










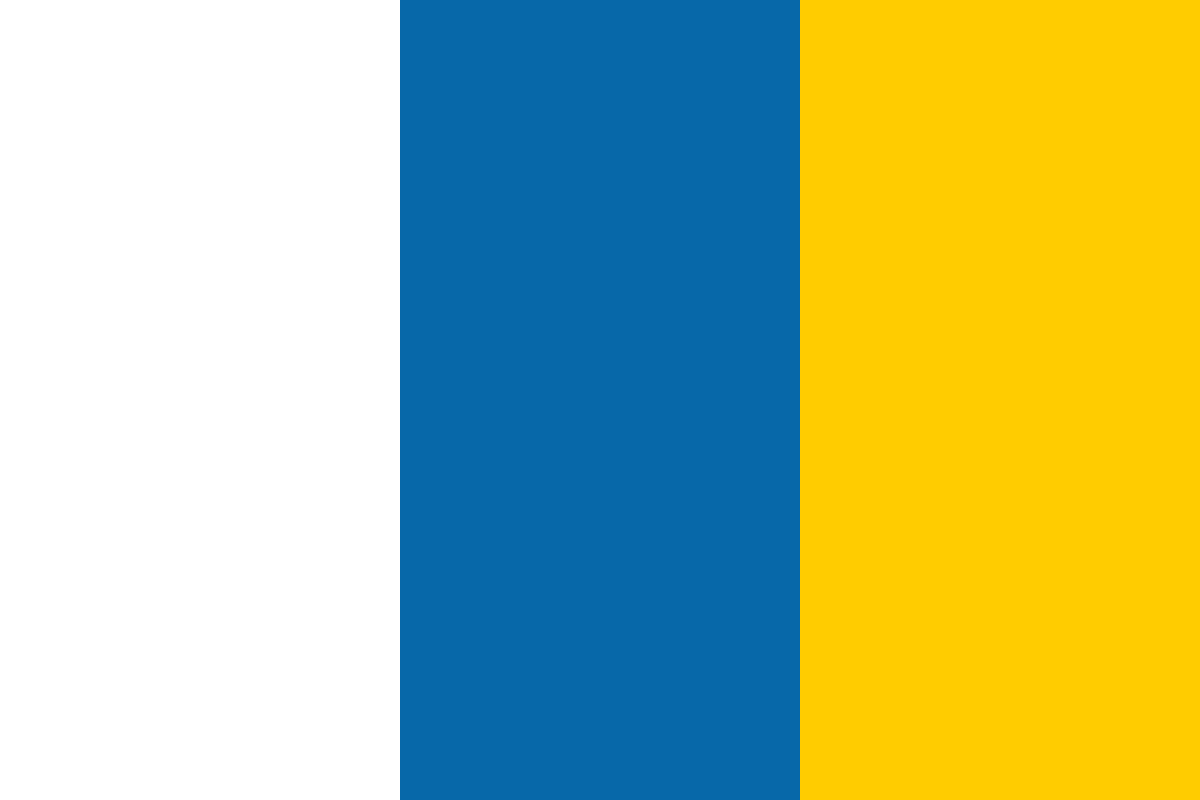

































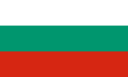








In this tutorial, we’ll develop the simple Java application further to add some more functions. Now that we can connect to our Twitter account via the Twitter4J API, it would be nice to use a login dialog instead of hard-coding our Twitter username and password in the Java application.
But before we start to build our enhanced SwingAndTweet application, let me show you how it will look like once we finish all the exercises in this part of the tutorial:
And now, let the show begin…
Basically, in the previous exercise we added all the Swing controls you’re going to need to type your username and password, so you can connect to your Twitter account. The twitterLogin dialog is going to take care of the login process for your SwingAndTweet application. We replaced the default names for the JTextField, the JPasswordField and the two JButton controls because it will be easier to identify them during the coding process of the application. On step 8 we used the Properties window of the twitterLogin dialog to change the title property and give your dialog a decent title. We also enabled the modal property on step 9, so you can’t just close the dialog and jump right to the SwingAndTweetUI main window; you’ll have to enter a valid Twitter username and password combination for that.
Ok, now we have a good-looking dialog called twitterLogin. The next step is to invoke it before our main SwingAndTweet JFrame component shows up, so we need to insert some code inside the SwingAndTweetUI() constructor method.
int loginWidth = twitterLogin.getPreferredSize().width;
int loginHeight = twitterLogin.getPreferredSize().height;
twitterLogin.setBounds(0,0,loginWidth,loginHeight);
twitterLogin.setVisible(true);
Now let’s take a look at the code we added to your twitterLogin dialog. On the first line,
int loginWidth = twitterLogin.getPreferredSize().width;
we declare an integer variable named loginWidth, and assign to it the preferred width of the twitterLogin dialog. The getPreferredSize method retrieves the value of the preferredSize property from the twitterLogin dialog through the .width field.
