










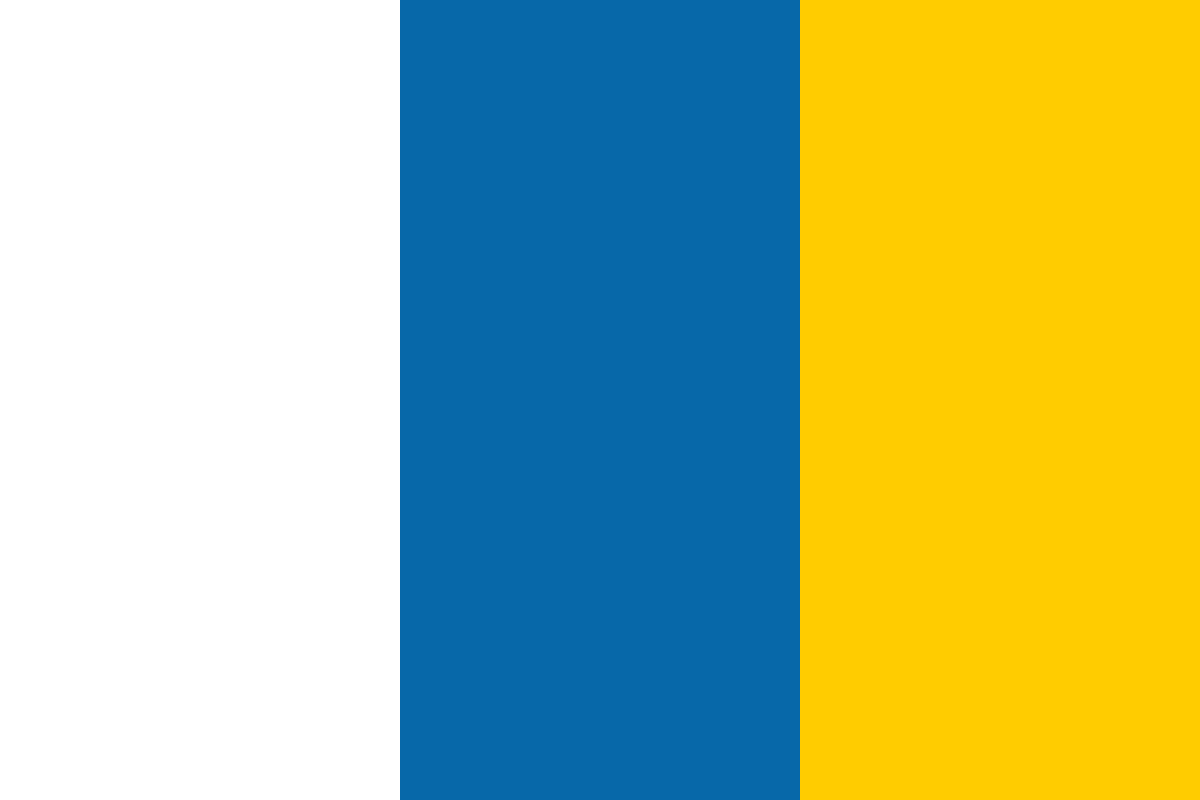

































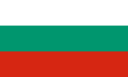








A fast-paced cookbook with recipes covering all that you wanted to know about developing with ASP.NET MVC
ASP.NET MVC provides a simple, but powerful, framework for validating forms. In this article, we'll start by creating a simple form, and then incrementally extend the functionality of our project to include client-side validation, custom validators, and remote validation.
The moment you create an action to consume a form post, you're validating. Or at least the framework is. Whether it is a textbox validating to a DateTime, or checkbox to a Boolean, we can start making assumptions on what should be received and making provisions for what shouldn't. Let's create a form.
public class Person {
[DisplayName("First Name")]
public string FirstName { get; set; }
[DisplayName("Middle Name")]
public string MiddleName { get; set; }
[DisplayName("Last Name")]
public string LastName { get; set; }
[DisplayName("Birth Date")]
public DateTime BirthDate { get; set; }
public string Email { get; set; }
public string Phone { get; set; }
public string Postcode { get; set; }
public string Notes { get; set; }
}
public ActionResult Index() {
return View(new Person());
}
<% using (Html.BeginForm()) {%>
<%: Html.EditorForModel() %>
<input type="submit" name="submit" value="Submit" />
<% } %>
[HttpPost]
public ActionResult Index(...
// Individual Parameters
public ActionResult Index(string firstName, DateTime birthdate...
// Model
Public ActionResult Index(Person person) {
[HttpPost]
public ActionResult Index(FormCollection form) {
var person = new Person();
UpdateModel(person);
return View(person);
}
The UpdateModel technique is a touch more long-winded, but comes with advantages. The first is that if you add a breakpoint on the UpdateModel line, you can see the exact point when an empty model becomes populated with the form collection, which is great for demonstration purposes.
The main reason I go back to UpdateModel time and time again, is the optional second parameter, includeProperties. This parameter allows you to selectively update the model, thereby bypassing validation on certain properties that you might want to handle independently.
With ASP.NET MVC, it sometimes feels like you're stripping the development process back to basics. I think this is a good thing; more control to render the page you want is good. But there is a lot of clever stuff going on in the background, starting off with Model Binders.
When you send a request (GET, POST, and so on) to an ASP.NET MVC application, the query string, route values and the form collection are passed through model binding classes, which result in usable structures (for example, your action's input parameters). These model binders can be overridden and extended to deal with more complex scenarios, but since ASP.NET MVC2, I've rarely made use of this. A good starting point for further investigation would be with DefaultModelBinder and IModelBinder.
What about that validation message in the last screenshot, where did it come from? Apart from LableFor and EditorFor, but we also have ValidationMessageFor. If the model binders fail at any point to build our input parameters, the model binder will add an error message to the model state. The model state is picked up and displayed by the ValidationMessageFor method, but more on that later.