothing gets messed up when showing your friends’ timeline.
Now that we have the statusPane ready to roll, we need to fill it up with the information related to each individual tweet from your friends. That’s why we need the paneContent variable. In step 6, you inserted the following line:
paneContent = paneContent + statusUser.getText() + "<br>" + statusText.getText() + "<hr>";
inside the for block to add the username and the text of each individual tweet to the paneContent variable. The <br> HTML tag inserts a line break so the username appears in one line and the text of each tweet appears in another line. The <hr> HTML tag inserts a horizontal line to separate one tweet from the other.
Once the for loop ends, we need to add the information from the paneContent variable to the JEditorPane object called statusPane. That’s why in step 7, you added the following line:
statusPane.setText(paneContent);
and then the
jTabbedPane1.add("Friends - Enhanced", statusPane);
line creates a new tab in the jTabbedPane1 component and adds the statusPane component to it, so you can see the friends timeline with HTML markup.
In step 10, you learned how to create a JScrollPane object called editorScrollPane to add scrollbars to your statusPane component and integrate them into the jTabbedPane1 container. In this example, the JScrollPane constructor requires arguments: the statusPane component, the vertical scrollbar policy and the horizontal scrollbar policy. There are three options you can choose for your vertical and horizontal scrollbars: show them as needed, never show them or always show them.
In this specific case, we need the vertical scrollbar to show up as needed, in case the list of your friends’ tweets doesn’t fit the screen, so we use the JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED policy. And since we don’t need the horizontal bar to show up because the statusPane component can adjust its horizontal size to fit your application’s window, we use the JScrollPane.HORIZONTAL_SCROLLBAR_NEVER policy.
The last line of code from step 10 adds the editorScrollPane component to the jTabbedPane1 container instead of adding the statusPane component directly, because now the JEditorPane component is contained within the JScrollPane component.
Now let’s see how to convert the URL links to real hyperlinks.