On the second line,
int loginHeight = twitterLogin.getPreferredSize().height;
we declare another integer variable named loginHeight, and assign to it the preferred height of the twitterLogin dialog. This time, the getPreferredSize() method retrieves the value of the preferredWidth property from the twitterLogin dialog through the .height field.
On the next line,
twitterLogin.setBounds(0,0,loginWidth,loginHeight);
we use the setBounds method to set the x,y coordinates where the dialog should appear on the screen, along with its corresponding width and height. The first two parameters are for the x,y coordinates; in this case, x=0 and y=0, which means the twitterLogin dialog will show up at the upper-left part of the screen. The last two parameters receive the value of the loginWidth and loginHeight variables to establish the twitterLogin dialog’s width and height, respectively.
The last line,
twitterLogin.setVisible(true);
makes the twitterLogin dialog appear on the screen. And since the modal property of this dialog is enabled, once it shows up on the screen it won’t let you do anything else with your SwingAndTweet1 application until you close it up or enter a valid Twitter username and password, as we’ll see in the next exercise.
Now your twitterLogin dialog is ready to roll! Basically, it won’t let you go to the SwingAndTweet main window until you’ve entered a valid Twitter username and password. And for doing that, we’re going to use the same login code from Build your own Application to access Twitter using Java and NetBeans: Part 1 of this article series.
Twitter twitter;
try { twitter = new Twitter(txtUsername.getText(), String.valueOf(txtPassword.getPassword())); twitter.verifyCredentials(); JOptionPane.showMessageDialog(null, "You're logged in!"); twitterLogin.dispose(); } catch (TwitterException e) { JOptionPane.showMessageDialog (null, "Login failed"); }
And now, let’s examine what we just accomplished. First you added the
Twitter twitter;
line to your application code. With this line we’re declaring a Twitter object named twitter, and it will be available throughout all the application code.
On step 4, you added some lines of code to the btnLoginActionPerformed method; this code will be executed every time you click on the Login button from the twitterLogin dialog. All the code is enclosed in a try block, so that if an error occurs during the login process, a TwitterException will be thrown and the code inside the catch block will execute.
The first line inside the try block is
twitter = new Twitter(txtUsername.getText(),String.valueOf(txtPassword.getPassword()));
This code creates the twitter object that we’re going to use throughout the application. It uses the text value you entered in the txtUsername and txtPassword fields to log into your Twitter account. The next line,
twitter.verifyCredentials();
checks to see if the username and password provided to the twitter object are correct; if that’s true, a message dialog box shows up in the screen with the You’re logged in! message and the rest of the code executes once you click on the OK button of this message dialog; otherwise, the code in the catch block executes and a message dialog shows up in the screen with the Login failed message, and the twitterLogin dialog keeps waiting for you to type a correct username and password combination.
The next line in the sequence,
JOptionPane.showMessageDialog(null, "You're logged in!");
shows the message dialog that we talked about before, and the last line inside the try block,
twitterLogin.dispose();
makes the twitterLogin dialog disappear from the screen once you’ve logged into your Twitter account successfully.
The only line of code inside the catch block is
JOptionPane.showMessageDialog (null, "Login failed");
This line executes when there’s an error in the Twitter login process; it shows the Login failed message in the screen and waits for you to press the OK button.
On step 9 we added one line of code to the btnExitActionPerformed method:
System.exit(0);
This line closes your SwingAndTweet application whenever you click on the Exit button.
Finally, on steps 10-12 we added another System.exit(0); line to the twitterLoginWindowClosing method, to close your SwingAndTweet application whenever you click on the Close(X) button of the twitterLogin dialog.
Now let’s see some real Twitter action! The following exercise will show you how to show your most recent tweets inside a text area.
