










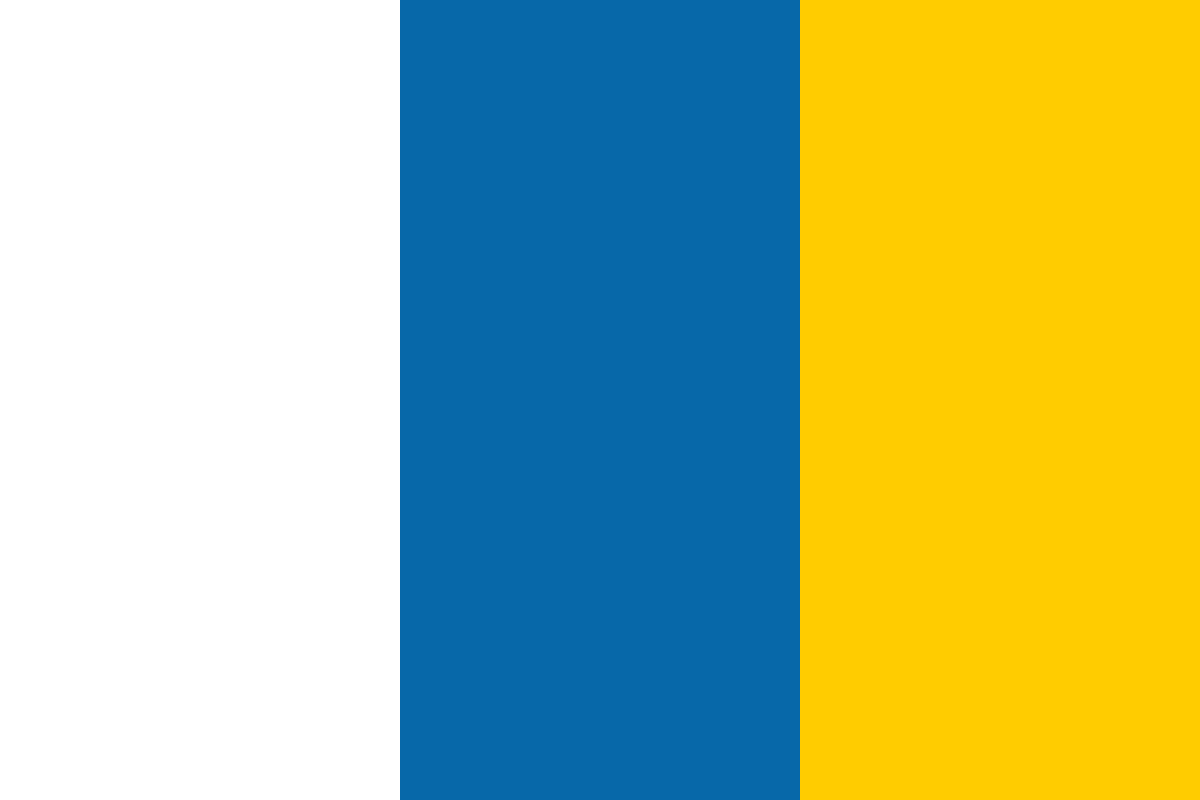

































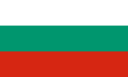








Our first module is going to fetch XML data from Goodreads, a free social networking site for avid readers. There, users track the books they are reading and have read, rate books and write reviews, and share their reading lists with friends.
Reading lists at Goodreads are stored in bookshelves. These bookshelves are accessible over a web-based XML/RSS API. We will use that API to display a reading list on the Philosopher Bios website(example website)
To integrate the Goodreads information in Drupal, we will create a small module. Since this is our first module, we will get into greater details.
In Drupal, every module is contained in its own directory. This simplifies organization; all of the module's files are located in one place.
To keep naming consistent throughout the module (a standard in Drupal), we will name our directory with the module name. Later, we will install this module in Drupal, but for development, the module directory can be wherever it is most convenient.
Once we have created a directory named goodreads, we can start creating files for our module. The first file we need to create is the .info (dot-info) file.
Before we start coding our new module, we need to create a simple text file that will hold some basic information about our module. Various Drupal components use the information in this file for module management.
The .info file is written as a PHP INI file, which is a simple configuration file format.
If you are interested in the details of INI file processing, you can visit http://php.net/manual/en/function.parse-ini-file.php for a description of this format and how it can be parsed in PHP.
Our .info file will only be five lines long, which is probably about average.
The .info file must follow the standard naming conventions for modules. It must be named <modulename>.info, where <modulename> is the same as the directory name. Our file, then, will be called goodreads.info.
Following are the contents of goodreads.info:
;$Id$
name = "Goodreads Bookshelf"
description = "Displays items from a Goodreads Bookshelf"
core = 6.x
php = 5.1
This file isn't particularly daunting. The first line of the file is, at first glance, the most cryptic. However, its function is mundane: it is a placeholder for Drupal's CVS server.
Drupal, along with its modules, is maintained on a central CVS (Concurrent Version System) server. CVS is a version control system. It tracks revisions to code over time. One of its features is its ability to dynamically insert version information into a file. However, it needs to know where to insert the information. The placeholder for this is the special string $Id$. But since this string isn't actually a directive in the .info file, it is commented out with the PHP INI comment character, ; (semi-colon).
You can insert comments anywhere in your .info file by beginning a line with the ; character.
The next four directives each provide module information to Drupal.
The name directive provides a human-readable display name for the module. Here's an example:
In this above screenshot, the names Aggregator and Blog are taken from the values of the name directives in these modules' .info files.
While making the module's proper name short and concise is good (as we did when naming the module directory goodreads above), the display name should be helpful to the user. That usually means that it should be a little longer, and a little more descriptive.
However, there is no need to jam all of the module information into the name directive. The description directive is a good place for providing a sentence or two describing the module's function and capabilities.
The third directive is the core directive.
The core and php directives are new in Drupal 6.
This directive specifies what version of Drupal is required for this module to function properly. Our value, 6.x, indicates that this module will run on Drupal 6 (including its minor revisions). In many cases, the Drupal packager will be able to automatically set this (correctly). But Drupal developers are suggesting that this directive be set manually for those who work from CVS.
Finally, the php directive makes it possible to specify a minimum version number requirement for PHP. PHP 5, for example, has many features that are missing in PHP 4 (and the modules in this book make use of such features). For that reason, we explicitly note that our modules require at least PHP version 5.1.
That's all there is to our first module .info file. What we have here is sufficient for our Goodreads module.
Now, we are ready to write some PHP code.
