










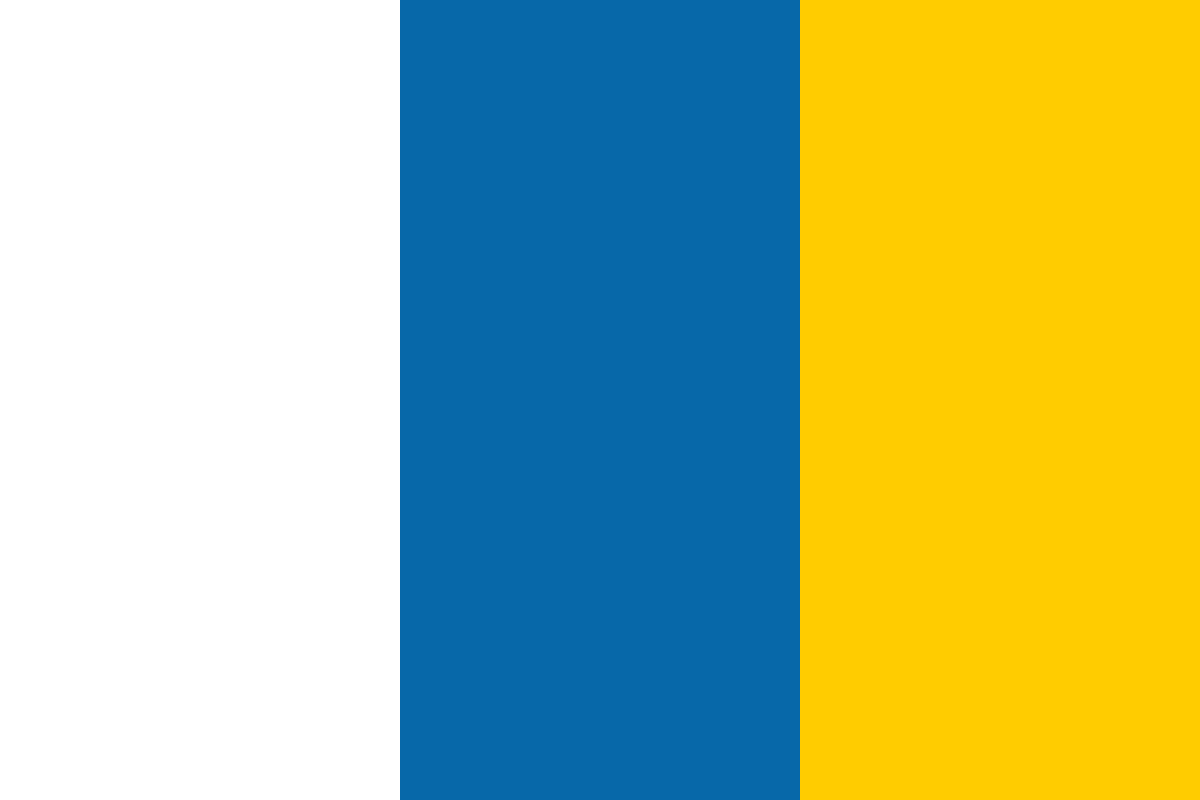

































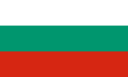








In this article by Fernando Monteiro author of the book Node.JS 6.x Blueprints we will understand some basic concepts of a Node.js application using a relational database (Mysql) and also try to look at some differences between Object Document Mapper (ODM) from MongoDB and Object Relational Mapper (ORM) used by Sequelize and Mysql.
For this we will create a simple application and use the resources we have available as sequelize is a powerful middleware for creation of models and mapping database.
We will also use another engine template called Swig and demonstrate how we can add the template engine manually.
(For more resources related to this topic, see here.)
The first step is to create another directory, I'll use the root folder.
Create a folder called chapter-02.
Open your terminal/shell on this folder and type the express command:
express –-git
Note that we are using only the –-git flag this time, we will use another template engine but we will install it manually.
The first step to do is change the default express template engine to use Swig, a pretty simple template engine very flexible and stable, also offers us a syntax very similar to Angular which is denoting expressions just by using double curly brackets {{ variableName }}.
More information about Swig can be found on the official website at: http://paularmstrong.github.io/swig/docs/
Open the package.json file and replace the jade line for the following:
"swig": "^1.4.2"
Open your terminal/shell on project folder and type:
npm install
Before we proceed let's make some adjust to app.js, we need to add the swig module.
Open app.js and add the following code, right after the var bodyParser = require('body-parser'); line:
var swig = require('swig');
Replace the default jade template engine line for the following code:
var swig = new swig.Swig();
app.engine('html', swig.renderFile);
app.set('view engine', 'html');
Let's change the views folder to the following new structure:
views
pages/
partials/
Remove the default jade files form views.
Create a file called layout.html inside pages folder and place the following code:
<!DOCTYPE html>
<html>
<head>
</head>
<body>
{% block content %}
{% endblock %}
</body>
</html>
Create a index.html inside the views/pages folder and place the following code:
{% extends 'layout.html' %}
{% block title %}{% endblock %}
{% block content %}
<h1>{{ title }}</h1>
Welcome to {{ title }}
{% endblock %}
Create a error.html page inside the views/pages folder and place the following code:
{% extends 'layout.html' %}
{% block title %}{% endblock %}
{% block content %}
<div class="container">
<h1>{{ message }}</h1>
<h2>{{ error.status }}</h2>
<pre>{{ error.stack }}</pre>
</div>
{% endblock %}
We need to adjust the views path on app.js, replace the code on line 14 for the following code:
// view engine setup
app.set('views', path.join(__dirname, 'views/pages'));
At this time we completed the first step to start our MVC application. In this example we will use the MVC pattern in its full meaning, Model, View, Controller.
Create a folder called controllers inside the root project folder.
Create a index.js inside the controllers folder and place the following code:
// Index controller
exports.show = function(req, res) {
// Show index content
res.render('index', {
title: 'Express'
});
};
Edit the app.js file and replace the original index route app.use('/', routes); with the following code:
app.get('/', index.show);
Add the controller path to app.js on line 9, replace the original code, with the following code:
// Inject index controller
var index = require('./controllers/index');
Now it's time to get if all goes as expected, we run the application and check the result.
Type on your terminal/shell the following command:
npm start
Check with the following URL: http://localhost:3000, you'll see the welcome message of express framework.
Remove the routes folder and its content.
Remove the user route from the app.js, after the index controller and on line 31.
Inside views/partials create a new file called head.html and place the following code:
<meta charset="utf-8">
<title>{{ title }}</title>
<link rel='stylesheet' href='https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-alpha.2/css/bootstrap.min.css'>
<link rel="stylesheet" href="/stylesheets/style.css">
Inside views/partials create a file called footer.html and place the following code:
<script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/2.2.1/jquery.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-alpha.2/js/bootstrap.min.js'></script>
Now is time to add the partials file to layout.html page using the include tag.
Open layout.html and add the following highlighted code:
<!DOCTYPE html>
<html>
<head>
{% include "../partials/head.html" %}
</head>
<body>
{% block content %}
{% endblock %}
{% include "../partials/footer.html" %}
</body>
</html>
Finally we are prepared to continue with our project, this time our directories structure looks like the following image:
Folder structure
In this article, we are discussing the basic concept of Node.js and Mysql database and we also saw how to refactor express engine template and use another resource like Swig template library to build a basic website.