private void btnUpdateStatusActionPerformed(java.awt.event.ActionEvent evt) { try { if (txtUpdateStatus.getText().isEmpty()) JOptionPane.showMessageDialog (null, "You must write something!"); else { twitter.updateStatus(txtUpdateStatus.getText()); jTextArea1.setText(null); java.util.List<Status> statusList = twitter.getUserTimeline(); for (int i=0; i<statusList.size(); i++) { jTextArea1.append(String.valueOf(statusList.get(i).getText())+"n"); jTextArea1.append("-----------------------------n"); } } } catch (TwitterException e) { JOptionPane.showMessageDialog (null, "A Twitter Error ocurred!"); } txtUpdateStatus.setText(""); jTextArea1.updateUI();
private void btnLoginActionPerformed(java.awt.event.ActionEvent evt) { try { twitter = new Twitter(txtUsername.getText(), String.valueOf(txtPassword.getPassword())); twitter.verifyCredentials(); // JOptionPane.showMessageDialog(null, "You're logged in!");
java.util.List<Status> statusList = twitter.getUserTimeline();
for (int i=0; i<statusList.size(); i++) {
jTextArea1.append(String.valueOf(statusList.get(i).getText())+"n");
jTextArea1.append("-----------------------------n");
}
twitterLogin.dispose();
} catch (TwitterException e) {
JOptionPane.showMessageDialog (null, "Login failed");
}
jTextArea1.updateUI();
}
That was cool, right? Now you have a much better-looking Twitter client! And you can update your status, too! Let’s examine the code we added in this last exercise…
private void btnUpdateStatusActionPerformed(java.awt.event.ActionEvent evt) { try { if (txtUpdateStatus.getText().isEmpty()) JOptionPane.showMessageDialog (null, "You must write something!"); else { twitter.updateStatus(txtUpdateStatus.getText()); jTextArea1.setText(null); java.util.List<Status> statusList = twitter.getUserTimeline(); for (int i=0; i<statusList.size(); i++) { jTextArea1.append(String.valueOf(statusList.get(i).getText())+"n"); jTextArea1.append("-----------------------------n"); } } } catch (TwitterException e) { JOptionPane.showMessageDialog (null, "A Twitter Error ocurred!"); } txtUpdateStatus.setText(""); jTextArea1.updateUI();
On step 7 we added some code to the btnUpdateStatusActionPerformed method. This code will execute whenever you click on the Update button to update your Twitter status. First, let’s look at the code inside the try block. The first two lines,
if (txtUpdateStatus.getText().isEmpty()) JOptionPane.showMessageDialog (null, "You must write something!");
are the first part of a simple if-else statement that checks to see if you’ve written something inside the txtUpdateStatus text field; if it’s empty, a message dialog will show the You must write something! message on the screen, and then it will wait for you to click on the OK button. If the txtUpdateStatus text field is not empty, the code inside the else block will execute instead of showing up the message dialog.
The next part of the code is the else block. The first line inside this block,
twitter.updateStatus(txtUpdateStatus.getText());
updates your twitter status with the text you wrote in the txtUpdateStatus text field; if an error occurs at this point, a TwitterException is thrown and the program execution will jump to the catch block. If your Twitter status was updated correctly, the next line to execute is
jTextArea1.setText(null);
This line erases all the information inside the jTextArea1 control. And the next line,
java.util.List<Status> statusList = twitter.getUserTimeline();
gets the 20 most recent tweets from your timeline and assigns them to the statusList variable. The next line is the beginning of a for statement:
for (int i=0; i<statusList.size(); i++) {
Basically, what this for block does is iterate through all the 20 most recent tweets in your timeline, one at a time, executing the two statements inside this block on each iteration:
jTextArea1.append(String.valueOf(statusList.get(i).getText())+"n"); jTextArea1.append("-----------------------------n");
Although the getUserTimeline() function retrieves the 20 most recent tweets, we need to use the statusList.size() statement as the loop continuation condition inside the for block to get the real number of tweets obtained, because they can be less than 20, and we can’t iterate through something that maybe doesn’t exist, right?
The first line appends the text of each individual tweet to the jTextArea1 control, along with a new-line character ("n") so each tweet is shown in one individual line, and the second line appends the "-----------------------------n" text as a separator between each individual tweet, along with a new-line character. The final result is a list of the 20 most recent tweets inside the jTextArea1 control.
The only line of code inside the catch block displays the A Twitter Error occurred! message in case something goes wrong when trying to update your Twitter status. The next line of code right after the catch block is
txtUpdateStatus.setText("");
This line just clears the content inside the txtUpdateStatus control, so you don’t accidentally insert the same message two times in a row.
And finally, the last line of code in the btnUpdateStatusActionPerformed method is
jTextArea1.updateUI();
This line updates the jTextArea1 control, so you can see the list of your 20 most recent tweets after updating your status.
private void btnLoginActionPerformed(java.awt.event.ActionEvent evt) { try { twitter = new Twitter(txtUsername.getText(), String.valueOf(txtPassword.getPassword())); twitter.verifyCredentials(); // JOptionPane.showMessageDialog(null, "You're logged in!");
java.util.List<Status> statusList = twitter.getUserTimeline();
for (int i=0; i<statusList.size(); i++) {
jTextArea1.append(String.valueOf(statusList.get(i).getText())+"n");
jTextArea1.append("-----------------------------n");
}
twitterLogin.dispose();
} catch (TwitterException e) {
JOptionPane.showMessageDialog (null, "Login failed");
}
jTextArea1.updateUI();
And now let’s have a look at the code we added inside the btnLoginActionPerformed method. The first thing you’ll notice is that we’ve added the '//' characters to the
// JOptionPane.showMessageDialog(null, "You're logged in!");
line; this means it’s commented out and it won’t be executed, because it’s safe to go directly to the SwingAndTweet main window right after typing your Twitter username and password. The next lines are identical to the ones inside the btnUpdateStatusActionPerformed method we saw before; the first line retrieves your 20 most recent tweets, and the for block displays the list of tweets inside the jTextArea1 control. And the last line of code,
jTextArea1.updateUI();
updates the jTextArea1 control so you can see the most recent information regarding your latest tweets.
Well, now your SwingAndTweet application looks better, don’t you think so? In this article, we enhanced the SwingAndTweet application which we build in the first part of the tutorials series. In short, we: