










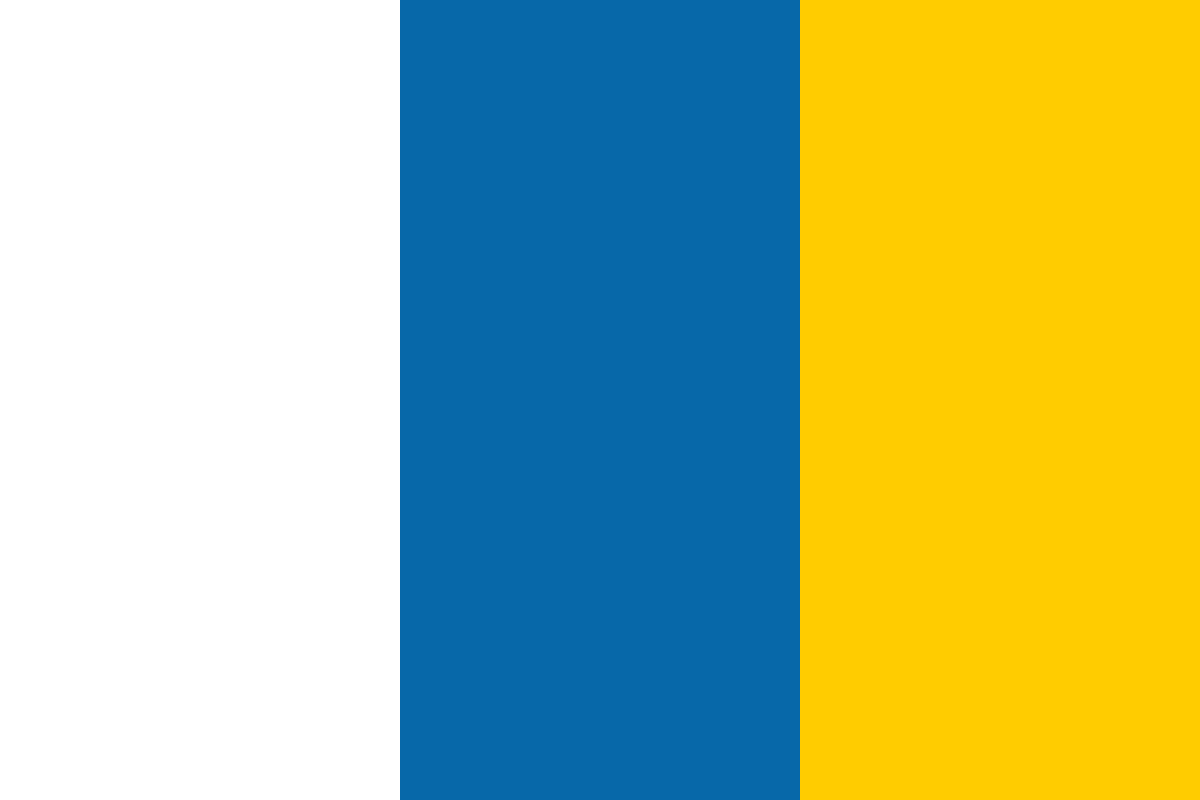

































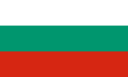








Over 60 practical recipes for integrating jQuery with ASP.NET
The reader can benefit from the previous article on ASP.NET: Creating Rich Content.
The datepicker is a popular control for date fields in online submission forms. In this recipe, let's see how to use the jQuery UI to attach a datepicker to an ASP.NET TextBox control.
<form id="form1" runat="server">
<div align="center">
<asp:Label ID="lblDate" runat="server">Search by
registration date: </asp:Label>
<asp:TextBox ID="txtDate" runat="server"></asp:TextBox>
<asp:Button ID="btnSubmit" Text="Search" runat="server" />
</div>
</form>
Thus, on page load, the web form appears as shown in the following screenshot:
We will now use jQuery UI to attach a datepicker to the TextBox control.
In the document.ready() function of the jQuery script block, apply the datepicker() method to the TextBox control:
$("#txtDate").datepicker();
Thus, the complete jQuery solution for the given problem is as follows:
<script language="javascript" type="text/javascript">
$(document).ready(function(){
$("#txtDate").datepicker();
});
</script>
Run the web form. On mouseclick on the TextBox control, the datepicker is displayed as shown in the following screenshot:
The desired date can be picked from the displayed calendar as required.
For detailed documentation on the jQuery UI datepicker widget, please visit http://jqueryui.com/demos/datepicker/.
jQuery UI provides a Progressbar widget to show the processing status during a wait time in an application. In this recipe, we will learn to create a Progressbar in ASP.NET.
<asp:Panel id="progressbar" runat="server"></asp:Panel>
#progressbar
{
width:300px;
height:22px;
}
.ui-progressbar-value { background-image: url(images/pbar- ani.gif); }
<asp:Panel id="contentArea" runat="server">Page successfully loaded</asp:Panel>
.hide 21
{
display:none;
}
Thus, the complete aspx markup of the form is as follows:
<form id="form1" runat="server">
<div align="center">
<asp:Panel id="progressbar" runat="server"></asp:Panel>
<asp:Panel id="contentArea" runat="server">Page successfully
loaded</asp:Panel>
</div>
</form>
Now, we will look at the jQuery solution for applying the Progressbar widget to the ASP.NET panel.
$("#contentArea").addClass("hide");
var cnt = 0;
var maxCnt = 100;
var id = setInterval(showprogress, 10);
function showprogress() {
if (cnt <= maxCnt) {
$("#progressbar").progressbar({
value: cnt
});
cnt++;
}
lse {
clearInterval(id);
$("#contentArea").removeClass("hide");
$("#progressbar").addClass("hide");
}
}
Thus, the complete jQuery solution is a follows:
<script language="javascript" type="text/javascript">
$(document).ready(function() {
$("#contentArea").addClass("hide");
var cnt = 0;
var maxCnt = 100;
var id = setInterval(showprogress, 10);
function showprogress() {
if (cnt <= maxCnt) {
$("#progressbar").progressbar({
value: cnt
});
cnt++;
}
else {
clearInterval(id);
$("#contentArea").removeClass("hide");
$("#progressbar").addClass("hide");
}
}
});
</script>
In this solution, we have used the JavaScript timer setInterval(customFunction, timeout) to call a custom function after the timeout (in milliseconds). Important points to note are:
Run the web form. You will see that the progressbar loads in steps, as shown in the following screenshot:
After the load is complete, the progressbar is hidden and the content panel is displayed instead, as follows:
For detailed documentation on the jQuery UI progressbar widget, please visit http://jqueryui.com/demos/progressbar/.