There are two files that every module must have (though many modules have more). The first, the .info file, we examined above. The second file is the .module (dot-module) file, which is a PHP script file. This file typically implements a handful of hook functions that Drupal will call at pre-determined times during a request.
Here, we will create a .module file that will display a small formatted section of information. Later in this article, we will configure Drupal to display this information to site visitors.
For our very first module, we will implement the hook_block() function. In Drupal parlance, a block is a chunk of auxiliary information that is displayed on a page alongside the main page content. Sounds confusing? An example might help.
Think of your favorite news website. On a typical article page, the text of the article is displayed in the middle of the page. But on the left and right sides of the page and perhaps at the top and bottom as well, there are other bits of information: a site menu, a list of links to related articles, links to comments or forums about this article, etc. In Drupal, these extra pieces are treated as blocks.
The hook_block() function isn't just for displaying block contents, though. In fact, this function is responsible for displaying the block and providing all the administration and auxiliary functions related to this block. Don't worry... we'll start out simply and build up from there.
Drupal follows rigorous coding and documentation standards (http://drupal.org/coding-standards). In this article, we will do our best to follow these standards. So as we start out our module, the first thing we are going to do is provide some API documentation.
Just as with the .info file, the .module file should be named after the module. Following is the beginning of our goodreads.module file:
<?php
// $Id$
/**
* @file
* Module for fetching data from Goodreads.com.
* This module provides block content retrieved from a
* Goodreads.com bookshelf.
* @seehttp://www.goodreads.com
*/
The .module file is just a standard PHP file. So the first line is the opening of the PHP processing instruction: <?php. Throughout this article you may notice something. While all of our PHP libraries begin with the <?php opening, none of them end with the closing ?> characters.
This is intentional, in fact, it is not just intentional, but conventional for Drupal. As much as it might offend your well-formed markup language sensibilities, it is good coding practice to omit the closing characters for a library.
Why? Because it avoids printing whitespace characters in the script's output, and that can be very important in some cases. For example, if whitespace characters are output before HTTP headers are sent, the client will see ugly error messages at the top of the page.
After the PHP tag is the keyword for the version control system:
// $Id$
When the module is checked into the Drupal CVS, information about the current revision is placed here.
The third part of this example is the API documentation. API documentation is contained in a special comment block, which begins /** and ends with a */. Everything between these is treated as documentation. Special extraction programs like Doxygen can pull out this information and create user-friendly programming information.
The Drupal API reference is generated from the API comments located in Drupal's source code. The program, Doxygen, (http://www.stack.nl/~dimitri/doxygen/) is used to generate the API documents from the comments in the code.
The majority of the content in these documentation blocks (docblocks, for short) is simply text. But there are a few additions to the text.
First, there are special identifiers that provide the documentation generating program with additional information. These are typically prefixed with an @ sign.
/**
* @file
* Module for fetching data from Goodreads.com.
* This module provides block content retrieved from a
* Goodreads.com bookshelf.
* @see http://www.goodreads.com
*/
In the above example, there are two such identifiers. The @file identifier tells the documentation processor that this comment describes the entire file, not a particular function or variable inside the file. The first comment in every Drupal PHP file should, by convention, be a file-level comment.
The other identifier in the above example is the @see keyword. This instructs the documentation processor to attempt to link this file to some other piece of information. In this case, that piece of information is a URL. Functions, constants, and variables can also be referents of a @see identifier. In these cases, the documentation processor will link this docblock to the API information for that function, constant, or variable.
With these formalities out of the way, we're ready to start coding our module.