










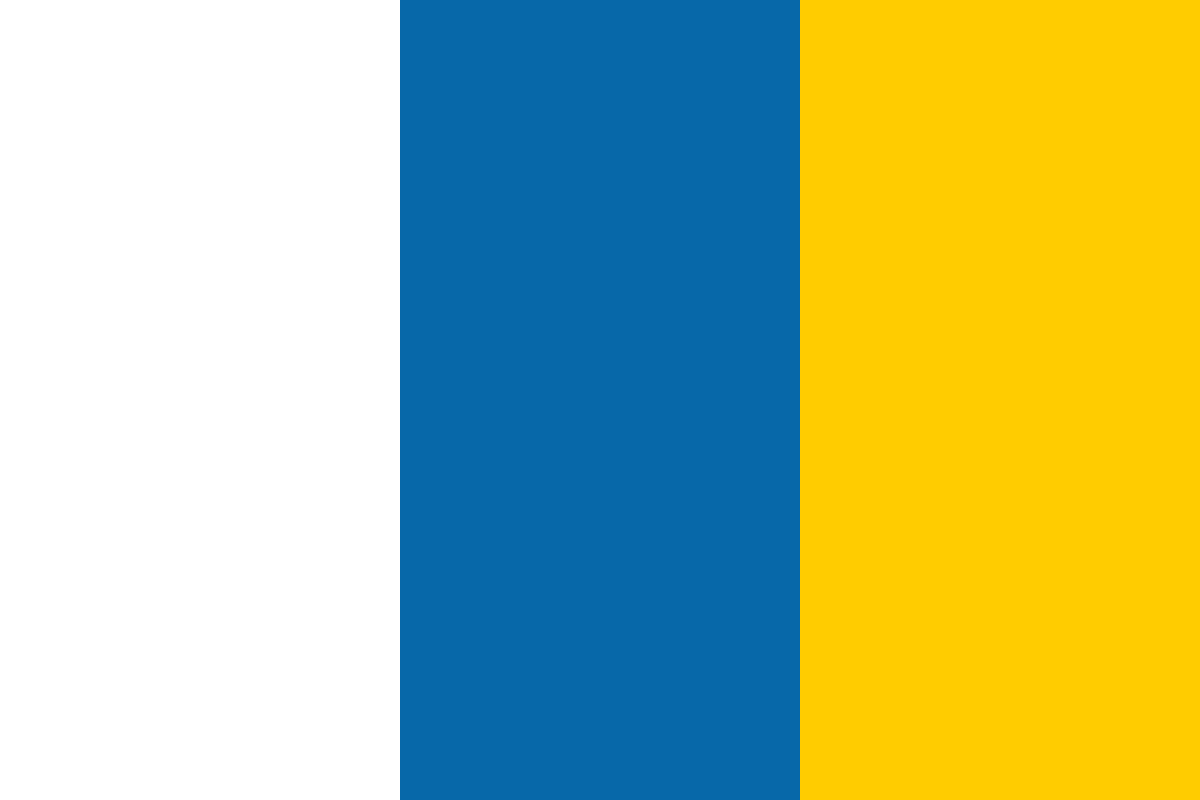

































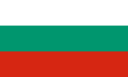








(For more resources related to this topic, see here.)
Animating the background color of an element is a great way to draw our user's eyes to the object we want them to see. Another use for animating the background color of an element is to show that something has happened to the element. It's typically used in this way if the state of the object changes (added, moved, deleted, and so on), or if it requires attention to fix a problem.
Due to the lack of support in jQuery 2.0 for animating background-color, we'll be using jQuery UI to give us the functionality we need to create this effect.
The animate() method is one of the most useful methods jQuery has to offer in its bag of tricks in the animation realm. With it, we’re able to do things like, move an element across the page or alter and animating the properties of colors, backgrounds, text, fonts, the box model, position, display, lists, tables, generated content, and so on.
Following the steps below, we're going to start by creating an example that changes the body background color.
Next, we'll need to include the jQuery UI library by adding this line directly under our jQuery library by adding this line:
<script src = "js/jquery-ui.min.js"></script>
A custom or stable build of jQuery UI can be downloaded from http://jqueryui.com, or you can link to the library using one of the three Content Delivery Networks (CDN) below. For fastest access to the library, go to http://jqueryui.com, scroll to the very bottom and look for the Quick Access section. Using the jQuery UI library JS file there will work just fine for our needs for the examples in this article.
Media Template: http://code.jquery.com
Google: http://developers.google.com/speed/libraries/devguide#jquery-ui
Microsoft: http://asp.net/ajaxlibrary/cdn.ashx#jQuery_Releases_on_the_CDN_0
Then, we'll add the following jQuery code to the anonymous function:
var speed = 1500; $( "body").animate({ backgroundColor: "#D68A85" },speed); $( "body").animate({ backgroundColor: "#E7912D" },speed); $( "body").animate({ backgroundColor: "#CECC33" },speed); $( "body").animate({ backgroundColor: "#6FCD94" },speed); $( "body").animate({ backgroundColor: "#3AB6F1" },speed); $( "body").animate({ backgroundColor: "#8684D8" },speed); $( "body").animate({ backgroundColor: "#DD67AE" },speed);
First we added in the jQuery UI library to our page. This was needed because of the lack of support for animating the background color in the current version of jQuery. Next, we added in the code that will animate our background. We then set the speed variable to 1500 (milliseconds) so that we can control the duration of our animation. Lastly, using the animate() method, we set the background color of the body element and set duration to the variable we set above named speed. We duplicated the same line several times, changing only the hexadecimal value of the background color.
The following screenshot is an illustration of colors the entire body background color animates through:
It's important to note that jQuery methods (animate() in this case) can be chained together. Our code mentioned previously would look like the following if we chained the animate() methods together:
$("body") .animate({ backgroundColor: "#D68A85"}, speed) //red .animate({ backgroundColor: "#E7912D"}, speed) //orange .animate({ backgroundColor: "#CECC33"}, speed) //yellow .animate({ backgroundColor: "#6FCD94"}, speed) //green .animate({ backgroundColor: "#3AB6F1"}, speed) //blue .animate({ backgroundColor: "#8684D8"}, speed) //purple .animate({ backgroundColor: "#DD67AE"}, speed); //pink
Here's another example of chaining methods together:
(selector).animate(properties).animate(properties).animate(properties)
In this example we used the animate() method and with some help from jQuery UI, we were able to animate the body background color of our page. Have a go at extending the script to use a loop, so that the colors continually animate without stopping once the script gets to the end of the function.
Q1. Which code will properly animate our body background color from red to blue using chaining?
$("body") .animate({ background: "red"}, "fast") .animate({ background: "blue"}, "fast");
$("body") .animate({ background-color: "red"}, "slow") .animate({ background-color: "blue"}, "slow");
$("body") .animate({ backgroundColor:"red" }) .animate({ backgroundColor:"blue" });
$("body") .animate({ backgroundColor,"red" }, "slow") .animate({ backgroundColor,"blue" }, "slow");